Vue:渐进式JavaScript框架
Vue基本使用
Vue文件cdn引用:https://cdn.bootcdn.net/ajax/libs/vue/2.6.8/vue.js
模版语法
指令-[v-cloak, v-on, v-model, v-text, v-html, v-pre, v-once, v-bind, v-for, v-if, v-else-if, v-else, v-show, v-slot]
数据绑定指令
双向数据绑定
事件绑定 (v-on:click,调用方法,传参)
事件绑定-修饰符 (事件修饰符,按键修饰符,自定义按键修饰符)
属性绑定 (v-on,v-model)
样式绑定(class样式处理,style样式处理)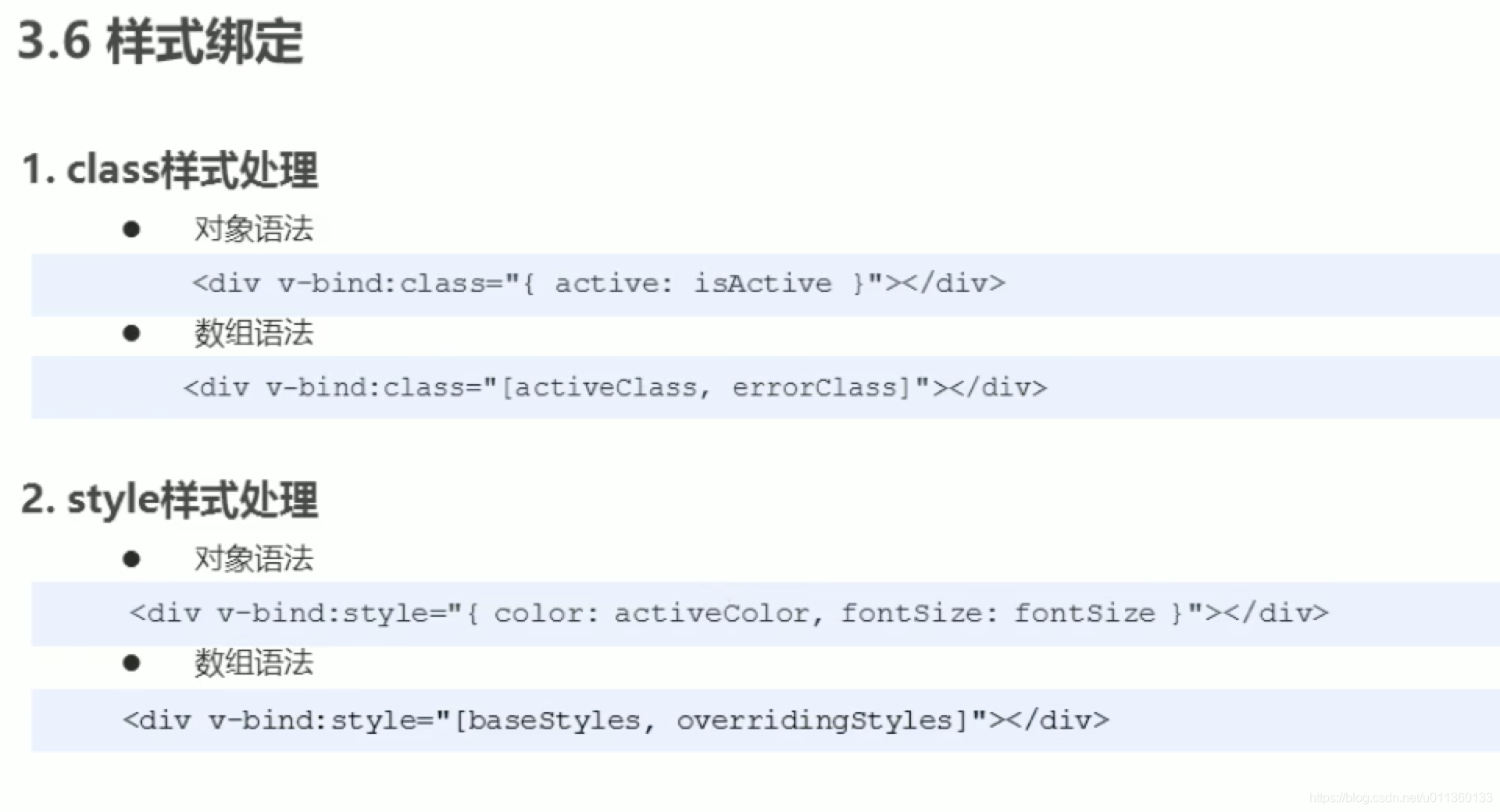
class样式处理代码(数组语法和对象语法混用,默认class处理)
style样式处理代码
分支结构
基础案例
tab选项卡切换
Vue常用特性
表单
表单域修饰符
lazy:失去焦点后v-model响应的值才会修改
自定义指令
组件中定义局部指令
计算属性
侦听器
过滤器
局部过滤器
带参数过滤器
生命周期
补充知识
数组添加响应式
对象添加响应式
综合案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
html,body{
padding: 0;
margin: 0;
}
.wrap{
width: 800px;
margin: 0 auto;
}
table{
width: 100%;
text-align: center;
border-collapse: collapse;
}
table td,table th{
margin: 0;
border: 1px solid #e6eee6;
height: 40px;
}
[v-cloak] { display: none }
</style>
</head>
<body>
<div class="wrap" id="app" v-cloak>
<div>
编号:
<input type="text"
v-model="id"/>
名称:
<input type="text"
v-model.lazy="name"
v-focus/>
<input type="submit" value="提交" @click="add" />
</div>
<table>
<thead>
<tr>
<th>编号</th>
<th>名称</th>
<th>时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="item in bookList"
:key="item.id"
>
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{ item.date | format('yyyy-MM-dd') }}</td>
<td>
<!-- <a href="" @click.prevent="toEdit(item)">修改</a> -->
<a href="" @click.prevent="toEdit(item.id)">修改</a>
<span>|</span>
<a href="" @click.prevent>删除</a>
</td>
</tr>
</tbody>
</table>
<div class="">{{bookCount}}</div>
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/vue/2.6.8/vue.js"></script>
<script>
// 过滤器
Vue.filter('format', function(value, format){
function dateFormat(date, format){
if(typeof date === "string"){
// /Date((\d+))/
var mts = date.match(/(\/Date\((\d+)\)\/)/);
if(mts && mts.length >= 3){
date = parseInt(mts[2]);
}
}
date = new Date(date);
if(!date || date.toUTCString() == "Invalid Date") {
return '';
}
var map = {
"M": date.getMonth() + 1, // 月份
"d": date.getDate(), // 日
"h": date.getHours(), // 小时 Hour
"m": date.getMinutes(), // 分 Minute
"s": date.getSeconds(), // 秒 Second
"q": Math.floor((date.getMonth() + 3) / 3), // 季度
"S": date.getMilliseconds() // 毫秒
};
format = format.replace(/([yMdhmsqS])+/g, function(all, t){
var v = map[t];
if (v !== undefined){
if (all.length > 1){
v = '0' + v;
v = v.substr(v.length - 2);
}
return v;
} else if (t === 'y'){
return (date.getFullYear() + '').substr(4 - all.length);
}
return all;
})
return format;
}
return dateFormat(value, format);
});
// 自定义指令
Vue.directive('focus', {
inserted: function (el) {
el.focus();
}
})
var vm = new Vue({
el: '#app',
data: {
id: '',
name: '',
date: '',
bookList: []
},
computed: {
bookCount() {
return this.bookList.length;
}
},
watch: {
name(val) {
console.log(val);
var flag = this.bookList.some(function(item){
return item.name == val;
});
console.log(flag);
if(flag){
console.log('名称已存在');
}else{
console.log('名称不存在')
}
}
},
methods: {
add() {
// 添加图书
var book = {};
book.id = this.id;
book.name = this.name;
book.date = this.date;
this.bookList.push(book);
// 清空表单
this.id = '';
this.name = '';
},
toEdit(id) {
var book = this.bookList.filter(function (item) {
return item.id == id;
})
console.log(book);
this.id = book[0].id;
this.name = book[0].name;
// console.log(item)
}
},
mounted() {
// 该生命周期钩子函数被出发的时候,模版已经可以使用
// 一般此时获取后台数据,然后把数据填充到模版
this.bookList = [{
id: 1,
name: '三国演义',
date: new Date()
},{
id: 2,
name: '西游记',
date: ''
},{
id: 3,
name: '水浒传',
date: ''
},]
},
})
</script>
</body>
</html>