http://www.javaworld.com/article/2074924/java-web-development/get-the-app-out.html
We all built EJBs, servlets, and JSPs before J2EE came upon the scene at 1999's JavaOne. In those days, EJBs were deployed into EJB containers (mostly in application servers such as WebLogic or Netscape Application Server) using proprietary deployment descriptors. Servlets and JSPs, meanwhile, were deployed into servlet engines such as JRun, ServletExec, and JServ. In 1999, Sun altered the landscape by consolidating all the Java server-side technologies under a single banner called Java 2 Enterprise Edition -- J2EE.
This article shows programmers how to assemble and deploy J2EE applications based on EJBs, servlets, and JSP. We will begin with a brief introduction to the J2EE platform and J2EE applications, then dive straight into the mechanics of assembly and deployment.
The J2EE platform and J2EE applications
But why J2EE? The various specifications were doing just fine on their own, right? Individually that's true. For example, EJBs helped to separate and isolate the business logic portion of an application and to hide the unnecessary plumbing infrastructure. Servlets and JSPs both provided a means to create Web-based applications easily. Java Transaction Architecture (JTA) and Java Transaction Service (JTS) provided transactional capabilities to Java applications. JNDI helped applications find each other, while JDBC allowed connectability to relational databases. Individually, each technology performed its task satisfactorily, but the synergy created with their combination finally allows true Java-based enterprise applications. J2EE is the glue that ties them all together in a coherent bundle by defining how they work together to form a complete enterprise platform.
J2EE applications are applications written using the J2EE platform and deployed on a J2EE application server. They are composed of one or more J2EE components (classes developed based on the J2EE platform) and a J2EE application deployment descriptor. The deployment descriptor lists the application's components as modules. A J2EE module represents a J2EE application's basic unit of composition.
The J2EE component model also allows the various modules to be deployed as individual components, component libraries, or J2EE applications. J2EE modules include:
- EJB JARs (EJBs)
- Web application WARs (servlets, JSPs, and HTML files)
- Application client JARs (typically, GUI programs running client-server, including applets)
That means that J2EE applications can not only be Web-based applications based on the J2EE platform, but also client-server applications, as long as they are based on the J2EE platform. Note that a J2EE application does not necessarily need to have EJBs -- it can contain just JSPs, servlets, and HTML files. It can also be simply a Java program (client-server) that accesses the J2EE platform, a gaggle of EJBs that work together, all three, or any two combinations.
This article describes packaging and deployment for EJBs and Web applications only. The deployment platform uses the Sun J2EE Reference Implementation (RI) Server version 1.2.1 running on Windows NT 4.0.
Assembly
As shown in Figure 1, the assembly of a J2EE application is hierarchical. First, the EJB classes are jarred up with a component deployment descriptor, then the Web resources such as the servlet, JSP, and HTML code are jarred up with their own component deployment descriptor. Those JAR and WAR files are then assembled and jarred into an Enterprise ARchive (EAR) file alongside an application deployment descriptor. Additional modules can be added as the application grows.
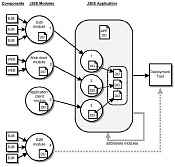
In the sections below, we'll examine the step-by-step process to create J2EE applications from EJB modules and Web applications.
EJBs
In J2EE, EJBs represent one of the three J2EE modules types included in a J2EE application. EJBs use the JAR format for packaging and delivery, so that all the necessary classes, interfaces, and other resources associated with the bean are included in one file. Be aware that if you are familiar with the EJB 1.0 packaging and deployment, you will notice that J2EE (which uses EJB 1.1) EJB packaging slightly differs. The packaging steps are:
-
Create a deployment descriptor with the file named
ejb-jar.xml
, which you place in a directory namedMETA-INF
. Note thatejb-jar.xml
must be at the top level of theMETA-INF
directory of the EJB jar file. Moreover, it must be a valid XML document, according to the document type definition (DTD) for a J2EE:application XML document. The deployment descriptor must include an XML DTD with aPUBLIC
identifier of:-//Sun Microsystems// DTD Enterprise JavaBeans 1.1//EN
Here's an example deployment descriptor:
<?xml version="1.0" encoding="Cp1252"?> <!DOCTYPE ejb-jar PUBLIC '-//Sun Microsystems, Inc.//DTD Enterprise JavaBeans 1.1//EN' 'http://java.sun.com/j2ee/dtds/ejb-jar_1_1.dtd'> <ejb-jar> <description>My First J2ee Application EJBs</description> <display-name>AppJar</display-name> <enterprise-beans> <session> <description>Description of the EJBs here</description> <display-name> AppJar </display-name> <ejb-name> AppJar </ejb-name> <home>com.sttarfire.sample.ejb.AppHome</home> <remote> com.sttarfire.sample.ejb.App</remote> <ejb-class> com.sttarfire.sample.ejb.AppBean</ejb-class> <session-type>Stateless</session-type> <transaction-type>Bean</transaction-type> </session> </enterprise-beans> </ejb-jar>
- Place all EJB class files in their respective directories. For example,
com.sttarfire.util.StringUtil.class
should be in a subdirectory namedcom/sttarfire/util/
. - Supporting classes can be placed into the directories as well.
