1.两数之和->hashmap
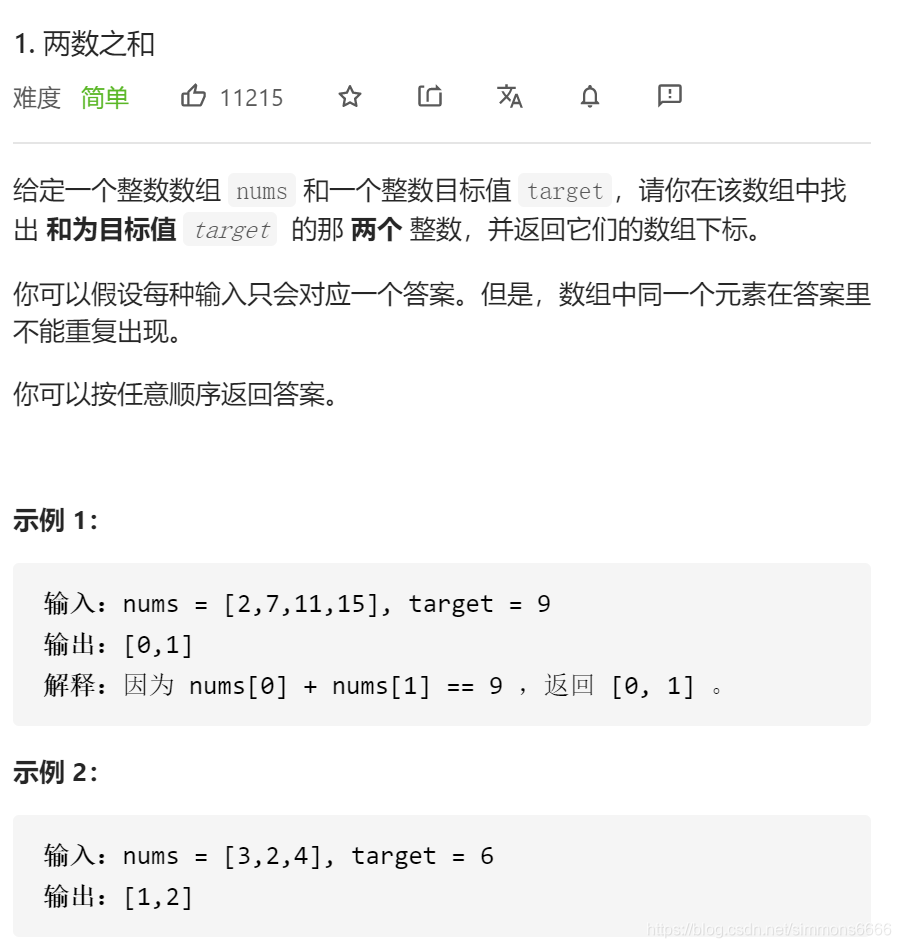
class Solution {
public int[] twoSum(int[] nums, int target) {
int[] res=new int[2];
if(nums.length==0){
return res;
}
Map<Integer,Integer> map=new HashMap<>();
for(int i=0;i<nums.length;i++){
if(map.containsKey(target-nums[i])){
res[0]=map.get(target-nums[i]);
res[1]=i;
}else{
map.put(nums[i],i);
}
}
return res;
}
}
*2.数值的整数次方->迭代+递归
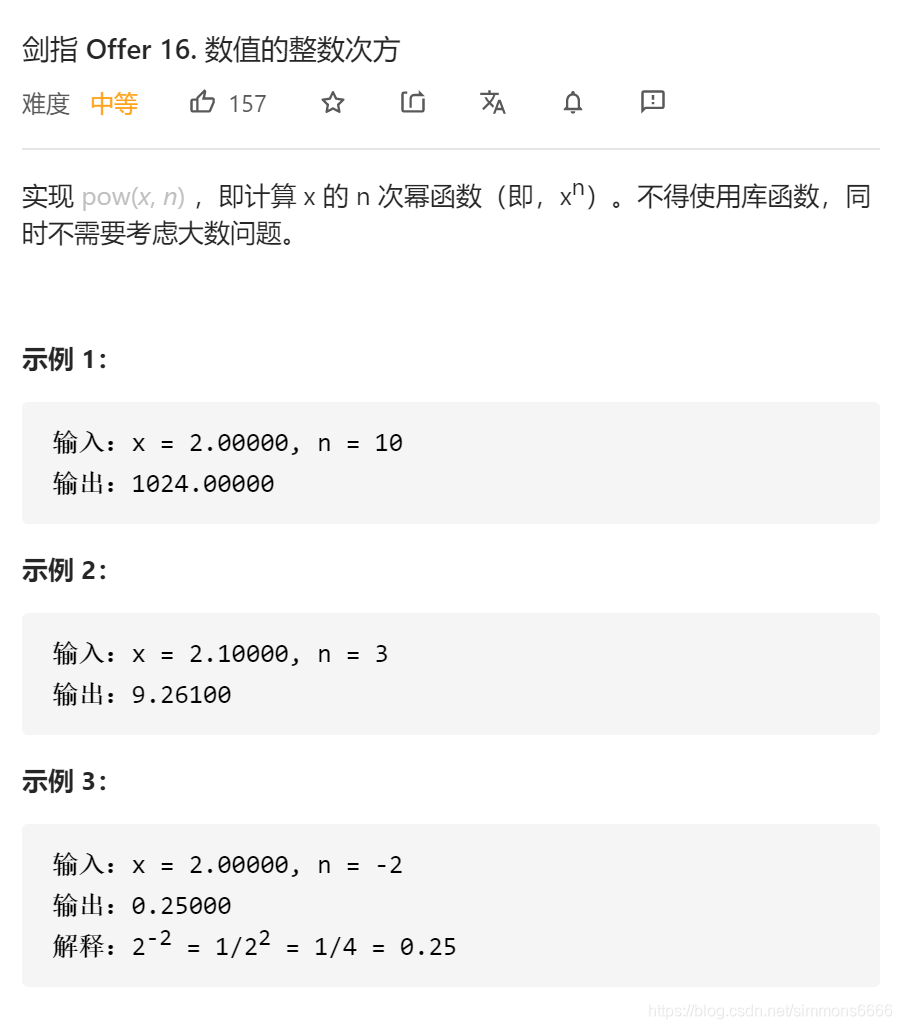
题解:
(1)迭代:
class Solution {
public double myPow(double x, int n) {
if(x==0) return 0;
long b=n;
double res=1.0;
if(b<0){
x=1/x;
b=-b;
}
while(b>0){
if((b&1)==1){
res*=x;
