Calendars in widespread use today include the Gregorian calendar, which is the de facto international standard, and is used almost everywhere in the world for civil purposes. The Gregorian reform modified the Julian calendar's scheme of leap years as follows:
Every year that is exactly divisible by four is a leap year, except for years that are exactly divisible by 100; the centurial years that are exactly divisible by 400 are still leap years. For example, the year 1900 is not a leap year; the year 2000 is a leap year.
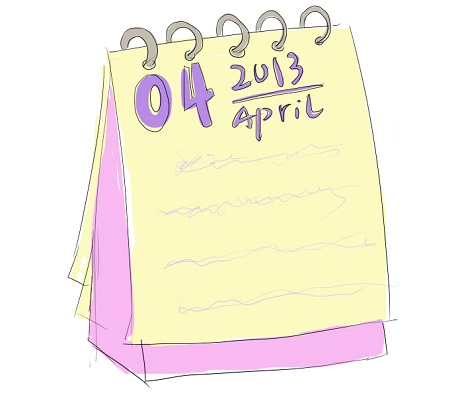
In this problem, you have been given two dates and your task is to calculate how many days are between them. Note, that leap years have unusual number of days in February.
Look at the sample to understand what borders are included in the aswer.
The first two lines contain two dates, each date is in the format yyyy:mm:dd (1900 ≤ yyyy ≤ 2038 and yyyy:mm:dd is a legal date).
Print a single integer — the answer to the problem.
1900:01:01 2038:12:31
50768
1996:03:09 1991:11:12
1579
using namespace std;
int main()
{
int year1,month1,day1,year2,month2,day2;
char a,b,c,d;//因为输入字符串要提取年月日很麻烦,就用a,b,c,d代替:
int i,j,x,y,z,sum,p;//p的作用就是不重复输出结果,如果用你用while循环输入两个日期就不需要p了
cin>>year1>>a>>month1>>b>>day1;
cin>>year2>>c>>month2>>d>>day2;
sum=0;
p=0;
if(year1<year2)//始终保持year1大于year2,这样算的时候方便
{
x=year1;
year1=year2;
year2=x;
y=month1;
month1=month2;
month2=y;
z=day1;
day1=day2;
day2=z;
}
else if(year1==year2)
{
if(month1<month2)
{
if((year2%400)==0||((year2%4==0)&&(year2%100)!=0))
{
for(i=month1;i<month2;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=29;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day2;
sum-=day1;
}
else
{
for(i=month1;i<month2;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=28;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day2;
sum-=day1;
}
p=1;
cout<<sum<<endl;
}
else if(month1==month2)
{
if(day1<day2)
{
cout<<day2-day1<<endl;
}
else if(day1==day2)
{
cout<<0<<endl;
}
else
cout<<day1-day2<<endl;
p=1;
}
else
{
if((year2%400)==0||((year2%4==0)&&(year2%100)!=0))
{
for(i=month2;i<month1;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=29;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day1;
sum-=day2;
}
else
{
for(i=month2;i<month1;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=28;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day1;
sum-=day2;
}
p=1;
cout<<sum<<endl;
}
}
for(i=year2+1;i<year1;i++)
{
if((i%400)==0||((i%4==0)&&(i%100)!=0))
sum+=366;
else
sum+=365;
}
if((year2%400)==0||((year2%4==0)&&(year2%100)!=0))
{
if(month2<=2)
{
for(i=month2;i<=12;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=29;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
}
else
{
for(i=month2;i<=12;i++)
{
if(i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
}
sum-=day2;
}
else
{
for(i=month2;i<=12;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10||i==12)
sum+=31;
if(i==2)
sum+=28;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum-=day2;
}
if((year1%400)==0||((year1%4==0)&&(year1%100)!=0))
{
if(month1>2)
{
for(i=1;i<month1;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10)
sum+=31;
if(i==2)
sum+=29;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day1;
}
else
{
if(month1==1)
sum+=day1;
else
{
sum+=31;
sum+=day1;
}
}
}
else
{
for(i=1;i<month1;i++)
{
if(i==1||i==3||i==5||i==7||i==8||i==10)
sum+=31;
if(i==2)
sum+=28;
if(i==4||i==6||i==9||i==11)
sum+=30;
}
sum+=day1;
}
if(p!=1)
cout<<sum<<endl;
}//如果有问题,或有什么疑惑,可以在评论中提出,小子我看到一定尽力解答