1. ReentrantLock是什么
Lock提供了比synchronized方法和语句更广泛的锁定操作。 更灵活的结构化,并且支持多个相关联的对象Condition。它实现了Lock、Serializable序列化接口。
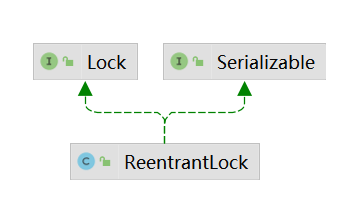
图1 ReentrantLock实现接口图
1.1 Lock
1.1.1 lock
// 获取锁
void lock();
1.1.2 lockInterruptibly
// 跟lock一样的功能--获取锁,但是lock中途不能被终端,lockInterruptibly允许中途被中断
void lockInterruptibly();
1.1.3 tryLock
// 尝试获取锁,成功true,失败false
boolean tryLock();
1.1.4 unlock
// 解锁,在finally中使用,不然容易死锁
void unlock();
1.1.5 newCondition
// 获取Condition对象(await、signal)
Condition newCondition();
1.2 ReentrantLock源码剖析
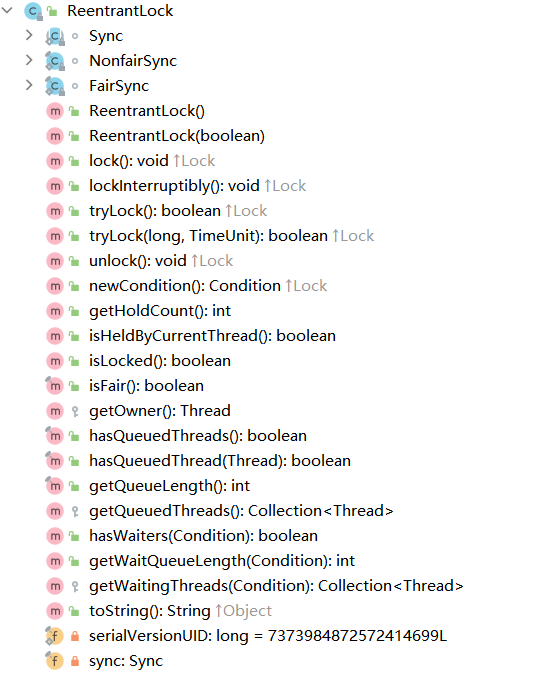
图2 ReentrantLock总方法
1.2.1 非公平锁
// 无参构造方法
public ReentrantLock() {
// 创建非公平锁
sync = new NonfairSync();
}
1.2.1.1 NonfairSync
// 非公平锁继承Sync
static final class NonfairSync extends Sync {
// 序列化ID
private static final long serialVersionUID = 7316153563782823691L;
// 获取锁
final void lock() {
// 通过Unsafe接口的CAS函数设置值,将对象的设置为使用状态
if (compareAndSetState(0, 1))
// 使用对象为当前操作线程
setExclusiveOwnerThread(Thread.currentThread());
else
// 获取不到,则加入等待队列
acquire(1);
}
// 尝试获取锁
protected final boolean tryAcquire(int acquires) {
return nonfairTryAcquire(acquires);
}
}
1.2.1.2 acquire
// 加入等待队列
public final void acquire(int arg) {
if (!tryAcquire(arg) &&
acquireQueued(addWaiter(Node.EXCLUSIVE), arg))
selfInterrupt();
}
1.2.1.3 tryAcquire
// 尝试是否为可重入锁
protected final boolean tryAcquire(int acquires) {
return nonfairTryAcquire(acquires);
}
//
final boolean nonfairTryAcquire(int acquires) {
// 获取当前线程
final Thread current = Thread.currentThread();
// 查看对象锁状态
int c = getState();
// 空闲状态
if (c == 0) {
// CAS设置占有状态
if (compareAndSetState(0, acquires)) {
// 设置当前线程持有
setExclusiveOwnerThread(current);
return true;
}
}
// 非空闲,但占有对象的线程为当前线程,则按可重入锁解决
else if (current == getExclusiveOwnerThread()) {
// 统计可重入锁次数
int nextc = c + acquires;
// 非法次数
if (nextc < 0) // overflow
throw new Error("Maximum lock count exceeded");
// 设置对象可重入锁次数
setState(nextc);
return true;
}
return false;
}
1.2.1.4 Sync
// 核心!抽象队列同步器(AQS)
abstract static class Sync extends AbstractQueuedSynchronizer {
// 序列ID
private static final long serialVersionUID = -5179523762034025860L;
// 获取锁
abstract void lock();
// 非公平锁实现方法,跟上面一样,不重复解释
final boolean nonfairTryAcquire(int acquires) {
final Thread current = Thread.currentThread();
int c = getState();
if (c == 0) {
if (compareAndSetState(0, acquires)) {
setExclusiveOwnerThread(current);
return true;
}
}
else if (current == getExclusiveOwnerThread()) {
int nextc = c + acquires;
if (nextc < 0) // overflow
throw new Error("Maximum lock count exceeded");
setState(nextc);
return true;
}
return false;
}
// 尝试释放锁
protected final boolean tryRelease(int releases) {
// 统计对象可重入锁次数
int c = getState() - releases;
// 当前线程不是对象持有锁对象,非法操作
if (Thread.currentThread() != getExclusiveOwnerThread())
throw new IllegalMonitorStateException();
// 对象操作中,有点"内存屏障"的意思
boolean free = false;
// 如果状态为0,不用操作了,没有线程占有该对象