}
x*=x;
b>>=1;
}
return res;
}
}
(2)递归:
class Solution {
public double myPow(double x, int n) {
if(n==0){
return 1;
}else if(n<0){
return 1/(x*myPow(x,-n-1));
}else if(n%2==1){
return x*myPow(x,n-1);
}else{
return myPow(x*x,n/2);
}
}
}
3.打印从1到n的最大的n位数
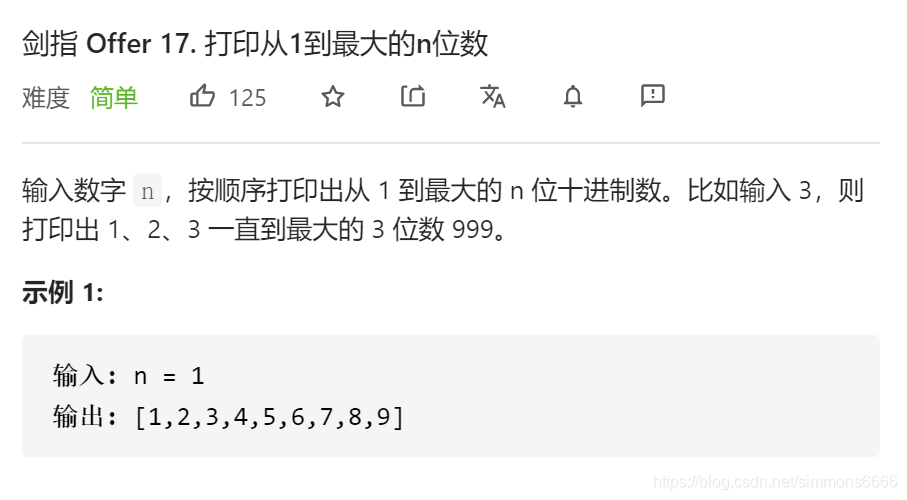
class Solution {
public int[] printNumbers(int n) {
int maxNum=(int)Math.pow(10,n)-1;
int[] res=new int[maxNum];
for(int i=1;i<=maxNum;i++){
res[i-1]=i;
}
return res;
}
}
4.删除链表中的节点
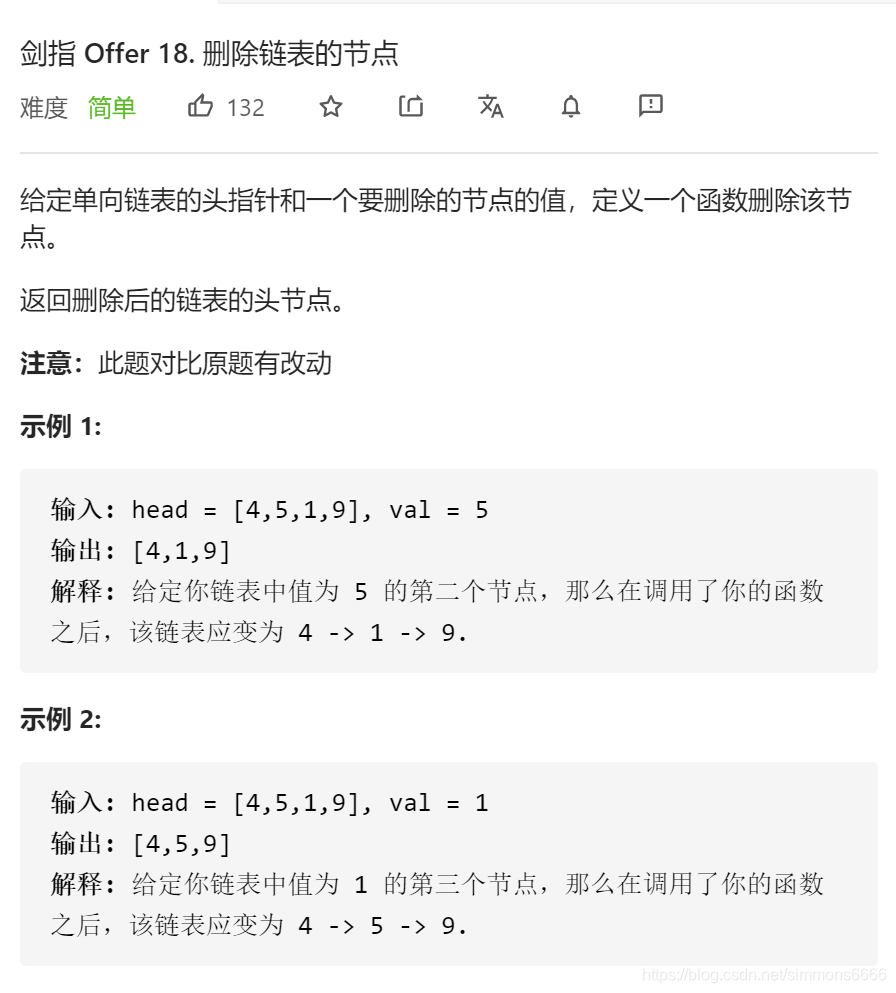
class Solution {
public ListNode deleteNode(ListNode head, int val) {
if(head==null){
