Box
Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Submission(s): 2247 Accepted Submission(s): 676
Problem Description
There are N boxes on the ground, which are labeled by numbers from 1 to N. The boxes are magical, the size of each one can be enlarged or reduced arbitrarily.
Jack can perform the “MOVE x y” operation to the boxes: take out box x; if y = 0, put it on the ground; Otherwise, put it inside box y. All the boxes inside box x remain the same. It is possible that an operation is illegal, that is, if box y is contained (directly or indirectly) by box x, or if y is equal to x.
In the following picture, box 2 and 4 are directly inside box 6, box 3 is directly inside box 4, box 5 is directly inside box 1, box 1 and 6 are on the ground.
The picture below shows the state after Jack performs “MOVE 4 1”:
Then he performs “MOVE 3 0”, the state becomes:
During a sequence of MOVE operations, Jack wants to know the root box of a specified box. The root box of box x is defined as the most outside box which contains box x. In the last picture, the root box of box 5 is box 1, and box 3’s root box is itself.
Jack can perform the “MOVE x y” operation to the boxes: take out box x; if y = 0, put it on the ground; Otherwise, put it inside box y. All the boxes inside box x remain the same. It is possible that an operation is illegal, that is, if box y is contained (directly or indirectly) by box x, or if y is equal to x.
In the following picture, box 2 and 4 are directly inside box 6, box 3 is directly inside box 4, box 5 is directly inside box 1, box 1 and 6 are on the ground.
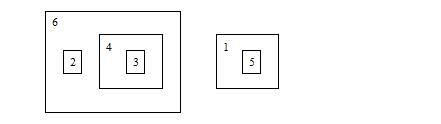
The picture below shows the state after Jack performs “MOVE 4 1”:
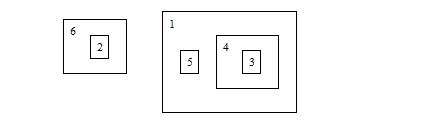
Then he performs “MOVE 3 0”, the state becomes:
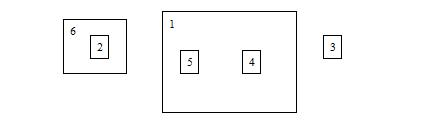
During a sequence of MOVE operations, Jack wants to know the root box of a specified box. The root box of box x is defined as the most outside box which contains box x. In the last picture, the root box of box 5 is box 1, and box 3’s root box is itself.
Input
Input contains several test cases.
For each test case, the first line has an integer N (1 <= N <= 50000), representing the number of boxes.
Next line has N integers: a1, a2, a3, ... , aN (0 <= ai <= N), describing the initial state of the boxes. If ai is 0, box i is on the ground, it is not contained by any box; Otherwise, box i is directly inside box ai. It is guaranteed that the input state is always correct (No loop exists).
Next line has an integer M (1 <= M <= 100000), representing the number of MOVE operations and queries.
On the next M lines, each line contains a MOVE operation or a query:
1. MOVE x y, 1 <= x <= N, 0 <= y <= N, which is described above. If an operation is illegal, just ignore it.
2. QUERY x, 1 <= x <= N, output the root box of box x.
For each test case, the first line has an integer N (1 <= N <= 50000), representing the number of boxes.
Next line has N integers: a1, a2, a3, ... , aN (0 <= ai <= N), describing the initial state of the boxes. If ai is 0, box i is on the ground, it is not contained by any box; Otherwise, box i is directly inside box ai. It is guaranteed that the input state is always correct (No loop exists).
Next line has an integer M (1 <= M <= 100000), representing the number of MOVE operations and queries.
On the next M lines, each line contains a MOVE operation or a query:
1. MOVE x y, 1 <= x <= N, 0 <= y <= N, which is described above. If an operation is illegal, just ignore it.
2. QUERY x, 1 <= x <= N, output the root box of box x.
Output
For each query, output the result on a single line. Use a blank line to separate each test case.