if (c == 0) {
// 对象可操作状态
free = true;
// 对象无线程占有
setExclusiveOwnerThread(null);
}
// 设置对象可重入锁次数
setState(c);
return free;
}
// 判断当前对象占有线程是否为此时操作线程,可重入锁的必要检查
protected final boolean isHeldExclusively() {
return getExclusiveOwnerThread() == Thread.currentThread();
}
// 获取Condition对象,可对对象进行阻塞(await)和就绪(signal)状态操作
final ConditionObject newCondition() {
return new ConditionObject();
}
// 获取对象占用线程
final Thread getOwner() {
return getState() == 0 ? null : getExclusiveOwnerThread();
}
// 获取对象锁可重入次数
final int getHoldCount() {
return isHeldExclusively() ? getState() : 0;
}
// 对象是否被占用
final boolean isLocked() {
return getState() != 0;
}
}
1.2.2 公平锁
// 传入true,则为创建公平锁
public ReentrantLock(boolean fair) {
sync = fair ? new FairSync() : new NonfairSync();
}
1.2.2.1 FairSync
// 公平锁
static final class FairSync extends Sync {
// 序列ID
private static final long serialVersionUID = -3000897897090466540L;
// 获取锁
final void lock() {
acquire(1);
}
// 获取可重入锁
protected final boolean tryAcquire(int acquires) {
// 当前线程
final Thread current = Thread.currentThread();
// 统计对象可重入次数
int c = getState();
// 对象无锁状态
if (c == 0) {
// 队列为空(这里与非公平不一样,队列没有等待线程他才可以持有)
if (!hasQueuedPredecessors() &&
// 设置对象占用状态
compareAndSetState(0, acquires)) {
// 设置值对象占用线程
setExclusiveOwnerThread(current);
return true;
}
}
// 与非公平锁解释相同
else if (current == getExclusiveOwnerThread()) {
int nextc = c + acquires;
if (nextc < 0)
throw new Error("Maximum lock count exceeded");
setState(nextc);
return true;
}
return false;
}
}
1.2.2.2 acquire
// 获取锁
public final void acquire(int arg) {
// 获取失败
if (!tryAcquire(arg) &&
// 加入队列成功
acquireQueued(addWaiter(Node.EXCLUSIVE), arg))
// 设置线程中断状态
selfInterrupt();
}
1.3 AbstractQueuedSynchronizer
AQS详见该文章:《AbstractQueuedSynchronizer从入门到踹门》
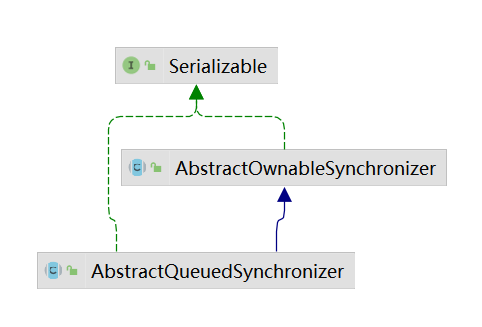
图3 AQS祖宗图
关键其实就是等待队列,也叫"CLH"(Craig, Landin, and Hagersten)锁队列。
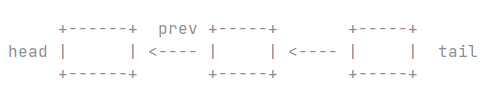
图4 CLH结构图(双端队列)
1.3.1 Node节点
static final class Node {
// 共享节点
static final Node SHARED = new Node();
// 排他节点
static final Node EXCLUSIVE = null;
// 取消状态:其实就是废物了,等待被GC
static final int CANCELLED = 1;
//
static final int SIGNAL = -1;
/** waitStatus value to indicate thread is waiting on condition */
static final int CONDITION = -2;
/**
* waitStatus value to indicate the next acquireShared should
* unconditionally propagate
*/
static final int PROPAGATE = -3;
/**
* Status field, taking on only the values:
* SIGNAL: The successor of this node is (or will soon be)
* blocked (via park), so the current node must
* unpark its successor when it releases or
* cancels. To avoid races, acquire methods must
* first indicate they need a signal,
* then retry the atomic acquire, and then,
* on failure, block.
* CANCELLED: This node is cancelled due to timeout or interrupt.
* Nodes never leave this state. In particular,
* a thread with cancelled node never again blocks.
