Time travel
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 1366 Accepted Submission(s): 303
Problem Description
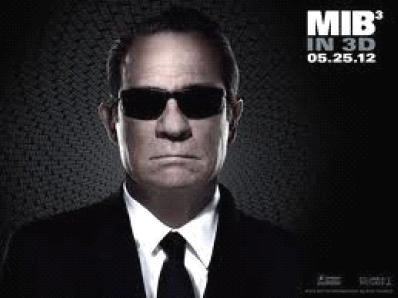
Agent K is one of the greatest agents in a secret organization called Men in Black. Once he needs to finish a mission by traveling through time with the Time machine. The Time machine can take agent K to some point (0 to n-1) on the timeline and when he gets to the end of the time line he will come back (For example, there are 4 time points, agent K will go in this way 0, 1, 2, 3, 2, 1, 0, 1, 2, 3, 2, 1, ...). But when agent K gets into the Time machine he finds it has broken, which make the Time machine can't stop (Damn it!). Fortunately, the time machine may get recovery and stop for a few minutes when agent K arrives at a time point, if the time point he just arrive is his destination, he'll go and finish his mission, or the Time machine will break again. The Time machine has probability Pk% to recover after passing k time points and k can be no more than M. We guarantee the sum of Pk is 100 (Sum(Pk) (1 <= k <= M)==100). Now we know agent K will appear at the point X(D is the direction of the Time machine: 0 represents going from the start of the timeline to the end, on the contrary 1 represents going from the end. If x is the start or the end point of the time line D will be -1. Agent K want to know the expectation of the amount of the time point he need to pass before he arrive at the point Y to finish his mission.
If finishing his mission is impossible output "Impossible !" (no quotes )instead.
Input
There is an integer T (T <= 20) indicating the cases you have to solve. The first line of each test case are five integers N, M, Y, X .D (0< N,M <= 100, 0 <=X ,Y < 100 ). The following M non-negative integers represent Pk in percentile.
Output
For each possible scenario, output a floating number with 2 digits after decimal point
If finishing his mission is impossible output one line "Impossible !"
(no quotes )instead.
If finishing his mission is impossible output one line "Impossible !"
(no quotes )instead.
Sample Input
2 4 2 0 1 0 50 50 4 1 0 2 1 100
Sample Output
8.14 2.00
Source
题意:
给出一个数轴,有一个起点和一个终点,某个人可以走1,2,3……m步,每一种情况有一个概率,初始有一个方向,走到头则返回,问到达终点的期望步数为多少。
思路:
高斯消元概率dp,dp[i]表示走到该点到达终点还需要的步数的期望。
那么有dp[i]=∑pk/100*(dp[i+k]+k)。
方程式很简单的,但是细节很多,具体见代码。
首先由于一些节点到达不了,需要bfs一遍编号。
有一个巧妙的方向处理就是虚拟出n-2个节点(除起点外其他点都有两个点),这样就不用考虑来回走了,加上对称性,如果开始方向为1,可以将起点sx变为cnt-sx,这样方向就不用处理了。
代码:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <cmath>
#include <string>
#include <map>
#include <stack>
#include <vector>
#include <set>
#include <queue>
#define maxn 205
#define MAXN 100005
#define mod 1000000009
#define INF 0x3f3f3f3f
#define pi acos(-1.0)
#define eps 1e-6
typedef long long ll;
using namespace std;
int n,m,sx,ex,dir,flag,cnt,tot;
int pk[maxn],vis[maxn],mp[maxn];
double a[maxn][maxn],x[maxn];
//方程的左边的矩阵和等式右边的值,求解之后xi存的就是第i个未知数的结果 下标从0开始
int equ,var;//方程数和未知数个数
int Gauss()
{
int i,j,k,col,max_r;
for(k=0,col=0; k<equ&&col<var; k++,col++)
{
max_r=k;
for(i=k+1; i<equ; i++)
if(fabs(a[i][col])>fabs(a[max_r][col]))
max_r=i;
if(fabs(a[max_r][col])<eps)return 0;
if(k!=max_r)
{
for(j=col; j<var; j++)
swap(a[k][j],a[max_r][j]);
swap(x[k],x[max_r]);
}
x[k]/=a[k][col];
for(j=col+1; j<var; j++)a[k][j]/=a[k][col];
a[k][col]=1;
for(i=0; i<equ; i++)
if(i!=k)
{
x[i]-=x[k]*a[i][k];
for(j=col+1; j<var; j++)a[i][j]-=a[k][j]*a[i][col];
a[i][col]=0;
}
}
return 1;
}
void bfs()
{
int i,j,t;
queue<int>q;
memset(vis,0,sizeof(vis));
tot=0;
vis[sx]=1;
q.push(sx);
while(!q.empty())
{
int nx=q.front();
mp[nx]=tot++;
q.pop();
for(j=1;j<=m;j++)
{
if(pk[j]==0) continue ;
int pos=(nx+j)%cnt;
if(!vis[pos])
{
vis[pos]=1;
q.push(pos);
}
}
}
}
void solve()
{
int i,j,t,cxx=-1,tmp;
equ=var=tot;
memset(a,0,sizeof(a));
memset(x,0,sizeof(x));
for(i=0; i<=n+n-3; i++) // 建立方程式
{
if(!vis[i]) continue ;
cxx++;
if(i==ex||i+ex==cnt) // 终点
{
a[cxx][mp[i]]=1;
x[cxx]=0;
continue ;
}
t=0;
for(j=1;j<=m;j++)
{
int pos=(i+j)%cnt;
a[cxx][mp[pos]]+=-pk[j];
t+=pk[j]*j;
}
a[cxx][mp[i]]+=100;
x[cxx]=t;
}
Gauss();
printf("%.2f\n",x[mp[sx]]);
}
int main()
{
int i,j,t;
scanf("%d",&t);
while(t--)
{
scanf("%d%d%d%d%d",&n,&m,&ex,&sx,&dir);
for(i=1; i<=m; i++)
{
scanf("%d",&pk[i]);
}
if(sx==ex) // 起点为终点特判 不然会mod 0
{
printf("0.00\n");
continue ;
}
cnt=n+n-2;
if(dir==1) sx=cnt-sx; // 由于对称性 可看做cnt-sx向右走(当然也可以不用 不过取模是要注意)
bfs(); // 给走的到的点编号
if(!vis[ex]&&!vis[cnt-ex]) // 这里要记得 虚拟节点后有两个终点 可能只能一个方向到达终点 如我写的数据
{
printf("Impossible !\n");
continue ;
}
solve();
}
return 0;
}
/*
1
8 7 1 6 0
0 0 0 0 0 0 100
*/