Sightseeing
Time Limit: 2000MS | Memory Limit: 65536K | |
Total Submissions: 6951 | Accepted: 2478 |
Description
Tour operator Your Personal Holiday organises guided bus trips across the Benelux. Every day the bus moves from one city S to another city F. On this way, the tourists in the bus can see the sights alongside the route travelled. Moreover, the bus makes a number of stops (zero or more) at some beautiful cities, where the tourists get out to see the local sights.
Different groups of tourists may have different preferences for the sights they want to see, and thus for the route to be taken from S to F. Therefore, Your Personal Holiday wants to offer its clients a choice from many different routes. As hotels have been booked in advance, the starting city S and the final city F, though, are fixed. Two routes from S to F are considered different if there is at least one road from a city A to a city B which is part of one route, but not of the other route.
There is a restriction on the routes that the tourists may choose from. To leave enough time for the sightseeing at the stops (and to avoid using too much fuel), the bus has to take a short route from S to F. It has to be either a route with minimal distance, or a route which is one distance unit longer than the minimal distance. Indeed, by allowing routes that are one distance unit longer, the tourists may have more choice than by restricting them to exactly the minimal routes. This enhances the impression of a personal holiday.
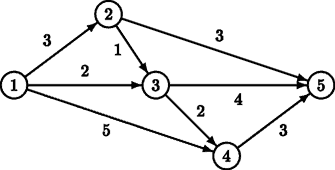
For example, for the above road map, there are two minimal routes from S = 1 to F = 5: 1 → 2 → 5 and 1 → 3 → 5, both of length 6. There is one route that is one distance unit longer: 1 → 3 → 4 → 5, of length 7.
Now, given a (partial) road map of the Benelux and two cities S and F, tour operator Your Personal Holiday likes to know how many different routes it can offer to its clients, under the above restriction on the route length.
Input
The first line of the input file contains a single number: the number of test cases to follow. Each test case has the following format:
-
One line with two integers N and M, separated by a single space, with 2 ≤ N ≤ 1,000 and 1 ≤ M ≤ 10, 000: the number of cities and the number of roads in the road map.
-
M lines, each with three integers A, B and L, separated by single spaces, with 1 ≤ A, B ≤ N, A ≠ B and 1 ≤ L ≤ 1,000, describing a road from city A to city B with length L.
The roads are unidirectional. Hence, if there is a road from A to B, then there is not necessarily also a road from B to A. There may be different roads from a city A to a city B.
-
One line with two integers S and F, separated by a single space, with 1 ≤ S, F ≤ N and S ≠ F: the starting city and the final city of the route.
There will be at least one route from S to F.
Output
For every test case in the input file, the output should contain a single number, on a single line: the number of routes of minimal length or one distance unit longer. Test cases are such, that this number is at most 109 = 1,000,000,000.
Sample Input
2
5 8
1 2 3
1 3 2
1 4 5
2 3 1
2 5 3
3 4 2
3 5 4
4 5 3
1 5
5 6
2 3 1
3 2 1
3 1 10
4 5 2
5 2 7
5 2 7
4 1
Sample Output
3
2
Hint
The first test case above corresponds to the picture in the problem description.
Source
题型:求最短路以及比最短路长度大一的次短路,并要求计数。
传送门:【POJ】3463 Sightseeing
