写在前面
以下是对GitHub项目 design-pattern 的快览,适合收藏学习,慢慢消化。本文参考《Java与模式》,给出的模式均为基础结构,实际项目中设计模式会有简化或者相互结合的情况。
目录
快览-README.md
关键信息
以下为项目 doc 目录下关于设计模式的 plantuml 类图,具体代码及示例请前往design-pattern 自行获取。
1.创建型
简单工厂模式
/**
* 产品接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Product {
}
/**
* 具体产品实现角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteProduct implements Product{
}
/**
* 工厂角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Creator {
public static Product factory(String type) {
// type=1, ConcreteProduct1
// type=2, ConcreteProduct2
return new ConcreteProduct();
}
}
示例代码:
/**
* 说明:水果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface Fruit {
/**
* 生长
*/
void grow();
/**
* 收获
*/
void harvest();
/**
* 种植
*/
void plant();
}
/**
* 说明:苹果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Apple implements Fruit{
private int treeAge;
@Override
public void grow() {
System.out.println("Apple is growing");
}
@Override
public void harvest() {
System.out.println("Apple has been harvested");
}
@Override
public void plant() {
System.out.println("Apple has been planted");
}
public int getTreeAge() {
return treeAge;
}
public void setTreeAge(int treeAge) {
this.treeAge = treeAge;
}
}
/**
* 说明:葡萄
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Grape implements Fruit {
private boolean seedless;
@Override
public void grow() {
System.out.println("Grape is growing");
}
@Override
public void harvest() {
System.out.println("Grape has been harvested");
}
@Override
public void plant() {
System.out.println("Grape has been planted");
}
public boolean isSeedless() {
return seedless;
}
public void setSeedless(boolean seedless) {
this.seedless = seedless;
}
}
/**
* 说明:草莓
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Strawberry implements Fruit {
@Override
public void grow() {
System.out.println("Strawberry is growing");
}
@Override
public void harvest() {
System.out.println("Strawberry has been harvested");
}
@Override
public void plant() {
System.out.println("Strawberry has been planted");
}
}
/**
* 说明:园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class FruitGardener {
/**
* 静态工厂
* @param witch
* @return
*/
public static Fruit factory(String witch) {
if (witch.equalsIgnoreCase("Apple")) {
return new Apple();
} else if (witch.equalsIgnoreCase("Grape")) {
return new Grape();
} else if (witch.equalsIgnoreCase("Strawberry")) {
return new Strawberry();
}
throw new BadFruitException("Bad fruit request");
}
}
/**
* 说明:客户端
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Client {
public static void main(String[] args) {
Fruit apple = FruitGardener.factory("Apple");
apple.grow();
}
}
工厂方法模式
/**
* 产品接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Product {
}
/**
* 工厂接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Creator {
Product factory();
}
/**
* 具体产品实现角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteProduct1 implements Product{
}
/**
* 具体产品实现角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteProduct2 implements Product{
}
/**
* 具体工厂
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteCreator1 implements Creator {
@Override
public Product factory() {
return new ConcreteProduct1();
}
}
/**
* 具体工厂
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteCreator2 implements Creator {
@Override
public Product factory() {
return new ConcreteProduct2();
}
}
/**
* 客户端
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
private static Creator creator1, creator2;
private static Product product1, product2;
