结构
继承关系
public class ViewSwitcher extends ViewAnimator
java.lang.Object
android.view.View
android.view.ViewGroup
android.widget.FrameLayout
android.widget.ViewAnimator
android.widget.ViewSwitcher
已知直接子类
ImageSwitcher, TextSwitcher
类概述
在两个视图间转换时显示动画,有一个可以创建这些视图的工厂类。你可以用工厂来创建这些视图,也可以自己创建。一个ViewSwitcher只允许包含两个子视图,且一次仅能显示一个。
(译者注:与ViewFlipper类相似,但该类不常用,常用其两个子类ImageSwitcher:转换图片时增加动画效果; TextSwitcher: 转换文字时增加动画效果; 其实例见apidemos中ImageSwitcher实例和TextSwitcher实例)
内部类
interface ViewSwitcher.ViewFactory
在一个ViewSwitcher里创建视图
构造函数
public ViewSwitcher (Context context)
构造一个新的空的视图转换器(ViewSwitcher)。
参数
context 应用环境(译者注:应用程序上下文)
public ViewSwitcher (Context context, AttributeSet attrs)
构造一个指定上下文、属性集合的空的视图转换器(ViewSwitcher)。
参数
context 应用环境(译者注:应用程序上下文)
attrs 属性集合
公共方法
public void addView(View child, int index, ViewGroup.LayoutParams params)
添加一个指定布局参数的子视图
参数
child 添加的子视图
index 添加的子视图的索引
params 子视图的布局参数
异常
IllegalStateException 如果切换器中已经包含了两个视图时。
public View getNextView ()
返回下一个要显示的视图
返回
视图切换之后将要显示出的下一个视图
public void reset ()
重置视图转换器(ViewSwitcher)来隐藏所有存在的视图,并使转换器达到一次动画都还没有播放的状态。
public void setFactory (ViewSwitcher.ViewFactory factory)
设置用来生成将在视图转换器中切换的两个视图的工厂。也可以调用两次 addView(android.view.View, int, android.view.ViewGroup.LayoutParams)来替代使用工厂的方法。
参数
factory 用来生成转换器内容的视图工厂
=================================================
案例:
定义两个xml文件
文件一:viewswitcher_one.xml内容如下:
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
02 | < Button xmlns:android = "http://schemas.android.com/apk/res/android" |
03 | android:id = "@+id/btn_loadmorecontacts" |
04 | android:text = "More..." |
05 | android:layout_width = "fill_parent" |
06 | android:layout_height = "wrap_content" |
07 | android:textAppearance = "?android:attr/textAppearanceLarge" |
08 | android:minHeight = "?android:attr/listPreferredItemHeight" |
09 | android:textColor = "#FFFFFF" |
10 | android:background = "@android:drawable/list_selector_background" |
11 | android:clickable = "true" |
12 | android:onClick = "onClick" /> |
文件一:viewswitcher_two.xml内容如下:
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
02 | < RelativeLayout xmlns:android = "http://schemas.android.com/apk/res/android" |
03 | android:layout_width = "wrap_content" |
04 | android:layout_height = "wrap_content" |
05 | android:gravity = "center_horizontal" |
06 | android:minHeight = "?android:attr/listPreferredItemHeight" > |
08 | android:id = "@+id/progressbar" |
09 | android:layout_width = "wrap_content" |
10 | android:layout_height = "wrap_content" |
11 | android:layout_centerVertical = "true" /> |
13 | android:text = "Loading…" |
14 | android:textAppearance = "?android:attr/textAppearanceLarge" |
15 | android:layout_height = "wrap_content" |
16 | android:layout_width = "wrap_content" |
17 | android:layout_toRightOf = "@+id/progressbar" |
18 | android:layout_centerVertical = "true" |
19 | android:gravity = "center" |
20 | android:padding = "10dip" |
21 | android:textColor = "#FFFFFF" /> |
三:之后就是java文件:
03 | import android.app.Activity; |
04 | import android.app.ListActivity; |
05 | import android.os.AsyncTask; |
06 | import android.os.Bundle; |
07 | import android.view.View; |
08 | import android.view.View.OnClickListener; |
09 | import android.view.Window; |
10 | import android.widget.ArrayAdapter; |