return head;
}
if(val==head.val){
return head.next;
}
ListNode cur=head;
ListNode pre=new ListNode(0);
pre.next=cur;
while(cur.val!=val){
cur=cur.next;
pre=pre.next;
}
ListNode next=cur.next;
pre.next=next;
return head;
}
}
5.正则表达式匹配->动归
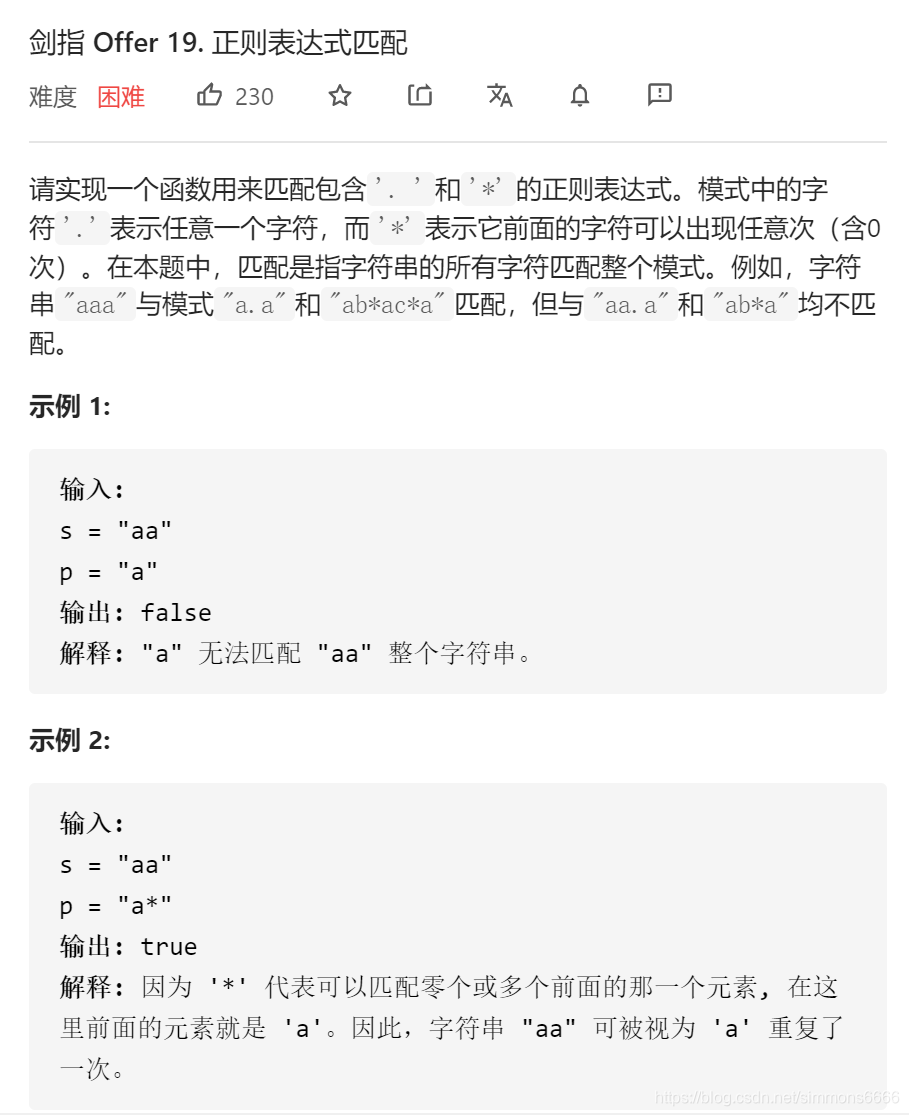
class Solution {
HashMap<String,Boolean> memo=new HashMap<>();
public boolean isMatch(String s, String p) {
return dp(0,0,s,p);
}
public boolean dp(int i,int j,String s, String p){
if(j==p.length()) return i==s.length();
if(memo.containsKey(i+""+j+"")){
return memo.get(i+""+j+"");
}