public static void main(String[] args) {
creator1 = new ConcreteCreator1();
product1 = creator1.factory();
creator2 = new ConcreteCreator2();
product2 = creator2.factory();
}
}
示例代码:
/**
* 说明:水果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface Fruit {
/**
* 生长
*/
void grow();
/**
* 收获
*/
void harvest();
/**
* 种植
*/
void plant();
}
/**
* 说明:苹果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Apple implements Fruit {
private int treeAge;
@Override
public void grow() {
System.out.println("Apple is growing");
}
@Override
public void harvest() {
System.out.println("Apple has been harvested");
}
@Override
public void plant() {
System.out.println("Apple has been planted");
}
public int getTreeAge() {
return treeAge;
}
public void setTreeAge(int treeAge) {
this.treeAge = treeAge;
}
}
/**
* 说明:葡萄
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Grape implements Fruit {
private boolean seedless;
@Override
public void grow() {
System.out.println("Grape is growing");
}
@Override
public void harvest() {
System.out.println("Grape has been harvested");
}
@Override
public void plant() {
System.out.println("Grape has been planted");
}
public boolean isSeedless() {
return seedless;
}
public void setSeedless(boolean seedless) {
this.seedless = seedless;
}
}
/**
* 说明:草莓
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Strawberry implements Fruit {
@Override
public void grow() {
System.out.println("Strawberry is growing");
}
@Override
public void harvest() {
System.out.println("Strawberry has been harvested");
}
@Override
public void plant() {
System.out.println("Strawberry has been planted");
}
}
/**
* 说明:园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface FruitGardener {
Fruit factory();
}
/**
* 说明:苹果园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class AppleGardener implements FruitGardener {
@Override
public Fruit factory() {
return new Apple();
}
}
/**
* 说明:葡萄园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class GrapeGardener implements FruitGardener {
@Override
public Fruit factory() {
return new Grape();
}
}
/**
* 说明:草莓园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class StrawberryGardener implements FruitGardener {
@Override
public Fruit factory() {
return new Strawberry();
}
}
/**
* 说明:客户端
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Client {
public static void main(String[] args) {
FruitGardener appleGarden = new AppleGardener();
appleGarden.factory();
appleGarden.factory().grow();
}
}
抽象工厂模式
/**
* 产品A接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface ProductA {
}
/**
* 产品B接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface ProductB {
}
/**
* 工厂接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Creator {
ProductA factoryA();
ProductB factoryB();
}
/**
* 具体产品A实现1角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ProductA1 implements ProductA {
}
/**
* 具体产品A实现2角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ProductA2 implements ProductA {
}
/**
* 具体产品B实现1角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ProductB1 implements ProductB {
}
/**
* 具体产品B实现2角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ProductB2 implements ProductB {
}
/**
* 具体工厂1
* 生产产品A1和产品B1
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteCreator1 implements Creator {
@Override
public ProductA factoryA() {
return new ProductA1();
}
@Override
public ProductB factoryB() {
return new ProductB1();
}
}
/**
* 具体工厂2
* 生产产品A2和产品B2
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteCreator2 implements Creator {
