php
hash
$redis = new Redis();
$redisKey = "car:user:".$uid; // 可视化工具中是car文件夹下user文件夹
// 查看key是否存在
$Exists = $redis->Exists($redisKey);
// 查询的数组放入HASH中 ($res is a Array)
$rs = $redis->hmset($redisKey ,$res);
// 设置超时时间
$rs = $redis->expire($redisKey);
//HASH中取出数组
$rs = $redis->hgetall($redisKey);
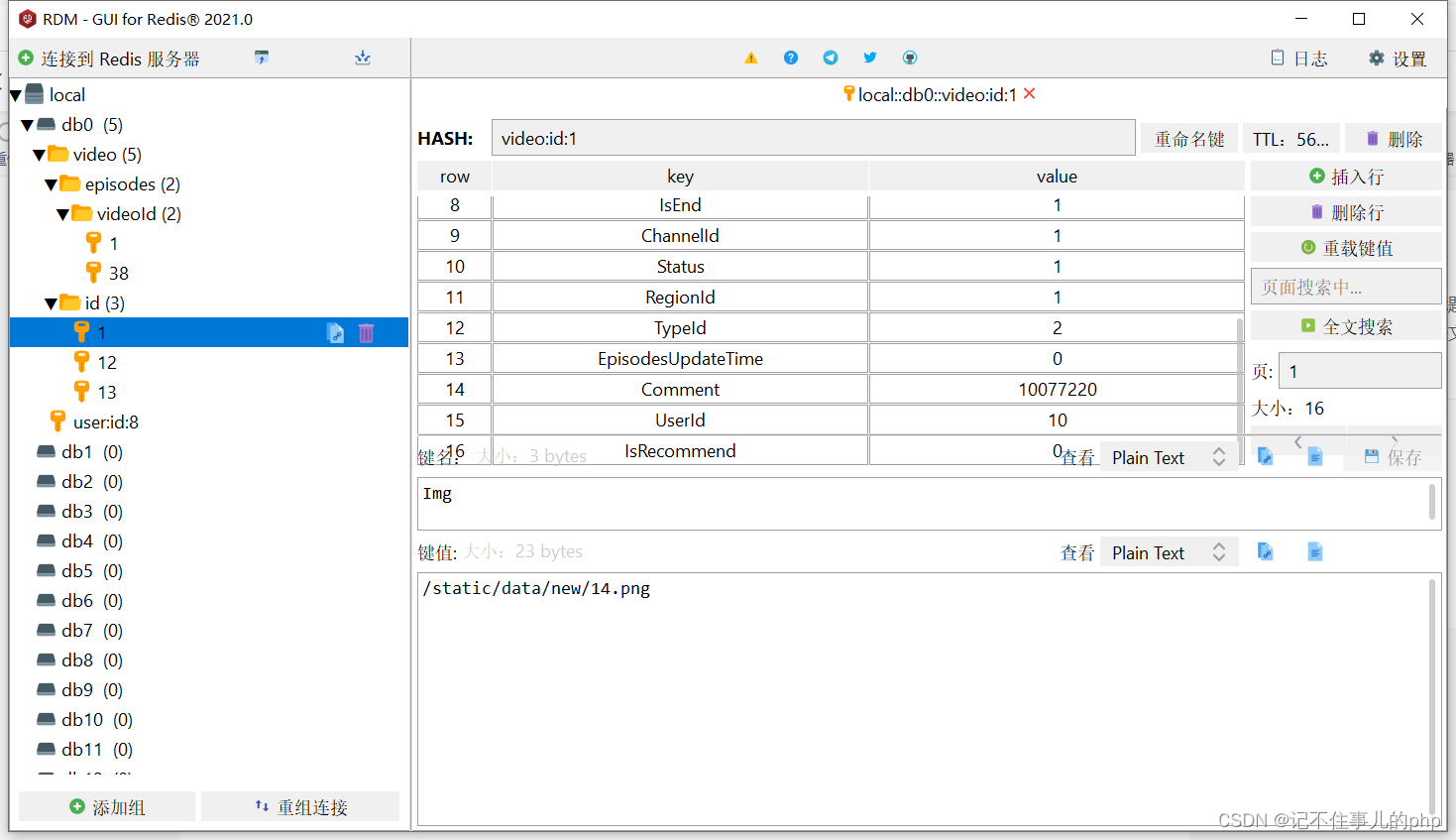
list
$redis = new Redis();
$video_id = input("videoId",0,"intval");
$redisKey = "video:episodes:videoId:".$video_id;
$exists = $redis->exists($redisKey);
if ($exists){
$len = $redis->llen($redisKey);
$data = $redis->lrange($redisKey,0,$len);
$episodes= [];
foreach ($data as $v){
$episodes[] = json_decode($v);
}
}else{
$episodes = Db::table("video_episodes")->where("video_id",$video_id)->order("num asc")->select();
// 存入redis
if ($episodes){
foreach ($episodes as $v){
