#include <iostream>
#include <string>
using namespace std;
//string赋值构造测试
int main()
{
string str("123456789");
char ch[] = "abcdefg";
//定义一个空字符串
string str1;
//构造函数,全部复制
string str2(str);
//从str的第二个元素开始取5个字符复制给str3
string str3(str, 2, 5);//34567
//将ch的前5个字符复制给str4
string str4(ch, 5);//abcde
//将5个A组成的字符串复制给str5
string str5(5, 'A');//AAAAA
//将str全部复制给str6
string str6(str.begin(), str.end());//123456789
system("pause");
return 0;
}
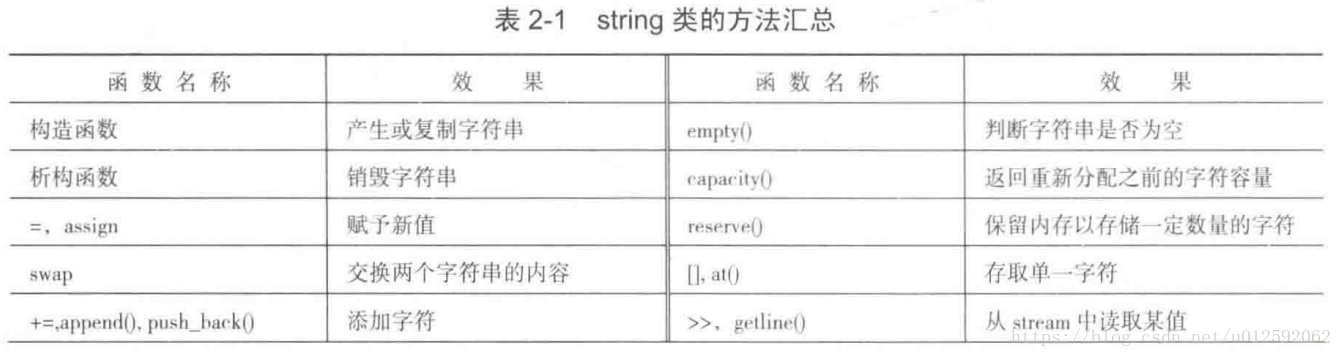
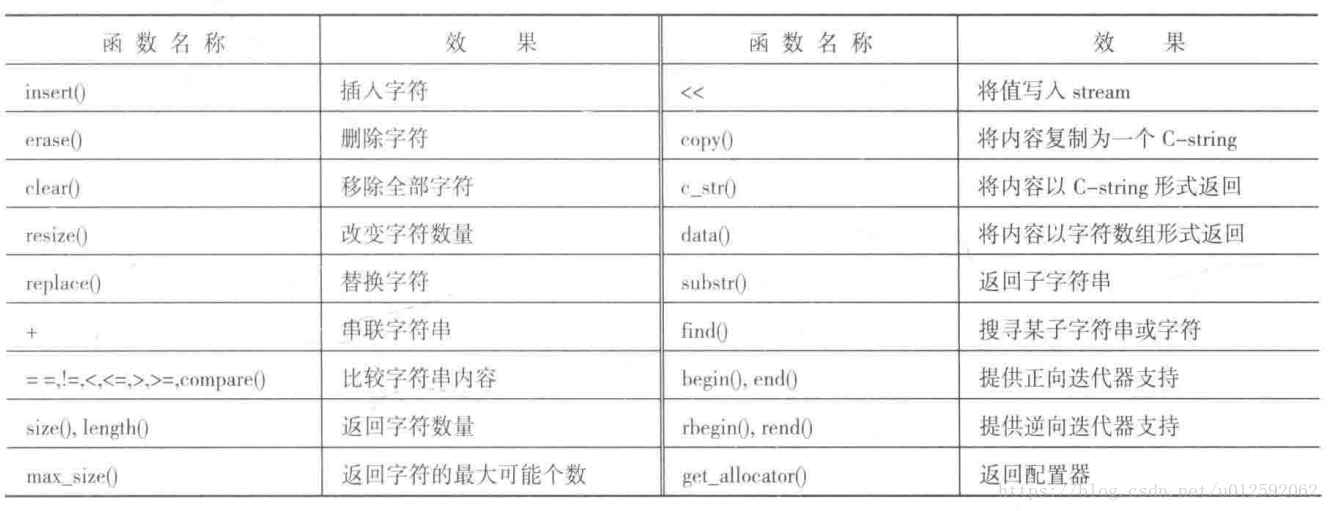
#include <iostream>
#include <string>
using namespace std;
//string大小和容量测试
int main()
{
int size = 0;
int length = 0;
unsigned long max_size = 0;
int capacity = 0;
string str("123456789");
string str1;
//"" size=0,capacity=15
str1.reserve(5);//没有重新分配内存
str1 = str;
//size=9
size = str1.size();
//调用resize()后,str1="12345"
//str1.resize(5);
//length=9
length = str1.length();
//max_size=4294967294
max_size = str1.max_size();
//capacity=15
capacity = str1.capacity();
system("pause");
return 0;
}
#include <iostream>
#include <string>
using namespace std;
//string元素的存取
int main()
{
const string cs("123456789");
string s("abcde");
char temp1=0;
char temp2=0;
char temp3=0;
char temp4=0;
char temp5=0;
char temp6=0;
//'c'
temp1 = s[2];
//'c'
temp2 = s.at(2);
//程序崩溃
temp3 = s[s.length()];
//抛出异常
temp4 = s.at(s.length());
//\0
temp5 = cs[cs.length()];
//抛出异常
temp6 = cs.at(cs.length());
system("pause");
return 0;
}
#include <iostream>
#include <string>
using namespace std;
//string元素的修改
int main()
{
string str("abcde");
//非常量字符串的at()和[]操作返回的是字符的引用
char &r1 = str[2];
char &r2 = str.at(2);
char *p = &str[3];
r1 = 'X';
*p = 'Y';
//abXYe
cout<<str<<endl;
str = "123456";
r1 = 'X';
*p = 'Y';
//12XY56
cout<<str<<endl;
system("pause");
return 0;
}
#include <iostream>
#include <string>
using namespace std;
/*
*date: 2018-07-13 13:46:32
*author: fxl
*
*/
//1,string初始化
static void test01()
{
string str("0123456789");
char ch[] = "abcdefgh";
//定义一个空字符串, a=""
string a;
//构造函数,全部复制, str1="0123456789"
string str1(str);
//从str的第2个字符开始复制5个字符给str2, str2="23456"