@Override
public ProductA factoryA() {
return new ProductA2();
}
@Override
public ProductB factoryB() {
return new ProductB2();
}
}
示例代码:
/**
* 说明:水果
* 标识接口
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface Fruit {
}
/**
* 说明:蔬菜
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface Veggie {
}
/**
* 说明:园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public interface Gardener {
/**
* 创建水果
*/
Fruit factoryFruit(String name);
/**
* 创建蔬菜
*/
Veggie factoryVeggie(String name);
}
/**
* 说明:北方水果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class NorthernFruit implements Fruit{
private String name;
public NorthernFruit(String name) {
System.out.println("NorthernFruit has been factory, name is " + name);
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
/**
* 说明:北方蔬菜
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class NorthernVeggie implements Veggie{
private String name;
public NorthernVeggie(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
/**
* 说明:热带水果
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class TropicalFruit implements Fruit{
private String name;
public TropicalFruit(String name) {
System.out.println("TropicalFruit has been factory, name is " + name);
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
/**
* 说明:热带蔬菜
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class TropicalVeggie implements Veggie{
private String name;
public TropicalVeggie(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
/**
* 说明:北方园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class NorthernGardener implements Gardener {
@Override
public Fruit factoryFruit(String name) {
return new NorthernFruit(name);
}
@Override
public Veggie factoryVeggie(String name) {
return new NorthernVeggie(name);
}
}
/**
* 说明:热带园丁
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class TropicalGardener implements Gardener {
@Override
public Fruit factoryFruit(String name) {
return new TropicalFruit(name);
}
@Override
public Veggie factoryVeggie(String name) {
return new TropicalVeggie(name);
}
}
/**
* 说明:客户端
*
* @author : qiuxb
* @date : 2022/4/13
*/
public class Client {
public static void main(String[] args) {
Gardener tropicalGardener = new TropicalGardener();
tropicalGardener.factoryFruit("Apple");
}
}
单例-恶汉模式
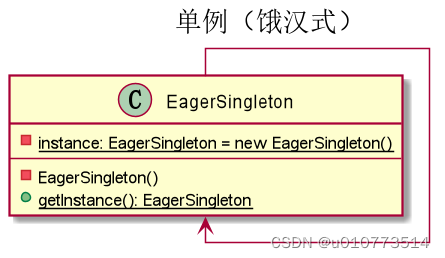
/**
* 饿汉模式
*
* @authoro qiuxb
* @date 2021/8/19
*/
public class HungarySingleton {
private static final HungarySingleton instance = new HungarySingleton();
public static HungarySingleton getInstance() {
return instance;
}
private HungarySingleton() {
}
}
单例-懒汉模式
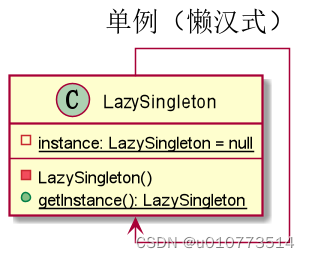
/**
* 懒汉模式
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class LazySingleton {
private volatile static LazySingleton instance;
private LazySingleton() {}
public static LazySingleton getInstance() {
if (instance == null) {
synchronized (LazySingleton.class) {
if (instance == null) {
instance = new LazySingleton();
}
}
}
return instance;
}
}
建造者模式
/**
* 产品
* 由多个部分组成
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Product {
}
/**
* 抽象建造者角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Builder {
abstract public void buildPart1();
abstract public void buildPart2();
abstract public Product retrieveResult();
}
/**
* 具体建造者角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteBuilder extends Builder{
private Product product = new Product();
@Override
public void buildPart1() {
}
@Override
public void buildPart2() {
}
@Override
public Product retrieveResult() {
