封装运动函数第一版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<p> </p>
<script>
/*
运动函数第一版
问题: 封装哪一段代码 ?
=> 封装的就是事件内的一段代码
问题: 封装的函数需要几个参数 ?
=> 让谁动 ?
=> 动哪一个样式 ?
=> 动到哪一个位置 ?
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// 调用运动函数
move(ele, 'left', 200)
// 定义一个初始值
// let num = 0
// // 定时器
// const timer = setInterval(() => {
// // 随着定时器 num 增长
// num += 5
// // 给元素赋值
// ele.style.left = num + 'px'
// // 当到达目标位置的时候, 停止
// if (num === 200) {
// clearInterval(timer)
// }
// }, 10)
}
// 获取元素
const p = document.querySelector('p')
// 通过事件触发
p.onclick = function () {
move(p, 'left', 300)
// 定义一个初始值
// let num = 0
// // 定时器
// const timer = setInterval(() => {
// // 随着定时器 num 增长
// num += 5
// // 给元素赋值
// p.style.left = num + 'px'
// // 当到达目标位置的时候, 停止
// if (num === 300) {
// clearInterval(timer)
// }
// }, 10)
}
// 封装
function move(ele, type, target) {
// ele 表示哪一个元素移动
// type 表示哪一个样式移动
// target 表示目标位置
// 定义一个初始值
let num = 0
// 定时器
const timer = setInterval(() => {
// 随着定时器 num 增长
num += 5
// 给元素赋值
// 涉及变量相关的时候, 必须要使用 数组关联语法
ele.style[type] = num + 'px'
// 当到达目标位置的时候, 停止
if (num === target) {
clearInterval(timer)
}
}, 10)
}
</script>
</body>
</html>
效果图;
--------->
封装运动函数第二版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 100px;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数第二版
问题: 如果我的开始和目标之差不是 5 的倍数 ?
=> 没有办法到达指定目标位置
=> 解决方案1: 每次+1
问题: 如果我的开始不是 0 ?
=> 会有从 0 开始的效果
=> 解决方案1: 把初始值设置成元素本身的位置
问题: 如果我同时运动两个样式 ?
=> 当两个方向上距离不一致的时候, 会导致出现不希望的运动轨迹
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// 调用运动函数
move(ele, 'left', 201)
move(ele, 'top', 501)
}
// 封装
function move(ele, type, target) {
// 解决问题2: 不把初始值设置为 0 了
// 设置成元素本身的位置
// 如何拿到元素本身的位置 ? 元素非行内样式
let num = parseInt(window.getComputedStyle(ele)[type])
const timer = setInterval(() => {
num += 1
ele.style[type] = num + 'px'
if (num === target) {
clearInterval(timer)
}
}, 10)
}
</script>
</body>
</html>
效果图:
封装运动函数第三版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 100px;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数最终版
问题: 如果我同时运动两个样式 ?
=> 当两个方向上距离不一致的时候, 会导致出现不希望的运动轨迹
解决:
=> 出现问题的原因是 速度一致
=> 解决的方案就是需要 速度不一致
=> 因为时间必须要一致
=> 需要运动的距离不一致
方案1:
=> 每一次运动总路程的 十分之一
方案2:
=> 需要走出一个 减速运动
=> 不能走总路程的 十分之一
=> 需要走 剩余路程 的 十分之一
问题: 不能到达目标位置 ?
