定义
vue的一个插件库,专门用来实现SPA应用。
什么是路由
- 一个路由就是一组映射关系,多个路由需要路由器(router)进行管理
- key为路径,value可能是fuction或compnent
示意图
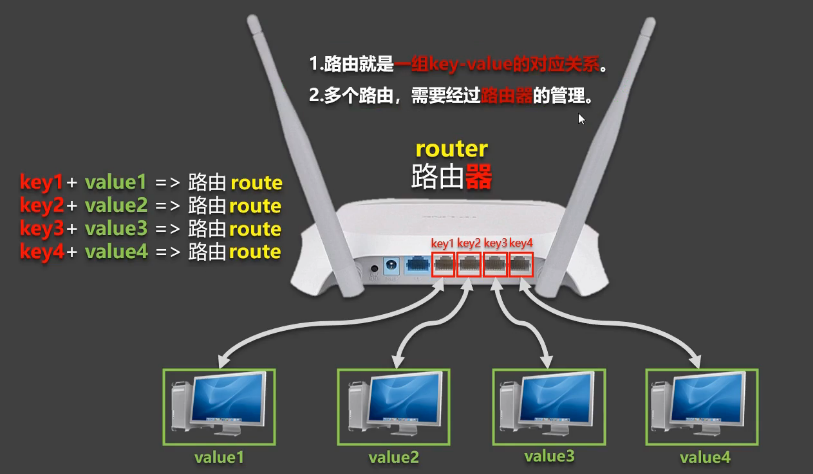
SPA应用的理解
- 单页Web应用
- 整个应用只有一个完整的页面(index.html)
- 点击页面中的导航链接不会刷新页面,只会做页面的局部更新
- 数据需要通过对ajax请求获取
路由的分类
前端路由:
(1)理解:value是component,用于展示页面内容
(2)工作过程:当浏览器的路径改变时,对应的组件就会展示
后端路由:(node)
(1)理解:value是fuction,用于处理客户端提交的请求
(2)工作过程:服务器接收到一个请求时,根据请求路径找到匹配的函数来处理请求,返回响应数据
路由基本使用
- 安装vue-router,命令
npm i vue-router
- 引入
import VueRouter from ''vue-router
- 使用
Vue.use(VueRouter )
- 编写router配置项
main.js
import Vue from "vue";
import App from "./App.vue";
import VueRouter from "vue-router";
import router from "./router";
Vue.use(VueRouter);
// 创建Vue实例对象
new Vue({
render: (h) => h(App),
router: router,
}).$mount("#app");
router/index.js
// 该文件专门用于创建整个应用的路由器引入vueRouter
import VueRouter from "vue-router";
// 引入布局
import About from "../components/About";
import Home from "../components/Home";
// 创建一个路由器实例对象及规则
export default new VueRouter({
routes: [
{ path: "/about", component: About },
{ path: "/home", component: Home },
],
});
App.vue 实现切换(active-class可配置高亮样式)
<router-link to="/about" active-class="active">About</router-link>
<router-link to="/home" active-class="active">Home</router-link>
<!-- 指定展示位置 -->
<router-view></router-view>
注意事项
- 路由组件通常放在pages文件夹;一般组件通常存放在components文件夹 。
- 通过切换,“隐藏”了的路由组件,默认是被销毁的,需要的时候再去挂载。
- 每个组件都有自己的
$route
属性,里面存储着自己的路由信息。- 整个应用只有一个router,可以通过组件的
$router
属性获取到。
嵌套(多级)路由
- 配置路由规则,使用children配置项:
// 该文件专门用于创建整个应用的路由器引入vueRouter import VueRouter from "vue-router"; // 引入布局 import About from "../components/About"; import Home from "../components/Home"; import News from "../components/News"; import Message from "../components/Message"; // 创建一个路由器实例对象及规则 export default new VueRouter({ routes: [ { path: "/about", component: About, }, { path: "/home", component: Home, children: [ //通过children配置子路由 { path: "news", //此处一定不要写/news component: News, }, { path: "message", //此处一定不要写/message component: Message, }, ], }, ], });
跳转(要写完整路径):
<template>
<div>
<router-link to="/home/news">news</router-link>
<router-link to="/home/message">message</router-link>
<router-view></router-view>
</div>
</template>
路由传参query
路由的query参数
- 传递参数
方式一:
<router-link :to="`/home/message/detail?id=${item.id}&title=${item.title}`">{{ item.title }}</router-link>
方式二:
<router-link :to="{
path:'/home/message/detail',
query:{
id:item.id,
title:item.title
}
}">{{ item.title }}</router-link>
- 接收参数
$route.query.id
命名路由
- 作用:可以简化路由的跳转。
- 如何使用:
(1)给路由命名:
(2)简化跳转{ path:'/demo', component:Demo children:[ { path:'test', component:Test, children:[ { name:'hello',//给路由命名 path:'welcome', component:Hello } ] } ] }
<!-- 简化前,需要写完整的路径--> <router-link to="/demo/test/welcome">跳转</routerlink> <!-- 简化后,直接通过名字跳转--> <router-link :to="{name:'hello'}">跳转</routerlink>
路由的params参数-方式一
配置路由,声明接收params参数
export default new VueRouter({
routes: [
{
path: "/about",
component: About,
},
{
path: "/home",
component: Home,
children: [
{
path: "news",
component: News,
},
{
path: "message",
component: Message,
children: [
{
name: "detail",
path: "detail/:id/:title", //使用占位符声明接收params参数
component: Detail,
},
],
},
],
},
],
});
传递参数
<!-- 跳转并携带params参数,to的字符串写法 -->
<router-link :to="`/home/message/detail/${item.id}/${item.title}`">
<!-- 跳转并携带params参数,to的对象写法 -->
<router-link
:to="{
name: 'detail',
params: {
id: item.id,
title: item.title,
},
}">{{ item.title }}</router-link>
特别注意:路由携带params参数,若使用to的对象写法,则不能使用path配置项,必须使用name配置!