return this.product;
}
}
/**
* 导演角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Director {
private Builder builder;
public void construct() {
builder = new ConcreteBuilder();
builder.buildPart1();
builder.buildPart2();
Product product = builder.retrieveResult();
}
}
原型模式
/**
* 原型接口
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Prototype extends Cloneable{
Prototype clone();
}
/**
* 具体原型实现
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcretePrototype implements Prototype {
@Override
public Prototype clone() {
try {
// Object#clone()
return (Prototype) super.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
return null;
}
}
}
/**
* 客户端
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
public static void main(String[] args) {
Prototype p = new ConcretePrototype();
System.out.println(p);
Prototype clone = p.clone();
System.out.println(clone);
// false
System.out.println(p == clone);
}
}
2.结构型
外观/门面模式
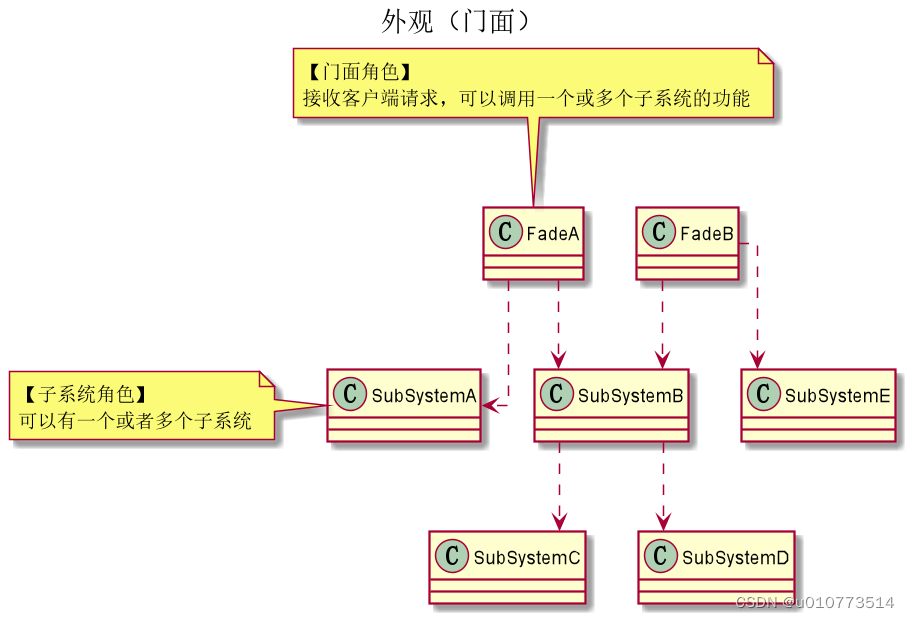
代理模式
/**
* 抽象主题
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Subject {
public abstract void request();
}
/**
* 具体主题
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class RealSubject extends Subject {
@Override
public void request() {
}
}
/**
* 代理主题
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ProxySubject extends Subject{
private Subject subject;
@Override
public void request() {
if (subject == null) {
subject = new RealSubject();
}
preRequest();
subject.request();
afterRequest();
}
private void preRequest() {
}
private void afterRequest() {
}
}
示例代码:
package cn.thinkinjava.design.pattern;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
/**
* JDK动态代理
*
* @author qiuxianbao
* @date 2023/12/27
*/
public class JDKProxyClient {
public static void main(String[] args) {
Car googleCar = new GoogleCar();
googleCar.start();
// 代理 googleCar,在start前进行 GoogleCar check
// Car proxy = (Car) Proxy.newProxyInstance(GoogleCar.class.getClassLoader(), GoogleCar.class.getInterfaces(), new InvocationHandler() {
Car proxy = (Car) Proxy.newProxyInstance(GoogleCar.class.getClassLoader(), new Class[]{Car.class}, new InvocationHandler() {
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
// 代理的方法
if ("start".equals(method.getName())) {
System.out.println("GoogleCar check");
}
return method.invoke(googleCar, args);
}
});
proxy.start();
}
}
/**
* 实现类
*/
class GoogleCar implements Car {
@Override
public void start() {
System.out.println("GoogleCar start");
}
@Override
public void run() {
System.out.println("GoogleCar run");
}
@Override
public void stop() {
System.out.println("GoogleCar stop");
}
}
/**
* 接口
*/
interface Car {
void start();
void run();
void stop();
}
以下是实际业务场景中,使用自定义注解@CustomFeignClient 和 @CustomRequestMapping
实现Http的请求操作。相比工具类,这种方式可以少写接口的实现类,具体请前往GitHub获取代码。
装饰者模式
/**
* 构件接口
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Component {
void sampleOperation();
}
/**
* 具体构件角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteComponent implements Component {
@Override
public void sampleOperation() {
}
}
/**
* 装饰者角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Decorator implements Component {
private Component component;
// 如果没有空参构造,子类就必须要实现了有参构造
public Decorator() {
}
public Decorator(Component component) {
this.component = component;
}
@Override
public void sampleOperation() {
component.sampleOperation();
}
}
/**
* 具体装饰者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteDecorator extends Decorator{
@Override
public void sampleOperation() {
super.sampleOperation();
// 以下为扩充功能
}
}
适配器模式
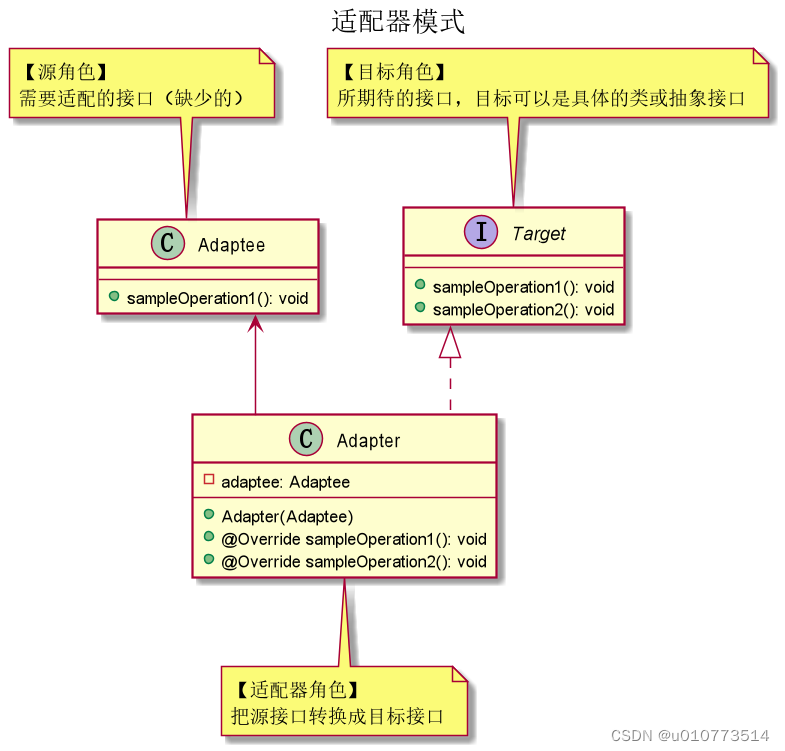
/**
* 源角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Adaptee {
public void sampleOperation1() {
}
}
/**
* 目标角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Target {
void sampleOperation1();
void sampleOperation2();
}
/**
* 适配器角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Adapter implements Target{
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void sampleOperation1() {
adaptee.sampleOperation1();
}
@Override
public void sampleOperation2() {
}
}
桥接模式
/**
* 实现化角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Implementor {
public abstract void operationImpl();
}
/**
* 抽象化角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Abstraction {
protected Implementor impl;
public void operation() {
impl.operationImpl();
}
}
/**
* 具体实现化角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteImplementor extends Implementor {
@Override
public void operationImpl() {
}
}
/**
* 修正抽象化角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class RefinedAbstraction extends Abstraction {
@Override
public void operation() {
// 重写抽象化角色
}
}
组合模式
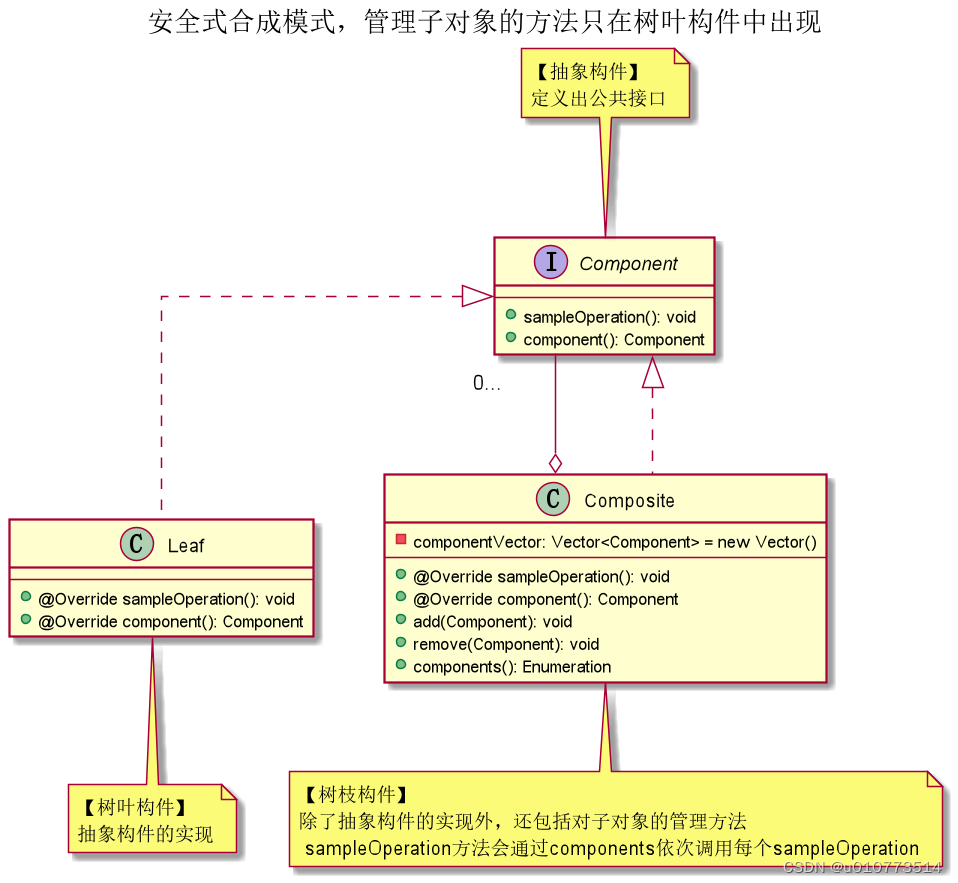
/**
* 抽象构件角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Component {
void sampleOperation();
Component component();
}
/**
* 树叶结点构件角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Leaf implements Component{
@Override
public void sampleOperation() {
}
@Override
public Component component() {
return this;
}
}
/**
* 树枝结点角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Composite implements Component {
private Vector<Component> componentVector = new Vector<>();
@Override
public void sampleOperation() {
Enumeration<Component> enumeration = components();
while (enumeration.hasMoreElements()) {
enumeration.nextElement().sampleOperation();
}
}
@Override
public Component component() {
return this;
}
public void add(Component c) {
componentVector.add(c);
}
public void remove(Component c) {
componentVector.remove(c);
}
public Enumeration<Component> components() {
return componentVector.elements();
}
}
享元模式
/**
* 抽象享元角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Flyweight {
abstract public void operation(String state);
}
/**
* 具体享元角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteFlyweight extends Flyweight {
// 内蕴状态
private Character intrinsicState;
public ConcreteFlyweight(Character intrinsicState) {
this.intrinsicState = intrinsicState;
}
@Override
public void operation(String state) {
System.out.println(String.format("intrinsicState is %s, state is %s", intrinsicState, state));
}
}
/**
* 享元工厂
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class FlyweightFactory {
private Map<Character, Flyweight> cache = new HashMap<>();
public Flyweight factory(Character intrinsicState) {
Flyweight fly = cache.get(intrinsicState);
if (fly == null) {
fly = new ConcreteFlyweight(intrinsicState);
cache.put(intrinsicState, fly);
}
return fly;
}
public void checkFlyweight() {
int i = 0;
for (Iterator it = cache.entrySet().iterator(); it.hasNext();) {
Map.Entry entry = (Map.Entry) it.next();
System.out.println(String.format("Item%s: key is %s", i++, entry.getKey()));
}
}
}
3.行为型
策略模式
/**
* 抽象策略角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Strategy {
public abstract void strategyInterface();
}
/**
* 具体策略角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteStrategy extends Strategy{
@Override
public void strategyInterface() {
}
}
/**
