摘要:在这篇文章中,我们将看到如何删除非空的目录/文件夹。您可以使用java。文件的删除文件夹,但是如果它不是空的,你不能删除它。
有多种方法可以做到这一点:
- Using java recursion(递归)
- Using Apache common IO
1.Using java recursion(递归)
做这件事是非常直接的。它将遍历文件夹中的所有文件。如果它是一个文件,那么我们可以直接删除它,但是如果它在里面找到了文件夹,那么我们再次调用相同的方法。
// Delete using recursion(递归)
public static void delete(File file) throws IOException {
if (file.isDirectory()) {
// We can directly delete if we found empty directory
if (file.list().length == 0) {
file.delete();
System.out.println("Deleting folder : " + file.getAbsolutePath());
} else {
// list all the files in directly
File[] files = file.listFiles();
for (File temp : files) {
// recursive delete
delete(temp);
}
// Check directory again, if we find it empty, delete it
if (file.list().length == 0) {
file.delete();
System.out.println("Deleting folder : " + file.getAbsolutePath());
}
}
} else {
// if file, then we can directly delete it
file.delete();
System.out.println("Deleting file : " + file.getAbsolutePath());
}
}
2.Using Apache common IO:
删除非空文件夹是非常直接的。您只需要调用fileutils.deletedirectory()方法即可。
// Delete using Apache common IO
public static void deleteUsingApacheIO(File file) {
try {
FileUtils.deleteDirectory(file);
} catch (IOException e) {
e.printStackTrace();
}
}
3.Java Program :
package cn.micai.io;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
/**
* 描述:How to delete non empty directory in java
* <p>
*
* @author: 赵新国
* @date: 2018/6/7 10:54
*/
public class DeleteNonEmptyDirectoryMain {
public static void main(String [] args) {
System.out.println("Deleting using recursion");
System.out.println("---------------");
File folder= new File("D://workspace_spring_1");
// Using recusrion
try {
delete(folder);
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("---------------");
System.out.println("Deleting using Apache IO");
File folder2= new File("D://workspace_spring_2");
deleteUsingApacheIO(folder2);
System.out.println("Deleting folder : " + folder2.getAbsolutePath());
}
// Delete using recursion(递归)
public static void delete(File file) throws IOException {
if (file.isDirectory()) {
// We can directly delete if we found empty directory
if (file.list().length == 0) {
file.delete();
System.out.println("Deleting folder : " + file.getAbsolutePath());
} else {
// list all the files in directly
File[] files = file.listFiles();
for (File temp : files) {
// recursive delete
delete(temp);
}
// Check directory again, if we find it empty, delete it
if (file.list().length == 0) {
file.delete();
System.out.println("Deleting folder : " + file.getAbsolutePath());
}
}
} else {
// if file, then we can directly delete it
file.delete();
System.out.println("Deleting file : " + file.getAbsolutePath());
}
}
// Delete using Apache common IO
public static void deleteUsingApacheIO(File file) {
try {
FileUtils.deleteDirectory(file);
} catch (IOException e) {
e.printStackTrace();
}
}
}
4.当我运行程序时,我得到了输出:
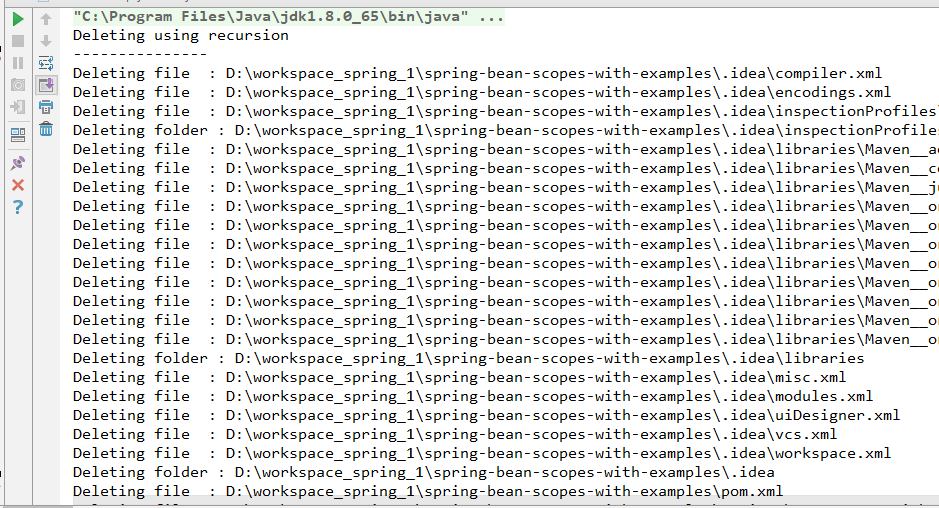