11 | import android.widget.Button; |
12 | import android.widget.ViewSwitcher; |
14 | public class ViewSwicherDemo_one extends ListActivity implements OnClickListener{ |
17 | static final String[] ITEMS = new String[] |
22 | private ViewSwitcher viewSwitcher; |
25 | protected void onCreate(Bundle savedInstanceState) { |
27 | super .onCreate(savedInstanceState); |
30 | requestWindowFeature(Window.FEATURE_NO_TITLE); |
32 | viewSwitcher = new ViewSwitcher( this ); |
34 | Button footer = (Button)View.inflate( this , R.layout.viewswitcher_one, null ); |
36 | View progress = View.inflate( this , R.layout.viewswitcher_two, null ); |
38 | viewSwitcher.addView(footer); |
39 | viewSwitcher.addView(progress); |
41 | getListView().addFooterView(viewSwitcher); |
70 | protected void onPostExecute(Object result) { |
72 | viewSwitcher.showPrevious(); |
四:执行后的效果如下:
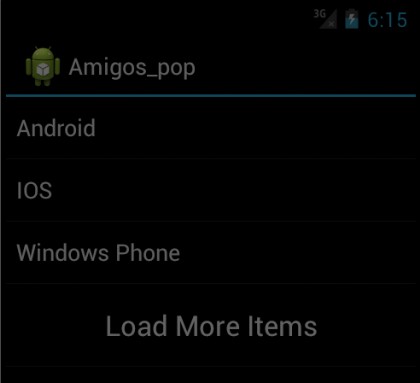
点击Load More Items之后:
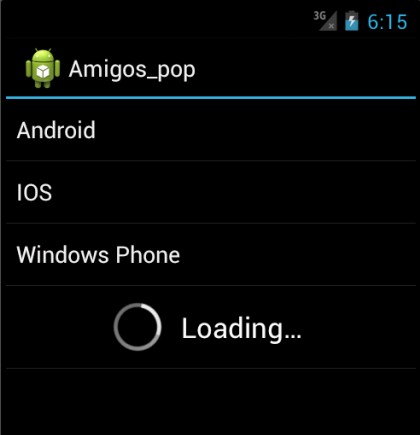
二:案例二
01 | <? xml version = "1.0" encoding = "utf-8" ?> |
02 | < LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android" |
03 | android:layout_width = "wrap_content" |
04 | android:layout_height = "wrap_content" |
05 | android:gravity = "center_horizontal" |
06 | android:minHeight = "?android:attr/listPreferredItemHeight" |
07 | android:orientation = "vertical" |
10 | android:id = "@+id/viewswitcher_button" |
11 | android:layout_width = "fill_parent" |
12 | android:inAnimation = "@android:anim/slide_in_left" |
13 | android:outAnimation = "@android:anim/slide_out_right" |
14 | android:layout_height = "wrap_content" > |
16 | android:src = "@drawable/android" |
18 | android:layout_width = "fill_parent" |
19 | android:layout_height = "wrap_content" > |
22 | android:src = "@drawable/blackberry" |
24 | android:layout_width = "fill_parent" |
25 | android:layout_height = "wrap_content" > |
29 | android:id = "@+id/btn_pre" |
31 | android:layout_width = "wrap_content" |
32 | android:layout_height = "wrap_content" |
35 | android:id = "@+id/btn_next" |
37 | android:layout_width = "wrap_content" |
38 | android:layout_height = "wrap_content" |
2.java代码:
02 | import android.app.Activity; |
03 | import android.os.Bundle; |
04 | import android.view.View; |
05 | import android.view.View.OnClickListener; |
06 | import android.widget.Button; |
07 | import android.widget.ViewSwitcher; |
08 | public class ViewSwicherDemo_Two extends Activity { |
09 | private ViewSwitcher viewSwitcher; |
10 | private Button btn_prev, btn_next; |
12 | protected void onCreate(Bundle savedInstanceState) |
15 | super .onCreate(savedInstanceState); |
16 | setContentView(R.layout.viewswitcher_three); |
18 | viewSwitcher = (ViewSwitcher)findViewById(R.id.viewswitcher_button); |
19 | btn_next = (Button) findViewById(R.id.btn_next); |
20 | btn_prev = (Button) findViewById(R.id.btn_pre); |
21 | viewSwitcher.setDisplayedChild( 0 ); |
23 | btn_prev.setOnClickListener(clickListener); |
24 | btn_next.setOnClickListener(clickListener); |
27 | private OnClickListener clickListener = new OnClickListener() { |
29 | public void onClick(View v) { |
33 | viewSwitcher.showPrevious(); |
36 | viewSwitcher.showNext(); |
3:执行效果:
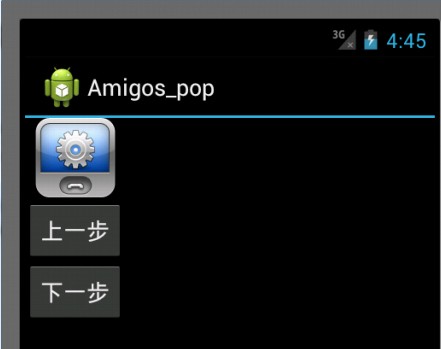