* 环境角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Context {
private Strategy strategy;
public void contextInterface() {
strategy.strategyInterface();
}
}
模板模式
/**
* 抽象模板角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class AbstractClass {
public void templateMethod() {
doOperation1();
doOperation2();
doOperation3();
}
protected abstract void doOperation1();
protected abstract void doOperation2();
private final void doOperation3() {
// 已经实现,不需要子类实现,以及继承
}
}
/**
* 具体模板角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteClass extends AbstractClass{
@Override
protected void doOperation1() {
}
@Override
protected void doOperation2() {
}
}
观察者模式
/**
* 观察者接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Observer {
void update();
}
/**
* 主题接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Subject {
void attach(Observer observer);
void detach(Observer observer);
void notifyObservers();
}
/**
* 具体观察者实现
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteObserver implements Observer{
@Override
public void update() {
}
}
/**
* 具体主题
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteSubject implements Subject {
private Vector<Observer> observers = new Vector<>();
@Override
public void attach(Observer observer) {
observers.addElement(observer);
}
@Override
public void detach(Observer observer) {
observers.removeElement(observer);
}
@Override
public void notifyObservers() {
Enumeration<Observer> observers = observers();
while (observers.hasMoreElements()) {
Observer observer = observers.nextElement();
observer.update();
}
}
private Enumeration<Observer> observers() {
return observers.elements();
}
}
迭代器模式
/**
* 迭代器接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Iterator {
void first();
void next();
boolean done();
Object currentItem();
}
/**
* 抽象集合
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Aggregation {
public abstract Iterator createIterator();
}
/**
* 具体集合
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteAggregation extends Aggregation{
private Object[] objects = {"a", "b", "c", "d"};
@Override
public Iterator createIterator() {
return new ConcreteIterator(this);
}
public int size() {
return objects.length;
}
public Object getElement(int index) {
if (index < objects.length) {
return objects[index];
} else {
return null;
}
}
}
/**
* 具体迭代器
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteIterator implements Iterator{
private ConcreteAggregation agg;
private int index, size;
public ConcreteIterator(ConcreteAggregation agg) {
this.agg = agg;
this.index = 0;
this.size = agg.size();
}
@Override
public void first() {
index = 0;
}
@Override
public void next() {
if (index < size) {
index++;
}
}
@Override
public boolean done() {
return index >= size;
}
@Override
public Object currentItem() {
return agg.getElement(index);
}
}
责任链模式
/**
* 抽象处理者角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Handler {
protected Handler successor;
public abstract void handlerRequest();
public void setSuccessor(Handler successor) {
this.successor = successor;
}
public Handler getSuccessor() {
return successor;
}
}
/**
* 具体处理者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteHandler extends Handler{
@Override
public void handlerRequest() {
Handler successor = getSuccessor();
if (successor != null) {
System.out.println("The request is passed to " + getSuccessor() );
successor.handlerRequest();
} else {
System.out.println( this + " handled request");
}
}
}
/**
* 客户端
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