$redis->lpush($redisKey ,json_encode($v));
}
$redis->expire($redisKey ,86400);
}
}
dump($episodes);
die;
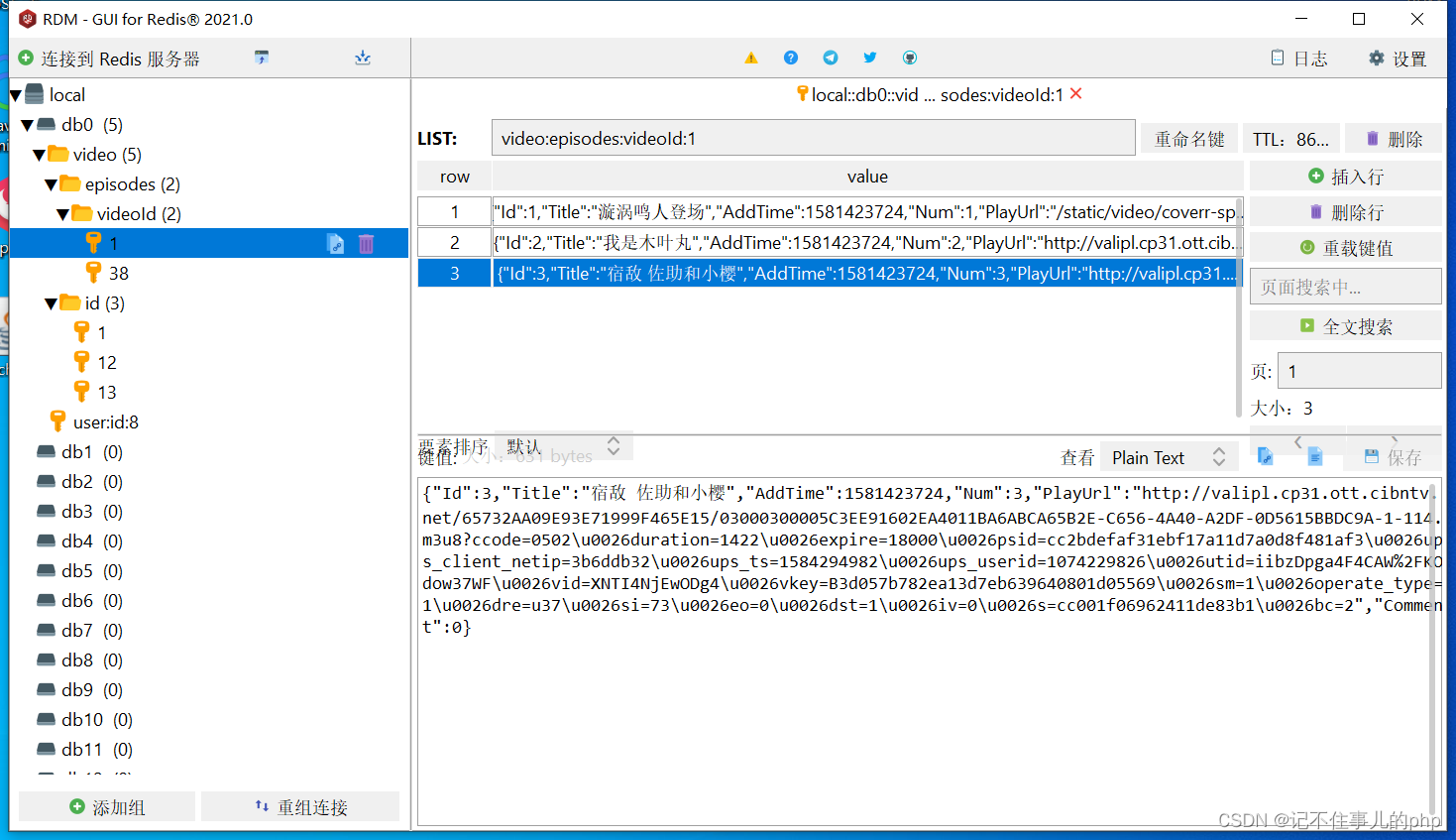
Zset
$redis = new Redis();
$channelId = input("channelId",0,"intval");
$redisKey = "video:top:channel:channelId:".$channelId;
$exists = $redis->exists($redisKey);
if (!$exists){
$videos_id = $redis->zrevrange($redisKey,0,10);
foreach ($videos_id as $v){
$videos[] = (new model())->RedisGetVideoInfo($v); // 之前的视频详情
}
}else{}
$videos = Db::table("video")->where("status",1)->where("channel_id",$channelId)->order("comment desc")->limit(10)->select();
foreach ($videos as $video){
$redis->zadd($redisKey,$video["comment"],$video["id"]);
}
$redis->expire($redisKey,86400);
// }
dump($videos);
die;
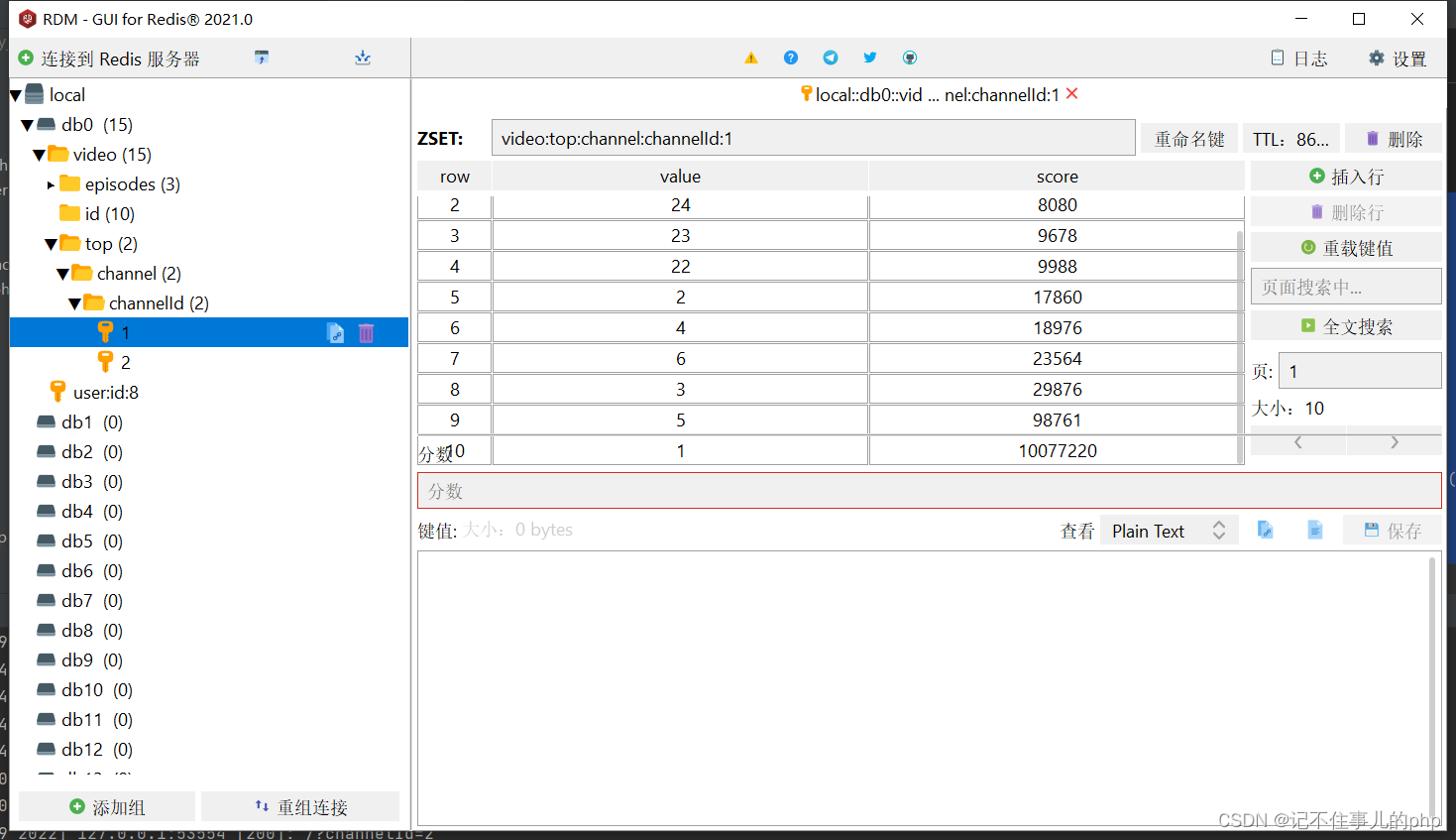
redigo
hash
type VideoData struct {
Id int
Title string
SubTitle string