Sample Input
2 0 1 5 QUERY 1 QUERY 2 MOVE 2 0 MOVE 1 2 QUERY 1 6 0 6 4 6 1 0 4 MOVE 4 1 QUERY 3 MOVE 1 4 QUERY 1
Sample Output
1 1 2 1 1
Source
题意:这个题有两种操作:
MOVE x,y 把x为根的子树放到y下
QUERY x查询x的最根父亲
思路:把每个箱子看做x和x+N两个箱子,在中间的就是套在其中的盒子,对于放在地上(即0)的盒子dfs建立书序,然后对着一段建立一棵树。
MOVE的时候,把x放到0下,把x+N方法到x下,然后把x的左子树和x+N的右子树拼接起来,然后把y放到0,紧挨着y的放在y的下面,然后把x当做y的右子树的左子树
#include<iostream>
#include<cstdio>
#include<string>
#include<cstring>
#include<vector>
#include<cmath>
#include<queue>
#include<stack>
#include<map>
#include<set>
#include<algorithm>
using namespace std;
const int maxn=50010*2;
int head[maxn];
int vis[maxn];
vector<int> edge[maxn];
int N,cnt,Q;
int ch[maxn][2],pre[maxn],size[maxn],key[maxn];
int root,tot1;
int a[maxn];
void init()
{
root=tot1=0;
ch[root][0]=ch[root][1]=pre[root]=key[root]=size[root]=0;
}
void NewNode(int &r,int f,int val)
{
r=val;
key[r]=val;
ch[r][0]=ch[r][1]=0;
size[r]=1;
pre[r]=f;
}
void build(int &x,int l,int r,int f)
{
if(l>r)return ;
int mid=(l+r)>>1;
NewNode(x,f,a[mid]);
build(ch[x][0],l,mid-1,x);
build(ch[x][1],mid+1,r,x);
}
void dfs(int u)
{
a[cnt++]=u;
for(int i=0;i<edge[u].size();i++)
dfs(edge[u][i]);
a[cnt++]=u+N;
}
void Rotate(int x,int kind)
{
int y=pre[x];
ch[y][!kind]=ch[x][kind];
pre[ch[x][kind]]=y;
if(pre[y])ch[pre[y]][ch[pre[y]][1]==y]=x;
pre[x]=pre[y];
ch[x][kind]=y;
pre[y]=x;
}
void Splay(int r,int goal)
{
while(pre[r]!=goal)
{
if(pre[pre[r]]==goal)
Rotate(r,ch[pre[r]][0]==r);
else
{
int y=pre[r];
int kind=(ch[pre[y]][0]==y);
if(ch[y][kind]==r)
{
Rotate(r,!kind);
Rotate(r,kind);
}
else
{
Rotate(y,kind);
Rotate(r,kind);
}
}
}
if(goal==0)root=r;
}
//判断y是不是x的孩子
bool can(int x,int y)
{
while(y)
{
if(ch[x+N][0]==y)return true;
y=pre[y];
}
return false;
}
int get_min(int r)
{
while(ch[r][0])
r=ch[r][0];
return r;
}
void Move(int x,int y)
{
if(x==y)return;
Splay(x,0);
Splay(x+N,x);
if(can(x,y))return;
int a=ch[x][0],b=ch[x+N][1];
ch[x][0]=ch[x+N][1]=pre[a]=pre[b]=0;
if(a&&b)
{
b=get_min(b);
ch[b][0]=a;
pre[a]=b;
}
if(y==0)return;
Splay(y,0);
b=get_min(ch[y][1]);
Splay(b,y);
ch[b][0]=x;
pre[x]=b;
}
void Query(int x)
{
Splay(x,0);
printf("%d\n",get_min(x));
}
int main()
{
char op[10];
bool first=true;
while(scanf("%d",&N)!=EOF)
{
if(first)first=false;
else printf("\n");
for(int i=0;i<=N;i++)edge[i].clear();
memset(vis,0,sizeof(vis));
for(int i=1;i<=N;i++)
{
int x;
scanf("%d",&x);
if(x)edge[x].push_back(i);
else vis[i]=true;
}
init();
for(int i=1;i<=N;i++)
{
if(vis[i])
{
cnt=0;
dfs(i);
build(root,0,cnt-1,0);
}
}
scanf("%d",&Q);
int x,y;
while(Q--)
{
scanf("%s%d",op,&x);//cout<<op<<endl;
if(op[0]=='M')
{
scanf("%d",&y);
Move(x,y);
}
else Query(x);
}
}
return 0;
}