一件简单的日历
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<script src="https://unpkg.com/vue/dist/vue.js"></script>
<style>
*{
box-sizing: border-box;
}
ul {
list-style-type: none;
}
body {
font-family: Verdana, sans-serif;
background: #E8F0F3;
}
#calendar{
width:80%;
margin: 0 auto;
box-shadow: 0 2px 2px 0 rgba(0,0,0,0.14), 0 3px 1px -2px rgba(0,0,0,0.1), 0 1px 5px 0 rgba(0,0,0,0.12);
}
.month {
width: 100%;
background: #00B8EC;
}
.month ul {
margin: 0;
padding: 0;
display: flex;
justify-content: space-between;
}
.year-month {
display: flex;
align-items: center;
justify-content: space-around;
}
.year-month:hover {
background: rgba(150, 2, 12, 0.1);
}
.arrow {
padding: 30px;
cursor: pointer;
}
.arrow:hover {
background: rgba(100, 2, 12, 0.1);
}
.month ul li {
color: white;
font-size: 12px;
text-transform: uppercase;
letter-spacing: 3px;
}
.weekdays {
margin: 0;
padding: 10px 0;
background-color: #00B8EC;
display: flex;
flex-wrap: wrap;
color: #FFFFFF;
justify-content: space-around;
}
.weekdays li {
display: inline-block;
width: 13.6%;
text-align: center;
}
.days {
padding: 0;
background: #FFFFFF;
margin: 0;
display: flex;
flex-wrap: wrap;
justify-content: space-around;
}
.days li {
list-style-type: none;
display: inline-block;
width: 14.2%;
text-align: center;
line-height: 50px;
height: 50px;
font-size: 1rem;
color: #000;
cursor: pointer;
}
.days li.active {
border-radius: 50%;
background: #00B8EC;
color: #fff;
}
.days li:hover {
background: #e1e1e1;
}
</style>
</head>
<body>
<div id="calendar">
<div class="month">
<ul>
<li class="arrow" @click="changeDate('yearDown')">❮❮</li>
<li class="arrow" @click="changeDate('monthDown')">❮</li>
<li class="arrow">{{monthShow}}</li>
<li class="arrow" @click="changeDate('monthUp')">❯</li>
<li class="arrow" @click="changeDate('yearUp')">❯❯</li>
</ul>
</div>
<!-- 星期 -->
<ul class="weekdays">
<li style="color:red">日</li>
<li>一</li>
<li>二</li>
<li>三</li>
<li>四</li>
<li>五</li>
<li style="color:red">六</li>
</ul>
<!-- 日期 -->
<ul class="days" v-for="(item, index) in calendarList" :key="index">
<!-- 核心 v-for循环 每一次循环用<li>标签创建一天 -->
<li v-for="(v, k) in item" :key="k" :class="{active: v.formatDate === dayShow}" @click="clickHandle(v.formatDate)">
{{v.date === 0 ? '' : v.date}}
</li>
</ul>
</div>
</body>
<script>
var myVue=new Vue({
el: '#calendar',
data: {
currentDate: new Date().getDate(),
currentMonth: new Date().getMonth() + 1,
currentYear: new Date().getFullYear(),
