我们致力于探索、分享和推荐最新的实用技术栈、开源项目、框架和实用工具。每天都有新鲜的开源资讯等待你的发现!
项目介绍
DotNetExercises是一个开源,免费的针对C#/.NET/.NET Core编程技巧的综合性练习库。
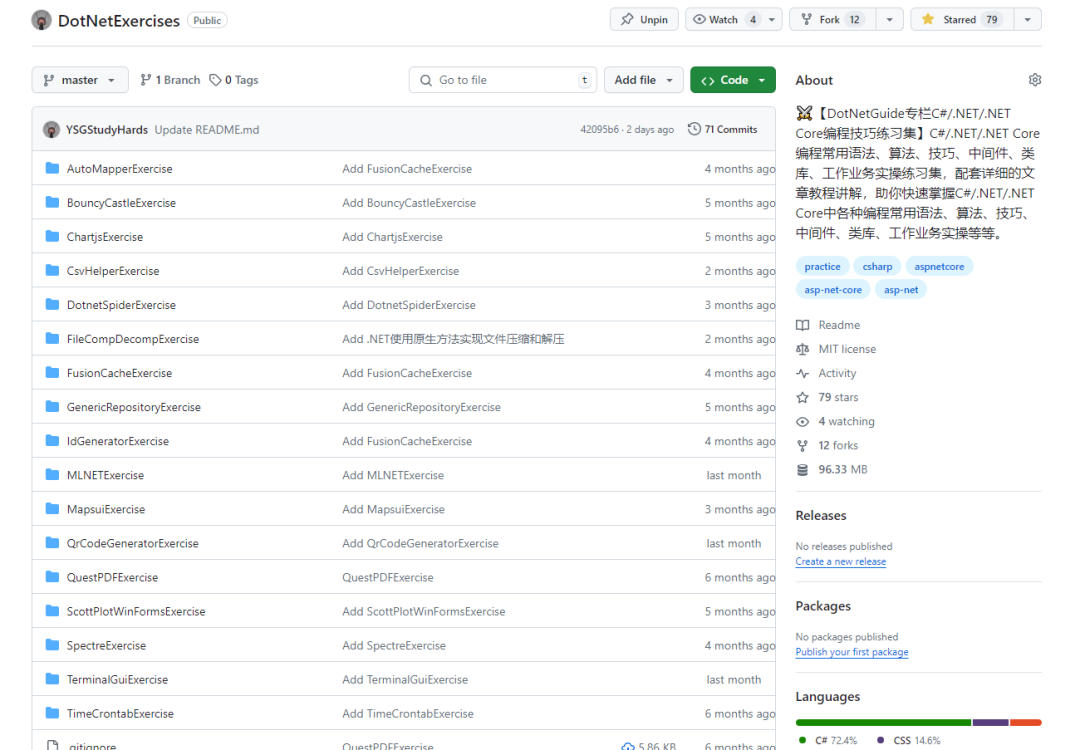
开源地址
https://github.com/YSGStudyHards/DotNetExercises
内容概览
常用语法练习:包含C#/.NET/.NET Core编程中常用的语法练习,帮助开发者巩固基础。
算法与技巧:提供算法练习和编程技巧,提升开发者的编程能力和问题解决能力。
中间件与类库:介绍并实践常用的中间件和类库,帮助开发者在实际项目中灵活运用。
工作业务实操:通过模拟实际工作场景,提供业务实操练习,增强开发者的实战能力。
文章详细教程
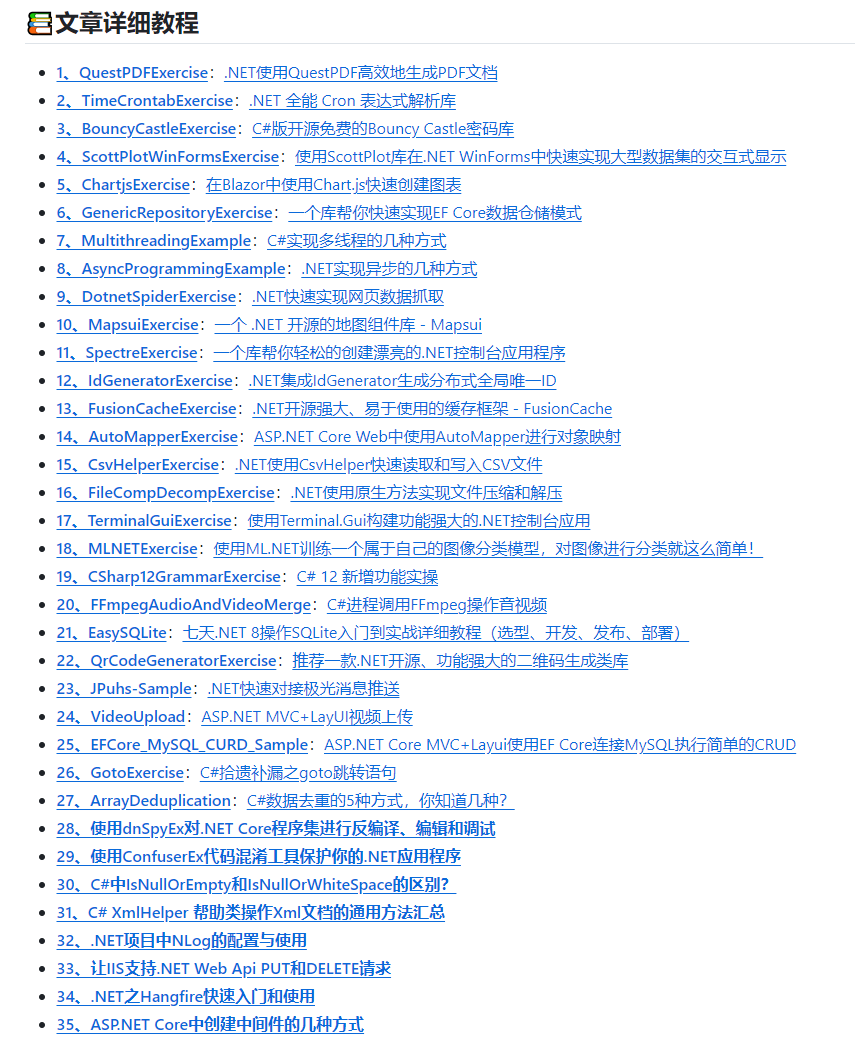
部分源代码
C#拾遗补漏之goto跳转语句
namespace HelloDotNetGuide.CSharp语法
{
public class GotoExercise
{
/// <summary>
/// 使用goto进行代码重试示例
/// </summary>
public static void GotoRetryUseExample()
{
int retryCount = 0;
for (int i = 0; i < 10; i++)
{
retryLogic:
try
{
//模拟可能出错的操作
Random random = new Random();
int result = random.Next(0, 2);
if (result == 0)
{
throw new Exception("Error occurred");
}
Console.WriteLine("Operation successful on attempt: " + retryCount);
}
catch (Exception ex)
{
retryCount++;
if (retryCount < 3)
{
Console.WriteLine("Error occurred, retrying...");
goto retryLogic; //跳转到重试逻辑
}
else
{
Console.WriteLine("Max retry limit reached.");
return;
}
}
}
}
/// <summary>
/// 不使用goto进行代码重试示例
/// </summary>
public static void NonGotoRetryUseExample()
{
int retryCount = 0;
for (int i = 0; i < 10; i++)
{
while (retryCount < 3)
{
try
{
//模拟可能出错的操作
Random random = new Random();
int result = random.Next(0, 2);
if (result == 0)
{
throw new Exception("Error occurred");
}
Console.WriteLine("Operation successful on attempt: " + retryCount);
break;
}
catch (Exception ex)
{
retryCount++;
Console.WriteLine("Error occurred, retrying...");
}
}
if (retryCount == 3)
{
Console.WriteLine("Max retry limit reached.");
return;
}
}
}
/// <summary>
/// goto正常输出使用示例
/// </summary>
