Demo最终效果图
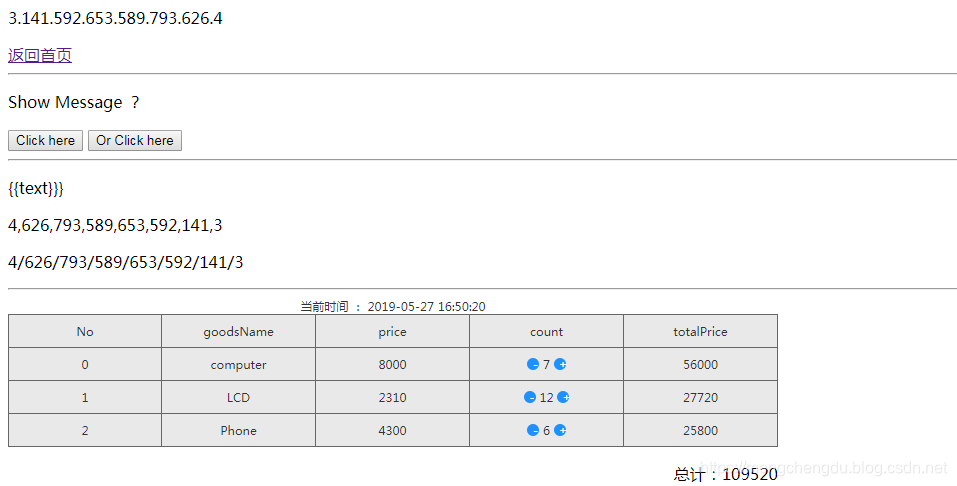
Vue 基本使用
- 基本的数据绑定
- {{}} 插值表达式绑定数据。这种情况返回输出的是纯字符串。
- 基本指令运用
- v-bind 绑定某一属性。如 v-bind:src=“http://…”
- v-on
- v-if
- v-html 如果想输出 HTML 文档
- v-pre 跳过当前元素和它子元素的编译过程。
- : 是 v-bind 的语法糖。v-bind:src="" 等价于 :src=""
- @ 是 v-on 的语法糖。v-on:click=“btnfunction()” 等价于 @click=“btnFunction()”
- 插值过滤器
- 通常用户格式化文本:{{date | filtersA}}
- 过滤器可以连接多个:{{date | filtersA | filterB}}
- 过滤器可以传参:{{date | formatDate(arg1, arg2)}}。但是接收参数时注意,第一个参数为数据本身,后面的参数依次为手动传入的参数。
- 计算属性 & 方法
- 相同点
- 二者的目的都是处理数据
- 二者内部的代码写法基本一样
- 不同点
- 调用方式不同
+ 调用方法时需要加小括号(),括号内可以传参,如果不加,也不会报错,但返回的是方法本身,而并非值。 - 队列缓存机制
+ 计算属性有自己的缓存机制,当计算属性依赖的数据发生变化时,才会重新取值,所以只要 text 不变,计算属性的值就不更新
+ 方法只要重新渲染DOM,就会被调用执行。
+ ★ 当处理大数据量时建议使用计算属性,因为缓存机制可以节省很多不必要的调用,除非业务需求不需要缓存。 - get & set
+ 方法没有 get & set 方法
+ 计算属性包含 get 和 set 方法。默认的用法只是利用 get 来读取。如果需要时,可以手动设置 set 方法。
代码
- CSS 部分(对table表格和button按钮略微调整)
<style>
table {
border-collapse: collapse;
border-width: 1;
font-size: 11px;
color: #333;
border-color: #666;
}
th,
td {
border-style: solid;
border-width: 1px;
padding: 8px;
border-color: #666;
text-align: center;
width: 137px;
height: 16px;
}
th {
background-color: #5f5f5f;
}
td {
background-color: #e9e9e9;
}
table button {
width: 12px;
height: 12px;
border-width: 0px;
border-radius: 50%;
background: #1E90FF;
cursor: pointer;
color: white;
font-size: 4px;
margin: 0 auto;
}
table button:hover {
background: #5599FF;
}
table button span {
line-height: 12px;
}
</style>
<div id="app">
<p>{{text}}</p>
<span v-html="link"></span>
<hr>
<p v-if='isShow'>Show Message ?</p>
<button v-on:click="btn_click">Click here</button>
<button @click="isShow = !isShow">Or Click here</button>
<hr>
<p v-pre>{{text}}}</p>
<p>{{reversedText2}}</p>
<p>{{reversedText1()}}</p>
<hr>
<div style="width:770px">
<table>
<caption>当前时间 : {{date | formatDate}}</caption>
<thead>
<tr>
<td>No</td>
<td>goodsName</td>
<td>price</td>
<td>count</td>
<td>totalPrice</td>
</tr>
</thead>
<tbody v-for="(item, index) in demoArray">
<tr>
<td>{{index}}</td>
<td>{{item.goodsName}}</td>
<td>{{item.price}}</td>
<td>
<button @click="reduce(index)" :disabled="item.count <= 0"><span>-</span>
</button>
{{item.count}}
<button @click="add(index)"><span>+</span>
</button>
</td>
<td>{{item.price * item.count}}</td>
</tr>
</tbody>
</table>
<p style="float:right">总计:{{totalPrice}}</p>
</div>
</div>
<script>
var pDate = function (value) {
return value < 10 ? '0' + value : value;
}
var app = new Vue({
el: '#app',
data: {
text: '3.141.592.653.589.793.626.4',
link: '<a href="./index.html">返回首页</a>',
isShow: true,
date: new Date(),
demoArray: [{
goodsName: 'computer',
price: 8000,
count: 7
}, {
goodsName: 'LCD',
price: 2310,
count: 12
}, {
goodsName: 'Phone',
price: 4300,
count: 6
}]
},
methods: {
btn_click: function () {
this.isShow = !this.isShow;
alert('onclick');
this.showMessageBox();
},
showMessageBox: function () {
alert('messageBox');
},
reversedText1: function () {
return this.text.split('.').reverse().join('/');
},
reduce: function (index) {
this.demoArray[index].count = this.demoArray[index].count - 1;
},
add: function (index) {
this.demoArray[index].count = this.demoArray[index].count + 1;
}
},
computed: {
reversedText2: function () {
return this.text.split('.').reverse().join(',');
},
totalPrice: {
get: function () {
var total = 0;
this.demoArray.forEach(item => {
total += item.price * item.count;
});
return total;
},
set: function () {
}
}
},
filters: {
formatDate: function (value) {
var date = new Date(value);
var year = date.getFullYear();
var month = pDate(date.getMonth() + 1);
var day = pDate(date.getDate());
var hour = pDate(date.getHours());
var miniutes = pDate(date.getMinutes());
var seconds = pDate(date.getSeconds());
return year + '-' + month + '-' + day + ' ' + hour + ':' + miniutes + ':' + seconds;
}
},
beforeCreate: function () {
console.log("实例初始化之前");
},
created: function () {
console.log("实例创建完成");
},
beforeMount: function () {
console.log("实例挂载之前");
},
mounted: function () {
console.log("实例挂载之后");
var _this = this;
this.timer = setInterval(function () {
_this.date = new Date();
}, 1000)
},
beforeUpdate: function () {
console.log("数据更新 DOM 重新渲染之前");
},
updated: function () {
console.log("数据更新 DOM 重新渲染之后");
},
beforeDestroy: function () {
console.log("实例销毁之前");
if (this.timer) {
clearInterval(this.timer);
}
},
destroyed: function () {
console.log("实例销毁之后");
},
});
</script>