一、新建工程
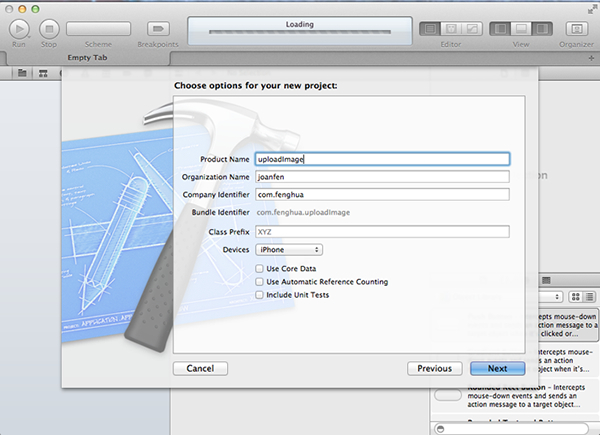
二、拖控件,创建映射
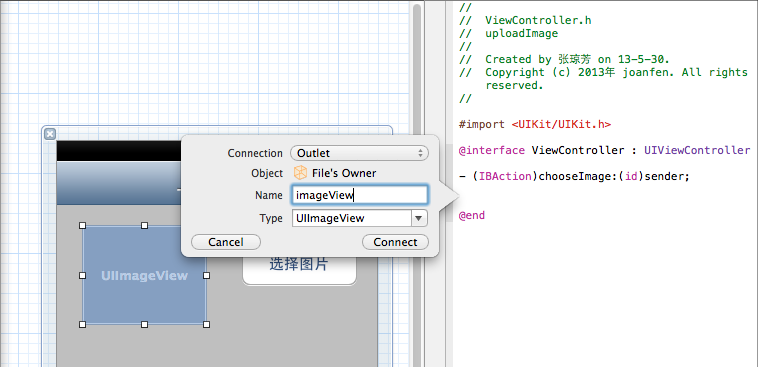
三、在.h中加入delegate
1 | @interface ViewController : UIViewController<UIActionSheetDelegate,UIImagePickerControllerDelegate,UINavigationControllerDelegate> |
四、实现按钮事件
01 | -(IBAction)chooseImage:(id)sender { |
07 | if ([UIImagePickerController isSourceTypeAvailable:UIImagePickerControllerSourceTypeCamera]) |
10 | sheet = [[UIActionSheet alloc] initWithTitle:@ "选择" delegate:self cancelButtonTitle:nil destructiveButtonTitle:@ "取消" otherButtonTitles:@ "拍照" ,@ "从相册选择" , nil]; |
16 | sheet = [[UIActionSheet alloc] initWithTitle:@ "选择" delegate:self cancelButtonTitle:nil destructiveButtonTitle:@ "取消" otherButtonTitles:@ "从相册选择" , nil]; |
22 | [sheet showInView:self.view]; |
五、实现actionSheet delegate事件
判断是否支持相机,跳转到相机或相册界面
01 | -( void ) actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex |
03 | if (actionSheet.tag == 255) { |
05 | NSUInteger sourceType = 0; |
08 | if ([UIImagePickerController isSourceTypeAvailable:UIImagePickerControllerSourceTypeCamera]) { |
10 | switch (buttonIndex) { |
16 | sourceType = UIImagePickerControllerSourceTypeCamera; |
21 | sourceType = UIImagePickerControllerSourceTypePhotoLibrary; |
26 | if (buttonIndex == 0) { |
30 | sourceType = UIImagePickerControllerSourceTypeSavedPhotosAlbum; |
36 | UIImagePickerController *imagePickerController = [[UIImagePickerController alloc] init]; |
38 | imagePickerController.delegate = self; |
40 | imagePickerController.allowsEditing = YES; |
42 | imagePickerController.sourceType = sourceType; |
44 | [self presentViewController:imagePickerController animated:YES completion:^{}]; |
46 | [imagePickerController release]; |
六、实现ImagePicker delegate 事件
01 | #pragma mark - image picker delegte |
02 | - ( void )imagePickerController:(UIImagePickerController *)picker didFinishPickingMediaWithInfo:(NSDictionary *)info |
04 | [picker dismissViewControllerAnimated:YES completion:^{}]; |
06 | UIImage *image = [info objectForKey:UIImagePickerControllerOriginalImage]; |
17 | [self saveImage:image withName:@ "currentImage.png" ]; |
19 | NSString *fullPath = [[NSHomeDirectory() stringByAppendingPathComponent:@ "Documents" ] stringByAppendingPathComponent:@ "currentImage.png" ]; |
