1.本题知识点
二叉树,递归
2. 题目描述
操作给定的二叉树,将其变换为源二叉树的镜像。
3. 思路
思路比较简单,首先先弄明白什么是镜像?
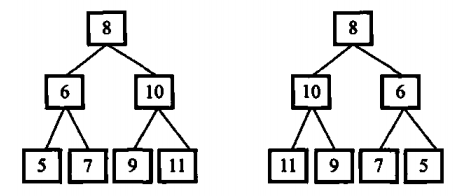
然后,每次输入根节点,交换其左右子节点即可。过程如下:
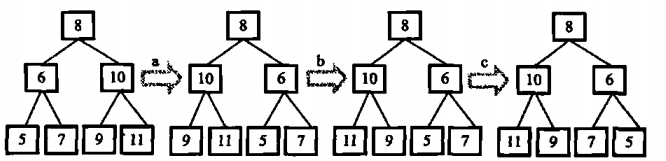
Java递归版本:
public class Solution {
public void Mirror(TreeNode root) {
if(root == null || (root.left == null && root.right == null))
return;
TreeNode temp = root.left;
root.left = root.right;
root.right = temp;
if(root.left != null)
Mirror(root.left);
if(root.right != null)
Mirror(root.right);
}
}
Java 非递归版本:
import java.util.Stack;
public class Solution {
public void Mirror(TreeNode root) {
if(root == null || (root.left == null && root.right == null))
return;
Stack<TreeNode> s = new Stack<>();
s.push(root);
while(!s.empty()){
TreeNode node = s.pop();
TreeNode temp = node.left;
node.left = node.right;
node.right = temp;
if(node.left != null)
s.push(node.left);
if(node.right != null)
s.push(node.right);
}
}
}