- If a library file is referred to, create a
manifest.mf
file and place it into theMETA-INF/
with the content pointing to the library file. - Package all the files with the JAR file format. The file must be named with a
.jar
extension.
The resulting jar file is a J2EE module that is deployed standalone or included into the J2EE application EAR file. If you open it up using a file archive utility, it'll look something like:
/com/sttarfire/samples/ejb/App.class /com/sttarfire/samples/ejb/AppHome.class /com/sttarfire/samples/ejb/AppBean.class /META-INF/ejb-jar.xml /META-INF/manifest.mf
Web applications
As explained earlier, J2EE applications can consist of EJBs, Web applications, and/or Java clients. Web applications represent collections of servlets, JSPs, HTML pages, classes, and other resources that can be bundled and run on multiple containers from multiple vendors. Prior to J2EE, those individual components were deployed separately; in J2EE they all become neatly packaged into a Web ARchive (WAR) file for deployment as a Web application into a J2EE server. A deployed Web application is rooted at a specific path within a Web server.
A Web application may consist of the following items:
- Servlets
- JSPs
- Utility classes
- Static documents (HTML, images, sounds, etc.)
- Client-side applets, beans, and classes
- Descriptive meta information that ties all of the above elements together
A Web application needs to be archived into a single WAR file prior to inclusion into the overall EAR file. Note that individual WAR files can also be deployed separately into a J2EE server.
Creating a WAR file is similar to the creation of an EJB JAR file:
-
First, create a deployment descriptor with the file named
web.xml
, which you'll place in a directory namedWEB-INF
. Again, note thatweb.xml
must be at the top level of theWEB-INF
directory of the war file. It must also be a valid XML document, according to the DTD for a J2EE:application XML document, just like the EJB deployment descriptor. The deployment descriptor must include an XML DTD with aPUBLIC
identifier of:-//Sun Microsystems// DTD Web Application 2.2//EN
Here's an example of a Web application deployment descriptor:
<?xml version="1.0" encoding="Cp1252"?> <!DOCTYPE web-app PUBLIC '-//Sun Microsystems, Inc.//DTD Web Application 2.2//EN' 'http://java.sun.com/j2ee/dtds/web-app_2.2.dtd'> <web-app> <display-name>AppWar</display-name> <description>My First J2EE Web Module</description> <servlet> <servlet-name>AppServlet</servlet-name> <display-name>AppServlet</display-name> <description>Description of the servlet here</description> <servlet-class>AppServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>AppServlet</servlet-name> <url-pattern>AppServlet</url-pattern> </servlet-mapping> <session-config> <session-timeout>0</session-timeout> </session-config> </web-app>
- Place all the servlet class files in the
WEB-INF/classes/
directory. - You can also place the supporting classes into the
WEB-INF/lib/
directory. - Package all the files using the JAR file format. The file must be named with a
.war
extension.
Like the EJB JAR, the Web application WAR file is a J2EE module that can be deployed standalone or included in the J2EE application EAR file. For programmers who have developed servlets and JSPs prior to J2EE, you will find this additional process to be very helpful during deployment. Indeed, you no longer need to figure out where to deploy the individual files; previously, you could place servlets, JSPs, HTML, and other resource files anywhere on a servlet engine (depending on the servlet engine in use). That situation could turn into a maintenance nightmare when different programmers placed servlets, JSPs, HTML, and other files in different places. Under the new model, deploying J2EE Web applications proves much simpler: Web applications are deployed to their respective context roots (described in the EAR deployment descriptor).