AddTime int64
Img string
Img1 string
EpisodesCount int
IsEnd int
Comment int
}
// redis缓存-获取视频详情
func RedisGetVideoInfo(videoId int) (Video, error) {
var video Video
conn := redisClient.PoolConnect()
defer conn.Close()
redisKey := "video:id:" + strconv.Itoa(videoId)
// 判断redis是否存在
exists, err := redis.Bool(conn.Do("exists", redisKey))
if exists {
// redis读取
res, _ := redis.Values(conn.Do("hgetall", redisKey))
err = redis.ScanStruct(res, &video)
} else {
// 数据库读取
o := orm.NewOrm()
err := o.Raw("select * from video where id=? limit 1", videoId).QueryRow(&video)
if err == nil {
//保存redis
_, err := conn.Do("hmset", redis.Args{redisKey}.AddFlat(video)...) //redigo 提供的函数转化struct存到redis中
if err == nil {
// 设置过期时间 1 day
conn.Do("expire", redisKey, 86400)
}
}
}
return video, err
}
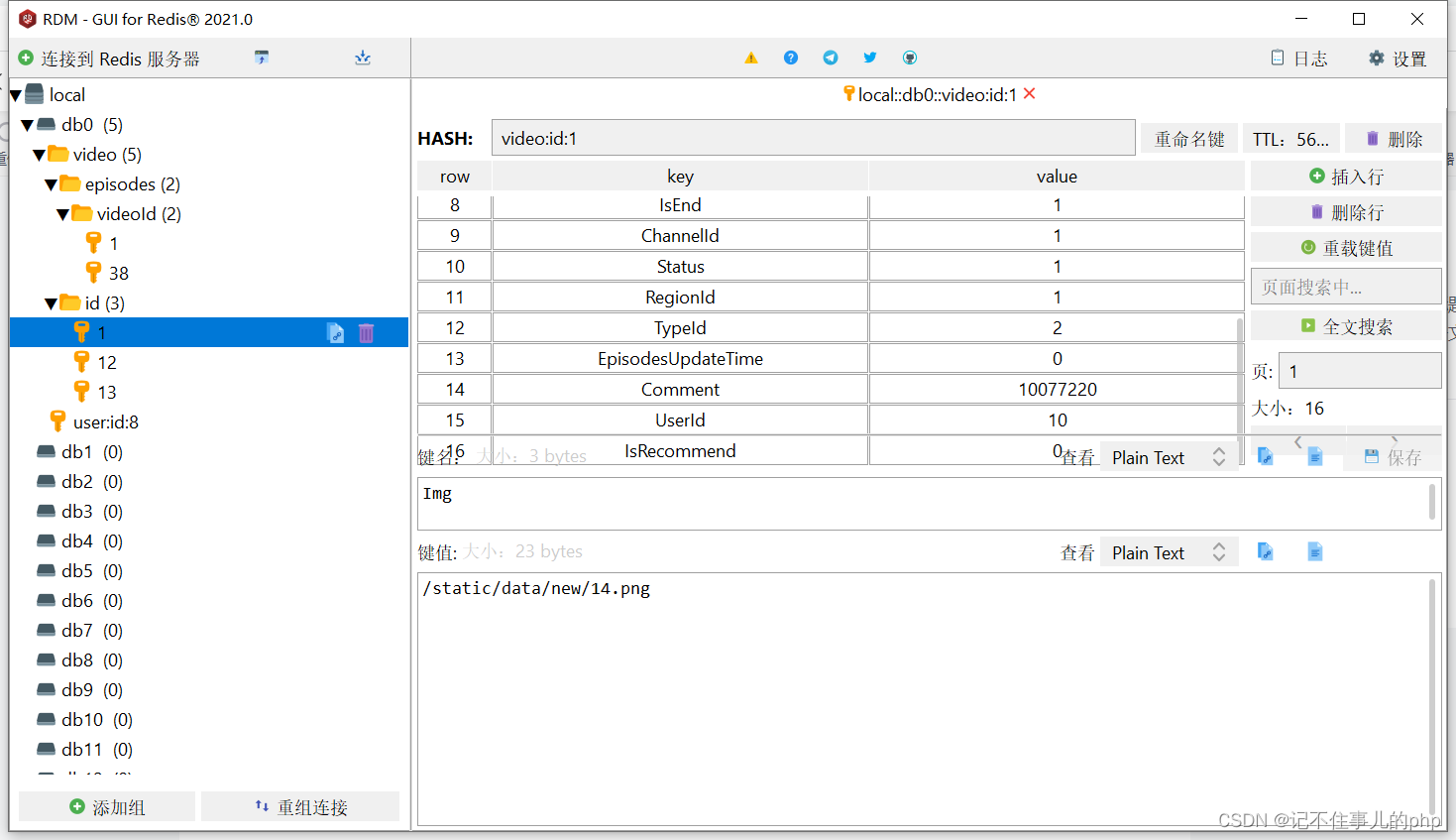