public static void main(String[] args) {
ConcreteHandler h3 = new ConcreteHandler();
ConcreteHandler h2 = new ConcreteHandler();
h2.setSuccessor(h3);
ConcreteHandler h1 = new ConcreteHandler();
h1.setSuccessor(h2);
// 调用
h1.handlerRequest();
}
}
命令者模式
/**
* 命令接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Command {
void execute();
}
/**
* 命令调用者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Invoker {
private Command command;
public Invoker(Command command) {
this.command = command;
}
public void action() {
command.execute();
}
}
/**
* 命令接收者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Receiver {
public Receiver() {
}
public void action() {
System.out.println("Receiver action");
}
}
/**
* 具体命令实现
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteCommand implements Command{
private Receiver receiver;
public ConcreteCommand(Receiver receiver) {
this.receiver = receiver;
}
@Override
public void execute() {
receiver.action();
}
}
/**
* 客户端
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
public static void main(String[] args) {
Receiver receiver = new Receiver();
Command command = new ConcreteCommand(receiver);
Invoker invoker = new Invoker(command);
invoker.action();
}
}
备忘录模式
/**
* 备忘录角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Memento {
private String state;
public Memento(String state) {
this.state = state;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
}
/**
* 发起人角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Originator {
private String state;
public Memento createMemento() {
return new Memento(state);
}
public void restoreMemento(Memento memento) {
this.state = memento.getState();
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
@Override
public String toString() {
return "Originator{" +
"state='" + state + '\'' +
'}';
}
}
/**
* 负责人角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Caretaker {
private Memento memento;
public void saveMemento(Memento memento) {
this.memento = memento;
}
public Memento retrieveMemento() {
return memento;
}
}
/**
* 客户端
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
public static void main(String[] args) {
Originator originator = new Originator();
originator.setState("up");
System.out.println(originator);
Caretaker caretaker = new Caretaker();
caretaker.saveMemento(originator.createMemento());
originator.setState("down");
System.out.println(originator);
originator.restoreMemento(caretaker.retrieveMemento());
System.out.println(originator);
}
}
状态模式
/**
* 状态接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface State {
void sampleOperation();
}
/**
* 具体状态角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ConcreteState implements State{
@Override
public void sampleOperation() {
}
}
/**
* 环境角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Context {
private State state;
public void sampleOperation() {
state.sampleOperation();
}
public void setState(State state) {
this.state = state;
}
}
访问者模式
/**
* 访问者接口角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public interface Visitor {
void visit(NodeA node);
void visit(NodeB node);
}
/**
* 抽象结点角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public abstract class Node {
public abstract void accept(Visitor visitor);
}
/**
* 具体结点角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class NodeA extends Node{
@Override
public void accept(Visitor visitor) {
System.out.println("NodeA accept");
visitor.visit(this);
}
public void operationA() {
System.out.println("NodeA operationA");
}
}
/**
