/*
*Corpyright (c)2013,烟台大学计算机学院
*All right reseved.
*作者:张梦佳
*完成日期:2014年4月21日
*版本号:v1.0
*输入描述:
*问题描述:时间累!
*程序输出:
*问题分析:
*算法设计:
*/
#include <iostream>
#include <cmath>
using namespace std;
class CTime
{
private:
unsigned short int hour; // 时
unsigned short int minute; // 分
unsigned short int second; // 秒
public:
CTime(int h=0,int m=0,int s=0)
{
hour=h;
minute=m;
second=s;
}
void timea();
void setTime(int h,int m,int s);
void display();
//二目的比较运算符重载
bool operator > (CTime &t);
bool operator < (CTime &t);
bool operator >= (CTime &t);
bool operator <= (CTime &t);
bool operator == (CTime &t);
bool operator != (CTime &t);
//二目的加减运算符的重载
//返回t规定的时、分、秒后的时间,例t1(8,20,25),t2(11,20,50),t1+t2为:41:15
CTime operator+(CTime &t);
CTime operator-(CTime &t);//对照+理解
CTime operator+(int s);//返回s秒后的时间
CTime operator-(int s);//返回s秒前的时间
//二目赋值运算符的重载
CTime operator+=(CTime &c);
CTime operator-=(CTime &c);
CTime operator+=(int s);//返回s秒后的时间
CTime operator-=(int s);//返回s秒前的时间
//一目运算符的重载
CTime operator++(int);//后置++,下一秒
CTime operator++();//前置++,下一秒,前置与后置返回值不一样
CTime operator--( int);//后置--,前一秒
CTime operator--();//前置--,前一秒
friend ostream& operator<<(ostream& output,CTime &t1);
friend istream& operator>>(istream& output,CTime &t1);
};
//下面实现所有的运算符重载代码。
ostream& operator<<(ostream& output,CTime &t1)
{
int s1,s2;
s1=t1.second/60;
t1.second=t1.second%60;
t1.minute=t1.minute+s1;
s2=t1.minute/60;
t1.minute=t1.minute%60;
t1.hour=t1.hour+s2;
t1.hour=t1.hour%24;
output<<t1.hour<<":"<<t1.minute<<":"<<t1.second<<endl;
return output;
}
istream& operator>>(istream& input,CTime &t)
{
int a,b,c;
input>>a>>b>>c;
t.setTime(a,b,c);
return input;
}
//自行编制用于测试的main()函数,有些结果不必依赖display()函数,提倡用单步执行查看结果
CTime CTime::operator++(int)
{
second=second+1;
return *this;
}
CTime CTime::operator++()
{
CTime t1;
t1.second=second;
t1.minute=minute;
t1.hour=hour;
second=second+1;
return t1;
}
CTime CTime::operator--(int)
{
second=second-1;
return *this;
}
CTime CTime::operator--()
{
CTime t1;
t1.second=second;
t1.minute=minute;
t1.hour=hour;
second=second-1;
return t1;
}
void CTime::timea()
{
int s1,s2;
s1=second/60;
second=second%60;
minute=minute+s1;
s2=minute/60;
minute=minute%60;
hour=hour+s2;
hour=hour%24;
}
//比较运算返回的是比较结果,是bool型的true或false
//可以直接使用已经重载了的运算实现新运算,例如果已经实现了 > ,则实现 <= 就可以很方便了……
void CTime::setTime(int h,int m,int s)
{
hour=h;
minute=m;
second=s;
}
bool CTime::operator >= (CTime &t) // 判断时间t1<=t2
{
int s1,s2;
CTime y;
y.second=second-t.minute+60;
y.minute=minute-t.minute+59;
s1=y.second/60;
y.second=y.second%60;
y.minute=y.minute+s1;
s2=y.minute/60;
y.minute=y.minute%60;
y.hour=hour+s2-1-t.hour;
if(t.hour>=0)
return false;
else
return true;
}
