PHP微信公众号开发--上传素材
- 制作文件上传的表单html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>上传素材</title>
</head>
<body>
<form action="post.php" method="post" enctype="multipart/form-data">
<p>
素材类型:
<select name="is_forever">
<option value="0">临时</option>
<option value="1">永久</option>
</select>
<input type="file" name="media" id="">
<input type="submit" value="提交素材">
</p>
</form>
</body>
</html>
- 图片上传到微信服务器的接口编写
public function getAccessToken()
{
$mem = new Memcache();
$mem->connect('127.0.0.1', 11211);
$men_name = 'auth_'.self::APPID;
$value = $mem->get($men_name);
if ($value)
{
return $value;
}
$wechat_url = 'https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=%s&secret=%s';
$wechat_url = sprintf($wechat_url, self::APPID, self::APPSECRET);
$json_data = $this->http_request($wechat_url);
$arr = json_decode($json_data, True);
$access_token = $arr['access_token'];
$mem->add($men_name, $access_token, 0, 7200);
return $access_token;
}
private function http_request_post($url, $data, $file='')
{
if(!empty($file))
{
$data['media'] = new CURLFile($file);
}
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST,FALSE);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
$output = curl_exec($curl);
if (curl_errno($curl) > 0)
{
echo curl_error($curl);
$output = 'http请求出错!'.'['.curl_error($curl).']';
}
curl_close($curl);
return $output;
}
public function uploadMaterial(string $path, string $type,$is_forever = 0)
{
if ($is_forever == 0)
{
$upload_url = 'https://api.weixin.qq.com/cgi-bin/media/upload?access_token=%s&type=%s';
}else{
$upload_url = 'https://api.weixin.qq.com/cgi-bin/material/add_material?access_token=%s&type=%s';
}
$url = sprintf($upload_url, $this->getAccessToken(), $type);
$json = $this->http_request_post($url, [], $path);
$arr = json_decode($json, true);
return $arr['media_id'];
}
- 接收表单数据,实现素材上传到自己服务器。
include './index.php';
$files = $_FILES['media'];
$extname = pathinfo($files['name'], PATHINFO_EXTENSION);
$name = time().'.'.$extname;
$realpath = __DIR__.'/uploads/'.$name;
move_uploaded_file($files['tmp_name'], $realpath);
$wx = new WechatTest();
echo $wx->uploadMaterial($realpath, 'image', $_POST['is_forever']);
- 得到media_id

- 修改被动回复消息接口,测试素材是否上传成功
private function acceptMsg()
{
$xml = file_get_contents('php://input');
$obj = simplexml_load_string($xml,'SimpleXMLElement',LIBXML_NOCDATA);
$this->writeLog($xml, 1);
$msgType = $obj->MsgType;
$fun = $msgType.'Fun';
echo $ret = $this->$fun($obj);
$this->writeLog($ret,2);
}
private function textFun($obj)
{
$content = $obj->Content;
if ($content == '图片素材')
{
return $this->createImage($obj, '0JYo9-5eoRTtAbhckWX5-eFQgavkz7Yow4zlXkw4NmLwl-H0olDArdVqdesh9A_z');
}
$content = '公众号回复你:'.$content;
return $this->createText($obj, $content);
}
private function createImage($obj, $mediaid)
{
$xml = '<xml>
<ToUserName><![CDATA[%s]]></ToUserName>
<FromUserName><![CDATA[%s]]></FromUserName>
<CreateTime>%s</CreateTime>
<MsgType><![CDATA[image]]></MsgType>
<Image>
<MediaId><![CDATA[%s]]></MediaId>
</Image>
</xml>';
if($mediaid)
{
$str = sprintf($xml, $obj->FromUserName, $obj->ToUserName, time(), $mediaid);
}else{
$str = sprintf($xml, $obj->FromUserName, $obj->ToUserName, time(), $obj->MediaId);
}
return $str;
}
- 测试结果如下:
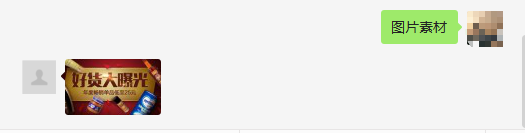