dingo/api - 4.响应
1. 默认格式
JSON
说明:
- 返回的对象需实现 ArrayObject 或 Illuminate\Support\Contracts\ArrayableInterface 接口
class UserController
{
public function index()
{
return User::all();
}
}
2. 响应头
The package will automatically format the response as JSON and set the Content-Type
header to application/json. --- dingo/api官方
3. 使用 Response Builder
条件:
- use Dingo\Api\Routing\Helpers trait
一般用在BaseController里,其他controller继承即可。- 返回几种形式: array / item / collection / pagination / no content / created response
// 返回Array
$user = User::findOrFail($id);
return $this->response->array($user->toArray());
// 返回 Single Item
$user = User::findOrFail($id);
return $this->response->item($user, new UserTransformer);
// 返回 A Collection Of Items
$users = User::all();
return $this->response->collection($users, new UserTransformer);
// 返回 Paginated Items
// 说明:分页结构在 body 里的meta元素里
$users = User::paginate(25);
return $this->response->paginator($users, new UserTransformer);
// 返回 No Content
return $this->response->noContent();
// 返回 Created Response
// 说明:可以用在返回token的时候,内容塞在 header里
return $this->response->created();
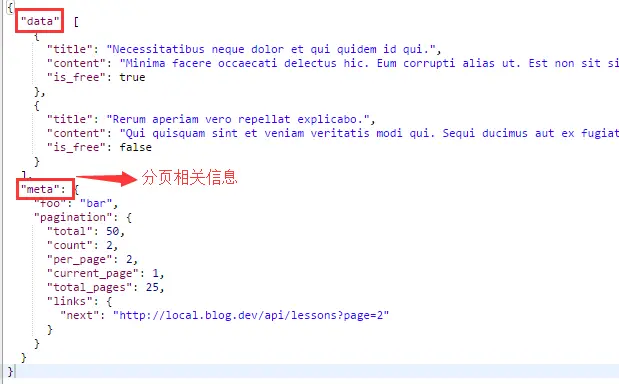
分页相关结果
4. 响应错误
分为两种:
- 自定义
- dingo/api 内置
- 详细参考 Dingo\Api\Http\Response\Factory
// 自定义
// A generic error with custom message and status code.
return $this->response->error('This is an error.', 404);
// A not found error with an optional message as the first parameter.
return $this->response->errorNotFound();
// A bad request error with an optional message as the first parameter.
return $this->response->errorBadRequest();
// A forbidden error with an optional message as the first parameter.
return $this->response->errorForbidden();
// An internal error with an optional message as the first parameter.
return $this->response->errorInternal();
// An unauthorized error with an optional message as the first parameter.
return $this->response->errorUnauthorized();
5. 响应头header
withHeader();
return $this->response->item($user, new UserTransformer)->withHeader('X-Foo', 'Bar');
6. 响应meta
addMeta();
return $this->response->item($user, new UserTransformer)->addMeta('foo', 'bar');
7. 设置响应状态码
setStatusCode()
return $this->response->item($user, new UserTransformer)->setStatusCode(200);
8. 自定义响应格式
说明:
- 默认为json,同时会设置 Content-Type
- 两种方式修改jsonp
- 配置文件 api.php
'formats' => [ 'json' => 'Dingo\Api\Http\Response\Format\Jsonp']
- 在 bootstrap file里:
Dingo\Api\Http\Response::addFormatter('json', new Dingo\Api\Http\Response\Format\Jsonp);
- 其他方式修改,参考文档:https://github.com/dingo/api/wiki/Responses#custom-response-formats
9. 事件监听
说明:
- 事件有:ResponseWasMorphed 和 ResponseIsMorphing
- 目的:改变特定元素的值,比如分页的上一页下一页链接美化
// 1. route部分
$api->get('lessons', 'LessonController@index');
// 2. controller部分
$lessons = Lesson::paginate(2);
return $this->response->paginator($lessons, new LessonTransformer());
// 3. event 部分
<?php
namespace App\Listeners;
use Dingo\Api\Event\ResponseWasMorphed;
class AddPaginationLinksToResponse
{
public function handle(ResponseWasMorphed $event)
{
if (isset($event->content['meta']['pagination'])) {
$links = $event->content['meta']['pagination']['links'];
$event->response->headers->set(
'link',
sprintf('<%s>; rel="next"', $links['next'])
);
}
}
}
// 4. 事件绑定(event bind)部分 --- app/Providers/EventServiceProvider.php 的 $listen数组
'Dingo\Api\Event\ResponseWasMorphed' => [
'App\Listeners\AddPaginationLinksToResponse'
]