Creating a ProgressDialog 创建进度对话框
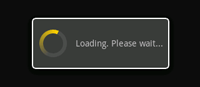
一个ProgressDialog(进度对话框)是AlertDialog的扩展。它可以显示一个进度的动画——进度环或者进度条。这个对话框也可以提供按钮,例如取消一个下载等。
打开一个进度对话框很简单,只需要调用 ProgressDialog.show()即可。例如,上图的对话框可以不通过onCreateDialog(int),而直接显示:
ProgressDialog dialog = ProgressDialog.show(MyActivity.this, "",
"Loading. Please wait...", true);
第一个参数是应用程序上下文。第二个为对话框的标题(这里为空),第三个为对话框内容, 最后一个为该进度是否为不可确定的(这只跟进度条的创建有关,见下一节)。
进度对话框的默认样式为一个旋转的环。如果你希望显示进度值,请看下一节。
Showing a progress bar 显示进度条
使用一个动画进度条来显示进度:
使用 ProgressDialog(Context)构造函数来初始化一个ProgressDialog对象。
将进度样式设置为"STYLE_HORIZONTAL",使用setProgressStyle(int)方法。并且设置其它属性,例如内容等。
在需要显示时调用show()或者从onCreateDialog(int)回调函数中返回该ProgressDialog。
你可以使用 setProgress(int)或者incrementProgressBy(int)来增加显示的进度。
例如,你的设置可能像这样:ProgressDialog progressDialog;
progressDialog = new ProgressDialog(mContext);
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressDialog.setMessage("Loading...");
progressDialog.setCancelable(false);
设置很简单。大部分创建进度对话框需要的代码是在更新它的进程中。你可能需要在一个新的线程中更新它,并使用Handler来将进度报告给Activity。如果你不熟悉使用Handler和另外的线程,请看下列例子,该例子使用了一个新的线程来更新进度。
Example ProgressDialog with a second thread 例--使用一个线程来显示进度对话框
这个例子使用一个线程来跟踪一个进程的进度(其实为从1数到100)。每当进度更新时,该线程通过Handler给主activity发送一个消息。主Activity更新ProgressDialog.package com.example.progressdialog;
打开一个进度对话框很简单,只需要调用 ProgressDialog.show()即可。例如,上图的对话框可以不通过onCreateDialog(int),而直接显示:
ProgressDialog dialog = ProgressDialog.show(MyActivity.this, "",
"Loading. Please wait...", true);
第一个参数是应用程序上下文。第二个为对话框的标题(这里为空),第三个为对话框内容, 最后一个为该进度是否为不可确定的(这只跟进度条的创建有关,见下一节)。
进度对话框的默认样式为一个旋转的环。如果你希望显示进度值,请看下一节。
Showing a progress bar 显示进度条
使用一个动画进度条来显示进度:
使用 ProgressDialog(Context)构造函数来初始化一个ProgressDialog对象。
将进度样式设置为"STYLE_HORIZONTAL",使用setProgressStyle(int)方法。并且设置其它属性,例如内容等。
在需要显示时调用show()或者从onCreateDialog(int)回调函数中返回该ProgressDialog。
你可以使用 setProgress(int)或者incrementProgressBy(int)来增加显示的进度。
例如,你的设置可能像这样:ProgressDialog progressDialog;
progressDialog = new ProgressDialog(mContext);
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressDialog.setMessage("Loading...");
progressDialog.setCancelable(false);
设置很简单。大部分创建进度对话框需要的代码是在更新它的进程中。你可能需要在一个新的线程中更新它,并使用Handler来将进度报告给Activity。如果你不熟悉使用Handler和另外的线程,请看下列例子,该例子使用了一个新的线程来更新进度。
Example ProgressDialog with a second thread 例--使用一个线程来显示进度对话框
这个例子使用一个线程来跟踪一个进程的进度(其实为从1数到100)。每当进度更新时,该线程通过Handler给主activity发送一个消息。主Activity更新ProgressDialog.package com.example.progressdialog;
- import android.app.Activity;
- import android.app.Dialog;
- import android.app.ProgressDialog;
- import android.os.Bundle;
- import android.os.Handler;
- import android.os.Message;
- import android.view.View;
- import android.view.View.OnClickListener;
- import android.widget.Button;
- public class NotificationTest extends Activity {
- static final int PROGRESS_DIALOG = 0;
- Button button;
- ProgressThread progressThread;
- ProgressDialog progressDialog;
- /** Called when the activity is first created. */
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- // Setup the button that starts the progress dialog
- button = (Button) findViewById(R.id.progressDialog);
- button.setOnClickListener(new OnClickListener(){
- public void onClick(View v) {
- showDialog(PROGRESS_DIALOG);
- }
- });
- }
- protected Dialog onCreateDialog(int id) {
- switch(id) {
- case PROGRESS_DIALOG:
- progressDialog = new ProgressDialog(NotificationTest.this);
- progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
- progressDialog.setMessage("Loading...");
- progressThread = new ProgressThread(handler);
- progressThread.start();
- return progressDialog;
- default:
- return null;
- }
- }
- // Define the Handler that receives messages from the thread and update the progress
- final Handler handler = new Handler() {
- public void handleMessage(Message msg) {
- int total = msg.getData().getInt("total");
- progressDialog.setProgress(total);
- if (total >= 100){
- dismissDialog(PROGRESS_DIALOG);
- progressThread.setState(ProgressThread.STATE_DONE);
- }
- }
- };
- /** Nested class that performs progress calculations (counting) */
- private class ProgressThread extends Thread {
- Handler mHandler;
- final static int STATE_DONE = 0;
- final static int STATE_RUNNING = 1;
- int mState;
- int total;
- ProgressThread(Handler h) {
- mHandler = h;
- }
- public void run() {
- mState = STATE_RUNNING;
- total = 0;
- while (mState == STATE_RUNNING) {
- try {
- Thread.sleep(100);
- } catch (InterruptedException e) {
- Log.e("ERROR", "Thread Interrupted");
- }
- Message msg = mHandler.obtainMessage();
- Bundle b = new Bundle();
- b.putInt("total", total);
- msg.setData(b);
- mHandler.sendMessage(msg);
- total++;
- }
- }
- /* sets the current state for the thread,
- * used to stop the thread */
- public void setState(int state) {
- mState = state;
- }
- }
- }
Creating a Custom Dialog 创建自定义对话框
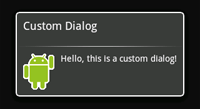
如果你想自定义一个对话框,你可以使用布局元素来创造你的对话框的布局。定义好布局后,将根View对象或者布局资源ID传给setContentView(View).