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// 调用运动函数
move(ele, 'left', 200)
move(ele, 'top', 500)
}
// 封装
function move(ele, type, target) {
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = parseInt(window.getComputedStyle(ele)[type])
// 2. 计算本次距离
let distance = (target - current) / 10
// 3. 判断 结束 还是 赋值
if (current === target) {
// 到位
clearInterval(timer)
return
}
// 没到位
// 赋值为 当前位置 + 本次距离
ele.style[type] = current + distance + 'px'
}, 10)
}
/*
let num = parseInt(window.getComputedStyle(ele)[type])
const timer = setInterval(() => {
// speed 算出来的是本次应该运动的距离
let speed = (target - num) / 10
// 赋值的时候, 应该用当前位置 + 本次的距离
// 你赋值以后, 你的位置变了, 但是 num 没有变
ele.style[type] = num + speed + 'px'
console.log(speed)
if (parseInt(window.getComputedStyle(ele)[type]) === target) {
clearInterval(timer)
}
}, 10)
*/
</script>
</body>
</html>
效果图: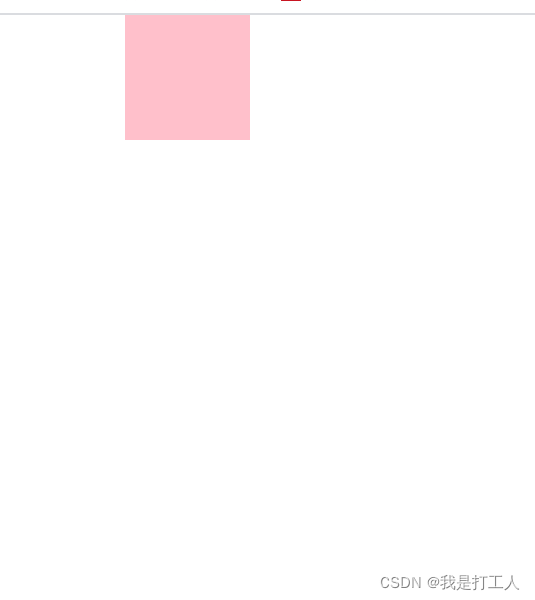
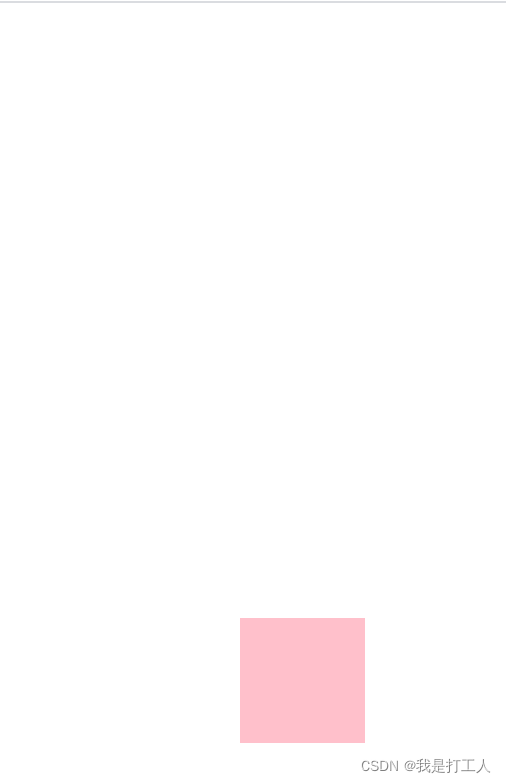
运动函数第四版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数最终版
问题: 不能到达目标位置 ?
分析问题:
+ 随着减速运动, 一定能有一个情况是在剩余距离不到 10, 是 9
+ 计算本次运动距离就是 0.9
+ 赋值的时候, 就是 191 + 0.9 赋值为 191.9
+ 因为浏览器描述不了不到 1px 的位置, 所以浏览器把元素还是放在 191 的位置
+ 你下次拿到的剩余距离还是 9
+ 计算出的运动距离还是 0.9
+ 赋值的时候, 还是 191 + 0.9 赋值为 191.9
解决问题:
+ 不足 1px 的向上进位到 1px
+ 向上取整
问题: 如果我做负方向运动 ?
分析问题
+ 因为当你值是负数的时候, 你有可能会拿到剩余距离是 -9px
+ 计算出的本次运动距离就是 -0.9 px
+ 因为向上取整, 导致为 0 本次运动距离是 0
+ 下一次拿到的剩余距离还是 -9px
解决问题:
+ 向下取整
+ 因为正方向运动需要向上取整
+ 判断, 如果 小于0 就向下取整, 如果大于0 就向上取整
问题: 我想运动一个 opacity ?
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// 调用运动函数
// move(ele, 'left', 203)
move(ele, 'opacity', 0)
// move(ele, 'top', 500)
}
// 封装
function move(ele, type, target) {
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = parseInt(window.getComputedStyle(ele)[type])
// 2. 计算本次距离
let distance = (target - current) / 10
distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
console.log(distance)
// 3. 判断 结束 还是 赋值
if (current === target) {
// 到位
clearInterval(timer)
return
}
// 没到位
// 赋值为 当前位置 + 本次距离
ele.style[type] = current + distance + 'px'
}, 10)
}
/*
let num = parseInt(window.getComputedStyle(ele)[type])
const timer = setInterval(() => {
// speed 算出来的是本次应该运动的距离
let speed = (target - num) / 10
// 赋值的时候, 应该用当前位置 + 本次的距离
// 你赋值以后, 你的位置变了, 但是 num 没有变
ele.style[type] = num + speed + 'px'
console.log(speed)
if (parseInt(window.getComputedStyle(ele)[type]) === target) {
clearInterval(timer)
}
}, 10)
*/
</script>
</body>
</html>
效果图:
封装函数第五版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
cursor: pointer;
opacity: 0.1;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数最终版
问题: 我想运动一个 opacity ?