bool CTime::operator < (CTime &t) // 判断时间t1<=t2
{
if (*this >= t)
return false;
else
return true;
}
bool CTime::operator > (CTime &t) // 判断时间t1<=t2
{
if (*this <= t)
return false;
else
return true;
}
bool CTime::operator <= (CTime &t) // 判断时间t1<=t2
{
int s1,s2;
CTime y;
y.second=second-t.minute+60;
y.minute=minute-t.minute+59;
s1=y.second/60;
y.second=y.second%60;
y.minute=y.minute+s1;
s2=y.minute/60;
y.minute=y.minute%60;
y.hour=hour+s2-1-t.hour;
if(t.hour<=0)
return false;
else
return true;
}
bool CTime::operator == (CTime &t) // 判断时间t1<=t2
{
int a,b,c;
a=second-t.second;
b=minute-t.minute;
c=hour-t.hour;
if((a+b+c)==0)
return true;
else
return false;
}
bool CTime::operator != (CTime &t) // 判断时间t1<=t2
{
if (*this == t)
return false;
else
return true;
}
//可以直接使用已经重载了的加减运算实现
//这种赋值, 例如 t1+=20,直接改变当前对象的值,所以在运算完成后,将*this作为返回值
CTime CTime::operator+=(CTime &c)
{
*this=*this+c;
return *this;
}
CTime CTime::operator-=(CTime &c)
{
*this=*this-c;
return *this;
}
CTime CTime::operator+=(int s)
{
CTime c(0,0,s);
*this=*this+c;
return *this;
}
CTime CTime::operator-=(int s)
{
CTime c(0,0,s);
*this=*this-c;
return *this;
}
CTime CTime::operator+(CTime &t)
{
CTime h;
h.second=second+t.second;
h.minute=minute+t.minute;
h.hour=hour+t.hour;
h.timea();
return h;
}
CTime CTime::operator-(CTime &t)
{
CTime c;
c.second=second-t.second+60;
c.minute=minute-t.minute+59;
c.hour=hour-t.hour+23;
c.timea();
return c;
}
void CTime::display()
{
int s1,s2;
s1=second/60;
second=second%60;
minute=minute+s1;
s2=minute/60;
minute=minute%60;
hour=hour+s2;
hour=hour%24;
cout<<hour<<":"<<minute<<":"<<second<<endl;
}
CTime CTime::operator+(int s)
{
CTime h;
h.hour=hour;
h.minute=minute;
h.second=second-s;
h.timea();
return h;
}
CTime CTime::operator-(int s)
{
CTime h;
h.hour=hour;
h.minute=minute;
h.second=second-s;
h.timea();
return h;
}
int main()
{
CTime t1(2,3,5),t2,t3,t4;
int a,b,c;
cout<<"c1=";
t1.display();
cout<<"请依次输入c2 时:分:秒"<<endl;
cin>>a>>b>>c;
t2.setTime(a,b,c);
t3=t1+t2;
cout<<"c1+c2=";
t3.display();
t3=t1-t2;
cout<<"c1-c2=";
t3.display();
cout<<"(t2+=t1)=";
t2+=t1;
t2.display();
cout<<"t1>t1"<<endl;
if(t1>t1)
cout<<"good!"<<endl;
else
cout<<"bad!"<<endl;
t1.display();
cout<<" (t1+=20(秒))=";
t1+=20;
t1.display();
cout<<" (t1-=20(秒))=";
t1-=20;
t1.display();
cout<<"t2="<<t2<<endl;
cout<<"t2++"<<endl;
t2++;
cout<<"t2="<<t2<<endl;
cout<<"t2--"<<endl;
t2--;
cout<<"t2="<<t2<<endl;
cout<<"t1="<<t1<<endl;
cout<<"t2=++t1"<<endl;
t2=++t1;
cout<<"t1="<<t1<<endl;
cin>>t4;
cout<<"t4="<<t4<<endl;
if(t1==t2)
cout<<"有错误!"<<endl;
else
cout<<"没有错误调试完毕!"<<endl;
}
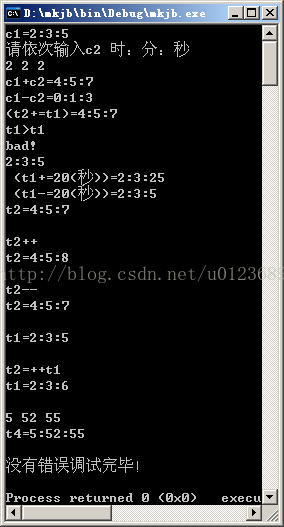
第九周项目二
最新推荐文章于 2023-04-20 10:22:56 发布