在开始java的旅途时,别人提议做一个简单的计算器。经过一段时间的积累,我终于在全方位的资料中完成了。当时感觉是很兴奋。能做点东西了!~ 呵呵 以下是部分截图以及代码。
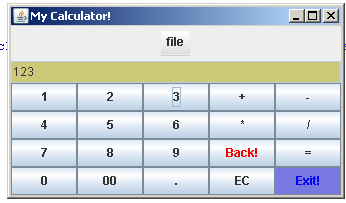
import
java.awt.BorderLayout;
import
java.awt.Color;
import
java.awt.FlowLayout;
import
java.awt.GridLayout;
import
java.awt.event.ActionEvent;
import
java.awt.event.ActionListener;

import
javax.swing.JButton;
import
javax.swing.JFrame;
import
javax.swing.JMenu;
import
javax.swing.JMenuBar;
import
javax.swing.JMenuItem;
import
javax.swing.JOptionPane;
import
javax.swing.JPanel;
import
javax.swing.JTextField;


public
class
JSanQi
implements
ActionListener
...
{
JFrame f1;

JPanel p1, p2, p3;

JTextField t1;

JButton bt[];

JButton bdoc, bexit, back, bzero, badd, bsub, bmul, bdiv, bcanl, bequl;

boolean Key;

JMenuBar menu;

JMenu m1;

JMenuItem mt1, mt2;

Double fnum;

int key, doc;

@SuppressWarnings("deprecation")

public JSanQi() ...{
fnum = 0.0;
Key = true;
doc = 0;
f1 = new JFrame("My Calculator!");
f1.setLayout(new BorderLayout());
p1 = new JPanel();
p2 = new JPanel();
p3 = new JPanel();
t1 = new JTextField();
menu = new JMenuBar();
m1 = new JMenu("file");
mt1 = new JMenuItem("nihao!");
mt2 = new JMenuItem("exit!");
t1.setEditable(false);
t1.setBackground(new Color(205,201,120));
f1.add(p2, BorderLayout.NORTH);
f1.add(p1);
p2.setLayout(new FlowLayout());
p2.add(menu, FlowLayout.LEFT);
menu.add(m1);
m1.add(mt1);
m1.add(mt2);
mt1.addActionListener(this);
mt2.addActionListener(this);
p1.setLayout(new BorderLayout());
p1.add(t1, BorderLayout.NORTH);
p1.add(p3);
bt = new JButton[10];

for (int i = 0; i < 10; i++) ...{
bt[i] = new JButton(Integer.toString(i));
}
bdoc = new JButton(".");
bexit = new JButton("Exit!");
back = new JButton("Back!");
back.setForeground(new Color(255, 0, 0));
bexit.setForeground(new Color(0, 0, 255));
bexit.setBackground(new Color(120,120,225));
bzero = new JButton("00");
badd = new JButton("+");
bsub = new JButton("-");
bmul = new JButton("*");
bdiv = new JButton("/");
bcanl = new JButton("EC");
bequl = new JButton("=");
//bequl.setLabel("123");
//bequl.addNotify();
p3.setLayout(new GridLayout(4, 5));

p3.add(bt[1]);
p3.add(bt[2]);
p3.add(bt[3]);
p3.add(badd);
p3.add(bsub);
p3.add(bt[4]);

p3.add(bt[5]);
p3.add(bt[6]);
p3.add(bmul);
p3.add(bdiv);
p3.add(bt[7]);
p3.add(bt[8]);
p3.add(bt[9]);
p3.add(back);
p3.add(bequl);
p3.add(bt[0]);
p3.add(bzero);
p3.add(bdoc);
p3.add(bcanl);
p3.add(bexit);

bt[0].addActionListener(this);
bt[1].addActionListener(this);
bt[2].addActionListener(this);
bt[3].addActionListener(this);
bt[4].addActionListener(this);
bt[5].addActionListener(this);
bt[6].addActionListener(this);
bt[7].addActionListener(this);
bt[8].addActionListener(this);
bt[9].addActionListener(this);

bdoc.addActionListener(this);
bexit.addActionListener(this);
back.addActionListener(this);
bzero.addActionListener(this);
badd.addActionListener(this);
bsub.addActionListener(this);
bmul.addActionListener(this);
bdiv.addActionListener(this);
bcanl.addActionListener(this);
bequl.addActionListener(this);

f1.setSize(290, 220);
f1.pack();
f1.setVisible(true);
}


public static void main(String[] args) ...{
new JSanQi();

}


public void actionPerformed(ActionEvent event) ...{
Object temp = event.getSource();


try ...{

for (int i = 0; i < 10; i++) ...{

if (temp == bt[i] && Key == true) ...{
t1.setText(t1.getText() + Integer.toString(i));
}
}

if (temp == badd || temp == bsub || temp == bmul || temp == bdiv) ...{

if (temp == badd && Key == true) ...{
key = 1;
fnum = Double.parseDouble(t1.getText());
// t1.setText(t1.getText()+fnum);
}

if (temp == bsub && Key == true) ...{
key = 2;
fnum = Double.parseDouble(t1.getText());
}

if (temp == bmul && Key == true) ...{
key = 3;
fnum = Double.parseDouble(t1.getText());
}

if (temp == bdiv && Key == true) ...{

if (Double.parseDouble(t1.getText()) == 0) ...{
t1.setText("Wrong action!");

} else ...{
key = 4;
fnum = Double.parseDouble(t1.getText());
}
}
t1.setText("");
doc=0;
}

if (temp == bequl && Key == true) ...{

switch (key) ...{
case 1:
fnum += Double.parseDouble(t1.getText());
t1.setText(Double.toString(fnum));
break;
case 2:
fnum -= Double.parseDouble(t1.getText());
t1.setText(Double.toString(fnum));
break;
case 3:
fnum *= Double.parseDouble(t1.getText());
t1.setText(Double.toString(fnum));
break;
case 4:
fnum /= Double.parseDouble(t1.getText());
t1.setText(Double.toString(fnum));
break;
default:
break;
}
doc=0;
}

if (temp == bcanl) ...{
t1.setText("");
Key = true;
doc=0;

}

if (temp == bexit || temp == mt2) ...{
System.exit(0);
}

if (temp == bzero && Key==true) ...{
t1.setText(t1.getText() + "00");
//doc=0;
}

if (temp == mt1) ...{
JOptionPane.showMessageDialog(p1, "How Are You! "
+ "Architect Is Topwise ^_^");

}

if (temp == bdoc && Key==true) ...{

if (doc == 0) ...{
t1.setText(t1.getText() + ".");
}

if(doc==1) ...{
t1.setText("Wrong Action");
Key = false;
doc = 0;
}
doc = 1;
}

if (temp==back && Key==true)...{
}


} catch (Exception e) ...{
t1.setText("Wrong Action!");
Key = false;
}
}

}
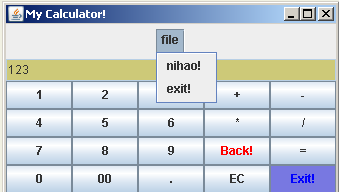
也许能帮上部分人的忙。有什么问题请赐教!! 有点遗憾的是BACK按钮没有写功能,
有什么问题也可以找我交流。