分析原因:
+ opacity 的取值是 0 ~ 1 之间
=> 一定会有 0.xxx 的时候
=> 因为你的 parseInt() 直接变成 0
+ 因为取整
=> 要么计算出的本次距离是 1 要么是 -1
+ 赋值的时候
=> 你加入了 px 为单位
=> 但是 opacity 是没有单位的值
解决取整问题:
+ 获取值的时候放大 100倍
+ 按照 0 ~ 100 进行计算
+ 最后赋值的时候缩小 100倍
问题: 从 1 到 0 运动 opacity, 当你从 0 ~ 1 运动 opacity 的时候 ?
+ 不能实现运动了
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// move(ele, 'left', 203)
move(ele, 'opacity', 1)
}
// 封装
function move(ele, type, target) {
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = 0
if (type === 'opacity') {
// opacity 获取值的时候, 放大 100 倍
current = window.getComputedStyle(ele)[type] * 100
} else {
current = parseInt(window.getComputedStyle(ele)[type])
}
// 2. 计算本次距离
let distance = (target - current) / 10
distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
console.log(distance)
// 3. 判断 结束 还是 赋值
// 到位
if (current === target) {
clearInterval(timer)
return
}
// 没到位
if (type === 'opacity') {
// opacity 赋值的时候 缩小 100 倍
ele.style[type] = (current + distance) / 100
} else {
ele.style[type] = current + distance + 'px'
}
}, 300)
}
</script>
</body>
</html>
封装函数第六版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
cursor: pointer;
opacity: 0.1;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数最终版
问题: 从 1 到 0 运动 opacity, 当你从 0 ~ 1 运动 opacity 的时候 ?
+ 不能实现运动了
分析问题:
+ 你计算的时候, 取值范围是 0 ~ 100 进行计算
+ 你调用 move 函数设置 opacity 的时候, 写的是 1
+ 你是按照 0 ~ 1 的区间进行设置, 按照 0 ~ 100 的区间进行计算赋值
解决:
+ 方案1: 你在调用 move 按照的时候, 按照 0 ~ 100 的区间进行赋值
+ 方案2: 你在调用 move 的时候, 还是按照 0 ~ 1 进行赋值
=> 我再 move 函数的一开始, 判断一下, 如果你设置的是 opacity 我来帮你放大 100 倍
问题: 需要同时运动 多个样式 的时候 ?
+ 不能一个 运动函数 运动多个样式
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
// move(ele, 'left', 203)
move(ele, 'opacity', 0.96)
}
// 封装
function move(ele, type, target) {
// 判断 opacity
if (type === 'opacity') target *= 100
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = 0
if (type === 'opacity') {
// opacity 获取值的时候, 放大 100 倍
current = window.getComputedStyle(ele)[type] * 100
} else {
current = parseInt(window.getComputedStyle(ele)[type])
}
// 2. 计算本次距离
let distance = (target - current) / 10
distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// 3. 判断 结束 还是 赋值
// 到位
if (current === target) {
clearInterval(timer)
return
}
// 没到位
if (type === 'opacity') {
// opacity 赋值的时候 缩小 100 倍
ele.style[type] = (current + distance) / 100
} else {
ele.style[type] = current + distance + 'px'
}
}, 10)
}
</script>
</body>
</html>
封装函数第七版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
cursor: pointer;
opacity: 1;
}
p {
width: 100px;
height: 100px;
background-color: skyblue;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<script>
/*
运动函数最终版
问题: 需要同时运动 多个样式 的时候 ?
+ 不能一个 运动函数 运动多个样式
解决: 封装一个多属性运动函数
问题: 如何设计函数的参数 ?
+ 方案1: function move(元素, 样式1, 值1, 样式2, 值2, 样式3, 值3, ...) {}
+ 方案2: function move(元素, { 样式名: 样式值, 样式名: 样式值, ... })
问题: 我想在运动结束做一些事情 ?