list
type Episodes struct {
Id int
Title string
AddTime int64
Num int
PlayUrl string
Comment int
}
// 获取视频剧集
func GetVideoEpisodes(videoId int) (int64, []Episodes, error) {
o := orm.NewOrm()
var episodes []Episodes
num, err := o.Raw("select id,title,add_time,num,play_url,comment from video_episodes where video_id=? and status=1 order by num asc", videoId).QueryRows(&episodes)
return num, episodes, err
}
// redis获取视频剧集
func RedisGetVideoEpisodes(videoId int) (int64, []Episodes, error) {
var (
episodes []Episodes
err error
num int64
)
conn := redisClient.PoolConnect()
defer conn.Close()
redisKey := "video:episodes:videoId:" + strconv.Itoa(videoId)
exists, err := redis.Bool(conn.Do("exists", redisKey))
if exists {
num, err := redis.Int64(conn.Do("llen", redisKey))
if err == nil {
values, _ := redis.Values(conn.Do("lrange", redisKey, "0", num))
var episodesInfo Episodes
for _, v := range values {
err = json.Unmarshal(v.([]byte), &episodesInfo)
if err == nil {
episodes = append(episodes, episodesInfo)
}
}
}
} else {
o := orm.NewOrm()
num, err = o.Raw("select id,title,add_time,num,play_url,comment from video_episodes where video_id=? and status=1 order by num asc", videoId).QueryRows(&episodes)
if err == nil {
// 遍历获得的数据 , json后保存
for _, v := range episodes {
jsonValue, err := json.Marshal(v)
if err == nil {
_, err = conn.Do("rpush", redisKey, jsonValue)
}
}
conn.Do("expire", redisKey, 86400)
}
}
return num, episodes, err
}
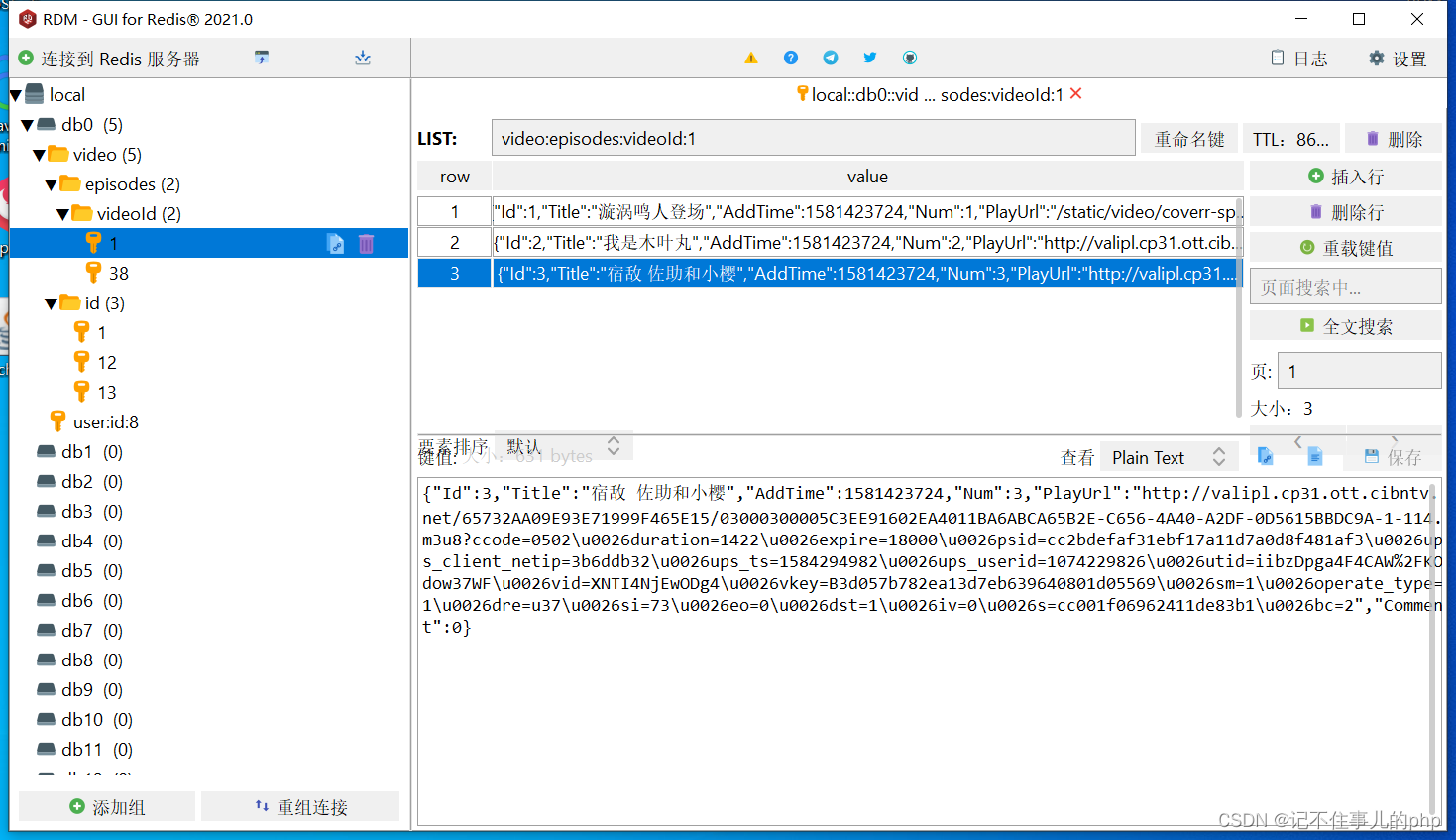
Zset
// 获取频道排行榜
func GetChannelTop(channelId int) (int64, []VideoData, error) {
o := orm.NewOrm()
var videos []VideoData
