前言
至简微博客户端APP根据微博开放品台API获取微博内容,UI展示
主要功能点:
- 好友微博列表
- 公共微博列表
- 微博详情
- 用户个人信息
- 微博个人信息
- 微博评论列表
- 每日一图
- 粉丝列表
- 关注列表
- 关注数、粉丝数、微博数、收藏数等
效果图
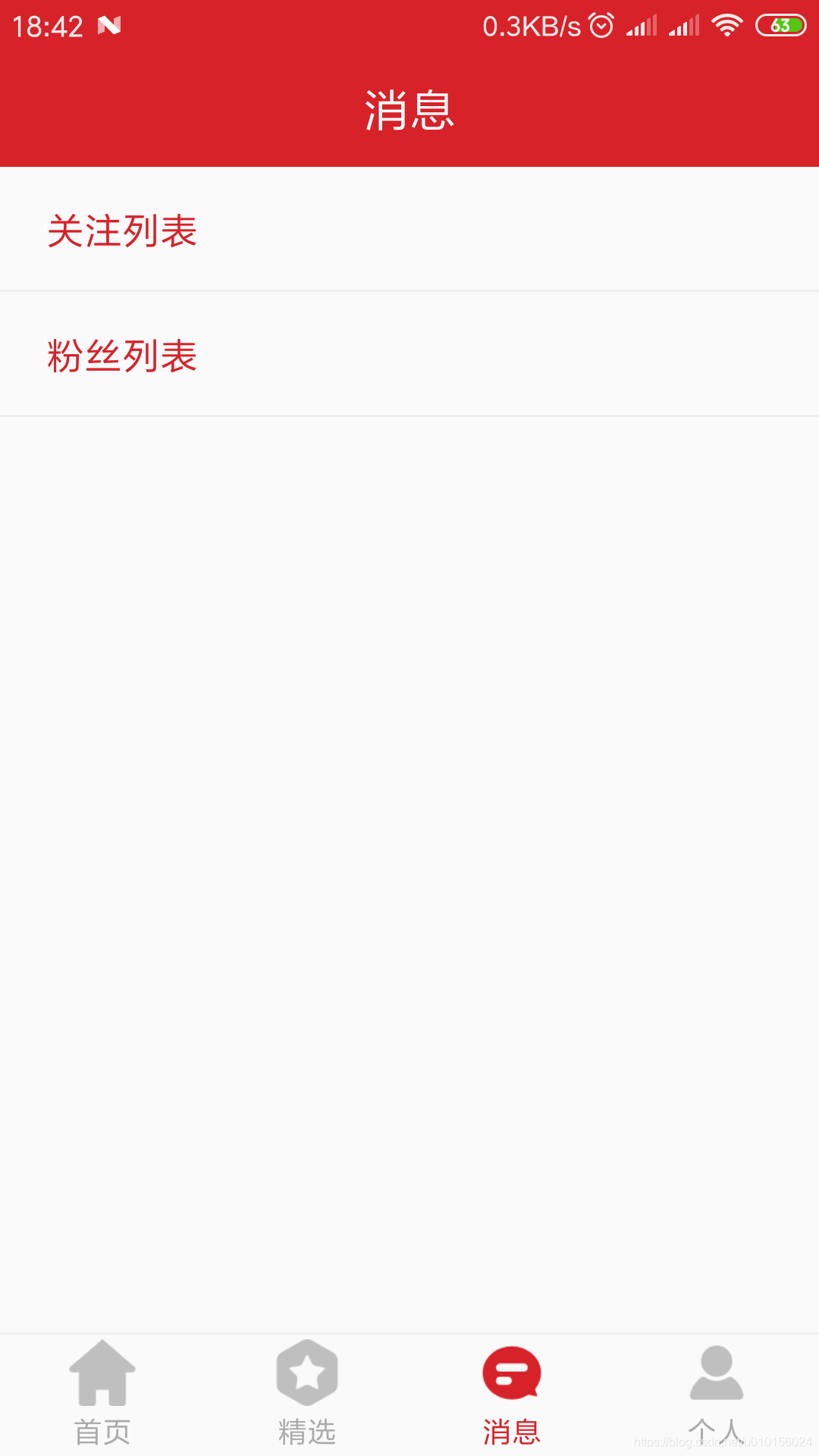
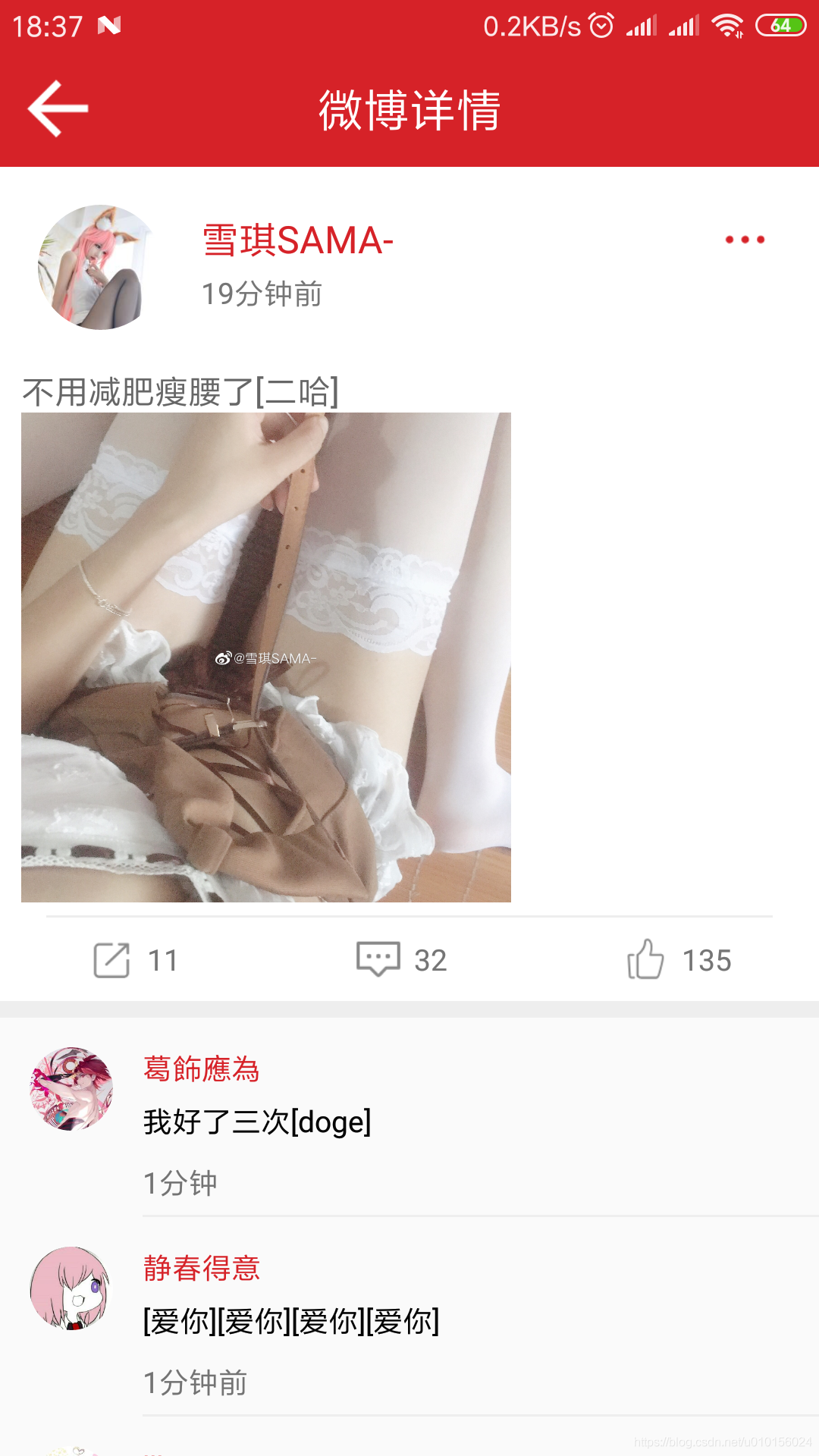
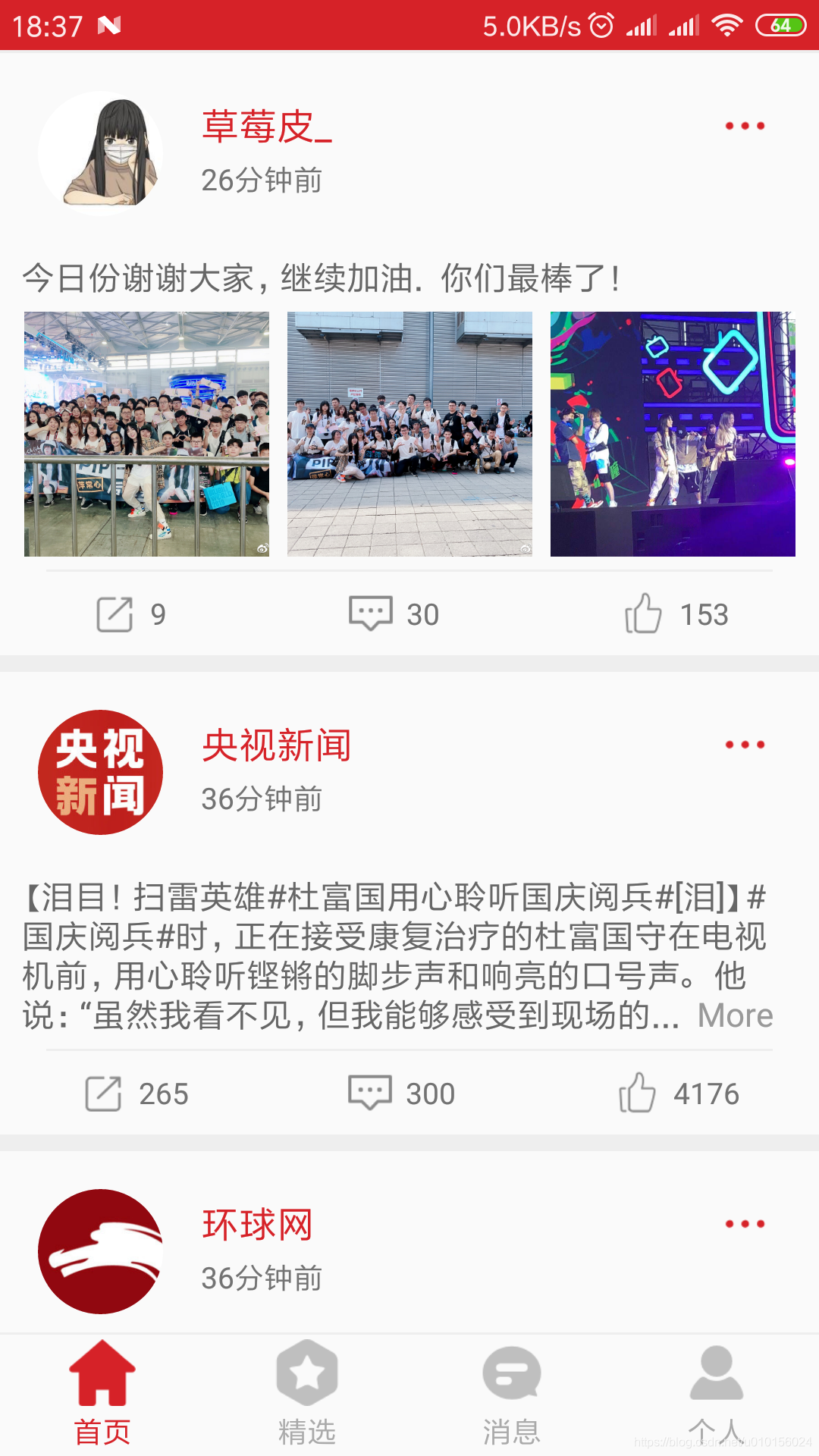
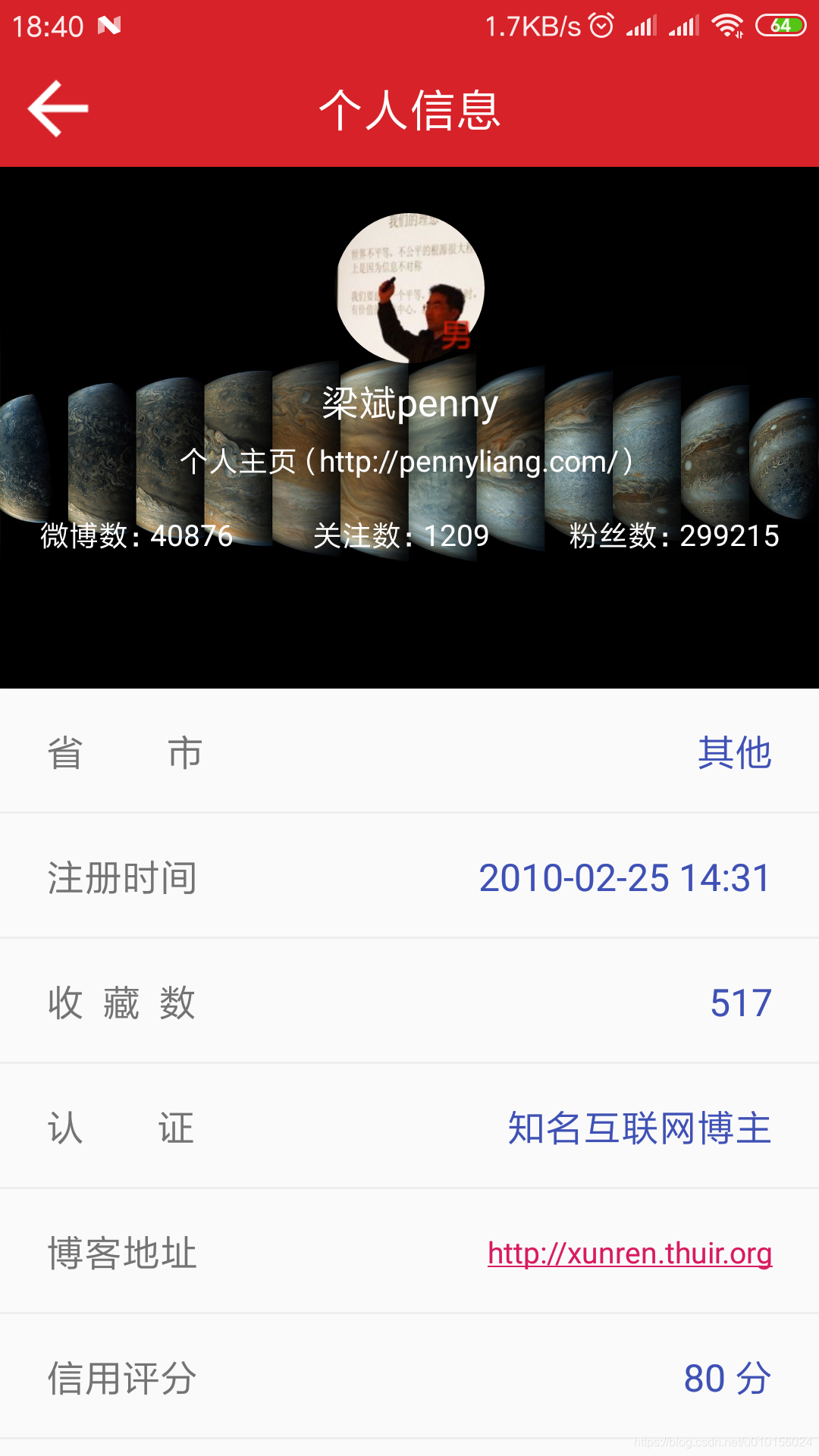
源码下载
如果需要源码,请联系我邮箱 nyyin@vip.qq.com
开发环境
- kotlin
- Retrofit
- Rxjava
- MVP
- AndroidX
- Glide
具体实现
一 微博平台
官网: https://open.weibo.com/wiki/%E9%A6%96%E9%A1%B5
打开微博平台官网注册,因为是开发APP,所以选择移动应用
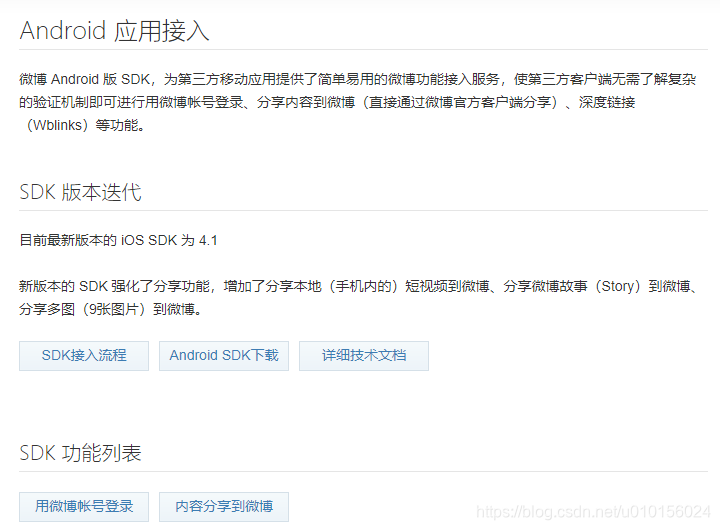
这里开发AndroidAPP,选择Android SDK下载
这个SDK主要是微博分享、微博授权等功能的具体实现的工具,微博提供了这一个方便的SDK方便开发者开发。
而对于微博API的具体调用,SDK中并没有什么帮助,需自己调用,文中开发的APP使用Retrofit + Rxjava来实现。
创建应用完成后如图:
应用注册成功后,会生成对应的appkey和appsecret值。另外需要设置的值有授权回调页。
授权回调页默认设置为:https://api.weibo.com/oauth2/default.html
二 集成SDK
AS中构建Android APP工程,集成微博SDK
api 'com.sina.weibo.sdk:core:2.0.3:openDefaultRelease@aar'
三 授权
private fun launchScope() {
if (this.isFinishing) {
return
}
val mAccessToken = AccessTokenKeeper.readAccessToken(this);
if (mAccessToken.isSessionValid) {
BlogApplication.mAccessToken = mAccessToken
launchMain()
return
}
getToken()
}
private fun getToken() {
mSsoHandler = SsoHandler(this)
mSsoHandler.authorize(object : WbAuthListener {
override fun onSuccess(token: Oauth2AccessToken?) {
LogUtil.i(token?.toString())
val isValid = token?.isSessionValid
if (isValid == true) {
BlogApplication.mAccessToken = token
AccessTokenKeeper.writeAccessToken(this@LoginActivity, token)
launchMain()
}
}
override fun onFailure(p0: WbConnectErrorMessage?) {
LogUtil.i("err code:" + p0?.errorCode + ", err msg:" + p0?.errorMessage)
UiUtil.toast(this@LoginActivity, "授权失败,请重试")
}
override fun cancel() {
LogUtil.i("on cancel.")
UiUtil.toast(this@LoginActivity, "授权已取消")
}
})
}
private fun launchMain() {
val intent = Intent(this, MainActivity::class.java)
startActivity(intent)
finish()
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
mSsoHandler.authorizeCallBack(requestCode, resultCode, data)
}
微博授权过程,以上代码即可实现。
以上授权成功后,AccessTokenKeeper类 会获取到四个值:
private static final String KEY_UID = "uid";
private static final String KEY_ACCESS_TOKEN = "access_token";
private static final String KEY_EXPIRES_IN = "expires_in";
private static final String KEY_REFRESH_TOKEN = "refresh_token";
uid、token、expires_in、refresh_token
这四个值其中token和uid尤为重要,其他不重要。授权过程中需要用户在登录页输入用户名和密码,但是APP是获取不到这些信息的,这些信息的页面不受APP控制,由微博登录页显示获取,然后,微博根据用户名和密码返回给APP以上四个值,这样一来APP与用户密码信息隔离,这就是auth2.0认证的大致过程了
四 调用微博API
interface WeiboRequest {
@GET("https://api.weibo.com/2/statuses/home_timeline.json")
fun getStatusesHomeTimeLineMore(@Query("since_id") since_id: String, @Query("count") count: Int): Observable<StatusesResponse>
@GET("https://api.weibo.com/2/statuses/home_timeline.json")
fun getStatusesHomeTimeLineRefresh(@Query("max_id") max_id: String, @Query("count") count: Int): Observable<StatusesResponse>
@GET("https://api.weibo.com/2/short_url/share/counts.json")
fun getShortUrlExpand(@Query("url_short") url_short: String): Observable<ShortUrlResponse>
// 必须是自己发出的微博
@GET("https://api.weibo.com/2/statuses/show.json")
fun getDetailInfo(@Query("id") id: String): Observable<StatusesDetailResponse>
@GET("https://api.weibo.com/2/comments/show.json")
fun getComments(@Query("id") id: String, @Query("since_id") since_id: String, @Query("max_id") max_id: String): Observable<CommentsResponse>
@GET("https://api.weibo.com/2/users/show.json")
fun getUserInfo(@Query("uid") uid: String, @Query("screen_name") screenName: String): Observable<UserInfoResponse>
@GET("https://api.weibo.com/2/friendships/friends.json")
fun getFollowList(@Query("uid") uid: String, @Query("cursor") cursor: Long): Observable<FollowListResponse>
@GET("https://api.weibo.com/2/friendships/followers.json")
fun getFollowerList(@Query("uid") uid: String, @Query("cursor") cursor: Long): Observable<FollowListResponse>
@GET("https://api.weibo.com/2/statuses/public_timeline.json")
fun getPublicTimeLine(@Query("page") page: Long): Observable<StatusesResponse>
}
以上是APP中用到的微博的一部分API,网络请求使用Retrofit+Rxjava来实现的
请求关注好友的微博列表,代码如下:
override fun getStatusesHomeLineLoad() {
RetrofitClient.instance.create(WeiboRequest::class.java)
.getStatusesHomeTimeLineMore(sinceId, COUNT)
.subscribeOn(Schedulers.newThread())
.observeOn(AndroidSchedulers.mainThread())
.subscribe(object : AbstractObserver<StatusesResponse>() {
override fun onSuc(t: StatusesResponse) {
LogUtil.i("statuses response :${t}")
iView?.setStatuses(t.statuses)
}
override fun onFail(e: Throwable) {
}
})
}
以上代码获取微博列表,返回json信息,微博对象如下:
class Statuses: Serializable {
var visible: Visible? = null
var created_at: String? = null
var id: Long = 0
var idstr: String? = null
var mid: String? = null
var can_edit: Boolean = false
var version: Int = 0
var show_additional_indication: Int = 0
var text: String? = null
var textLength: Int = 0
var source_allowclick: Int = 0
var source_type: Int = 0
var source: String? = null
var favorited: Boolean = false
var truncated: Boolean = false
var in_reply_to_status_id: String? = null
var in_reply_to_user_id: String? = null
var in_reply_to_screen_name: String? = null
var pic_urls: List<PicUrls>? = null
var thumbnail_pic: String? = null
var bmiddle_pic: String? = null
var original_pic: String? = null
var geo: Geo? = null
var is_paid: Boolean = false
var mblog_vip_type: Int = 0
var user: User? = null
var retweeted_status: Statuses? = null
var annotations: List<Annotations>? = null
var reposts_count: Int = 0
var comments_count: Int = 0
var attitudes_count: Int = 0
var pending_approval_count: Int = 0
var isLongText: Boolean = false
var reward_exhibition_type: Int = 0
var hide_flag: Int = 0
var mlevel: Int = 0
var biz_feature: Long = 0
var expire_time: Long = 0
var page_type: Int = 0
var hasActionTypeCard: Int = 0
var darwin_tags: List<String>? = null
var hot_weibo_tags: List<String>? = null
var text_tag_tips: List<String>? = null
var mblogtype: Int = 0
var userType: Int = 0
var extend_info: ExtendInfo? = null
var more_info_type: Int = 0
var cardid: String? = null
var number_display_strategy: NumberDisplayStrategy? = null
var positive_recom_flag: Int = 0
var content_auth: Int = 0
var gif_ids: String? = null
var is_show_bulletin: Int = 0
var comment_manage_info: CommentManageInfo? = null
var pic_num: Int = 0
}
五 UI展示
获取微博列表后,UI展示:
具体UI描述:
- 头像
- 名字
- 微博发布时间
- 微博内容
- 微博图片
- 转发数
- 评论数
- 点赞数
- 转发微博内容等
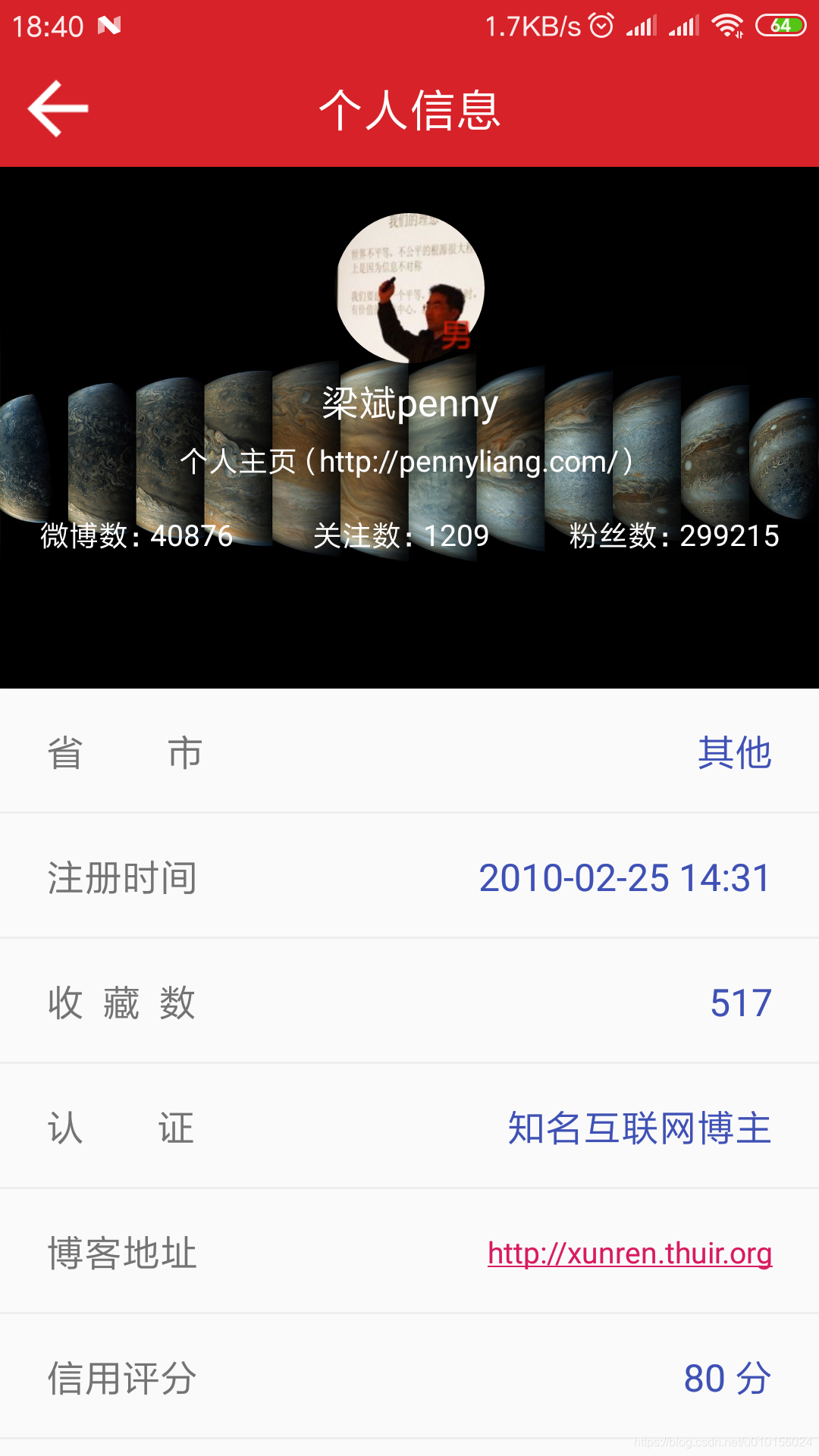
个人信息展示:
- 头像
- 名字
- 用户描述
- 省市
- 注册时间
- 收藏数、微博数、关注数、粉丝数
- 认证原因
- 博客地址
- 信用分数
声明
代码仅作为学习之用,严禁商业之用。
如有任何问题,不负责任!!
欢迎关注公众号:技术印象