工具类:POST和GET两个类都需要调用这个工具类。
package com.example.httppost;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.params.ConnManagerParams;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.params.BasicHttpParams;
import org.apache.http.params.HttpConnectionParams;
import org.apache.http.params.HttpParams;
import org.apache.http.protocol.HTTP;
import android.util.Log;
/**
* @ClassName: HttpClientUtil
* @Description: TODO
* @author: Administrator
* @date: 2014-7-25 下午2:41:48
*/
public class HttpClientUtil {
// 服务器URL
public static final String SERVER_PATH = "http://192.168.0.149/";
private static final int REQUEST_TIMEOUT = 10 * 1000; // 连接超时
private static final int SO_TIMEOUT = 10 * 1000; // 请求超时(等待数据超时)
private static final int POOL_TIMEOUT = 2 * 1000; // 连接池中取连接的超时时间
public HttpClientUtil() {
super();
}
private static HttpClient getHttpClient() {
HttpParams httpParams = new BasicHttpParams();
// 连接超时时间
HttpConnectionParams.setConnectionTimeout(httpParams, REQUEST_TIMEOUT);
// 请求超时时间
HttpConnectionParams.setSoTimeout(httpParams, SO_TIMEOUT);
// 连接池中取连接的超时时间
ConnManagerParams.setTimeout(httpParams, POOL_TIMEOUT);
HttpClient client = new DefaultHttpClient(httpParams);
return client;
}
public static HttpResponse executeWithGet(String request,
Map<String, String> params) throws Exception {
// 拼接参数
String paramString = "";
StringBuffer buffer = new StringBuffer();
if ((params != null) && (params.size() > 0)) {
Iterator<String> it = params.keySet().iterator();
while (it.hasNext()) {
String key = it.next();
String value = params.get(key);
buffer.append(key).append("=").append(value).append("&");
}
if (buffer.length() > 0) {
paramString = "?"
+ buffer.toString().substring(0, buffer.length() - 1);
}
}
Log.i("HttpClientUtil", "[executeWithGet]URL:" + request + ", params:"
+ paramString);
// 拼接服务器URL,创建URL对象
String reqUrl = "";
if (request.startsWith("http"))
{
reqUrl = request + paramString;
} else {
reqUrl = SERVER_PATH + request + paramString;
}
HttpGet httpGet = new HttpGet(reqUrl);
HttpClient client = HttpClientUtil.getHttpClient();
HttpResponse response = client.execute(httpGet);
return response;
}
public static HttpResponse executeWithPost(String request,
Map<String, String> params) throws Exception {
// 设置HTTP POST请求参数必须用NameValuePair对象
// 拼接参数
List<NameValuePair> reqParams = new ArrayList<NameValuePair>();
if ((params != null) && (params.size() > 0)) {
Iterator<String> it = params.keySet().iterator();
while (it.hasNext()) {
String key = it.next();
String value = params.get(key);
reqParams.add(new BasicNameValuePair(key, value));
}
}
Log.i("HttpClientUtil", "[executeWithPost]URL:" + request + ", params:"
+ reqParams);
// 拼接服务器URL,创建HttpPost对象
HttpPost httpPost = new HttpPost(SERVER_PATH + request);
// 设置httpPost请求参数
httpPost.setEntity(new UrlEncodedFormEntity(reqParams, HTTP.UTF_8));
HttpClient client = HttpClientUtil.getHttpClient();
HttpResponse response = client.execute(httpPost);
return response;
}
}
HttpPost 类 开启线程,进行服务器连接,获得的结果通过handler传到Activity,msg中的内容分别是 what= 1表示网络连接成功,=0表示连接失败,obj表示从服务器返回的结果,agr1表示请求的类型,在Activity中会定义requestcode = agr1,用于区别不同的请求。
package com.example.httppost;
/**
* 传入handler和requestcode,访问服务器后,返回result,respondcode,requestcode.
* msg.obj = result
* msg.arg1 = requestcode
* msg.what表示请求是否成功
*/
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.util.Map;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.conn.ConnectTimeoutException;
import org.apache.http.util.EntityUtils;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Handler;
import android.os.Message;
import android.util.Log;
import cn.leature.istarbaby.utils.ResizeImage;
public class HttpPostUtil
{
public static final int POST_SUCCESS_CODE = 1;
public Handler mHandler;
public int mRequestCode;
public HttpPostUtil(Handler handler,int requestCode)
{
this.mHandler = handler;
this.mRequestCode = requestCode;
}
public void sendPostMessage(final String requestUrl,
final Map<String, String> param)
{
new Thread(new Runnable()
{
@Override
public void run()
{
toSendPostMessage(requestUrl, param);
}
}).start();
}
private void toSendPostMessage(String requestUrl, Map<String, String> param)
{
String responseResult = "";
int responseCode = 0;
try
{
HttpResponse httpResponse = HttpClientUtil.executeWithPost(
requestUrl, param);
if (httpResponse.getStatusLine().getStatusCode() == HttpStatus.SC_OK)
{
// 使用getEntity方法获得返回结果
responseResult = EntityUtils.toString(httpResponse.getEntity());
responseCode = POST_SUCCESS_CODE;
}
else
{
responseResult = "网络异常,请确定您当前网络。";
}
}
catch (ConnectTimeoutException e)
{
e.printStackTrace();
responseResult = "连接超时,请检查网络连接状况。";
}
catch (Exception e)
{
e.printStackTrace();
responseResult = "网络异常,请确定您当前网络。";
}
// 返回结果
Message msg = new Message();
msg.what = responseCode;
msg.arg1 = mRequestCode;
msg.obj = responseResult;
mHandler.sendMessage(msg);
}
}
|
android---HttpPost访问服务器
最新推荐文章于 2022-10-15 18:58:00 发布
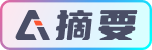