用jsonp,解决跨域,请求url后面要加上 output=jsonp,直接复制代码,换上自己的key可用,先上效果图
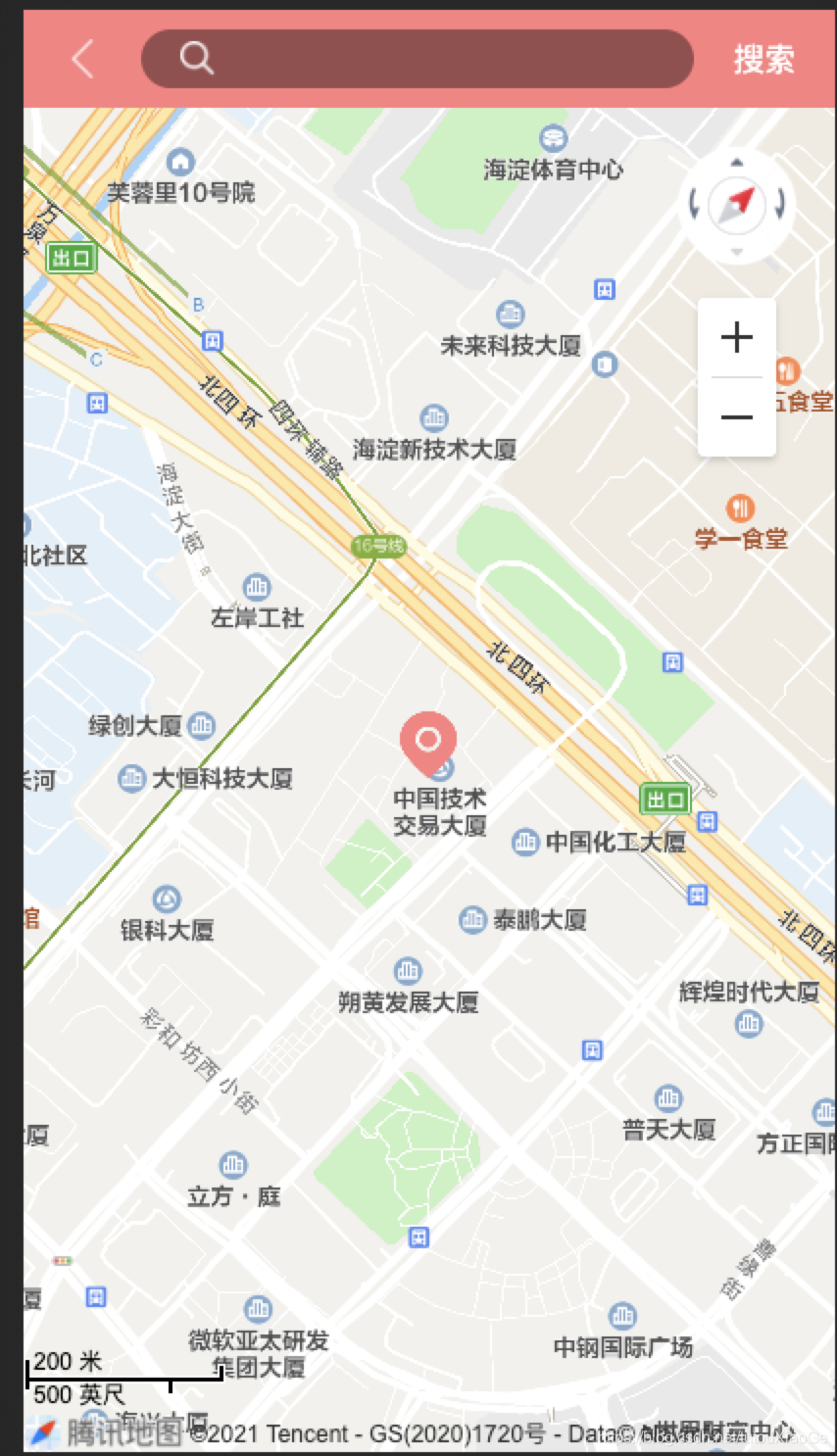
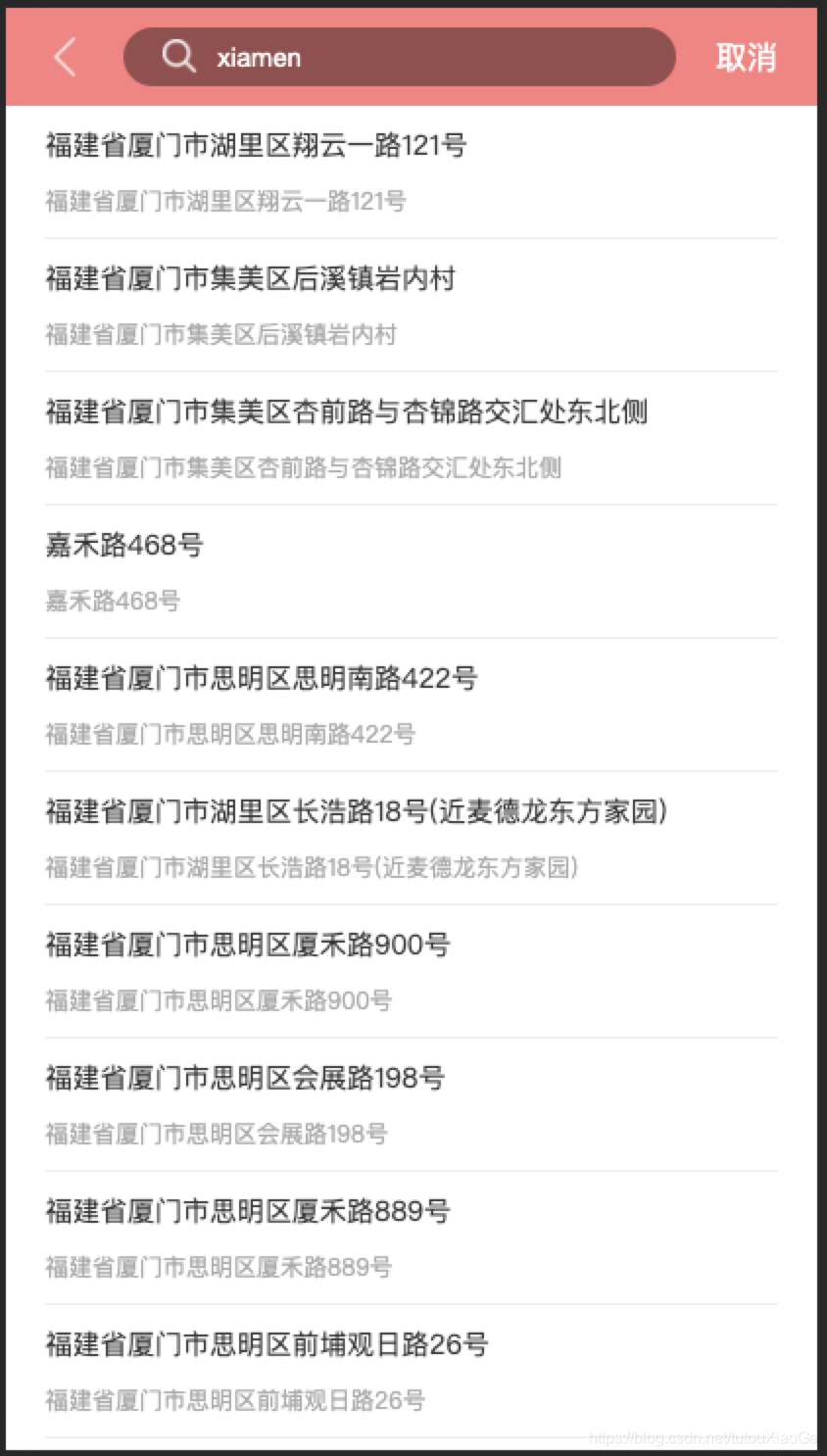
$.ajax({
type: 'get',
url: 'https://apis.map.qq.com/ws/place/v1/suggestion?output=jsonp',
async: false,
data: params,
dataType: 'jsonp',
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'GET,POST',
},
success: function (result) {
reslove(result)
},
error: function (xhr, errorType, error) {
console.log('xhr', xhr)
console.log('errorType', errorType)
console.log('error', error)
reject(xhr)
},
complete: function () { },
})
<style>
* {
margin: 0;
padding: 0;
}
#container {
position: fixed;
top: 50px;
width: 100vw;
bottom: 0;
}
.search_part {
position: fixed;
top: 0;
width: 100%;
box-sizing: border-box;
color: #fff;
height: 50px;
display: flex;
align-items: center;
padding: 0 20px;
line-height: 50px;
background: #ff8080;
}
#place_list {
position: fixed;
top: 50px;
width: 100vw;
bottom: 0;
z-index: 2;
overflow-y: scroll;
padding: 0 20px;
box-sizing: border-box;
background: #fff;
display: none;
}
#cancel_btn{
display: none;
}
.back {
width: 20px;
}
.search_wrap {
flex: 1;
position: relative;
height: 30px;
display: flex;
align-items: center;
line-height: 30px;
margin: 0 20px;
overflow: hidden;
border-radius: 15px;
background: rgba(0, 0, 0, 0.4);
}
.search_wrap input {
border: none;
height: 100%;
outline: none;
flex: 1;
color: #fff;
margin-left: 10px;
background: transparent;
}
.icon_search {
width: 18px;
height: 18px;
margin-left: 20px;
}
.no_place {
text-align: center;
margin-top: 100px;
color: #aaa;
}
.placeItem{
padding: 10px 0;
border-bottom: 1px solid #eee;
}
.place_title{
color: #333;
font-size: 14px;
}
.sub_place{
color: #aaa;
margin-top: 10px;
font-size: 12px;