21 | UIImage *savedImage = [[UIImage alloc] initWithContentsOfFile:fullPath]; |
24 | [self.imageView setImage:savedImage]; |
26 | self.imageView.tag = 100; |
29 | - ( void )imagePickerControllerDidCancel:(UIImagePickerController *)picker |
31 | [self dismissViewControllerAnimated:YES completion:^{}]; |
七、保存图片
高保真压缩图片方法
NSData * UIImageJPEGRepresentation ( UIImage *image, CGFloat compressionQuality
)
此方法可将图片压缩,但是图片质量基本不变,第二个参数即图片质量参数。
01 | #pragma mark - 保存图片至沙盒 |
02 | - ( void ) saveImage:(UIImage *)currentImage withName:(NSString *)imageName |
05 | NSData *imageData = UIImageJPEGRepresentation(currentImage, 0.5); |
08 | NSString *fullPath = [[NSHomeDirectory() stringByAppendingPathComponent:@ "Documents" ] stringByAppendingPathComponent:imageName]; |
11 | [imageData writeToFile:fullPath atomically:NO]; |
八、实现点击图片预览功能,滑动放大缩小,带动画
01 | -( void )touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event |
04 | isFullScreen = !isFullScreen; |
05 | UITouch *touch = [touches anyObject]; |
07 | CGPoint touchPoint = [touch locationInView:self.view]; |
09 | CGPoint imagePoint = self.imageView.frame.origin; |
13 | if (imagePoint.x <= touchPoint.x && imagePoint.x +self.imageView.frame.size.width >=touchPoint.x && imagePoint.y <= touchPoint.y && imagePoint.y+self.imageView.frame.size.height >= touchPoint.y) |
16 | [UIView beginAnimations:nil context:nil]; |
18 | [UIView setAnimationDuration:1]; |
23 | self.imageView.frame = CGRectMake(0, 0, 320, 480); |
27 | self.imageView.frame = CGRectMake(50, 65, 90, 115); |
31 | [UIView commitAnimations]; |
九、上传图片,使用ASIhttpRequest类库实现,由于本文重点不是网络请求,故不对ASIHttpRequest详细讲述,只贴出部分代码
01 | ASIFormDataRequest *requestReport = [[ASIFormDataRequest alloc] initWithURL:服务器地址]; |
03 | NSString *Path = [[NSHomeDirectory() stringByAppendingPathComponent:@ "Documents" ] stringByAppendingPathComponent:@ "currentImage.png" ]; |
05 | [requestReport setFile:Path forKey:@ "picturepath" ]; |
07 | [requestReport buildPostBody]; |
09 | requestReport.delegate = self; |
11 | [requestReport startAsynchronous]; |
效果图如下:
->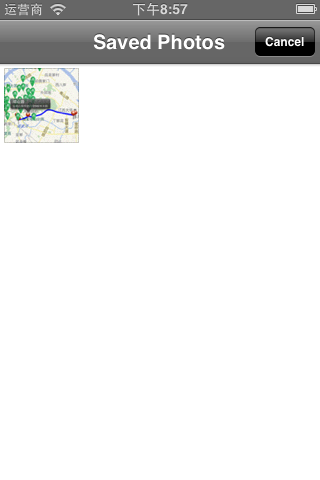
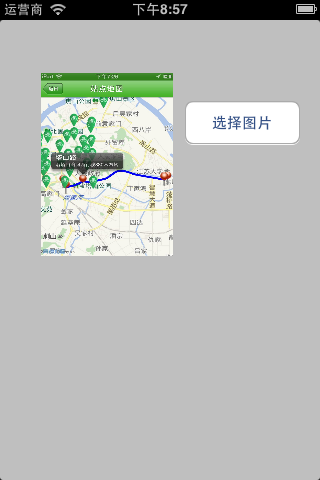
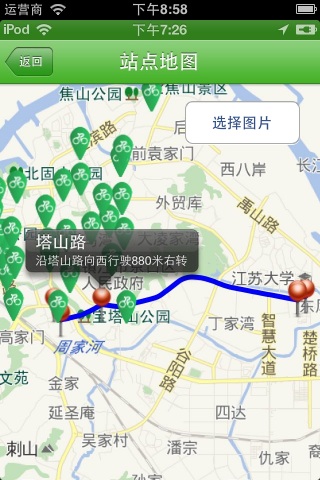
ps:模拟器添加图片
1.模拟器无法调用相机;
2.模拟器添加图片方法:将图片拖至模拟器主屏,会由模拟器safari打开,长按可保存至模拟器相册,即可进行模拟器调试了。
3.关于调用相机,是系统自带的,不知道如何修改英文标题为中文,如cancel换为取消等,望知道的大虾们不吝告知,万分感谢