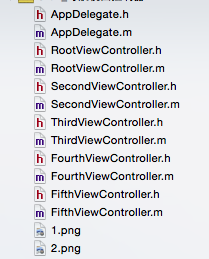
AppDelegate.m
#import "AppDelegate.h"
#import "RootViewController.h"
@interface AppDelegate ()
@end
@implementation AppDelegate
- (void)dealloc
{
[_window release];
[super dealloc];
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
self.window.backgroundColor = [UIColor whiteColor];
[self.window makeKeyAndVisible];
[_window release];
1.先创建一个视图控制器对象.
RootViewController *rootVC = [[RootViewController alloc] init];
2.创建一个导航视图控制器.
UINavigationController *naVC = [[UINavigationController alloc] initWithRootViewController:rootVC];
3.指定导航视图控制器称为window的根视图控制器
self.window.rootViewController = naVC;
4.内存管理
[rootVC release];
[naVC release];
return YES;
}
RootViewController.m
#import "RootViewController.h"
#import "SecondViewController.h"
@interface RootViewController ()
@end
@implementation RootViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor greenColor];
self.navigationController.navigationBar.translucent = NO;
#warning 导航视图控制器里的navigationbar默认是半透明效果,但是不透明效果会修改坐标系的起点,如果想要半透明效果,为了让视图全部显示,需要把坐标向下平移64.
UIScrollView *view = [[UIScrollView alloc] initWithFrame:CGRectMake(0, 0, 100, 100)];
view.backgroundColor = [UIColor blueColor];
[self.view addSubview:view];
[view release];
self.title = @"标题";
self.navigationItem.title = @"标题";
UISegmentedControl *segmentedC = [[UISegmentedControl alloc] initWithItems:@[@"通话", @"信息"]];
self.navigationItem.titleView = segmentedC;
self.navigationItem.leftBarButtonItem = [[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemCamera target:self action:@selector(click:)] autorelease];
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithTitle:@"测试" style:UIBarButtonItemStylePlain target:self action:@selector(click:)] autorelease];
UIButton *itemButton = [UIButton buttonWithType:UIButtonTypeCustom];
[itemButton setImage:[UIImage imageNamed:@"1"] forState:UIControlStateNormal];
itemButton.frame = CGRectMake(0, 0, 40, 40);
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithCustomView:itemButton] autorelease];
UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem];
[button setTitle:@"下一页" forState:UIControlStateNormal];
button.layer.borderWidth = 1;
button.layer.cornerRadius = 10;
[button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside];
button.frame = CGRectMake(100, 100, 150, 50);
[self.view addSubview:button];
}
- (void)buttonAction:(UIButton *)button {
SecondViewController *secondVC = [[SecondViewController alloc] init];
[self.navigationController pushViewController:secondVC animated:YES];
[secondVC release];
NSLog(@"%@", self.navigationController);
}
- (void)click:(UIBarButtonItem *)item {
NSLog(@"我被点击了");
}
SecondViewController.m
#import "SecondViewController.h"
#import "ThirdViewController.h"
@interface SecondViewController ()
@end
@implementation SecondViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.title = @"第二页";
self.view.backgroundColor = [UIColor cyanColor];
UIButton *itemButton = [UIButton buttonWithType:UIButtonTypeCustom];
[itemButton setImage:[UIImage imageNamed:@"1"] forState:UIControlStateNormal];
itemButton.frame = CGRectMake(0, 0, 40, 40);
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithCustomView:itemButton] autorelease];
UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem];
[button setTitle:@"下一页" forState:UIControlStateNormal];
button.layer.borderWidth = 1;
button.layer.cornerRadius = 10;
[button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside];
button.frame = CGRectMake(100, 100, 150, 50);
[self.view addSubview:button];
}
- (void)buttonAction:(UIButton *)button {
ThirdViewController *thirdVC = [[ThirdViewController alloc] init];
[self.navigationController pushViewController:thirdVC animated:YES];
[thirdVC release];
NSLog(@"%@", self.navigationController);
}
ThirdViewController.m
#import "ThirdViewController.h"
#import "FourthViewController.h"
@interface ThirdViewController ()
@end
@implementation ThirdViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.title = @"第三页";
self.view.backgroundColor = [UIColor grayColor];
UIButton *itemButton = [UIButton buttonWithType:UIButtonTypeCustom];
[itemButton setImage:[UIImage imageNamed:@"1"] forState:UIControlStateNormal];
itemButton.frame = CGRectMake(0, 0, 40, 40);
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithCustomView:itemButton] autorelease];
UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem];
[button setTitle:@"下一页" forState:UIControlStateNormal];
button.layer.borderWidth = 1;
button.layer.cornerRadius = 10;
[button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside];
button.frame = CGRectMake(100, 100, 150, 50);
[self.view addSubview:button];
}
- (void)buttonAction:(UIButton *)button {
FourthViewController *fourthVC = [FourthViewController alloc];
[self.navigationController pushViewController:fourthVC animated:YES];
[fourthVC release];
NSLog(@"%@", self.navigationController.viewControllers);
}
FourthViewController.m
#import "FourthViewController.h"
#import "FifthViewController.h"
@interface FourthViewController ()
@end
@implementation FourthViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.title = @"第四页";
self.view.backgroundColor = [UIColor yellowColor];
UIButton *itemButton = [UIButton buttonWithType:UIButtonTypeCustom];
[itemButton setImage:[UIImage imageNamed:@"1"] forState:UIControlStateNormal];
itemButton.frame = CGRectMake(0, 0, 40, 40);
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithCustomView:itemButton] autorelease];
UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem];
[button setTitle:@"下一页" forState:UIControlStateNormal];
button.layer.borderWidth = 1;
button.layer.cornerRadius = 10;
[button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside];
button.frame = CGRectMake(100, 100, 150, 50);
[self.view addSubview:button];
}
- (void)buttonAction:(UIButton *)button {
FifthViewController *fifthVC = [[FifthViewController alloc] init];
[self.navigationController pushViewController:fifthVC animated:YES];
[fifthVC release];
NSLog(@"%@", self.navigationController.viewControllers);
}
FifthViewController.m
#import "FifthViewController.h"
@interface FifthViewController ()
@end
@implementation FifthViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.title = @"第五页";
self.view.backgroundColor = [UIColor redColor];
UIButton *itemButton = [UIButton buttonWithType:UIButtonTypeCustom];
[itemButton setImage:[UIImage imageNamed:@"1"] forState:UIControlStateNormal];
itemButton.frame = CGRectMake(0, 0, 40, 40);
self.navigationItem.rightBarButtonItem = [[[UIBarButtonItem alloc] initWithCustomView:itemButton] autorelease];
UIButton *button = [UIButton buttonWithType:UIButtonTypeSystem];
[button setTitle:@"返回" forState:UIControlStateNormal];
button.layer.borderWidth = 1;
button.layer.cornerRadius = 10;
[button addTarget:self action:@selector(buttonAction:) forControlEvents:UIControlEventTouchUpInside];
button.frame = CGRectMake(100, 100, 150, 50);
[self.view addSubview:button];
}
- (void)buttonAction:(UIButton *)button {
[self.navigationController popToRootViewControllerAnimated:YES];
}