题目大意:给你n个点m条边的有向图(unidirectional是有向!),让你求最短路以及长度比最短路大一的路的数量。题目保证数量不超过10^9。(2 ≤ N ≤ 1,000 and 1 ≤ M ≤ 10,000)
题目分析:
如果直接在Dijkstra算法中求最短路的数量以及次短路的数量的话,那么算法中就有四种情况存在:
1.比最短路短
2.和最短路一样短
3.比次短路短
4.和次短路一样长
都要考虑到,且要注意细节。
每次可以在最短路上增广或者次短路上增广,最短路上增广包括所有四种情况,次短路增广包括后两种情况(次短路得到的路肯定不可能比最短路短),那么判断是否标号永久化就需要对同一个结点永久化两次,一次是最短路,一次是次短路。
代码如下:
#include <stdio.h>
#include <string.h>
#include <algorithm>
using namespace std ;
#define REPF( i , a , b ) for ( int i = a ; i <= b ; ++ i )
#define REPV( i , a , b ) for ( int i = a ; i >= b ; -- i )
#define REP( i , n ) for ( int i = 0 ; i < n ; ++ i )
#define clear( A , X ) memset ( A , X , sizeof A )
typedef int type_d ;
const int maxN = 1005 ;
const int maxQ = 100005 ;
const int maxH = 100005 ;
const int maxE = 100005 ;
const int oo = 0x3f3f3f3f ;
struct Heap {
int num ;
int kind ;
type_d d ;
Heap () {}
Heap ( type_d D , int Num , int Kind ) :
d(D) , num(Num) , kind(Kind) {}
} ;
struct Edge {
int v , n , w ;
Edge () {}
Edge ( int V , int W , int N ) :
v(V) , w(W) , n(N) {}
} ;
struct Priority_Queue {
Heap heap[maxH] ;
int top ;
void swap ( Heap &a , Heap &b ) {
Heap tmp ;
tmp = a ;
a = b ;
b = tmp ;
}
int cmp ( const Heap a , const Heap b ) {
return a.d < b.d ;
}
void Clear () {
top = 1 ;//下标从1开始
}
int Empty () {
return 1 == top ;
}
void Push ( type_d d , int num , int kind ) {
heap[top] = Heap ( d , num , kind ) ;
int o = top ++ ;
while ( o > 1 && cmp ( heap[o] , heap[o >> 1] ) ) {
swap ( heap[o] , heap[o >> 1] ) ;
o >>= 1 ;
}
}
int Front () {
return heap[1].num ;
}
int Kind () {
return heap[1].kind ;
}
void Pop () {
heap[1] = heap[-- top] ;
int o = 1 , p = o , l = o << 1 , r = o << 1 | 1 ;
while ( 1 ) {
if ( l < top && cmp ( heap[l] , heap[p] ) )
p = l ;
if ( r < top && cmp ( heap[r] , heap[p] ) )
p = r ;
if ( p == o )
break ;
swap ( heap[o] , heap[p] ) ;
o = p , l = o << 1 , r = o << 1 | 1 ;
}
}
} ;
struct Dij {
Priority_Queue Q ;
Edge edge[maxE] ;
int adj[maxN] , cntE ;
int done[2][maxN] ;
type_d d[2][maxN] ;
//int p[maxN] ;
int cnt[2][maxN] ;
void addedge ( int u , int v , int w = 0 ) {
edge[cntE] = Edge ( v , w , adj[u] ) ;
adj[u] = cntE ++ ;
//edge[cntE] = Edge ( u , w , adj[v] ) ;
//adj[v] = cntE ++ ;
}
void Init () {
cntE = 0 ;
Q.Clear () ;
clear ( d , oo ) ;
clear ( cnt , 0 ) ;
clear ( adj , -1 ) ;
//clear ( p , -1 ) ;
}
void Dijkstra ( int s ) {
clear ( done , 0 ) ;
d[0][s] = 0 ;
cnt[0][s] = 1 ;
Q.Push ( d[0][s] , s , 0 ) ;
while ( !Q.Empty () ) {
int u = Q.Front () ;
int kind = Q.Kind () ;
Q.Pop () ;
if ( done[kind][u] )
continue ;
done[kind][u] = 1 ;
for ( int i = adj[u] ; ~i ; i = edge[i].n ) {
int v = edge[i].v ;
int val = d[kind][u] + edge[i].w ;
if ( d[0][v] > val ) {
d[1][v] = d[0][v] ;
cnt[1][v] = cnt[0][v] ;
Q.Push ( d[1][v] , v , 1 ) ;
d[0][v] = val ;
cnt[0][v] = cnt[kind][u] ;
Q.Push ( d[0][v] , v , 0 ) ;
}
else if ( d[0][v] == val )
cnt[0][v] += cnt[kind][u] ;
else if ( d[1][v] > val ) {
d[1][v] = val ;
cnt[1][v] = cnt[kind][u] ;
Q.Push ( d[1][v] , v , 1 ) ;
}
else if ( d[1][v] == val )
cnt[1][v] += cnt[kind][u] ;
}
}
}
} ;
Dij D ;
void work () {
int n , m ;
int s , t ;
int u , v , w ;
D.Init () ;
scanf ( "%d%d" , &n , &m ) ;
REP ( i , m ) {
scanf ( "%d%d%d" , &u , &v , &w ) ;
D.addedge ( u , v , w ) ;
}
scanf ( "%d%d" , &s , &t ) ;
D.Dijkstra ( s ) ;
int ans = D.cnt[0][t] ;
//printf ( "%d %d\n" , D.d[0][t] , D.d[1][t] ) ;
if ( D.d[1][t] == D.d[0][t] + 1 )
ans += D.cnt[1][t] ;
