任务要求:
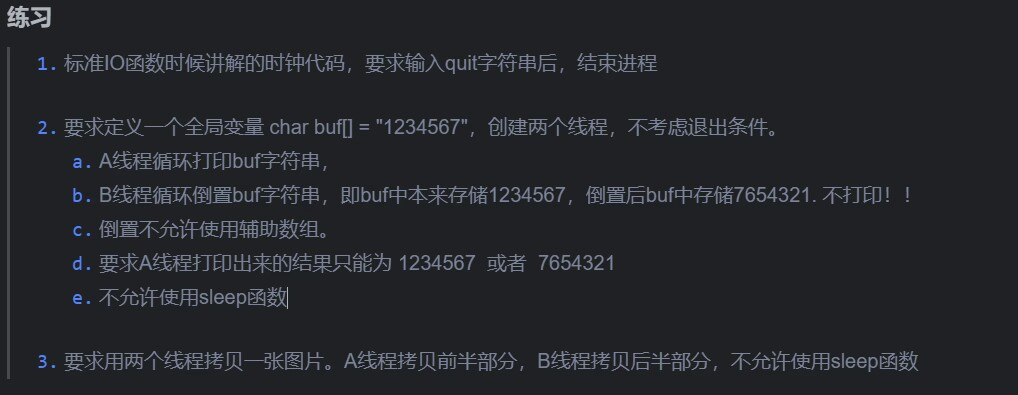
第一题代码如下
#define ERR_MSG(msg) {fprintf(stderr,"出错可能的行号%d\n",__LINE__);\
perror(msg);} //定义报错信息
#include<stdio.h>
#include<time.h>
#include<pthread.h>
#include<sys/stat.h>
#include<sys/types.h>
#include<unistd.h>
#include<string.h>
//子进程
void* Clock(void*t) //接受的时间结构体变量
{
struct tm*t1=t;
while(1)
{
printf("%4d年%2d月%2d日\n",t1->tm_year+1900,t1->tm_mon+1,t1->tm_mday);
sleep(1);
}
return NULL;
}
int main(int argc, const char *argv[])
{
//定义一个进程号
pthread_t No1;
time_t S =time(NULL); //获得到现在为止的时间变量,没问题
struct tm* t=localtime(&S);
if(NULL==t)
{
ERR_MSG("localtime");
return -1;
}
//printf("%4d年%2d月%2d日\n",(t->tm_year)+1900,(t->tm_mon)+1,t->tm_mday);
//时间结构体没有问题
//创建1号线程No1,用于显示时间
if(0!=(pthread_create(&No1,NULL,Clock,t)))
{
printf("可能出错的行号:%d\n",__LINE__);
return -1;
};
char key[20]="0";
while(1)
{
printf("输入信息\n");
sleep(1);
scanf("%s",key);
if(0==strcmp(key,"quit"))
{
if(0!=(pthread_cancel(No1))) //给No1发送中断标记
{
printf("错误可能行号%d\n",__LINE__);
};
printf("结束");
break; //测试过,不是因为break而结束的主线程
}
else
printf("指令错误\n");
}
// while()
//结束进程
return 0;
}
运行效果图如下:
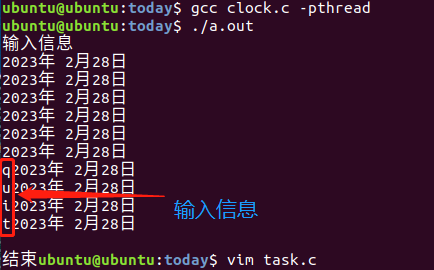
第二题代码如下
#include<stdio.h>
#include<string.h>
#include<pthread.h>
#include<stdlib.h>
#include<unistd.h>
//char arr[10]="1234567";//创一个全局变量数组
//倒置函数
void* Updata(void*b)
{
//找到数字指针头
char *a=(char*)b;
char arr;
int size=strlen(a);
//printf("数字为:%s\n",(arr+size-1));
printf("数据大小为:%d\n",size);
for(int i=0;i<(size/2);i++) //将数列倒置
{
arr=*(a+i); //arr接收头地址的数据
*(a+i)=*(a+(size-1-i)); //位地址数据覆盖到头地址
*(a+(size-1-i))=arr; //arr再去覆盖尾地址的数据
} //完成一次颠倒,循环(size/2)次
return NULL;
}
int main(int argc, const char *argv[])
{
//主程序创建一个
char arr[10]="1234567";
//输出函数
printf("改变前数组内容为%s\n",arr); //改变前
//创建子进程
pthread_t No1; //子进程1编号为No1
if(0!=pthread_create(&No1,NULL,Updata,arr)) //检验子程序1是否运行成功
{
printf("错误的行号可能为%d\n",__LINE__);
return -1;
};
//等待子函数回应,回应后再输出
printf("等待No1程序回应\n");
pthread_join(No1,NULL); //等待响应
printf("改变后的数组内容为:%s\n",arr); //再次输出
