Single Linked List单链表
文章目录
文件:
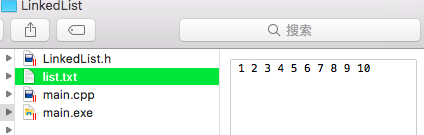
主函数:
#include <fstream> //调用文件输入输出流类
#include "LinkedList.h" //调用单链表头文件
using namespace std; //声明命名空间
int main(){
List<int> list; //实例化类List
ifstream fin("list.txt"); //这里将输入文件设为ifstream类型
assert(fin); //判断文件读取过程是否成功编译
fin >> list; //调用输入流operator>>,将文件中的数据一一输入链表list
//这句话的效果相当于operator>>(fin,list);
//因为ifstream公有继承了iostream
cout << "The initial list in the file is:\n" << list << endl;
list.Inverse(); //逆转这个单链表
cout << "Inverse the list, then the list is:\n" << list << endl;
cout << "========================================\n";
int i, elem; //定义两个整数变量
cout << "Test the Insert, Remove and Search function:\n";
cout << "Each test will terminate by an invaid input.";
cout << "\n----------------------------------------\n";
cout << "1. Test the Insert(int i, T &elem):\n";
while (1){
cout << "Input the index i and data elem to insert: ";
cin >> i >> elem;
if (!cin){
//结束条件1:输入异常。
//这是异常处理的一种,当碰到cin了错误的类型时
cin.clear(); //clear将cin的数据元素failbit修改到原来的状态
cin.ignore(100,'\n'); //清空缓存区
break;
}
if (i < 0) break; //结束条件2:输入负数
if (list.Insert(i, elem)) cout << "Insert successful!\n";
else cout << "Insert failed!\n";
}
cout << "\nAfter inserted\n" << list << endl;
cout << "----------------------------------------\n";
cout << "2. Test the Remove(int i, T &elem):\n";
while (1){
cout << "Input the index i in which you want to remove: ";
cin >> i;
if (!cin){
cin.clear();
cin.ignore(100,'\n');
break;
}
if (i < 0) break;
if (list.Remove(i, elem)) cout << "The element " << elem << " has been removed!\n";
else cout << "Remove failed!\n";
}
cout << "\nAfter removed\n" << list << endl;
cout << "----------------------------------------\n";
cout << "3. Test the Search(T &elem):\n";
while (1){
cout << "Input the element you want to search: ";
cin >> elem;
if (!cin){
cin.clear();
cin.ignore(100,'\n');
break;
}
if (elem < 0) break;
LinkNode<int> *p = list.Search(elem);
if (p) cout << "The element " << elem << " is in the list.\n";
else cout << "The element is not exist!\n";
}
cout << "\n----------------------------------------\n";
cout << "End test!" << endl;
return 0;
}
单链表结点类定义
template <typename T>struct LinkNode{
T data; //数据域
LinkNode<T> *link; //指针域
LinkNode(LinkNode<T> *ptr = NULL){
//构造函数,默认指针域为空
link = ptr;
}
LinkNode(const T &item, LinkNode<T> *ptr = NULL){
data = item; //传入一个类型为T的数据,构造函数中定义为数据元素
link = ptr; //指针域,若未赋值初始化为NULL
}
};
单链表类定义
template <typename T>class List{
public:
List(){
//无参构造函数,其中也调用了LinkNode的无参构造函数
first = new LinkNode<T>; //first指向新的内存空间LinkNode<T>
}
List(const T &x){
//有参构造函数,其中也调用了LinkNode的有参构造函数
first = new LinkNode<T>(x);
//first指向新的内存空间LinkNode<T>,数据成员为x
}
List(List<T> &L); //拷贝构造函数
~List(){
//析构函数
makeEmpty(); //释放首节点之后的所有结点
delete first; //释放首节点
}
void makeEmpty(); //声明清空函数
int Length()const;//声明计算长度函数
LinkNode<T> *getHead()const{
//返回指向结点指针的函数(访问私有成员first)
return first;
}
LinkNode<T> *Search(const T &x);//声明查找函数,返回指向结点的指针
LinkNode<T> *Locate(int i); //声明定位函数,返回指向结点的指针
bool getData(int i,T&x)const;//获取数据函数,传入结点号i,T类型数据x
void setData(int i, T &x); //设置数据函数,传入i和x
bool Insert(int i, T &x); //插入函数,传入i和x
bool Remove(int i, T &x); //删除函数,传入i和x
bool IsEmpty()const{
//判断单链表是否为空
return (first->link == NULL)?true: