An interesting mobile game
Time Limit: 3000/1000 MS (Java/Others) Memory Limit: 65535/32768 K (Java/Others)
Total Submission(s): 222 Accepted Submission(s): 104
Problem Description
XQ,one of the three Sailormoon girls,is usually playing mobile games on the class.Her favorite mobile game is called “The Princess In The Wall”.Now she give you a problem about this game.
Can you solve it?The following picture show this problem better.
This game is played on a rectangular area.This area is divided into some equal square grid..There are N rows and M columns.For each grid,there may be a colored square block or nothing.
Each grid has a number.
“0” represents this grid have nothing.
“1” represents this grid have a red square block.
“2” represents this grid have a blue square block.
“3” represents this grid have a green square block.
“4” represents this grid have a yellow square block.
1. Each step,when you choose a grid have a colored square block, A group of this block and some connected blocks that are the same color would be removed from the board. no matter how many square blocks are in this group.
2. When a group of blocks is removed, the blocks above those removed ones fall down into the empty space. When an entire column of blocks is removed, all the columns to the right of that column shift to the left to fill the empty columns.
Now give you the number of the row and column and the data of each grid.You should calculate how many steps can make the entire rectangular area have no colored square blocks at least.
Can you solve it?The following picture show this problem better.
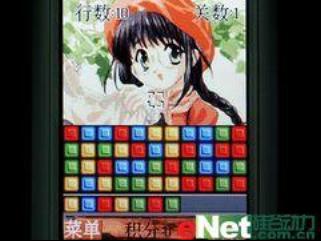
This game is played on a rectangular area.This area is divided into some equal square grid..There are N rows and M columns.For each grid,there may be a colored square block or nothing.
Each grid has a number.
“0” represents this grid have nothing.
“1” represents this grid have a red square block.
“2” represents this grid have a blue square block.
“3” represents this grid have a green square block.
“4” represents this grid have a yellow square block.
1. Each step,when you choose a grid have a colored square block, A group of this block and some connected blocks that are the same color would be removed from the board. no matter how many square blocks are in this group.
2. When a group of blocks is removed, the blocks above those removed ones fall down into the empty space. When an entire column of blocks is removed, all the columns to the right of that column shift to the left to fill the empty columns.
Now give you the number of the row and column and the data of each grid.You should calculate how many steps can make the entire rectangular area have no colored square blocks at least.
Input
There are multiple test cases. Each case starts with two positive integer N, M,(N, M <= 6)the size of rectangular area. Then n lines follow, each contains m positive integers X.(0<= X <= 4)It means this grid have a colored square block or nothing.
Output
Please output the minimum steps.
Sample Input
5 6 0 0 0 3 4 4 0 1 1 3 3 3 2 2 1 2 3 3 1 1 1 1 3 3 2 2 1 4 4 4
Sample Output
4Hint0 0 0 3 4 4 0 0 0 4 4 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 3 3 3 0 0 3 3 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2 2 1 2 3 3 0 0 3 3 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 3 3 2 2 2 3 3 0 2 2 2 4 4 0 2 2 0 0 0 0 0 0 0 0 0 0 2 2 1 4 4 4 2 2 4 4 4 0 2 2 4 4 4 0 2 2 2 0 0 0 0 0 0 0 0 0
Author
B.A.C
Source
ps:这题和hdu4090很像,基本方法和它一样。
思路:
先bfs查找块(能一次消除的),然后对这些块dfs,然后模拟下降操作和左移操作就够了。
注意:
数组传递,传递的是指针,如果修改值则修改的是实际值,可以好好利用这一特性,同时也要避免犯错。
代码:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#define maxn 7
#define INF 0x3f3f3f3f
using namespace std;
int n,m,ans,sx,sy;
int mp[maxn][maxn];
int dx[]= {-1,1,0,0};
int dy[]= {0,0,-1,1};
struct Node
{
int cnt;
int x[50],y[50];
};
struct node
{
int x,y,c;
}cur,now,q[50];
void bfs(int cxx,int tmp[][7],Node tt[])
{
int i,j,nx,ny,tx,ty,cnt=0;
int head=0,tail=-1;
cur.x=sx;
cur.y=sy;
cur.c=tmp[sx][sy];
tmp[sx][sy]=0;
q[++tail]=cur;
while(head<=tail)
{
now=q[head];
head++;
cnt++;
nx=tt[cxx].x[cnt]=now.x;
ny=tt[cxx].y[cnt]=now.y;
for(i=0;i<4;i++)
{
tx=nx+dx[i];
ty=ny+dy[i];
if(tx<1||tx>n||ty<1||ty>m||tmp[tx][ty]!=now.c) continue ;
cur.x=tx;
cur.y=ty;
cur.c=tmp[tx][ty];
tmp[tx][ty]=0;
q[++tail]=cur;
}
}
tt[cxx].cnt=cnt;
}
int solve(int tmp[][7],Node tt[])
{
int i,j,cxx=0;
for(i=1;i<=n;i++)
{
for(j=1;j<=m;j++)
{
if(tmp[i][j])
{
sx=i,sy=j;
cxx++;
bfs(cxx,tmp,tt);
}
}
}
return cxx;
}
void change(int tmp[7][7])
{
int i,j,pc,flag;
int pp[7][7]={0},hh[7][7]={0},pos[7];
for(j=1;j<=m;j++)
{
pos[j]=n;
}
for(i=n;i>=1;i--) // 模拟下降
{
for(j=1;j<=m;j++)
{
if(tmp[i][j])
{
pp[pos[j]][j]=tmp[i][j];
pos[j]--;
}
}
}
pc=1;
for(j=1;j<=m;j++)
{
flag=0;
for(i=1;i<=n;i++)
{
if(pp[i][j]) flag=1;
}
if(flag)
{
for(i=1;i<=n;i++)
{
hh[i][pc]=pp[i][j];
}
pc++;
}
}
memcpy(tmp,hh,sizeof(hh));
}
void dfs(int p[7][7],int step)
{
int i,j,t;
if(step>=ans) return ;
int tmp[7][7];
Node tt[50];
memcpy(tmp,p,sizeof(tmp)); // 第一次发现这里不能写sizeof(p) 汗 debug了一会
t=solve(tmp,tt);
if(!t)
{
if(ans>step) ans=step;
return ;
}
for(i=1;i<=t;i++)
{
memcpy(tmp,p,sizeof(tmp));
for(j=1;j<=tt[i].cnt;j++)
{
tmp[tt[i].x[j]][tt[i].y[j]]=0;
}
change(tmp);
dfs(tmp,step+1);
}
}
int main()
{
int i,j;
while(~scanf("%d%d",&n,&m))
{
memset(mp,0,sizeof(mp));
for(i=1; i<=n; i++)
{
for(j=1; j<=m; j++)
{
scanf("%d",&mp[i][j]);
}
}
ans=INF;
dfs(mp,0);
printf("%d\n",ans);
}
return 0;
}