public static void GotoGeneralUseExample(int num)
{
if (num < 0)
{
goto LessThanZero;
}
else if (num == 0)
{
goto EqualToZero;
}
else
{
goto GreaterThanZero;
}
LessThanZero:
Console.WriteLine("数字小于零");
goto End;
EqualToZero:
Console.WriteLine("数字等于零");
goto End;
GreaterThanZero:
Console.WriteLine("数字大于零");
goto End;
End:
Console.WriteLine("End...");
}
/// <summary>
/// 不使用goto正常输出使用示例
/// </summary>
public static void NonGotoGeneralUseExample(int num)
{
if (num < 0)
{
Console.WriteLine("数字小于零");
}
else if (num == 0)
{
Console.WriteLine("数字等于零");
}
else
{
Console.WriteLine("数字大于零");
}
Console.WriteLine("End...");
}
}
}
.NET集成IdGenerator生成分布式全局唯一ID
using Yitter.IdGenerator;
namespace IdGeneratorExercise
{
internal class Program
{
static void Main(string[] args)
{
#region 第一步:全局初始化(应用程序启动时执行一次)
// 创建 IdGeneratorOptions 对象,可在构造函数中输入 WorkerId:
// options.WorkerIdBitLength = 10; // 默认值6,限定 WorkerId 最大值为2^6-1,即默认最多支持64个节点。
// options.SeqBitLength = 6; // 默认值6,限制每毫秒生成的ID个数。若生成速度超过5万个/秒,建议加大 SeqBitLength 到 10。
// options.BaseTime = Your_Base_Time; // 如果要兼容老系统的雪花算法,此处应设置为老系统的BaseTime。
// WorkerId:WorkerId,机器码,最重要参数,无默认值,必须 全局唯一(或相同 DataCenterId 内唯一),必须 程序设定,缺省条件(WorkerIdBitLength取默认值)时最大值63,理论最大值 2^WorkerIdBitLength-1(不同实现语言可能会限定在 65535 或 32767,原理同 WorkerIdBitLength 规则)。不同机器或不同应用实例 不能相同,你可通过应用程序配置该值,也可通过调用外部服务获取值。
// ...... 其它参数参考 IdGeneratorOptions 定义。
var idGeneratorOptions = new IdGeneratorOptions(1) { WorkerIdBitLength = 6 };
// 保存参数(务必调用,否则参数设置不生效):
YitIdHelper.SetIdGenerator(idGeneratorOptions);
// 以上过程只需全局一次,且应在生成ID之前完成。
#endregion
#region 第二步:生成分布式ID
for (int i = 0; i < 1000; i++)
{
// 初始化后,在任何需要生成ID的地方,调用以下方法:
var newId = YitIdHelper.NextId();
Console.WriteLine($"Number{i},{newId}");
}
#endregion
}
}
}