单链表
package com.zhuang.lianbian;
public class Node {
public Node next=null;
public int data;
public Node(int data){
this.data=data;
}
@Override
public String toString() {
return "这个节点数据为:" +this.data;
}
}
package com.zhuang.lianbian;
public class LinkedList {
Node head =null;
public void addNode(int data){
Node node = new Node(data);
if(head==null){
head=node;
return;
}
Node temp = head;
while(temp.next!=null){
temp=temp.next;
}
temp.next=node;
}
public int length(){
int length = 0;
Node temp = head;
while(temp != null){
length++;
temp = temp.next;
}
return length;
}
public boolean deleteNode(int index){
if(index < 1 && index > length()){
return false;
}
if(index == 1){
head = head.next;
return true;
}
int i = 2;
Node preNode = head;
Node curNode = preNode.next;
while(curNode != null){
if(index == i){
preNode.next = curNode.next;
return true;
}
preNode = curNode;
curNode = curNode.next;
i++;
}
return true;
}
public void printList(){
Node temp = head;
System.out.print("链表顺序为:");
while(temp != null){
System.out.print(temp.data + "、");
temp = temp.next;
}
}
public Node orderList(){
Node curNode = head;
int temp;
while(curNode != null){
Node nextNode = curNode.next;
while(nextNode != null){
if(curNode.data > nextNode.data){
temp = curNode.data;
curNode.data = nextNode.data;
nextNode.data = temp;
}
nextNode = nextNode.next;
}
curNode = curNode.next;
}
return head;
}
public boolean addNode(int index , int data){
if(index < 1 && index > length()){
return false;
}
Node insertNode = new Node(data);
if(index == 1){
insertNode.next = head;
head = insertNode;
return true;
}
Node preNode = head;
Node curNode = head.next;
int i = 2;
while(curNode != null){
if(i == index){
preNode.next = insertNode;
insertNode.next = curNode;
return true;
}
preNode = curNode;
curNode = curNode.next;
i++;
}
return true;
}
public void deleteDuplecate(Node head){
Node p = head;
while(p != null){
Node q = p;
while(q.next != null){
if(p.data == q.next.data){
q.next = q.next.next;
}else
q=q.next;
}
p = p.next;
}
}
public Node findRevK(Node head,int k){
if(k<1 || k > length()){
return null;
}
Node p = head;
Node q = head;
for(int i=0;i<k-1;i++){
q = q.next;
}
while(q.next != null){
p = p.next;
q = q.next;
}
return p;
}
public Node findMedia(Node head){
Node fast = head;
Node slow = head;
while(fast != null && fast.next!=null&&fast.next.next!=null){
fast = fast.next.next;
slow = slow.next;
}
return slow;
}
public boolean isLoop(Node head){
Node fast = head;
