/*
Copyright (c)2017,烟台大学计算机与控制工程学院
All rights reserved.
文件名称:5.cpp
作 者:尚文哲
完成日期:2017年9月17日
问题描述: 领会“0207将算法变程序”部分建议的方法,建设自己的专业基础设施算法库。
这一周,建的是顺序表的算法库。算法库包括两个文件:
1.头文件:list.h,包含定义顺序表数据结构的代码、宏定义、要实现算法的函数的声明;
2.源文件:list.cpp,包含实现各种算法的函数的定义
请采用程序的多文件组织形式,在项目1的基础上,建立如上的两个文件,另外再建立一个源文件,
编制main函数,完成相关的测试工作。请完成后,发布博文,展示你的实践成果。
输入描述: 若干数据 。
程序输出: 1.线性表的长度。
2.第3个元素及其值的大小。
3.8在表中的第几位。
4.删除的元素。
5.删除后的线性表。
6.删除线性表。
*/
#include<stdio.h>
#include<malloc.h>
#define MaxSize 50
typedef int ElemType;
typedef struct
{
ElemType data[MaxSize];
int length;
}SqList;
void CreateList(SqList *&L, ElemType a[], int n);
void DispList(SqList *L);
bool ListEmpty(SqList *L);
int ListLength(SqList *L);
bool GetElem(SqList*L,int i,ElemType &e);
int LocateElem(SqList *L, ElemType e);
int ListLength(SqList *L)
{
return (L->length);
}
bool GetElem(SqList*L,int i,ElemType &e)
{
if(i<1||i>L->length)
return false;
e=L->data[i-1];
return true;
}
int LocateElem(SqList *L, ElemType e)
{
int i=0;
while (i<L->length && L->data[i]!=e) i++;
if (i>=L->length)
return 0;
else
return i+1;
}
void CreateList(SqList *&L,ElemType a[],int n)
{
int i;
L=(SqList *)malloc(sizeof(SqList));
for(i=0;i<n;i++)
L->data[i]=a[i];
L->length=n;
}
void DispList(SqList *L)
{
int i;
if (ListEmpty(L))
return;
for (i=0; i<L->length; i++)
printf("%d ",L->data[i]);
printf("\n");
}
bool ListEmpty(SqList *L)
{
return (L->length==0);
}
void InitList(SqList *&L);
void DestroyList(SqList *&L);
bool ListInsert(SqList *&L,int i,ElemType e);
bool ListDelete(SqList *&L,int i,ElemType &e);
void InitList(SqList *&L)
{
L=(SqList *)malloc(sizeof(SqList));
L->length=0;
}
void DestroyList(SqList *&L)
{
free(L);
}
bool ListInsert(SqList *&L,int i,ElemType e)
{
int j;
if(i<1||i>L->length+1)
return false;
i--;
for(j=L->length;j>i;j--)
L->data[j]=L->data[j-1];
L->data[i]=e;
L->length++;
return true;
}
bool ListDelete(SqList *&L,int i,ElemType e)
{
int j;
if(i<1||i>L->length+1)
return false;
i--;
e=L->data[i];
for(j=i;j<L->length-1;j++)
L->data[j]=L->data[j+1];
L->length--;
return true;
}
int main()
{
SqList *sq;
InitList(sq);
printf("构造的新的:\n");
DispList(sq);
ListInsert(sq, 1, 5);
ListInsert(sq, 2, 3);
ListInsert(sq, 1, 4);
printf("插入后的:\n");
DispList(sq);
ListDelete(sq, 1, 5);
ListDelete(sq, 2, 3);
printf("删除后的:\n");
DispList(sq);
DestroyList(sq);
printf("销毁后的:\n");
DispList(sq);
return 0;
}
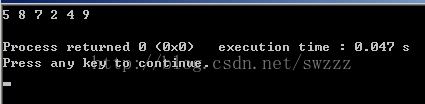
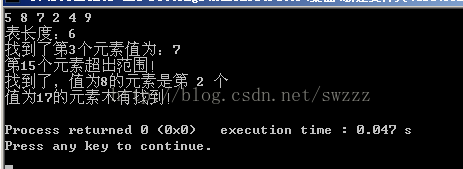
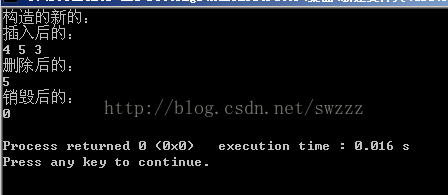