}
.no_more{
font-size: 14px;
padding: 20px 0;
text-align: center;
color: #aaa;
}
.loader {
position: fixed;
top: 0;
bottom: 0;
left: 0;
right: 0;
display: flex;
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
margin: 0 auto;
z-index: 9;
background: rgba(255, 255, 255, 0.6);
/* Delay both the second and third circle at the start */
}
.loader .circle {
background-color: #ff8080;
margin-right: 4px;
width: 10px;
height: 10px;
border-radius: 50%;
animation: loadingcircle 1s infinite;
animation-iteration-count: infinite;
}
.loader .circle:last-child {
margin-right: 0px;
}
.loader .circle:nth-child(2) {
animation-delay: 0.1s;
}
.loader .circle:nth-child(3) {
animation-delay: 0.2s;
}
@keyframes loadingcircle {
0% {
transform: scale(1);
}
50% {
transform: scale(0);
}
100% {
transform: scale(1);
}
}
</style>
<body>
<div class="search_part">
<div class="search_wrap">
<img class="icon_search" src="./static/search.png" alt="" />
<input id="keyword" type="text" />
</div>
<div id="search_btn">搜索</div>
<div id="cancel_btn">取消</div>
</div>
<div id="place_list">
<div class="no_place">
<img src="./static/no_address.png" alt="" />
</div>
</div>
<!-- 定义地图显示容器 -->
<div id="container"></div>
</body>
<script src="https://map.qq.com/api/gljs?v=1.exp&key=腾讯地图key"></script>
<script src="./js/jquery-3.5.1.js"></script>
<script src="./js/lodash.js"></script>
<script>
$(function () {
let placeList = []
let map = null
let pager = {
page_index: 1,
page_size: 20
}
let keyword = ''//关键词
let more = true
// 地图初始化
initMap()
$('#keyword').focus(function () {
$('#place_list').show()
})
$('#cancel_btn').click(function () {
$('#place_list').hide()
$(this).hide()
$('#search_btn').show()
})
$('#search_btn').click(function () {
