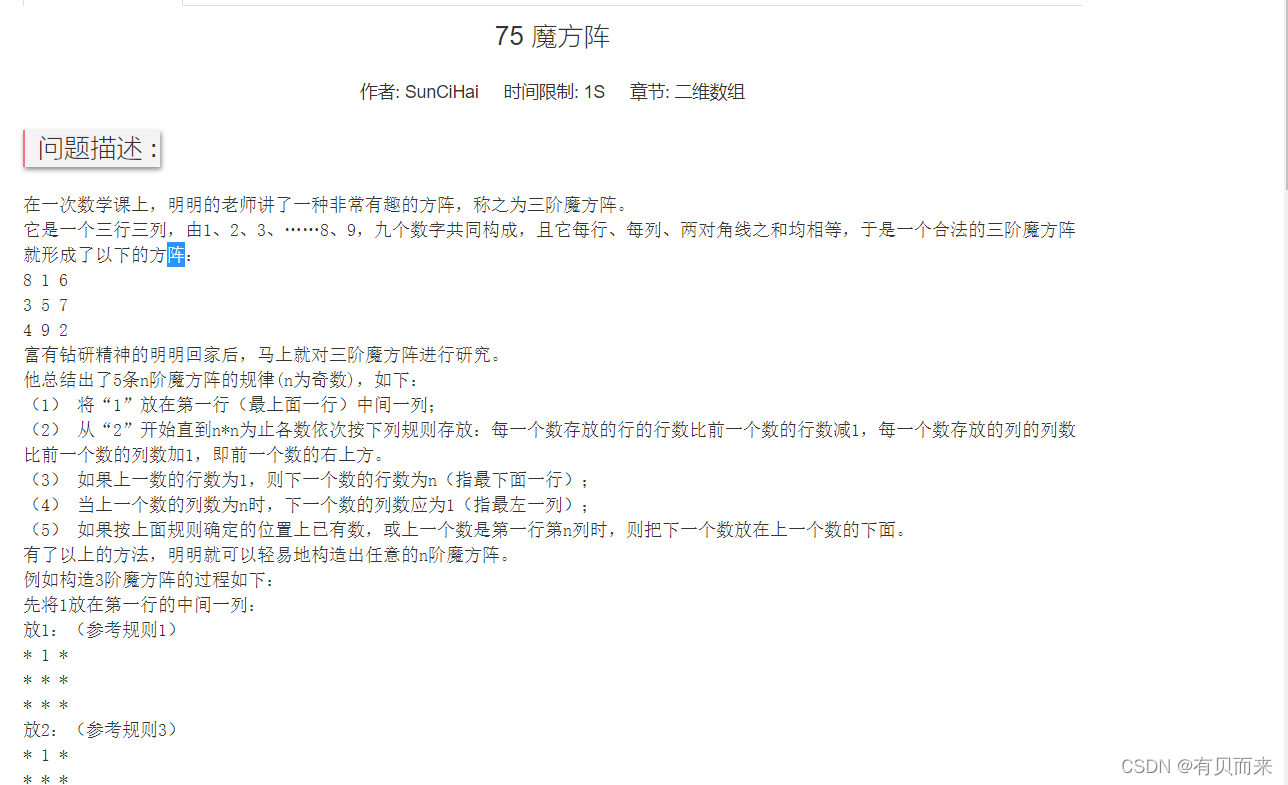
#include<bits/stdc++.h>
#include<bitset>
#include<unordered_map>
#define pb push_back
#define bp __builtin_popcount
#define TIME cout << "RuningTime: " << clock() << "ms\n", 0
#define ls x<<1
#define rs x<<1|1
using namespace std;
typedef long long ll;
const int inf=0x3f3f3f3f;
const int maxn=10+10;
const int MOD=9901;
const int mod = 1e9+7;
const double PI=acos(-1);
int lowbit(int x){return x&-x;}
ll gcd(ll x, ll y){ return y == 0 ? x : gcd(y, x%y); }
ll lcm(ll x, ll y){ return x / gcd(x, y)*y; }
inline ll dpow(ll a, ll b){ ll r = 1, t = a; while (b){ if (b & 1)r = (r*t) % MOD; b >>= 1; t = (t*t) % MOD; }return r; }
inline ll fpow(ll a, ll b){ ll r = 1, t = a; while (b){ if (b & 1)r = (r*t); b >>= 1; t = (t*t); }return r; }
priority_queue<int, vector<int>, greater<int> > q;
int a[maxn][maxn],n,m,vis[maxn][maxn];
void dfs(int x,int y,int now)
{
if(now==n*n+1)return ;
if(vis[x][y])
{
dfs(x+2,y-1,now);
}
else if(x==1&&y==n)
{
a[x][y]=now;
vis[x][y]=1;
dfs(x+1,y,now+1);
}
else{
a[x][y]=now;
vis[x][y]=1;
int dx=x-1;
if(dx==0)dx=n;
int dy=y+1;
if(dy==n+1)dy=1;
dfs(dx,dy,now+1);
}
}
int main()
{
while(cin>>n)
{
memset(a,0,sizeof a);
memset(vis,0,sizeof vis);
dfs(1,n/2+1,1);
for(int i=1;i<=n;i++)
{
for(int j=1;j<=n;j++)
{
if(j!=n)
cout<<a[i][j]<<" ";
else cout<<a[i][j]<<endl;
}
}
cout<<endl;
}
return 0;
}