weekDaysList: [],
calendarList: [],
monthShow: '',
},
created: function() {
this.initWeek()
this.initData();
},
methods: {
initWeek() {
this.dayShow = this.currentYear + '-' + (this.currentMonth < 10 ? '0' + this.currentMonth : this.currentMonth) + '-' + (this.currentDate < 10 ? '0' + this.currentDate : this.currentDate)
this.weekDaysList = [
{
name: '日',
value: 0,
},
{
name: '一',
value: 1,
},
{
name: '二',
value: 2,
},
{
name: '三',
value: 3,
},
{
name: '四',
value: 4,
},
{
name: '五',
value: 5,
},
{
name: '六',
value: 6,
},
]
},
initData() {
const dayTemp = new Date(this.currentYear + '-' + this.currentMonth)
const firstDay = dayTemp.getDay()
const monthDays = new Date(this.currentYear, this.currentMonth, 0).getDate()
const weeksList = []
const weeks = this.getWeeks()
this.monthShow = this.currentYear + '-' +(this.currentMonth < 10 ? '0' + this.currentMonth : this.currentMonth)
let days = 0
for (let index = 0; index < weeks; index++) {
weeksList.push([])
for (let dayIndex = 0; dayIndex < 7; dayIndex++) {
if (days === 0 && firstDay !== dayIndex) {
weeksList[index].push({
date: 0,
formatDate: 0
})
continue
}
if (days >= monthDays) {
weeksList[index].push({
date: 0,
formatDate: 0
})
continue
}
days++
weeksList[index].push({
year: this.currentYear,
month: this.currentMonth,
date: days,
formatDate: this.currentYear + '-' + (this.currentMonth < 10 ? '0' + this.currentMonth : this.currentMonth) + '-' + (days < 10 ? '0' + days : days),
})
}
}
this.calendarList = weeksList
},
getWeeks() {
const dayTemp = new Date(this.currentYear + '-' + this.currentMonth)
const firstDay = dayTemp.getDay()
const monthDays = new Date(this.currentYear, this.currentMonth, 0).getDate()
let weeks
if (firstDay === 0) {
weeks = Math.floor(monthDays / 7) + (monthDays % 7 === 0 ? 0 : 1)
} else {
const lastDays = monthDays - (7 - firstDay)
weeks = 1 + Math.floor(lastDays / 7) + (lastDays % 7 === 0 ? 0 : 1)
}
console.log(weeks)
return weeks
},
changeDate(type) {
if (type === 'yearUp') {
this.currentYear++
} else if (type === 'yearDown') {
this.currentYear--
} else if (type === 'monthUp') {
if (this.currentMonth < 12) {
this.currentMonth++
} else {
this.currentYear++
this.currentMonth = 1
}
} else if (type === 'monthDown') {
if (this.currentMonth > 1) {
this.currentMonth--
} else {
this.currentYear--
this.currentMonth = 12
}
}
this.initData()
},
clickHandle(date){
console.log(date)
}
},
});
</script>
</html>
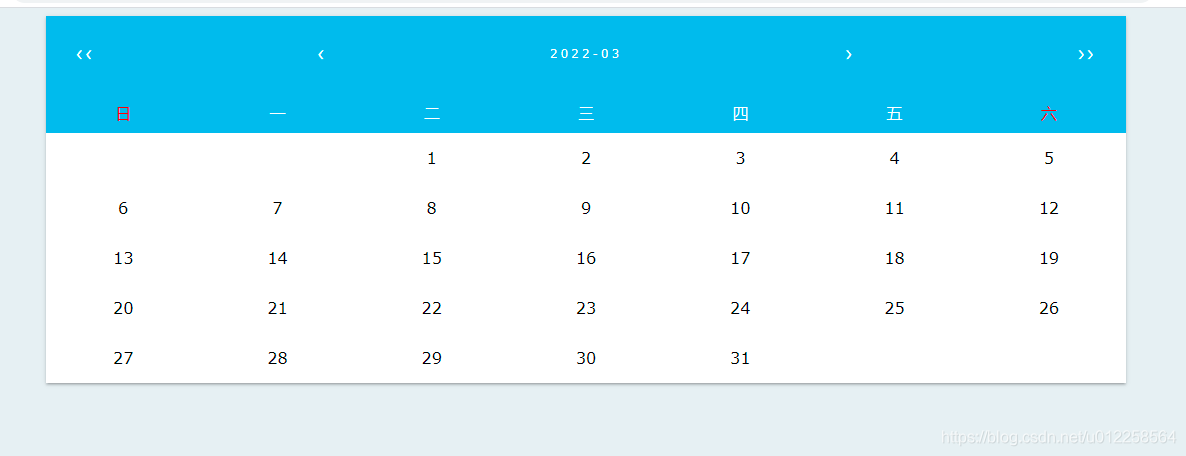