$(this).hide()
$('#cancel_btn').show()
$('#keyword').focus()
})
$('#keyword').focus(function () {
$('#cancel_btn').show()
$('#search_btn').hide()
})
// 地址搜索
$('#keyword').on(
'input propertychange',
_.debounce(function () {
keyword = $(this).val()
if (keyword != '') {
getSearchResult()
}
}, 500)
)
// 触底加载
$('#place_list').on('scroll', function (res) {
let scrollHeight = $(this)[0].scrollHeight
let scrollTop = $(this).scrollTop()
let height = $(this).height()
if ((scrollTop + height >= scrollHeight) && more) {
console.log('触底了')
pager.page_index++
getSearchResult()
}
})
// 地点选择
$(document).delegate('.placeItem', 'click', function () {
let lat = $(this).attr('lat')
let lng = $(this).attr('lng')
console.log()
$('#place_list').hide()
map.panTo([Number(lat), Number(lng)])
// map.setCenter(new TMap.LatLng(lat, lng))
})
function getSearchResult() {
let data = {
...pager, keyword
}
showLoading()
placeSearch(data).then(res => {
let html = ''
let result = res.data
console.log(res.data)
for (let index in result) {
let item = result[index]
html += `<div class="placeItem" lat="${item.location.lat}" lng="${item.location.lng}">
<div class="place_title">${item.address}</div>
<div class="sub_place">${item.address}</div>
</div>`
}
if (result.length == 0 && data.page_index == 1) {
more = false
html = `<div class="no_place">
<img src="./static/no_address.png" alt="" />
</div>`
} else if ((data.page_index > 1 && data.page_size > result.length) || (data.page_index == 1 && data.page_size > result.length)) {
// 加载完全部
more = false
html += '<div class="no_more">没有更多啦~</div>'
}
if (data.page_index == 1) {
placeList = result
$('#place_list').html(html)
} else {
placeList.concat(result)
$('#place_list').append(html)
}
$('.loader').remove()