num, err := o.Raw("select id,title,sub_title,img,img1,add_time,episodes_count,is_end from video where status=1 and channel_id=? order by comment desc limit 10", channelId).QueryRows(&videos)
return num, videos, err
}
// 获取频道排行榜
func RedisGetChannelTop(channelId int) (int64, []VideoData, error) {
var (
videos []VideoData
num int64
)
conn := redisClient.PoolConnect()
defer conn.Close()
redisKey := "video:top:channel:channelId:" + strconv.Itoa(channelId)
exists, err := redis.Bool(conn.Do("exists", redisKey))
if exists {
num = 0
res, _ := redis.Values(conn.Do("zrevrange", redisKey, "0", "10", "WITHSCORES"))
fmt.Println(res)
for k, v := range res {
if k%2 == 0 {
videoId, err := strconv.Atoi(string(v.([]byte)))
fmt.Println(videoId)
videoInfo, err := RedisGetVideoInfo(videoId)
if err == nil {
var videoDataInfo VideoData
videoDataInfo.Id = videoInfo.Id
videoDataInfo.Img = videoInfo.Img
videoDataInfo.Img1 = videoInfo.Img1
videoDataInfo.IsEnd = videoInfo.IsEnd
videoDataInfo.SubTitle = videoInfo.SubTitle
videoDataInfo.Title = videoInfo.Title
videoDataInfo.AddTime = videoInfo.AddTime
videoDataInfo.Comment = videoInfo.Comment
videoDataInfo.EpisodesCount = videoInfo.EpisodesCount
videos = append(videos, videoDataInfo)
num++
}
}
}
} else {
o := orm.NewOrm()
num, err = o.Raw("select id,title,sub_title,img,img1,add_time,episodes_count,is_end from video where status=1 and channel_id=? order by comment desc limit 10", channelId).QueryRows(&videos)
if err == nil {
// 保存redis zset
for _, v := range videos {
conn.Do("zadd", redisKey, v.Comment, v.Id)
//conn.Do("zincrby", redisKey, score, value) // 加分操作
}
conn.Do("expire", redisKey, 86400*30)
}
}
return num, videos, err
}
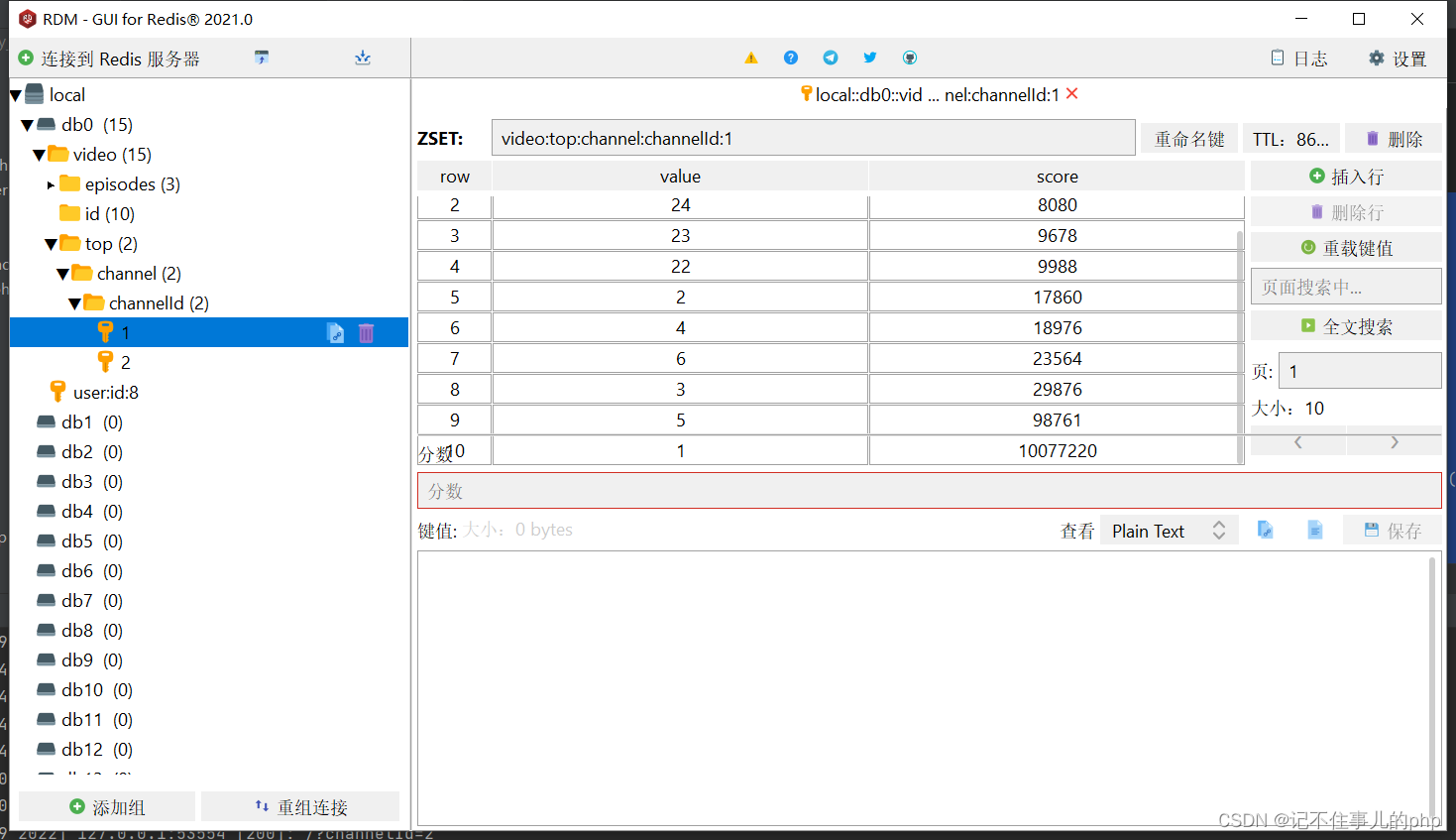