return 0;
}
运行效果图如下:
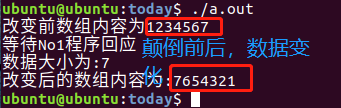
第三题代码如下:
#include<stdio.h>
#include<sys/stat.h>
#include<sys/types.h>
#include<unistd.h>
#include<pthread.h>
#include<string.h>
#include<fcntl.h>
void* copypng(void *fp)
{
int fpOut1=open("/home/ubuntu/图片/123",O_RDONLY); //以只读的形式打开文件
int fpIn1=open("./1.png",O_WRONLY|O_CREAT,0777); //写入追加的形式获取
struct stat getsize;
if(EOF==stat("/home/ubuntu/图片/123",&getsize))
{
perror("stat");
return NULL;
}
long int task1=0; //测试循环次数
char a;
off_t size1=getsize.st_size;
printf("No1线程中截获的文件大小为:%ld\n",size1); //获取文件大小没问题
off_t x=size1%2; //判断数据大小的奇偶性,避免数据丢失
lseek(fpOut1,(size1/2),SEEK_SET);
lseek(fpIn1,(size1/2),SEEK_SET);
for(int i=0 ;i<(size1/2+x);i++) //循环次数
{
if(EOF==read(fpOut1,&a,1))
{
perror("read");
fprintf(stderr,"错误可能行数为:%d\n",__LINE__);
return NULL;
};
if(EOF==write(fpIn1,&a,1))
{
perror("write");
fprintf(stderr,"错误可能行数为%d\n",__LINE__);
return NULL;
}
task1++;
}
printf("No1线程中循环搬运次数为:%ld\n",task1); //循环搬运校准
return NULL;
}
int main(int argc, const char *argv[])
{
int fpOut=open("/home/ubuntu/图片/123",O_RDONLY); //打开文件
if(EOF==fpOut)
{
perror("open");
return -1;
}
int fpIn=open("./1.png",O_WRONLY|O_CREAT,0777); //创建文件
if(EOF==fpIn)
{
perror("open");
return -1;
}
struct stat getsize; //获取文件的相关信息的变量
if(EOF==stat("/home/ubuntu/图片/123",&getsize))
{
perror("stat");
return -1;
};
long int task=0; //测试循环次数
off_t size=getsize.st_size; //获取文件大小
printf("主线程中的文件大小为:%ld\n",size);
//创建进程No1
pthread_t No1;
if(0!=pthread_create(&No1,NULL,copypng,NULL))
{
fprintf(stderr,"错误行数可能为%d\n",__LINE__);
return -1;
};
//主线程进入搬运工作,搬运前先偏移指针
lseek(fpIn,0,SEEK_SET);
lseek(fpOut,0,SEEK_SET);
char x;
for(int i=0;i<size/2;i++) //一个字符一个字符的搬运,文件大小为搬运次数
{
if(EOF==read(fpOut,&x,1)) //取出信息
{
perror("read");
return -1;
}
if(EOF==write(fpIn,&x,1)) //放入信息
{
perror("write");
return -1;
}
task++; //搬运次数累加
}
printf("主线程搬运次数%ld\n",task); //搬运次数校准
pthread_join(No1,NULL); //No1线程也执行完毕就退出
return 0;
}
效果图:
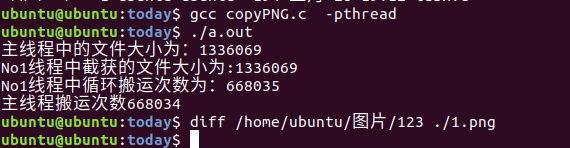