接收参数$route.params.title
路由的params参数-方式二
作用:让路由组件更方便的收到参数
传递参数
{
name:'xiangqing',
path:'detail/:id',
component:Detail,
//第一种写法:props值为对象,该对象中所有的key-value的组合最终都会通过props传递给Detail组件
//props:{a:100},
//第二种写法:props值为布尔值,布尔值为true,则把路由收到的所有***params参数**通过props传递给Detail组件
//props:true
//第三种写法:props值为函数,该函数返回的对象中每一组key-value都会通过props传给Detail组件
props(route){
return {
id:route.query.id,
title:route.query.title
}
}
}
接收参数props: ["id", "title"]
<router-link>
的replace属性
- 作用:控制路由跳转时操作浏览器历史记录的模式
- 浏览器的历史记录有两种写入方式:分别为push和replace,push是追加历史记录,replace是替换当前记录。路由跳转时候默认为push
- 如何开始replace模式:
<router-link replace......>xxxx</router-link>
编程式路由导航
- 作用:不借助实现路由跳转,让路由跳转更加灵活
- 具体编码:
//$router的两个API
this.$router.push({
name:"xiangqing",
params:{
id:xxx,
title:xxxx
}
})
this.$router.replace({
name:"xiangqing",
params:{
id:xxx,
title:xxxx
}
})
this.go()//可前进可后退
this.forward()//前进
this.back()//后退
缓存路由组件
- 作用:让不展示的路由组件保持挂载,不被销毁。
- 具体编码:
<!--News为组件名 -->
<keep-alive include="News">
<router-view><router-view>
</keey-alive>
<!--缓存多个组件时使用数组 -->
<keep-alive :include="['News','Message']">
<router-view><router-view>
</keey-alive>
- include不写,则所有组件进行缓存
新的生命周期钩子(路由独有)
- 作用:路由组件所独有的两个钩子,用于捕获路由组件的激活状态。
- 具体名字(写在缓存的组件中) activated 激活 deactivated 失活
路由守卫 (权限)
- 作用:对路由进行权限控制
- 分类:全局守卫、独享守卫、组件内守卫
// 创建一个路由器实例对象及规则
const router = new VueRouter({
routes: [
{
path: "/about",
component: About,
meta: {
isAuth: true,
title: "关于",
},
},
{
path: "/home",
component: Home,
meta: {
isAuth: false,
title: "首页",
},
},
],
});
// 全局前置守卫,初始化时执行,每次路由切换前执行
router.beforeEach((to, from, next) => {
console.log("前置路由");
if (to.meta.isAuth) {
//判断当前路由是否需要进行权限控制
if (localStorage.getItem("user") == "zhangsan") {
next();
}
alert("暂无权限");
} else {
next();
}
});
// 全局后置守卫,初始化时执行,每次路由切换后执行
router.afterEach((to, from) => {
console.log("后置路由");
if (to.meta.title) {
document.title = to.meta.title;
}
});
export default router
独享路由守卫
beforeEnter(to, from, next) {
if (to.meta.isAuth) {
//判断当前路由是否需要进行权限控制
if (localStorage.getItem("user") == "zhangsan") {
next();
}
alert("暂无权限");
} else {
next();
}
}
组件内路由守卫
//进入守卫:通过路由规则,进入该组件时调用
beforeRouteEnter(to,from,next){
},
//离开守卫,通过路由规则,离开该组件时调用
beforeRouteLeave(to,from,next){
}
history模式与hash模式
- 对于一个url来说,什么事hash值?——#及其后面的内容就是hash值
- hash值不会包含在HTTP请求中,即:hash值不会带给服务器
- hash模式:
(1)地址中永远带着#号,不美观。
(2)若以后将地址通过第三方手机App分享,若App校验严格,则地址会被标记为不合法。
(3)兼容性较好。- history模式:
(1)地址干净,美观。
(2)兼容性和hash模式相比略差。
(3)应用部署上线时需要后端人员支持,解决刷新页面服务器端404的问题。
const router = new VueRouter({
mode:"history",
routes: [],
});