+ 运动完全结束以后, 开始实现一些效果, 会出现多次
*/
// 获取元素
const ele = document.querySelector('div')
// 通过事件触发
ele.onclick = function () {
move(ele, { left: 200, top: 303, width: 400, height: 302 })
}
// 封装多属性运动函数
function move(ele, options) {
// ele 表示要运动的元素
// options 表示要运动的所有样式
// options 内有多少个样式, 开启多少个定时器
// 循环遍历 opaions, 循环一次开启一个定时器
for (let k in options) {
// 在循环内, k 每一个 样式名, options[k] 是每一个目标值
const type = k
const target = options[k]
// 判断 opacity
if (type === 'opacity') target *= 100
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = 0
if (type === 'opacity') {
// opacity 获取值的时候, 放大 100 倍
current = window.getComputedStyle(ele)[type] * 100
} else {
current = parseInt(window.getComputedStyle(ele)[type])
}
// 2. 计算本次距离
let distance = (target - current) / 10
distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// 3. 判断 结束 还是 赋值
// 到位
if (current === target) {
clearInterval(timer)
console.log('运动结束了')
return
}
// 没到位
if (type === 'opacity') {
// opacity 赋值的时候 缩小 100 倍
ele.style[type] = (current + distance) / 100
} else {
ele.style[type] = current + distance + 'px'
}
}, 20)
}
}
// 封装
// function move(ele, type, target) {
// // 判断 opacity
// if (type === 'opacity') target *= 100
// const timer = setInterval(() => {
// // 1. 拿到当前位置
// let current = 0
// if (type === 'opacity') {
// // opacity 获取值的时候, 放大 100 倍
// current = window.getComputedStyle(ele)[type] * 100
// } else {
// current = parseInt(window.getComputedStyle(ele)[type])
// }
// // 2. 计算本次距离
// let distance = (target - current) / 10
// distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// // 3. 判断 结束 还是 赋值
// // 到位
// if (current === target) {
// clearInterval(timer)
// return
// }
// // 没到位
// if (type === 'opacity') {
// // opacity 赋值的时候 缩小 100 倍
// ele.style[type] = (current + distance) / 100
// } else {
// ele.style[type] = current + distance + 'px'
// }
// }, 10)
// }
</script>
</body>
</html>
封装函数第八版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
cursor: pointer;
opacity: 1;
}
p {
width: 100px;
height: 100px;
background-color: orange;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<p> </p>
<script>
/*
运动函数最终版
问题: 我想在运动结束做一些事情 ?
+ 运动完全结束以后, 开始实现一些效果, 会出现多次
解决:
+ 不管你开启了多少个定时器, 当最后一个定时器结束的时候, 才算运动完全结束
问题: 如何知道最后一个定时器结束了 ?
+ 计数器
+ 你开一个定时器 计数器++
+ 关一个定时器 计数器--
+ 当计数器为 0 的时候, 所有定时器都关闭了
问题: 如果我使用运动函数运动多个元素 ?
+ 我希望一号元素运动完毕以后改变背景颜色
+ 我希望二号元素运动完毕以后浏览器弹出层提示
+ 在不同的运动结束之后做不同的事情
*/
// 获取元素
const ele = document.querySelector('div')
const p = document.querySelector('p')
// 通过事件触发
ele.onclick = function () {
move(ele, { left: 200, top: 303, width: 400, height: 302 })
}
p.onclick = function () {
move(p, { top: 300 })
}
// 封装多属性运动函数
function move(ele, options) {
// 准备一个变量当做 计数器
let count = 0
for (let k in options) {
// 随着循环, 每循环一次, 就会开启一个定时器
count++
const type = k
const target = options[k]
// 判断 opacity
if (type === 'opacity') target *= 100
const timer = setInterval(() => {
// 1. 拿到当前位置
let current = 0
if (type === 'opacity') {
current = window.getComputedStyle(ele)[type] * 100
} else {
current = parseInt(window.getComputedStyle(ele)[type])
}
// 2. 计算本次距离
let distance = (target - current) / 10
distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// 3. 判断 结束 还是 赋值
// 到位
if (current === target) {
clearInterval(timer)
// 这里的代码每执行一次, 定时间关闭一个
count--
if (count === 0) ele.style.backgroundColor = 'skyblue'
return
}
// 没到位
if (type === 'opacity') {
ele.style[type] = (current + distance) / 100
} else {
ele.style[type] = current + distance + 'px'