printf ( "%d\n" , ans ) ;
}
int main () {
int T ;
scanf ( "%d" , &T ) ;
while ( T -- )
work () ;
return 0 ;
}
然后是另一种方法:
Dijkstra依旧求出最短路的数量,然后用DP在反图上倒着记忆化搜索,如果d[u] == d[v] + w[v,u],说明到目前为止还在最短路上,那么可以在继续递归中得到次短路的数量cnt1,则属于该结点的次短路数量cnt += cnt1。如果d[u] == d[v] + w[v,u] - 1,说明正在次短路的一条边上,后面一定都是最短路的边了,则下面直接得到最短路数量cnt2,则次短路数量cnt += cnt2。感觉比上面的方法还要简单~
代码如下:
#include <stdio.h>
#include <string.h>
#include <algorithm>
using namespace std ;
#define REPF( i , a , b ) for ( int i = a ; i <= b ; ++ i )
#define REPV( i , a , b ) for ( int i = a ; i >= b ; -- i )
#define REP( i , n ) for ( int i = 0 ; i < n ; ++ i )
#define clear( A , X ) memset ( A , X , sizeof A )
typedef int type_d ;
const int maxN = 1005 ;
const int maxQ = 100005 ;
const int maxH = 100005 ;
const int maxE = 100005 ;
const int oo = 0x3f3f3f3f ;
struct Heap {
int num ;
type_d d ;
Heap () {}
Heap ( type_d D , int Num ) :
d(D) , num(Num) {}
} ;
struct Edge {
int v , n , w ;
Edge () {}
Edge ( int V , int W , int N ) :
v(V) , w(W) , n(N) {}
} ;
struct Priority_Queue {
Heap heap[maxH] ;
int top ;
void swap ( Heap &a , Heap &b ) {
Heap tmp ;
tmp = a ;
a = b ;
b = tmp ;
}
int cmp ( const Heap a , const Heap b ) {
return a.d < b.d ;
}
void Clear () {
top = 1 ;//下标从1开始
}
int Empty () {
return 1 == top ;
}
void Push ( type_d d , int num ) {
heap[top] = Heap ( d , num ) ;
int o = top ++ ;
while ( o > 1 && cmp ( heap[o] , heap[o >> 1] ) ) {
swap ( heap[o] , heap[o >> 1] ) ;
o >>= 1 ;
}
}
int Front () {
return heap[1].num ;
}
void Pop () {
heap[1] = heap[-- top] ;
int o = 1 , p = o , l = o << 1 , r = o << 1 | 1 ;
while ( 1 ) {
if ( l < top && cmp ( heap[l] , heap[p] ) )
p = l ;
if ( r < top && cmp ( heap[r] , heap[p] ) )
p = r ;
if ( p == o )
break ;
swap ( heap[o] , heap[p] ) ;
o = p , l = o << 1 , r = o << 1 | 1 ;
}
}
} ;
struct Dij {
Priority_Queue Q ;
Edge edge[maxE] , E[maxE] ;
int adj[maxN] , Adj[maxN] , cntE , cntEE ;
int done[maxN] ;
type_d d[maxN] ;
//int p[maxN] ;
int cnt[maxN] ;//最短路数量
int cnt2[maxN] ;//次短路数量
void addedge ( int u , int v , int w = 0 ) {
edge[cntE] = Edge ( v , w , adj[u] ) ;
adj[u] = cntE ++ ;
E[cntEE] = Edge ( u , w , Adj[v] ) ;
Adj[v] = cntEE ++ ;
}
void Init () {
cntE = 0 ;
cntEE = 0 ;
Q.Clear () ;
clear ( d , oo ) ;
clear ( cnt , 0 ) ;
clear ( cnt2 , -1 ) ;
clear ( adj , -1 ) ;
clear ( Adj , -1 ) ;
//clear ( p , -1 ) ;
}
void Dijkstra ( int s ) {
clear ( done , 0 ) ;
d[s] = 0 ;
cnt[s] = 1 ;
Q.Push ( d[s] , s ) ;
while ( !Q.Empty () ) {
int u = Q.Front () ;
Q.Pop () ;
if ( done[u] )
continue ;
done[u] = 1 ;
for ( int i = adj[u] ; ~i ; i = edge[i].n ) {
int v = edge[i].v ;
int val = d[u] + edge[i].w ;
if ( d[v] > val ) {
d[v] = val ;
cnt[v] = cnt[u] ;
Q.Push ( d[v] , v ) ;
}
else if ( d[v] == val )
cnt[v] += cnt[u] ;
}
}
}
int dp ( int u ) {
if ( cnt2[u] != -1 )
return cnt2[u] ;
cnt2[u] = 0 ;
for ( int i = Adj[u] ; ~i ; i = E[i].n ) {
int v = E[i].v ;
if ( d[u] == d[v] + E[i].w )
cnt2[u] += dp ( v ) ;
else if ( d[u] == d[v] + E[i].w - 1 )
cnt2[u] += cnt[v] ;
}
return cnt2[u] ;
}
} ;
Dij D ;
void work () {
int n , m ;
int s , t ;
int u , v , w ;
D.Init () ;
scanf ( "%d%d" , &n , &m ) ;
REP ( i , m ) {
scanf ( "%d%d%d" , &u , &v , &w ) ;
D.addedge ( u , v , w ) ;
}
scanf ( "%d%d" , &s , &t ) ;
D.Dijkstra ( s ) ;
//printf ( "%d\n" , D.cnt[t] ) ;
printf ( "%d\n" , D.cnt[t] + D.dp ( t ) ) ;
}
int main () {
int T ;
scanf ( "%d" , &T ) ;
while ( T -- )
work () ;
return 0 ;
}