Node slow = head;
if(fast == null){
return false;
}
while(fast != null && fast.next != null ){
fast = fast.next.next;
slow = slow.next;
if(fast == slow){
return true;
}
}
return !(fast==null || fast.next == null );
}
public Node findLoopPort(Node head){
Node slow = head;
Node fast = head;
if(slow == null){
return null;
}
while(fast != null && fast.next != null){
slow = slow .next;
fast = fast.next.next;
if(slow == fast){
Node firstMeet = slow;
int LoopLength = 1;
while(firstMeet.next != slow){
firstMeet = firstMeet.next;
LoopLength++;
}
slow = head;
fast = head;
for(int i = 0;i < LoopLength;i++){
fast = fast.next;
}
while(slow != fast){
slow = slow.next;
fast = fast.next;
}
return slow;
}
}
return null;
}
}
双链表
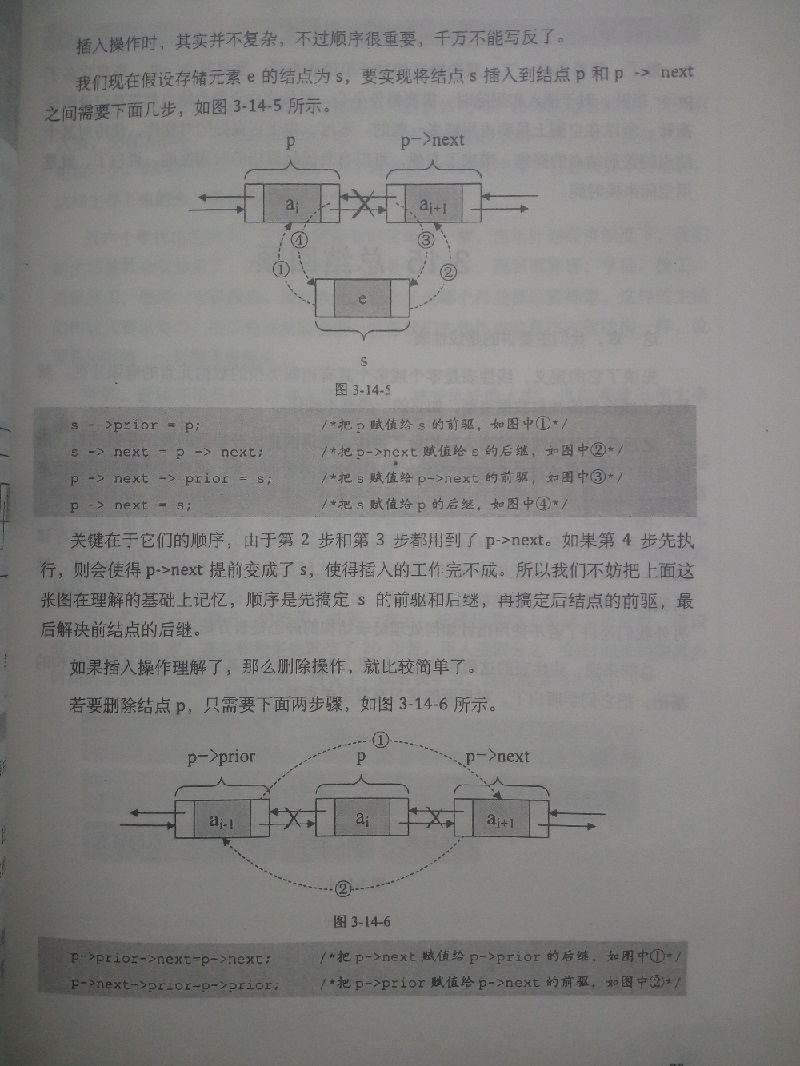
public class DoubleLink<T> {
private DNode<T> mHead;
private int mCount;
private class DNode<T> {
public DNode prev;
public DNode next;
public T value;
public DNode(T value) {
this.value = value;
}
}
public DoubleLink() {
mHead = new DNode<T>(null);
mHead.prev = mHead.next = mHead;
mCount = 0;
}
public int size() {
return mCount;
}
public boolean isEmpty() {
return mCount==0;
}
private DNode<T> getNode(int index) {
if (index<0 || index>=mCount)
throw new IndexOutOfBoundsException();
if (index <= mCount/2) {
DNode<T> node = mHead.next;
for (int i=0; i<index; i++)
node = node.next;
return node;
}
DNode<T> rnode = mHead.prev;
int rindex = mCount - index -1;
for (int j=0; j<rindex; j++)
rnode = rnode.prev;
return rnode;
}
public T get(int index) {
return getNode(index).value;
}
public T getFirst() {
return getNode(0).value;
}
public T getLast() {
return getNode(mCount-1).value;
}
public void insert(int index, T t) {
if (index==0) {
DNode<T> node = new DNode<T>(t);
node.next = mHead.next;
node.prev = mHead;
mHead.next.prev = node;
mHead.next = node;
mCount++;
return ;
}
DNode<T> inode = getNode(index);
DNode<T> tnode = new DNode<T>(t);
tnode.next = inode.next;
tnode.prev = inode;
inode.next.prev = tnode;
inode.next = tnode;
mCount++;
return ;
}
public void insertFirst(T t) {
insert(0, t);
}
public void appendLast(T t) {
DNode<T> node = new DNode<T>(t);
node.next = mHead;
node.prev = mHead.prev;
mHead.next = node;
mHead.prev.next = node;
mCount++;
}
public void del(int index) {
DNode<T> inode = getNode(index);
inode.prev.next = inode.next;
inode.next.prev = inode.prev;
inode = null;
mCount--;
}
public void deleteFirst() {
del(0);
}
public void deleteLast() {
del(mCount-1);
}
}