示例
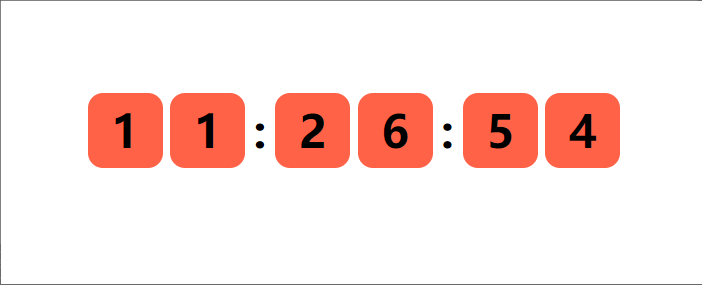
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
div {
width: 500px;
height: 50px;
margin: 200px auto;
}
span {
width: 50px;
height: 50px;
float: left;
margin-left: 5px;
background-color: tomato;
color: black;
line-height: 50px;
text-align: center;
font-size: 30px;
font-weight: bold;
border-radius: 10px;
}
.colon {
width: 10px;
background-color: white;
}
</style>
</head>
<body>
<div>
<span class="num">1</span>
<span class="num">2</span>
<span class="colon">:</span>
<span class="num">3</span>
<span class="num">4</span>
<span class="colon">:</span>
<span class="num">6</span>
<span class="num">7</span>
</div>
</body>
</html>
<script src="./js/jquery-3.1.1.min.js"></script>
<script>
$(function () {
//1. 指定倒计时的到期时间(2018-5-30 14:00:)
//可以直接使用年月日时分秒来创建一个date对象,但是月是从0开始的
var to = new Date(2018, 4, 30, 12, 34, 10);
function antitime() {
var now = new Date();
//2. 拿到当前时间和过期时间之间的时间差(毫秒)
var deltaTime = to - now; //到期时间和当前时间相差的毫秒数
//如果超时了,就停止倒计时
if (deltaTime <= 0) {
//停止计时器
window.clearInterval(timer);
//停止执行下面的代码
return;
}
//已知毫秒数,算出几分几秒几秒
var m = Math.floor(deltaTime / (60 * 1000));
//算出有多少秒
var s = Math.floor(deltaTime / 1000 % 60);
//算出多少毫秒, 毫秒数只显示10位和百位
var ms = Math.floor(deltaTime % 1000 / 10);
//把时间的数字转成字符串, 如果分秒毫秒不足10, 则前面补0
var timeStr = "" + (m < 10 ? "0" + m : m) + (s < 10 ? "0" + s : s) + (ms < 10 ? "0" + ms : ms);
// console.log(timeStr);
// each是用来遍历.num元素
$(".num").each(function (index, span) {
//console.log(span);
// substring 是用来提取字符串中介于两个指定下标之间的字符
$(span).html(timeStr.substring(index, index + 1));
});
}
//每十毫秒执行一次
var timer = setInterval(antitime, 10);
})
</script>