Note: the META-INF/
and WEB-INF/
directories are case-sensitive and should be entirely uppercase.
When you finish, the war file's structure should resemble:
/index.html /howto.jsp /feedback.jsp /images/banner.gif /images/somesample.gif /WEB-INF/web.xml /WEB-INF/lib/jspbean.jar /WEB-INF/classes/com/sttarfire/servlets/AppServlet.class /WEB-INF/classes/com/sttarfire/util/MyUtils.class
J2EE application
A J2EE application is a combination of one or more EJB JAR files, Web application WAR files, and application client JAR files (not discussed in detail here). As mentioned before, J2EE modules such as EJBs and the Web applications do not have to be packaged into a EAR file. Indeed, they can be deployed individually. But for a cleaner packaging of a single application, including all the necessary modules in a single file is much easier.
You package a J2EE application using the JAR file format into a file with an .ear
extension. A minimal J2EE application package will contain only J2EE modules and the application deployment descriptor. Larger J2EE application packages may also include libraries referenced by J2EE modules, help files, and documentation to aid the deployer and other interested parties.
With that in mind, to create a EAR file, you will need to do the following:
-
Create a deployment descriptor for the J2EE application, an XML file with the file name
application.xml
. Again, that file must be in a directory namedMETA-INF
and at the top level of theMETA-INF
directory of the application EAR file. As before, it must be a valid XML document, according to the DTD for a J2EE:application XML document. The deployment descriptor must include an XML document type definition with aPUBLIC
identifier of:-//Sun Microsystems//J2EE Application Deployment 1.0//EN
An example of the J2EE application deployment descriptor is given below:
<?xml version="1.0" encoding="Cp1252"?> <!DOCTYPE application PUBLIC '-//Sun Microsystems, Inc.//DTD J2EE Application 1.2//EN' 'http://java.sun.com/j2ee/dtds/application_1_2.dtd'> <application> <display-name>MyJ2EEApp</display-name> <description>My First J2EE Application</description> <module> <ejb>AppJar.jar</ejb> </module> <module> <web> <web-uri>AppWeb.war</web-uri> <context-root>AppRoot</context-root> </web> </module> </application>
- Place the J2EE modules at the root directory level of the EAR file. As mentioned earlier, J2EE modules are EJB jar files, Web application WAR files, and standalone client jar files.
- Place any library files accessed from the various J2EE modules in the
library/
directory. - Place any server-specific deployment descriptors in whichever directory they need to go. For example, the Sun Reference Implementation of the J2EE Server has an additional deployment descriptor named
sun-j2ee-ri.xml
. - Package all the files with the JAR file format. The file must be named with an
.ear
extension. That is the final archive file used for deployment in any J2EE-compliant server.
Here's what your EAR file might look like:
/AppJar.jar /AppWar.war /library/library.jar /META-INF/application.xml /META-INF/sun-j2ee-ri.xml
Deployment
The J2EE component model allows the various modules to be deployed as individual components, component libraries, or J2EE applications. If creating all those archive files sounds like a whole lot of work to you, don't worry. Most application servers come with a deployment tool to assist in the creation of the various archive files, as well as to automate the deployment process. Deployment tools vary with each application server. For example, the Sun RI J2EE Server deploys an EAR file with the following command:
deploytool -deploy J2EEApp.ear myServerName
For more options, please refer to the documentation for the RI J2EE Server.
How to build an EAR file using Apache Ant
However, if you prefer to deploy by hand, an alternate way of automating the process exists. Ant, an open source build utility included in the Apache Group's Jakarta project, can compile the various Java source files and package an EAR file from the various classes. After setting up Ant to run, the following build file can be used to housekeep and compile the source code, then archive it as mentioned above into a JAR, WAR, and at the final stage, an EAR file. Using such a tool helps in the tedious task of deleting files and recreating archives during development.
