2018.5.24
1.创建web项目
2.引入jar包
在原来jar包基础上引入aop相关的jar包

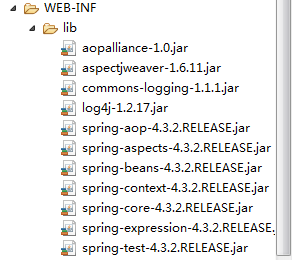
3.日志文件
log4j.properties
4.准备目标对象
先创建接口
再创建实现类
package
com.lu.spring.aop.service;
public
class
UserServiceImpl
implements
UserService
{
@Override
public
void
save
(String name) {
int
a =
9
/
0
;
System.out.println(
"保存用户"
);
}
@Override
public
void
deltete
() {
System.out.println(
"删除用户"
);
}
@Override
public
void
update
() {
System.out.println(
"更新用户"
);
}
@Override
public
void
select
() {
System.out.println(
"查询用户"
);
}
}
|
5.编写通知
- 前置通知:在目标方法之前调用
- 后置通知(如果出现异常不调用):在目标方法之后调用的
- 后置通知(无论异常都会调用):在目标方法之后调用的
- 环绕通知:在目标方法之前后调用
- 异常通知:出现异常在调用,不出现异常则不调用、
package com.lu.spring.aop.advice;
import org.aspectj.lang.ProceedingJoinPoint;
/**
* 通知
* @author bitware13
*
*/
public
class
TransactionAdvice
{
/* 前置通知:在目标方法之前调用
后置通知(如果出现异常不调用):在目标方法之后调用的
后置通知(无论异常都会调用):在目标方法之后调用的
环绕通知:在目标方法之前后调用
异常通知:出现异常在调用,不出现异常则不调用*/
public
void
before
() {
System.
out
.println(
"前置通知被执行"
);
}
public
void
after
() {
System.
out
.println(
"后置通知被执行,无论异常都会调用"
);
}
public
void
afterReturning
() {
System.
out
.println(
"后置通知被执行,如果出现异常不调用"
);
}
public
void
afterException
() {
System.
out
.println(
"异常通知被执行"
);
}
public
Object
around
(ProceedingJoinPoint point) throws Throwable {
System.
out
.println(
"环绕通知前"
);
Object proceed = point.proceed();
//调用目标方法
System.
out
.println(
"环绕通知后"
);
return
proceed;
}
}
|
6.配置织入,将通知织入到目标对象
expression="execution(* com.lu.spring.aop.service..*ServiceImpl.*(..))"
所有的返回值类型,service包下所有的子包中所有impl包下的所有有参无参的方法
加入AOP名称空间
<?
xml version=
"1.0"
encoding=
"UTF-8"
?>
<beans
xmlns
=
"http://www.springframework.org/schema/beans"
xmlns:xsi
=
"http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop
=
"http://www.springframework.org/schema/aop"
xmlns:context
=
"http://www.springframework.org/schema/context"
xsi:schemaLocation
=
"http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd"
>
<!-- 目标对象 -->
<bean
name
=
"userService"
class
=
"com.lu.spring.aop.service.UserServiceImpl"
></bean>
<!-- 通知对象 -->
<bean
name
=
"transactionAdvice"
class
=
"com.lu.spring.aop.advice.TransactionAdvice"
></bean>
<!-- 将通知对象织入目标对象 -->
<aop:config>
<!-- 选择切入点 “* ”:返回任意值 .*(..):所有的方法都织入通知 ..匹配所有有参无参的情况-->
<aop:pointcut
expression
=
"execution(* com.lu.spring.aop.service..*ServiceImpl.*(..))"
id
=
"pointcut"
/>
<aop:aspect
ref
=
"transactionAdvice"
>
<aop:before
method
=
"before"
pointcut-ref
=
"pointcut"
/>
<aop:after-returning
method
=
"afterReturning"
pointcut-ref
=
"pointcut"
/>
<aop:after
method
=
"after"
pointcut-ref
=
"pointcut"
/>
<!-- 无论是否出现异常都会调用 -->
<aop:around
method
=
"around"
pointcut-ref
=
"pointcut"
/>
<aop:after-throwing
method
=
"afterException"
pointcut-ref
=
"pointcut"
/>
</aop:aspect>
</aop:config>
</beans>
|
7.测试
package
com
.lu.spring.aop.service;
import
javax
.annotation.Resource;
import
org
.junit.Test;
import
org
.junit.runner.RunWith;
import
org
.springframework.test.context.ContextConfiguration;
import
org
.springframework.test.context.junit4.SpringJUnit4ClassRunner;
@
RunWith
(
SpringJUnit4ClassRunner
.
class
)//创建容器
@ContextConfiguration(
"classpath:applicationContext.xml"
)//从xxx.xml中找
public class AopTest {
@
Resource
(
name
="
userService
")
private UserService userService;
@
Test
public void testUpdate() {
userService
.update();
}
@
Test
public void testSave() {
userService
.save("
helen
");
}
}
|
结果:
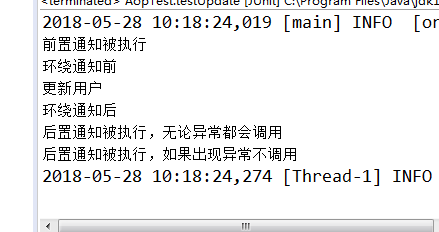
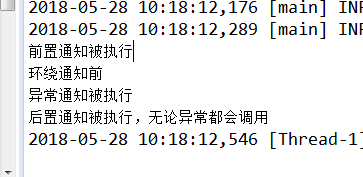