* 具体结点角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class NodeB extends Node{
@Override
public void accept(Visitor visitor) {
System.out.println("NodeB accept");
visitor.visit(this);
}
public void operationB() {
System.out.println("NodeB operationB");
}
}
/**
* 具体访问者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class VisitorA implements Visitor{
@Override
public void visit(NodeA node) {
System.out.println("VisitorA visit NodeA");
node.operationA();
}
@Override
public void visit(NodeB node) {
System.out.println("VisitorA visit NodeB");
node.operationB();
}
}
/**
* 具体访问者
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class VisitorB implements Visitor{
@Override
public void visit(NodeA node) {
System.out.println("VisitorB visit NodeA");
node.operationA();
}
@Override
public void visit(NodeB node) {
System.out.println("VisitorB visit VisitorB");
node.operationB();
}
}
/**
* 结点对象角色
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class ObjectStruct {
private Vector<Node> nodes;
public ObjectStruct() {
this.nodes = new Vector<>();
}
public void action(Visitor visitor) {
Enumeration<Node> elements = nodes.elements();
while (elements.hasMoreElements()) {
Node node = elements.nextElement();
node.accept(visitor);
}
}
public void add(Node node) {
nodes.addElement(node);
}
}
/**
* 客户端
*
* @author qiuxianbao
* @date : 2023/1/13
*/
public class Client {
public static void main(String[] args) {
ObjectStruct struct = new ObjectStruct();
struct.add(new NodeA());
struct.add(new NodeB());
struct.action(new VisitorB());
}
}
示例代码:
/**
* 访问者
* @author qiuxianbao
* @since 1.0.0
*/
public interface Visitor {
void visit(Engineer engineer);
void visit(Manager manager);
}
/**
* 员工
* @author qiuxianbao
* @since 1.0.0
*/
public abstract class Staff {
public String name;
public int kpi;
public Staff(String name) {
this.name = name;
this.kpi = new Random().nextInt(10);
}
public abstract void accept(Visitor visitor);
}
/**
* 员工实现类
* @author qiuxianbao
* @since 1.0.0
*/
public class Engineer extends Staff{
public Engineer(String name) {
super(name);
}
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
/**
* 员工实现类
* @author qiuxianbao
* @since 1.0.0
*/
public class Manager extends Staff{
public Manager(String name) {
super(name);
}
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
/**
* CEO
* @author qiuxianbao
* @since 1.0.0
*/
public class CEOVisitor implements Visitor{
@Override
public void visit(Engineer engineer) {
System.out.println(engineer.name + ", " + engineer.kpi + ", ceo other");
}
@Override
public void visit(Manager manager) {
System.out.println(manager.name + ", " + manager.kpi + ", ceo other");
}
}
/**
* CTO
* @author qiuxianbao
* @since 1.0.0
*/
public class CTOVisitor implements Visitor{
@Override
public void visit(Engineer engineer) {
System.out.println(engineer.name + ", " + engineer.kpi + ", cto other");
}
@Override
public void visit(Manager manager) {
System.out.println(manager.name + ", " + manager.kpi + ", cto other");
}
}
/**
* 访问者模式
* 让访问者来访问元素,具体不同放在访问者处
*
* 角色:元素接口/抽象类(accept)、元素实现类、元素集合
* 访问者(visit)、访问者实现类
*
* 场景:报表
*
* @author qiuxianbao
* @since 1.0.0
*/
public class Client {
public static void main(String[] args) {
Report report = new Report();
System.out.println("====CEO====");
report.show(new CEOVisitor());
System.out.println("====CTO====");
report.show(new CTOVisitor());
// 变体
Staff s = new Engineer("engineer");
s.accept(new CTOVisitor());
}
}
中介者模式
解释者模式
…
写在后面
如果本文内容对您有价值或者有启发的话,欢迎点赞、关注、评论和转发。您的反馈和陪伴将促进我们共同进步和成长。