The following command runs Ant with the build file named app.xml
:
ant -buildfile app.xml
And here's the file:
<?xml version="1.0"?> <!-- ======================================================================= --> <!-- My First J2EE Application build file --> <!-- ======================================================================= --> <project name="app" default="app" basedir="."> <!-- Properties and variables --> <property name="Name" value="J2EEApp"/> <property name="version" value="1.0"/> <property name="src.dir" value="/sttarfire/samples/src/"/> <property name="bin.dir" value="/sttarfire/sample/deploy/app/jar/"/> <property name="jar.dir" value="/sttarfire/sample/deploy/app/jar/"/> <property name="war.dir" value="/sttarfire/sample/deploy/app/war/"/> <property name="ear.dir" value="/sttarfire/sample/deploy/app/ear/"/> <!-- Deletes previously generated classes --> <target name="delete_bin"> <delete dir="${jar.dir}/com/sttarfire/samples/app" includes="*.class"/> <delete dir="${jar.dir}/ com/sttarfire/samples/app/ejb" includes="*.class"/> </target> <!-- compile all java source code --> <target name=" compile" depends=" delete_bin"> <javac srcdir="${src.dir}" destdir="${bin.dir}" classpath="${classpath}" debug="on" deprecation="off" optimize="on" > <include name="**/*.java"/> <exclude name="**/version.txt" /> <exclude name="**/optional/**" /> </javac> </target> <!-- Create the EJB JAR file --> <target name=" create_jar" depends=" compile"> <copyfile src="${jar.dir}/AppServlet.class" dest="${war.dir}/WEB-INF/classes/AppServlet.class" /> <delete file="${jar.dir}/AppServlet.class" /> <delete file="${jar.dir}/AppJar.jar" /> <jar jarfile="${jar.dir}/ AppJar.jar" basedir="${jar.dir}/" /> </target> <!-- Create the Web application WAR file --> <target name=" create_war" depends=" create_jar"> <delete file="${war.dir}/AppWar.war" /> <jar jarfile="${war.dir}/ AppWar.war" basedir="${war.dir}/" /> </target> <!-- Create the J2EE application EAR file --> <target name=" create_ear" depends="create_jar, create_war"> <copyfile src="${jar.dir}/AppJar.jar" dest="${ear.dir}/AppJar.jar" /> <copyfile src="${war.dir}/AppWar.war" dest="${ear.dir}/AppWar.war" /> <delete file="${ear.dir}/App.ear" /> <jar jarfile="${ear.dir}/App.ear" basedir="${ear.dir}/" /> </target> <!-- main build --> <target name="app" depends=" create_ear"> </target> </project>Ant can also deploy the J2EE application automatically (not shown above), as well as perform other tasks. Ant is cross-platform -- a big advantage. Instead of creating a batch build file for Windows NT/2000 development and a Unix shell build file for Unix-based development, you can just use Ant in both platforms. It's generally a useful tool, compared to proprietary IDEs that can be expensive and not as portable to multiple platforms.
Conclusion
We have covered the internals of J2EE applications, as well as the basic mechanics of deploying them by hand (or by Ant). J2EE is a consolidating platform for all Java-based enterprise specifications such as EJB, servlet, JSP, and so on. J2EE applications combine one or more J2EE modules, which can be an EJB, a Web application, or a Java client (either application or applet). A J2EE application is archived into a EAR file for deployment, although J2EE modules can deployable separately. We also learned the creation process for the various archive files.
Packaging and deploying a J2EE application is typically done by the J2EE application server's tools, so programmers generally do not need to deploy by hand. However, knowing how the process works is still important, especially in cases in which your application's portability is critical. More importantly (and unfortunately), most of the J2EE server implementations are less than perfect. Deployment for a new platform often takes up considerably more time than anticipated because of various assumptions based on a frequently used (and favorite) application server. As a result, J2EE applications created by a deployment tool from one vendor might not be directly portable to another application server. Knowing the deployment process and knowing how the deployment process works in your J2EE server platform of choice goes a long way toward helping you debug your J2EE application deployment.
Chang Sau Sheong is VP of product engineering and cofounder of elipva (previously known as starfire.com), an e-business software and services provider headquartered in Singapore. He is one of the two original designers of the flagship product, the elipva Portal Application Framework, a J2EE-based application framework that allows developers to build and deploy portals quickly. He has been programming in Java for the past four years and developing servlets and EJBs since the respective specifications first appeared.