例如,创建如图所示的对话框:
创建一个xml布局custom_dialog.xml:
例如,创建如图所示的对话框:
创建一个xml布局custom_dialog.xml:
- http://schemas.android.com/apk/res/android"
- android:id="@+id/layout_root"
- android:orientation="horizontal"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:padding="10dp"
- >
- android:layout_width="wrap_content"
- android:layout_height="fill_parent"
- android:layout_marginRight="10dp"
- />
- android:layout_width="wrap_content"
- android:layout_height="fill_parent"
- android:textColor="#FFF"
- />
该xml定义了一个LinearLayout中的一个ImageView 和一个TextView。
将以上布局设为对话框的content view,并且定义ImageView 和 TextView的内容:
将以上布局设为对话框的content view,并且定义ImageView 和 TextView的内容:
- Context mContext = getApplicationContext();
- Dialog dialog = new Dialog(mContext);
- dialog.setContentView(R.layout.custom_dialog);
- dialog.setTitle("Custom Dialog");
- TextView text = (TextView) dialog.findViewById(R.id.text);
- text.setText("Hello, this is a custom dialog!");
- ImageView image = (ImageView) dialog.findViewById(R.id.image);
- image.setImageResource(R.drawable.android);
在初始化Dialog之后,使用setContentView(int),将布局资源id传给它。现在Dialog有一个定义好的布局,你可以使用findViewById(int)来找到该元素的id并修改它的内容。
使用前面所讲的方法显示对话框。
一个使用Dialog类建立的对话框必须有一个标题。如果你不调用setTitle(),那么标题区域会保留空白。如果你不希望有一个标题,那么你应该使用AlertDialog类来创建自定义对话框。然而,由于一个AlertDialog使用AlertDialog.Builder类来建立最方便,所以你没有方法使用setContentView(int),而是只能使用setView(View)。该方法接受一个View对象,所以你需要从xml中展开你的根View。
要展开一个xml布局,使用 getLayoutInflater() (或 getSystemService())取得LayoutInflater,然后调用inflate(int, ViewGroup),第一个参数为布局id,而第二个参数为根view的id。现在,你可以使用展开后的布局来找到View对象并定义ImageView和TextView元素的内容。然后实例化AlertDialog.Builder并使用setView(View)来为对话框设置展开后的布局。例如:
使用前面所讲的方法显示对话框。
一个使用Dialog类建立的对话框必须有一个标题。如果你不调用setTitle(),那么标题区域会保留空白。如果你不希望有一个标题,那么你应该使用AlertDialog类来创建自定义对话框。然而,由于一个AlertDialog使用AlertDialog.Builder类来建立最方便,所以你没有方法使用setContentView(int),而是只能使用setView(View)。该方法接受一个View对象,所以你需要从xml中展开你的根View。
要展开一个xml布局,使用 getLayoutInflater() (或 getSystemService())取得LayoutInflater,然后调用inflate(int, ViewGroup),第一个参数为布局id,而第二个参数为根view的id。现在,你可以使用展开后的布局来找到View对象并定义ImageView和TextView元素的内容。然后实例化AlertDialog.Builder并使用setView(View)来为对话框设置展开后的布局。例如:
- AlertDialog.Builder builder;
- AlertDialog alertDialog;
- Context mContext = getApplicationContext();
- LayoutInflater inflater = (LayoutInflater) mContext.getSystemService(LAYOUT_INFLATER_SERVICE);
- View layout = inflater.inflate(R.layout.custom_dialog,
- (ViewGroup) findViewById(R.id.layout_root));
- TextView text = (TextView) layout.findViewById(R.id.text);
- text.setText("Hello, this is a custom dialog!");
- ImageView image = (ImageView) layout.findViewById(R.id.image);
- image.setImageResource(R.drawable.android);
- builder = new AlertDialog.Builder(mContext);
- builder.setView(layout);
- alertDialog = builder.create();
使用AlertDialog来自定义对话框,可以利用其内置特性例如按钮、选择列表、标题、图标等。