MyBatis-Plus中的Mapper用法
- MySQL和MyBatis-Plus包
- SpringBoot配置信息
- @Mapper注解
- BaseMapper< T >接口
1、准备数据库与表
CREATE DATABASE `mybatis_plus`
USE `mybatis_plus`;
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`id` BIGINT NOT NULL AUTO_INCREMENT COMMENT '主键ID',
`user_name` VARCHAR(30) CHARACTER SET utf8mb3 COLLATE utf8_general_ci DEFAULT NULL COMMENT '姓名',
`age` INT DEFAULT NULL COMMENT '年龄',
`email` VARCHAR(50) DEFAULT NULL COMMENT '邮箱',
`sex` VARCHAR(10) DEFAULT NULL,
PRIMARY KEY (`id`)
);
INSERT INTO `user`(`id`,`user_name`,`age`,`email`,`sex`) VALUES
(1,'李四',21,'list@qq.com','男'),
(2,'Billie',24,'test5@qq.com','男'),
(3,'HeXi',20,'test6@qq.com','男'),
(4,'馨馨',21,'xinxin@qq.com','女'),
(5,'小红',22,'xiaohong@qq.com','女'),
(6,'兮兮',20,'xixi@qq.com','女');
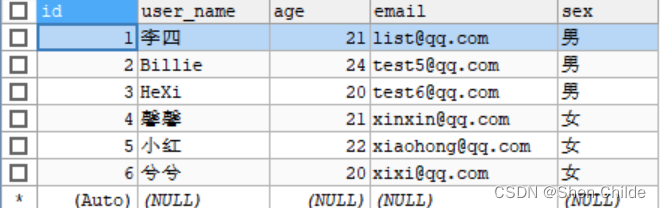
2、创建SpringBoot项目SQL(MySQL Driver)
3、pom.xml导入jar包配置刷新Maven
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
4、配置application.yml
# 连接数据库信息
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/mybatis_plus?serverTimezone=UTC
username: root
password: 111111
5、启动类
package com.sgz.mybatisplus;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Day66MybatisplusMapperApplication {
public static void main(String[] args) {
SpringApplication.run(Day66MybatisplusMapperApplication.class, args);
}
}
6、创建pojo类
package com.sgz.mybatisplus.pojo;
public class User {
private Integer id;
private String userName;
private Integer age;
private String email;
private String sex;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", userName='" + userName + '\'' +
", age=" + age +
", email='" + email + '\'' +
", sex='" + sex + '\'' +
'}';
}
}
7、mapper接口继承BaseMapper< T >
package com.sgz.mybatisplus.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.sgz.mybatisplus.pojo.User;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
8、测试类
package com.sgz.mybatisplus;
import com.sgz.mybatisplus.mapper.UserMapper;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
class Day66MybatisplusMapperApplicationTests {
@Autowired
private UserMapper userMapper;
@Test
void contextLoads() {
System.out.println(userMapper.selectList(null));
}
}