* CONDITION: This node is currently on a condition queue.
* It will not be used as a sync queue node
* until transferred, at which time the status
* will be set to 0. (Use of this value here has
* nothing to do with the other uses of the
* field, but simplifies mechanics.)
* PROPAGATE: A releaseShared should be propagated to other
* nodes. This is set (for head node only) in
* doReleaseShared to ensure propagation
* continues, even if other operations have
* since intervened.
* 0: None of the above
*
* The values are arranged numerically to simplify use.
* Non-negative values mean that a node doesn't need to
* signal. So, most code doesn't need to check for particular
* values, just for sign.
*
* The field is initialized to 0 for normal sync nodes, and
* CONDITION for condition nodes. It is modified using CAS
* (or when possible, unconditional volatile writes).
*/
volatile int waitStatus;
/**
* Link to predecessor node that current node/thread relies on
* for checking waitStatus. Assigned during enqueuing, and nulled
* out (for sake of GC) only upon dequeuing. Also, upon
* cancellation of a predecessor, we short-circuit while
* finding a non-cancelled one, which will always exist
* because the head node is never cancelled: A node becomes
* head only as a result of successful acquire. A
* cancelled thread never succeeds in acquiring, and a thread only
* cancels itself, not any other node.
*/
volatile Node prev;
/**
* Link to the successor node that the current node/thread
* unparks upon release. Assigned during enqueuing, adjusted
* when bypassing cancelled predecessors, and nulled out (for
* sake of GC) when dequeued. The enq operation does not
* assign next field of a predecessor until after attachment,
* so seeing a null next field does not necessarily mean that
* node is at end of queue. However, if a next field appears
* to be null, we can scan prev's from the tail to
* double-check. The next field of cancelled nodes is set to
* point to the node itself instead of null, to make life
* easier for isOnSyncQueue.
*/
volatile Node next;
/**
* The thread that enqueued this node. Initialized on
* construction and nulled out after use.
*/
volatile Thread thread;
/**
* Link to next node waiting on condition, or the special
* value SHARED. Because condition queues are accessed only
* when holding in exclusive mode, we just need a simple
* linked queue to hold nodes while they are waiting on
* conditions. They are then transferred to the queue to
* re-acquire. And because conditions can only be exclusive,
* we save a field by using special value to indicate shared
* mode.
*/
Node nextWaiter;
/**
* Returns true if node is waiting in shared mode.
*/
final boolean isShared() {
return nextWaiter == SHARED;
}
/**
* Returns previous node, or throws NullPointerException if null.
* Use when predecessor cannot be null. The null check could
* be elided, but is present to help the VM.
*
* @return the predecessor of this node
*/
final Node predecessor() throws NullPointerException {
Node p = prev;
if (p == null)
throw new NullPointerException();
else
return p;
}
Node() { // Used to establish initial head or SHARED marker
}
Node(Thread thread, Node mode) { // Used by addWaiter
this.nextWaiter = mode;
this.thread = thread;
}
Node(Thread thread, int waitStatus) { // Used by Condition
this.waitStatus = waitStatus;
this.thread = thread;
}
}
1.4 总结
概念 | 解释 | ||||
非公平锁与公平锁的区别 | 非公平锁:
公平锁:
| ||||
ReentrantLock与synchronized的区别 |
|
lock流程图
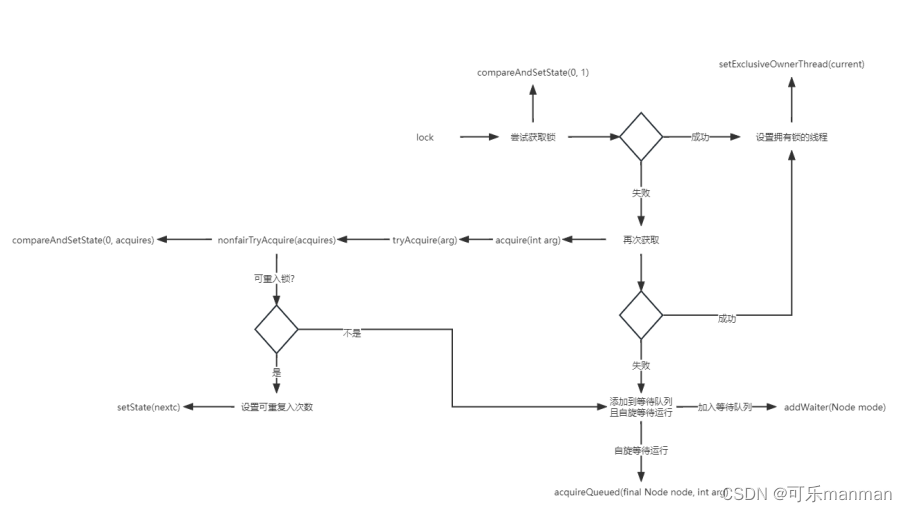