Hibernate是什么
Hibernate是一个轻量级的ORMapping框架
ORMapping原理(Object Relational Mapping)就是把对象里面的数据和数据库里面的数据,按照一定的规则进行映射的过程。
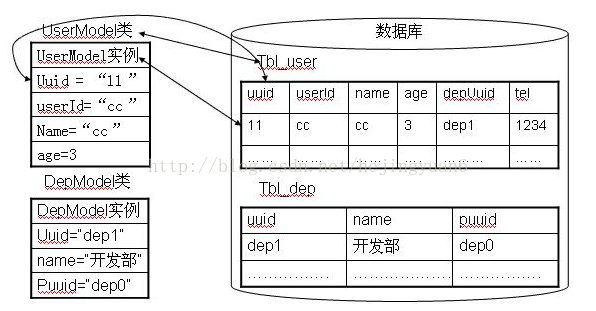
ORMapping基本对应规则:
1:类跟表相对应
2:类的属性跟表的字段相对应
3:类的实例与表中具体的一条记录相对应
Hibernate的实现方式
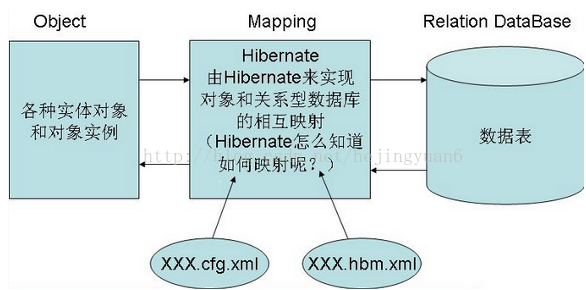
Hibernate解决的问题:
通过上图我们也能发现,Hibernate主要用来实现Java对象和表之间的映射,除此之外还提供数据查询和获取数据的方法,可以大幅度减少开发时人工使用SQL和JDBC处理数据的时间。
Hibernate的目标是对于开发者通常的数据持久化相关的编程任务中解放出来。
Hibernate可以帮助你消除或者包装那些针对特定厂商的SQL代码,并且帮你把结果集从表格式的表示形式转换到一系列的对象去。
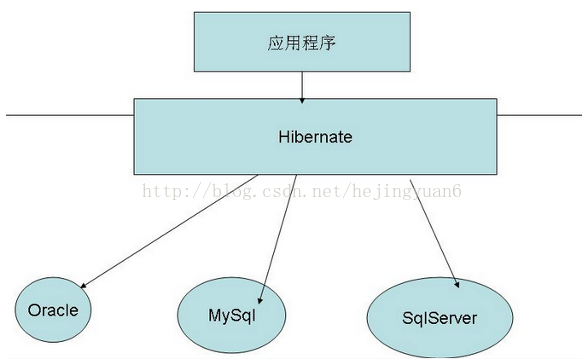
hibernate优点:
1、封装了jdbc,简化了很多重复性代码。
2、简化了DAO层编码工作,使开发更对象化了。
3、移植性好,支持各种数据库,如果换个数据库只要在配置文件中变换配置就可以了,不用改变hibernate代码。
4、支持透明持久化,因为hibernate操作的是纯粹的(pojo)java类,没有实现任何接口,没有侵入性。所以说它是一个轻量级框架。
下面结合应用实例帮助大家理解:
实体类:
- import java.util.Date;
- public class User {
-
- private String id;
- private String username;
- private String userpassword;
- private String createTime;
- private String expireTime;
-
- public String getId() {
- return id;
- }
- public void setId(String id) {
- this.id = id;
- }
- public String getUsername() {
- return username;
- }
- public void setUsername(String name) {
- this.username = name;
- }
- public String getUserpassword() {
- return userpassword;
- }
- public void setUserpassword(String password) {
- this.userpassword = password;
- }
- public String getCreateTime() {
- return createTime;
- }
- public void setCreateTime(String createTime) {
- this.createTime = createTime;
- }
- public String getExpireTime() {
- return expireTime;
- }
- public void setExpireTime(String expireTime) {
- this.expireTime = expireTime;
- }
- }
创建hibernate映射文件User.hbm.xml
映射文件告诉Hibernate它应该访问数据库里面的哪个表(table)和应该使用表里面的哪些字段(column)。
因为这里只有一个Class ---User和一个Table --- t_user,你只需要建立一个映射文件---User.hbm.xml,来对应User类和t_user表之间的关系。
- <hibernate-mapping package="com.bjpowernode.hibernate">
- <class name="User" table="t_user">
- <id name="id">
- <generator class="uuid"></generator>
- </id>
- <property name="username"></property>
- <property name="userpassword"></property>
- <property name="createTime"></property>
- <property name="expireTime"></property>
- </class>
-
- </hibernate-mapping>
配置Hibernate描述文件hibernate.cfg.xml
Hibernate描述文件可以是一个properties或xml文件,其中最重要的是定义数据库的连接。我这里列出的是一个XML格式的hibernate.cfg.xml描述文件。
- <hibernate-configuration>
- <session-factory >
- <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property>
- <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate_first</property>
- <property name="hibernate.connection.username">root</property>
- <property name="hibernate.connection.password">hejingyuan</property>
-
- <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
- <property name="hibernate.show.sql">true</property>
- <property name="hibernate.show.format_sql">true</property>
-
- <mapping resource="com/bjpowernode/hibernate/User.hbm.xml"/>
- </session-factory>
- </hibernate-configuration>
生成表方法
-
-
-
-
-
- public class ExportDB {
-
- public static void main(String[] args) {
-
- Configuration cfg = new Configuration().configure();
- SchemaExport export = new SchemaExport(cfg);
- export.create(true, true);
- }
- }
添加用户
- public static void main(String[] args) {
-
- Configuration cfg=new Configuration().configure();
-
-
- SessionFactory factory=cfg.buildSessionFactory();
-
-
- Session session=null;
- try{
- session=factory.openSession();
-
- session.beginTransaction();
- User user=new User();
- user.setUsername("张三");
- user.setUserpassword("123");
- user.setCreateTime(new Date().toString());
- user.setExpireTime(new Date().toString());
-
-
- session.save(user);
-
- session.getTransaction().commit();
-
- }catch(Exception e){
- e.printStackTrace();
-
- session.getTransaction().rollback();
- }finally{
- if(session != null){
- if(session.isOpen()){
-
- session.close();
- }
- }
- }
-
- }
总结:
千里之行始于足下,这篇文章仅是迈向Hibernate大道的一个起点。以上只是简单介绍了Hibernate解决的问题,其实Hibernate就是一个转换器,完成对象实例与数据库表的转换,对JDBC访问数据库的代码进行封装,简化了数据访问层繁琐的重复性代码。