string str2(str, 2, 5);
//将ch的前5个元素复制给str3, str3="abcde"
string str3(ch, 5);
//将3个x复制给str4, str4="xxx"
string str4(3, 'x');
//把str的全部字符串复制给str5, str5="0123456789"
string str5(str.begin(), str.end());
}
//2,string的大小和容量
static void test02()
{
int size = 0;
int length = 0;
unsigned long maxsize = 0;
int capacity = 0;
string str = "0123456789";
string str_custom;
//当前编译器环境下不起作用
str_custom.reserve(5);
//str_custom="0123456789"
str_custom = str;
//size=10
size = str_custom.size();
//length=10
length = str_custom.length();
//重新给str_custom分配内存了, str_custom="01234"
str_custom.resize(5);
//size=5
size = str_custom.size();
//length=5
length = str_custom.length();
//maxsize=4294967294
maxsize = str_custom.max_size();
//capacity=15
capacity = str_custom.capacity();
}
//3,string元素的访问和修改
static void test03()
{
string str = "0123456789";
//返回字符的引用
char &ch1 = str[3];
char &ch2 = str.at(4);
//修改后str="012g456789"
ch1 = 'g';
//修改后str="012gw56789"
ch2 = 'w';
//越界访问后会引发程序崩溃
char ch3 = str[10];
//越界访问后会抛出异常
char ch4 = str.at(10);
}
//4,string字符串的比较
static void test04()
{
string str1 = "0123";
string str2 = "abcd";
string str3 = "Abcd";
string str4 = "abcd";
//按字符串的字典顺序来比较
//nCompare=-1, 表示str1小于str2
int nCompare = str1.compare(str2);
//nCompare=1, 表示str2大于str3
nCompare = str2.compare(str3);
//nCompare=0, 表示str2等于str4
nCompare = str2.compare(str4);
}
//5,string字符串的查找和替换
static void test05()
{
string str = "0123455678999";
//从左往右查找第一个"1"所在位置的索引, index=1
int index = str.find("1");
//从右往左查找第一个"5"所在位置的索引, index=6
index = str.rfind("5");
//从左往右查找第一个不是"0"所在位置的索引, index=1
index = str.find_first_not_of("0");
//从左往右查找第一个"3"所在位置的索引, index=1
index = str.find_first_of("3");
//从右往左查找最后一个不是"9"所在位置的索引, index=9
index = str.find_last_not_of("9");
//从左往右查找最后一个"5"所在位置的索引, index=6
index = str.find_last_of("5");
//将3位置开始的5个字符替换为aaa, str2 = "012aaa78999"
string str2 = str.replace(3,5,"aaa");
string str3 = "abcdef";
//将3位置开始的2个字符替换为5个X, str4 = "abcXXXXXf"
string str4 = str3.replace(3,2,5,'X');
}
int main()
{
test05();
//test04();
//test03();
//test02();
//test01();
system("pause");
return 0;
}