})
}
function placeSearch(data = {}) {
return new Promise((reslove, reject) => {
let params = {
key: 'OLBBZ-APHKX-AQD4Y-TX3NS-SU6Q5-BHBTH',
...data
}
$.ajax({
type: 'get',
url: 'https://apis.map.qq.com/ws/place/v1/suggestion?output=jsonp',
async: false,
data: params,
dataType: 'jsonp',
headers: {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Methods': 'GET,POST',
},
success: function (result) {
reslove(result)
},
error: function (xhr, errorType, error) {
console.log('xhr', xhr)
console.log('errorType', errorType)
console.log('error', error)
reject(xhr)
},
complete: function () { },
})
})
}
//地图初始化函数,本例取名为init,开发者可根据实际情况定义
function initMap() {
//定义地图中心点坐标
var center = new TMap.LatLng(39.98412, 116.307484)
//定义map变量,调用 TMap.Map() 构造函数创建地图
map = new TMap.Map(document.getElementById('container'), {
center: center, //设置地图中心点坐标
zoom: 16, //设置地图缩放级别
pitch: 0, //设置俯仰角
rotation: 45, //设置地图旋转角度
})
var marker = marker = new TMap.MultiMarker({
id: 'marker-layer',
map: map,
styles: {
"marker": new TMap.MarkerStyle({
"width": 35,
"height": 35,
"src": './static/location.png'
})
},
geometries: [{
"styleId": 'marker',
"position": new TMap.LatLng(39.98412, 116.307484),
"properties": {
"title": "marker"
}
}]
});
map.on("click", function (evt) {
let lat = evt.latLng.getLat().toFixed(6);
let lng = evt.latLng.getLng().toFixed(6);
if (marker) {
marker.setMap(null);
marker = null;
}
marker = new TMap.MultiMarker({
id: 'marker-layer',
map: map,
styles: {
"marker": new TMap.MarkerStyle({
"width": 35,
"height": 35,
"src": './static/location.png'
})
},
geometries: [{
"styleId": 'marker',
"position": new TMap.LatLng(lat, lng),
"properties": {
"title": "marker"
}
}]
});
map.panTo([lat, lng])
})
}
})
</script>```