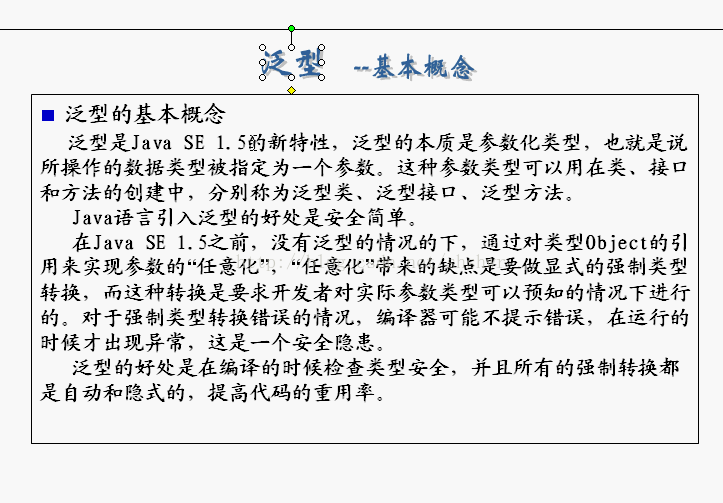
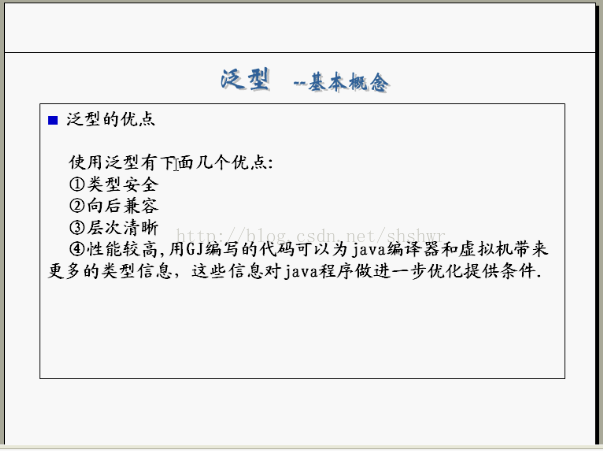
package hanshunping;
import java.util.ArrayList;
public class Test {
public static void main(String[] args) {
ArrayList<Dog> al=new ArrayList<Dog>();
Dog dog1=new Dog();
al.add(dog1);
Dog temp=(Dog)al.get(0);//有安全隐患
Cat temp2=(Cat)al.get(0);
}
}
class Cat{
private String color;
private int age;
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
class Dog
{
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
package hanshunping;
import java.lang.reflect.Method;
public class Test2 {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
Gen<Bird> gen1=new Gen<Bird>(new Bird());
gen1.showTypeName();
}
}
//定义一个鸟类
class Bird
{
public void test1(){
System.out.println("aa");
}
public void count(int a,int b){
System.out.println(a+b);
}
}
//定义一个类
class Gen<T>
{
private T o;
//构造函数
public Gen(T a){
o=a;
}
//得到T的类型名称
public void showTypeName(){
System.out.println("类型是:"+o.getClass().getName());
//通过反射机制,可以拿到一个类的很多信息。
Method [] m=o.getClass().getDeclaredMethods();
//打印
for (int i=0;i<m.length;i++){
System.out.println(m[i].getName());
}
}
}