const formatterCN = new Intl.DateTimeFormat("zh-CN", {
timeZone: "Asia/Shanghai",
year: "numeric",
month: "2-digit",
day: "2-digit",
hour: "2-digit",
minute: "2-digit",
second: "2-digit"
});
export function expirationDate(d) {
if (d.toString().length > 10) {
return formatterCN
.format(d)
.replace(/\//g, "-")
.substring(0, 10);
} else {
return d;
}
}
// 今年第一季度
export function getFirstQuarter() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() + "-01-01";
// 获取结束日期
const endDate = today.getFullYear() + "-03-31";
return {
startDate, // 2023/1/1
endDate // 2023/3/31
};
}
// 今年第二季度
export function getSecondQuarter() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() + "-04-01";
// 获取结束日期
const endDate = today.getFullYear() + "-06-30";
return {
startDate, // 2023/1/1
endDate // 2023/3/31
};
}
// 今年第三季度
export function getThirdQuarter() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() + "-07-01";
// 获取结束日期
const endDate = today.getFullYear() + "-09-30";
return {
startDate, // 2023/1/1
endDate // 2023/3/31
};
}
// 今年第四季度
export function getFourthQuarter() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() + "-10-01";
// 获取结束日期
const endDate = today.getFullYear() + "-12-31";
return {
startDate, // 2023/1/1
endDate // 2023/3/31
};
}
// 昨天and明天
export function getYestDayOrNextDay(flag = true) {
// 获取当前日期
const today = new Date();
// 计算前一天的日期
const yesterday = new Date(today);
if (flag) {
yesterday.setDate(today.getDate() - 1);
} else {
yesterday.setDate(today.getDate() + 1);
}
return expirationDate(yesterday);
}
// console.log(getYestDayOrNextDay()); // 2023/8/5 昨天
// console.log(getYestDayOrNextDay(false)); // 2023/8/7 明天
// 上周
export function getLastWeek() {
let thisWeek = getThisWeek(); // 上面获取本周的函数
let startDate = new Date(
new Date(thisWeek.startDate).getTime() - 7 * 86400000
);
let endDate = new Date(new Date(thisWeek.endDate).getTime() - 7 * 86400000);
return {
startDate: expirationDate(startDate), // 2023/7/31
endDate: expirationDate(endDate) // 2023/8/4
};
}
// 本周
export function getThisWeek() {
let today = new Date();
let dayOfWeek = today.getDay() - 1;
let startDate = new Date(today.getTime() - dayOfWeek * 86400000);
let endDate = new Date(startDate.getTime() + 6 * 86400000);
return {
startDate: expirationDate(startDate), // 2023/8/7
endDate: expirationDate(endDate) // 2023/8/11
};
}
// 下周
export function getNextWeek() {
let thisWeek = getThisWeek();
let startDate = new Date(
new Date(thisWeek.startDate).getTime() + 7 * 86400000
);
let endDate = new Date(new Date(thisWeek.endDate).getTime() + 7 * 86400000);
return {
startDate: expirationDate(startDate), // 2023/8/14
endDate: expirationDate(endDate) // 2023/8/18
};
}
// 本月
export function getThisMonth() {
let today = new Date();
let year = today.getFullYear();
let month = today.getMonth() + 1;
let daysInMonth = new Date(year, month, 0).getDate();
let startDate = new Date(year, month - 1, 1);
let endDate = new Date(year, month - 1, daysInMonth);
return {
startDate: expirationDate(startDate), // 2023/8/1
endDate: expirationDate(endDate) // 2023/8/31
};
}
// 上月
export function getLastMonth() {
let thisMonth = getThisMonth();
let startDate = new Date(
new Date(thisMonth.startDate).setMonth(
new Date(thisMonth.startDate).getMonth() - 1
)
);
let endDate = new Date(
new Date(thisMonth.endDate).setMonth(
new Date(thisMonth.endDate).getMonth(),
0
)
);
return {
startDate: expirationDate(startDate), // 2023/7/1
endDate: expirationDate(endDate) // 2023/7/31
};
}
// 本月为第几季度
export function getQuarter() {
let today = new Date();
let month = today.getMonth() + 1;
return Math.ceil(month / 3); // 当前8月份 值为:3
}
// 本季度
export function getThisQuarter() {
let today = new Date();
let year = today.getFullYear();
let quarter = getQuarter();
let startDate, endDate;
if (quarter == 1) {
startDate = new Date(year, 0, 1);
endDate = new Date(year, 2, 31);
} else if (quarter == 2) {
startDate = new Date(year, 3, 1);
endDate = new Date(year, 5, 30);
} else if (quarter == 3) {
startDate = new Date(year, 6, 1);
endDate = new Date(year, 8, 30);
} else if (quarter == 4) {
startDate = new Date(year, 9, 1);
endDate = new Date(year, 11, 31);
}
return {
startDate: startDate.toLocaleDateString(), // 2023/7/1
endDate: endDate.toLocaleDateString() // 2023/9/30
};
}
// 上季度
export function getLastQuarter() {
let thisQuarter = getThisQuarter();
let startDate = new Date(
new Date(thisQuarter.startDate).setMonth(
new Date(thisQuarter.startDate).getMonth() - 3
)
);
let endDate = new Date(
new Date(thisQuarter.endDate).setMonth(
new Date(thisQuarter.endDate).getMonth() - 3
)
);
return {
startDate: startDate.toLocaleDateString(), // 2023/4/1
endDate: endDate.toLocaleDateString() // 2023/6/30
};
}
// 本年
export function getThisYear() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() + "-01-01";
// 获取结束日期
const endDate = today.getFullYear() + "-12-31";
return {
startDate, // 2023/1/1
endDate // 2023/12/31
};
}
// 上一年
export function getLastYear() {
// 获取当前日期
const today = new Date();
// 获取起始日期
const startDate = today.getFullYear() - 1 + "-01-01";
// 获取结束日期
const endDate = today.getFullYear() - 1 + "-12-31";
return {
startDate, // 2023/1/1
endDate // 2023/12/31
};
}
// 获得某月的开始日期
export function getMonthStartDate(paraYear,paraMonth){
var monthStartDate = new Date(paraYear, paraMonth, 1);
return formatDate(monthStartDate);
}
// 获得某月的结束日期
export function getMonthEndDate(paraYear,paraMonth){
var monthEndDate = new Date(paraYear,paraMonth, getMonthDays(paraYear,paraMonth));
return formatDate(monthEndDate);
}
export function getMonthDays(paraYear,paraMonth){
var monthStartDate = new Date(paraYear, paraMonth, 1);
var monthEndDate = new Date(paraYear, paraMonth + 1, 1);
var days = (monthEndDate - monthStartDate)/(1000 * 60 * 60 * 24);
return days;
}
// 格式化日期:yyyy-MM-dd
export function formatDate(date) {
var myyear = date.getFullYear();
var mymonth = date.getMonth()+1;
var myweekday = date.getDate();
if(mymonth < 10){
mymonth = "0" + mymonth;
}
if(myweekday < 10){
myweekday = "0" + myweekday;
}
return (myyear+"-"+mymonth + "-" + myweekday);
}
// 中国标准时间转年月日
export function getDateString(dateValue) {
let date = new Date(dateValue);
let y = date.getFullYear();
let m = date.getMonth() + 1;
let d = date.getDate();
let day = y + '-' + (m < 10 ? ('0' + m) : m) + '-' + (d < 10 ? ('0' + d) : d);
return day;
}
vue js 日期获取
于 2024-04-23 19:22:51 首次发布
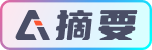