boolean first_match=(i<s.length()) && (p.charAt(j)==s.charAt(i) || p.charAt(j)=='.');
boolean ans;
if(j<=p.length()-2 && p.charAt(j+1)=='*'){
ans= dp(i,j+2,s,p) || (first_match && dp(i+1,j,s,p));
}else{
ans= first_match && dp(i+1,j+1,s,p);
}
memo.put(i+""+j+"",ans);
return ans;
}
}
6.调整数组顺序使奇数位于偶数前面->双指针
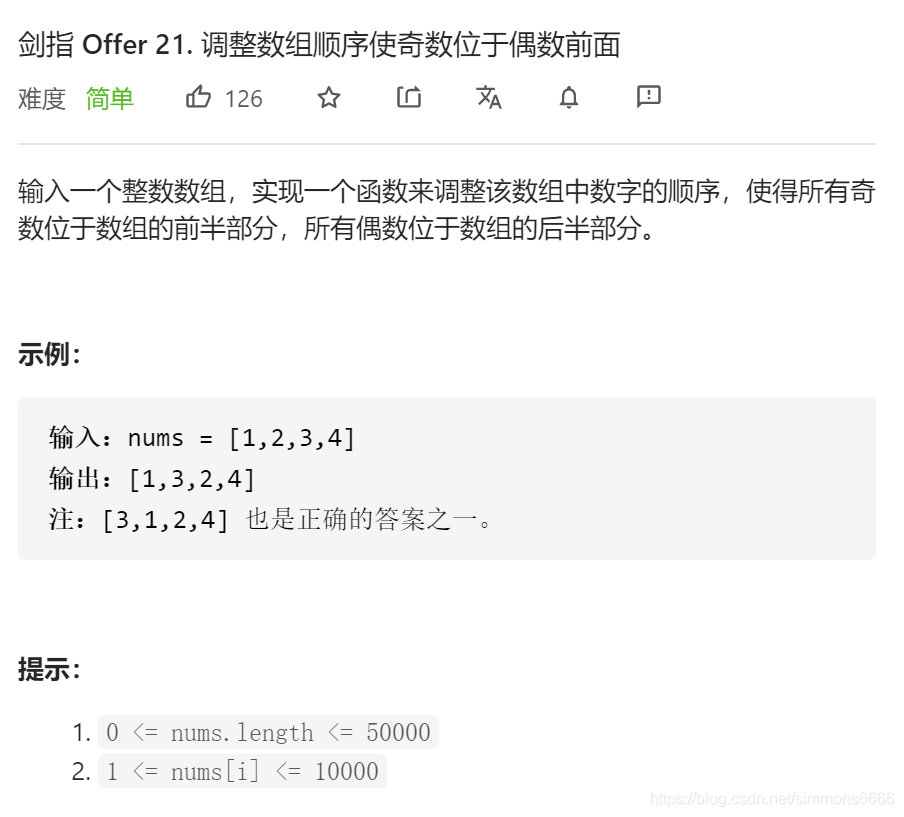
class Solution {
public int[] exchange(int[] nums) {
int n=nums.length;
int left=0,right=n-1;
while(left<right){
if((nums[left]&1)!=0){
left++;
continue;
}
if((nums[right]&1)!=1){
right--;
continue;
}
int temp=nums[left];
nums[left]=nums[right];
nums[right]=temp;
left++;
right--;
}
return nums;
}
}