本文讨论在Spring 框架下各种注释的用法以及注意事项
1 创建一个简单的Springboot 工程, 包括一个controller 和 service
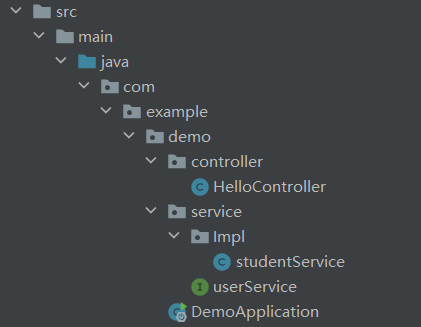
package com.example.demo.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@RequestMapping("/hello")
public String hello() {
return "hello";
}
}
package com.example.demo.service;
public interface userService{
void printService();
}
package com.example.demo.service.Impl;
import com.example.demo.service.userService;
import org.springframework.stereotype.Service;
@Service
public class studentService implements userService {
@Override
public void printService() {
System.out.println("student");
}
}
studentService 需要用 @Service 修饰, 普通的组件用 @component 修饰, 处于数据层的组件用 @Repository修饰
2 在controller 调用studentService, 会提示 "Field injection is not recommended"
package com.example.demo.controller;
import com.example.demo.service.userService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
// 字段变量
@Autowired
private userService studentService;
//-----------------------------------------
// 构造器的依赖注入
private final userService studentService;
public HelloController(userService studentService) {
this.studentService = studentService;
}
// ----------------------------------------
// Setter的依赖注入
private userService studentService;
@Autowired
public void setStudentService(userService studentService) {
this.studentService = studentService;
}
@RequestMapping("/hello")
public String hello() {
studentService.printService();
return "hello";
}
}
一共有3种依赖注入方式 基于构造器的依赖注入, 基于Setter的依赖注入, 和基于字段变量的依赖注入,所以可以通过构造器和Setter经行依赖注入
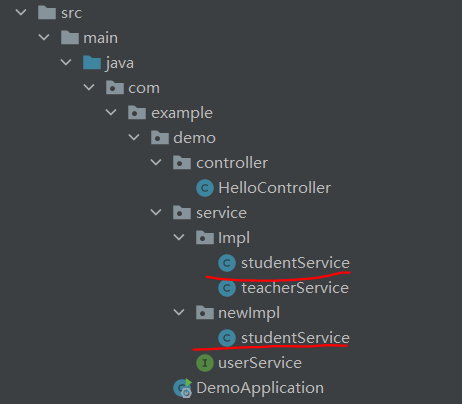
在这种情况下,有两个类都叫 studentService, 如果启动项目的话,会有报错
org.springframework.beans.factory.BeanDefinitionStoreException: Failed to parse configuration class [com.example.demo.DemoApplication]; nested exception is org.springframework.context.annotation.ConflictingBeanDefinitionException: Annotation-specified bean name 'studentService' for bean class [com.example.demo.service.newImpl.studentService] conflicts with existing, non-compatible bean definition of same name and class [com.example.demo.service.Impl.studentService]
在@Service后加上特定的名字 newStudentService
package com.example.demo.service.newImpl;
import com.example.demo.service.userService;
import org.springframework.stereotype.Service;
@Service("newStudentService")
public class studentService implements userService {
@Override
public void printService() {
System.out.println("student from new Impl");
}
}
在controller 里加上 @Qualifier 经行区分
@Autowired
@Qualifier("newStudentService")
private userService studentService;
//
private final userService studentService;
public HelloController(@Qualifier("newStudentService") userService studentService) {
this.studentService = studentService;
}
//
private userService studentService;
@Autowired
public void setStudentService(@Qualifier("newStudentService")userService studentService) {
this.studentService = studentService;
}