版权声明:本文为 小异常 原创文章,非商用自由转载-保持署名-注明出处,谢谢!
本文网址:https://blog.csdn.net/sun8112133/article/details/80465584
还是老规矩,在学习新的知识点前,先去泡一泡 HelloWorld 这个大温泉,本文以一个众所周知的 HelloWorld 帮助大家快速打开 AOP 这扇大门。上一篇已经为大家讲解过了什么是 AOP,相信大家已经对它有了一个简单的认识,它就是在程序运行的时候动态的改变程序结构的一种技术,也是一种新的编程思想。
通过前几篇的博客,大家已经知道了配置 Spring 有两种方式,一种是基于 XML 方式,还有一种是基于 注解方式,接下来我们就以这两种方式分别写一个 HelloWorld。
本文大体可以分为三大部分:
一、AspectJ 与 Spring AOP 关系
二、HelloWorld(基于XML方式)
三、HelloWorld(基于注解方式)
一、AspectJ 与 Spring AOP 关系
网上大多数文章在讲到这里的时候很容易会给初学者带来误解,初始者们会认为 AspectJ 是 Spring AOP 中的一部分。其实不是这样的,AspectJ 是一个真正的面向切面的框架,它才是真正意义上的 AOP(AspectJ 简称 AOP),而 Spring 只是将 AspectJ 这种思想融入到了自己的世界里,经过加工变成了 Spring AOP。
二、HelloWorld(基于XML方式)
1、导入 jar包
com.springsource.org.aopalliance-1.0.0.jar
com.springsource.org.aspectj.weaver-1.6.8.RELEASE.jar
spring-aop-4.0.0.RELEASE.jar
commons-logging-1.1.1.jar
spring-beans-4.0.0.RELEASE.jar
spring-context-4.0.0.RELEASE.jar
spring-core-4.0.0.RELEASE.jar
spring-expression-4.0.0.RELEASE.jar
注:红字字样的是 新增 的 jar包。
jar包详解:
1)com.springsource.org.aopalliance-1.0.0.jar:(AOP联盟包)是 Spring AOP 的依赖包,里面包含了针对面向切面的接口,通常 Spring 等其它具备动态织入功能的框架依赖此包;
2)com.springsource.org.aspectj.weaver-1.6.8.RELEASE.jar:(AOP织入包)也是 Spring AOP 的依赖包,处理事务和 AOP 所需的包;
3)spring-aop-4.0.0.RELEASE.jar:(AOP包)这个 jar包 主要包含了 使用 Spring 的 AOP 特性时所需的类,还有一些基于AOP 的 Spring特性。
2、创建一个 HelloWorld 的类:
public class HelloWorld {
public void hello() {
System.out.println("Hello AOP!!");
}
}
3、创建一个 Time 的切面类:
public class Time {
// 在 hello() 方法前执行
public void startTime() {
System.out.println("计时开始~~~~~");
}
// 在 hello() 方法后执行
public void endTime() {
System.out.println("计时结束~~~~~");
}
}
4、配置 XML:
<!-- HelloWorld类的bean -->
<bean id="helloWorld" class="com.demo.HelloWorld"></bean>
<!-- Time类的bean -->
<bean id="time" class="com.demo.Time"></bean>
<!-- 配置 AOP -->
<aop:config>
<!-- 切面 -->
<aop:aspect ref="time">
<!-- 前置通知 -->
<aop:before method="startTime"
pointcut="execution(public * com.demo.HelloWorld.hello(..))" />
<!-- 后置通知 -->
<aop:after method="endTime"
pointcut="execution(public * com.demo.HelloWorld.hello(..))" />
</aop:aspect>
</aop:config>
注: 1)切面类和目标类先要在 IOC容器 中配置 bean;
2)再配置 AOP;
3)前置通知和后置通知的 method 属性指定 切面类中的 方法名;
4)pointcut 属性指定给哪个切入点设置 前置通知 和 后置通知,execution是执行的意思,*代表通用(以上代码中代表任意返回类型,但不能代表任意访问修饰符),..代表任意参数。
5、测试方法:
public static void main(String[] args) {
// 1、创建 Spring 的 IOC 容器对象
ApplicationContext ioc = new ClassPathXmlApplicationContext("beans.xml");
// 2、从 IOC 容器中获取 bean 实例
HelloWorld helloWorld = (HelloWorld) ioc.getBean("helloWorld");
// 3、调用 hello方法
helloWorld.hello();
}
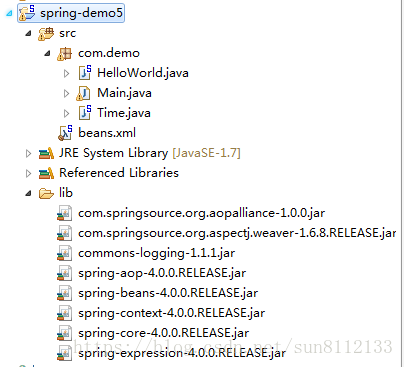
三、HelloWorld(基于注解方式)
1、导入 jar包
com.springsource.org.aopalliance-1.0.0.jar
com.springsource.org.aspectj.weaver-1.6.8.RELEASE.jar
spring-aop-4.0.0.RELEASE.jar
commons-logging-1.1.1.jar
spring-beans-4.0.0.RELEASE.jar
spring-context-4.0.0.RELEASE.jar
spring-core-4.0.0.RELEASE.jar
spring-expression-4.0.0.RELEASE.jar
2、创建一个 HelloWorld 的类:
@Component
public class HelloWorld {
public void hello() {
System.out.println("Hello AOP!!");
}
}
3、创建一个 Time 的切面类:
@Aspect
@Component
public class Time {
// 在 hello() 方法前执行
@Before("execution(public * com.demo.HelloWorld.hello(..))")
public void startTime() {
System.out.println("计时开始~~~~~");
}
// 在 hello() 方法后执行
@After("execution(public * com.demo.HelloWorld.hello(..))")
public void endTime() {
System.out.println("计时结束~~~~~");
}
}
4、配置 XML:
<!-- 扫描指定包中的注解,放入到 IOC 容器中 -->
<context:component-scan base-package="com.demo" />
<!-- 扫描 @aspect 注解 -->
<aop:aspectj-autoproxy />
5、测试方法:
public static void main(String[] args) {
// 1、创建 Spring 的 IOC 容器对象
ApplicationContext ioc = new ClassPathXmlApplicationContext("beans.xml");
// 2、从 IOC 容器中获取 bean 实例
HelloWorld helloWorld = (HelloWorld) ioc.getBean("helloWorld");
// 3、调用 hello方法
helloWorld.hello();
}