}
}, 20)
}
}
// 封装
// function move(ele, type, target) {
// // 判断 opacity
// if (type === 'opacity') target *= 100
// const timer = setInterval(() => {
// // 1. 拿到当前位置
// let current = 0
// if (type === 'opacity') {
// // opacity 获取值的时候, 放大 100 倍
// current = window.getComputedStyle(ele)[type] * 100
// } else {
// current = parseInt(window.getComputedStyle(ele)[type])
// }
// // 2. 计算本次距离
// let distance = (target - current) / 10
// distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// // 3. 判断 结束 还是 赋值
// // 到位
// if (current === target) {
// clearInterval(timer)
// return
// }
// // 没到位
// if (type === 'opacity') {
// // opacity 赋值的时候 缩小 100 倍
// ele.style[type] = (current + distance) / 100
// } else {
// ele.style[type] = current + distance + 'px'
// }
// }, 10)
// }
</script>
</body>
</html>
效果图:
封装函数第九版
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
div {
width: 100px;
height: 100px;
background-color: pink;
position: absolute;
left: 99px;
cursor: pointer;
opacity: 1;
}
p {
width: 100px;
height: 100px;
background-color: orange;
position: absolute;
top: 130px;
}
</style>
</head>
<body>
<div></div>
<p> </p>
<script src="../utils.js"></script>
<script>
/*
运动函数最终版
问题: 如果我使用运动函数运动多个元素 ?
+ 我希望一号元素运动完毕以后改变背景颜色
+ 我希望二号元素运动完毕以后浏览器弹出层提示
+ 在不同的运动结束之后做不同的事情
解决问题: 回调函数
+ 你把你想在最后做的事情放在一个 "盒子" 里面
+ 传递给 move 函数, 告诉 move 函数, 在运动结束以后, 把 "盒子" 内的代码执行一遍
+ 可以装代码的盒子 --- 函数
*/
// 获取元素
const ele = document.querySelector('div')
const p = document.querySelector('p')
// 通过事件触发
ele.onclick = function () {
move(ele, { left: 200, top: 303, width: 400, height: 302 }, function () {
ele.style.backgroundColor = 'skyblue'
})
}
p.onclick = function () {
move(p, { top: 300 }, () => {
alert('运动结束了')
})
}
// 封装多属性运动函数
// function move(ele, options, cb = function () {}) {
// // cb 需要在运动结束执行的函数
// let count = 0
// for (let k in options) {
// count++
// const type = k
// const target = options[k]
// if (type === 'opacity') target *= 100
// const timer = setInterval(() => {
// // 1. 拿到当前位置
// let current = 0
// if (type === 'opacity') {
// current = window.getComputedStyle(ele)[type] * 100
// } else {
// current = parseInt(window.getComputedStyle(ele)[type])
// }
// // 2. 计算本次距离
// let distance = (target - current) / 10
// distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// // 3. 判断 结束 还是 赋值
// // 到位
// if (current === target) {
// clearInterval(timer)
// // 这里的代码每执行一次, 定时间关闭一个
// count--
// // 在运动完全结束以后, 调用函数
// if (count === 0) cb()
// return
// }
// // 没到位
// if (type === 'opacity') {
// ele.style[type] = (current + distance) / 100
// } else {
// ele.style[type] = current + distance + 'px'
// }
// }, 20)
// }
// }
// 封装
// function move(ele, type, target) {
// // 判断 opacity
// if (type === 'opacity') target *= 100
// const timer = setInterval(() => {
// // 1. 拿到当前位置
// let current = 0
// if (type === 'opacity') {
// // opacity 获取值的时候, 放大 100 倍
// current = window.getComputedStyle(ele)[type] * 100
// } else {
// current = parseInt(window.getComputedStyle(ele)[type])
// }
// // 2. 计算本次距离
// let distance = (target - current) / 10
// distance = distance < 0 ? Math.floor(distance) : Math.ceil(distance)
// // 3. 判断 结束 还是 赋值
// // 到位
// if (current === target) {
// clearInterval(timer)
// return
// }
// // 没到位
// if (type === 'opacity') {
// // opacity 赋值的时候 缩小 100 倍
// ele.style[type] = (current + distance) / 100
// } else {
// ele.style[type] = current + distance + 'px'
// }
// }, 10)
// }
</script>
</body>
</html>