目录
6、提供给外界调用的方式。如果不想要编辑按钮可以设置为字符串
效果图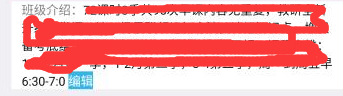
自定义AppcompatTextview
1、创建类继承AppcompatText
public class CustomTextView extends AppCompatTextView {
}
2、实现构造方法
public CustomTextView(Context context) {
this(context, null);
}
public CustomTextView(Context context, AttributeSet attrs) {
this(context, attrs, -1);
}
public CustomTextView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initPaint();
}
3、初始化代码
private TextPaint mPaint;//画笔
private int screenWidth;//屏幕宽度
private String start; // 起始文字
private String content; // 中间内容
private String end; // 结尾按钮
private int textSize = DensityUtil.dip2px(getContext(), 14);//画笔大小
private int topx; // 按钮左边的起始位置
private int topy; // 按钮右边的起始位置
private void initPaint() {
DisplayMetrics dm = getResources().getDisplayMetrics();
screenWidth = dm.widthPixels;
mPaint = new TextPaint();
mPaint.setTextSize(textSize);
mPaint.setColor(Color.RED);
mPaint.setAntiAlias(true);
setOnTouchListener((v, event) -> {
float x = event.getX();
float y = event.getY();
if (x > topx && x < topx + DensityUtil.dip2px(getContext(), 40)
&& y > topy && y < topy + DensityUtil.dip2px(getContext(), 20)) {
LogUtil.i("点击范围内");
if (null != onClick) {
onClick.onClick(v);
}
}
return false;
});
}
4、通过ondraw方法进行绘制
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
mPaint.setColor(getContext().getResources().getColor(R.color.transparency));
canvas.drawRect(0, 0, getMeasuredWidth(), getMeasuredHeight(), mPaint);
Rect bounds1 = new Rect();
if (!TextUtils.isEmpty(start)) {
mPaint.getTextBounds(start, 0, start.length(), bounds1);
// 绘制开始的文字。并获取到问自己的宽度
mPaint.setColor(getContext().getResources().getColor(R.color.color_999999));
canvas.drawText(start, 0, bounds1.height(), mPaint);
}
if (!TextUtils.isEmpty(content)) {
// 绘制中间的文字,通过递归的方式
mPaint.setColor(getContext().getResources().getColor(R.color.color_333333));
int contentWidth = bounds1.width() + DensityUtil.dip2px(getContext(), 10);
lineFeed(canvas, content, contentWidth, textSize);
}
// 绘制最后按钮的位置
String cropText = SPUtils.getInstance().getString("cropText");
int cropHeight = SPUtils.getInstance().getInt("cropHeight", -1);
Rect bounds2 = new Rect();
mPaint.getTextBounds(TextUtils.isEmpty(cropText) ? "" : "测" + cropText, 0, cropText.length(), bounds2);
if (screenWidth - bounds2.width() - DensityUtil.dip2px(getContext(), 111) > 0 && !TextUtils.isEmpty(cropText)) {
// 是否是第一行
if (cropText.equals(content)) { // 第一行的操作
topx = bounds2.width() + DensityUtil.dip2px(getContext(), 10) + bounds1.width();
topy = 0;
} else { // 其余行数的操作
topx = bounds2.width() + DensityUtil.dip2px(getContext(), 10);
topy = cropHeight - textSize;
}
} else {
if (textSize == cropHeight) { // 第一行
topx = 0;
topy = cropHeight + DensityUtil.dip2px(getContext(), 12);
} else { // 其余行的位置
topx = 0;
topy = cropHeight + DensityUtil.dip2px(getContext(), 12);
}
}
if (!TextUtils.isEmpty(end)) {
// 绘制按钮背景
Bitmap bitmap = getBitmap(getContext(), R.drawable.device_st_mc_btn_bg);
canvas.drawBitmap(bitmap, topx, topy, mPaint);
// 绘制按钮文字
mPaint.setColor(getContext().getResources().getColor(R.color.white));
mPaint.setTextSize(DensityUtil.dip2px(getContext(), 11));
canvas.drawText(end, topx + DensityUtil.dip2px(getContext(), 8)
, textSize == cropHeight ? topy + cropHeight : topy + bounds2.height() + DensityUtil.dip2px(getContext(), 1), mPaint);
}
setHeight(cropHeight + DensityUtil.dip2px(getContext(), 30));
}
/**
* @param canvas 画布
* @param str 绘制内容
* @param heigth 每一行的高度
*/
private void lineFeed(Canvas canvas, String str, int x, int heigth) {
if (TextUtils.isEmpty(str)) {
return;
}
int lineWidth = screenWidth - x - DensityUtil.dip2px(getContext(), 60);
//计算当前宽度(width)能显示多少个汉字
int subIndex = mPaint.breakText(str, 0, str.length(), true, lineWidth, null);
//截取可以显示的汉字
String mytext = str.substring(0, subIndex);
canvas.drawText(mytext, x, heigth, mPaint);
// 动态获取文字的宽高
Rect bounds1 = new Rect();
mPaint.getTextBounds(mytext, 0, mytext.length(), bounds1);
//计算剩下的汉字
String ss = str.substring(subIndex, str.length());
if (mytext.equals(content)) { // 如果只有一行的时候
// 判断第一行设置后是否还有空余的位置放置按钮 51为按钮占据的宽度+距离右边文字的距离+1像素
if (lineWidth - bounds1.width() - DensityUtil.dip2px(getContext(), 51) > 0) {
SPUtils.getInstance().put("cropHeight", heigth);
SPUtils.getInstance().put("cropText", str);
} else {
SPUtils.getInstance().put("cropHeight", textSize);
SPUtils.getInstance().put("cropText", ss);
}
} else {
// 判断是否需要换行 51为按钮占据的宽度+距离右边文字的距离+1像素
if (lineWidth - bounds1.width() - DensityUtil.dip2px(getContext(), 51) > 0) {
SPUtils.getInstance().put("cropText", str);
SPUtils.getInstance().put("cropHeight", heigth);
} else {
SPUtils.getInstance().put("cropText", str);
SPUtils.getInstance().put("cropHeight", heigth);
}
}
if (ss.length() > 0) {
lineFeed(canvas, ss, 0, heigth + textSize + DensityUtil.dip2px(getContext(), 6));
}
}
/**
* 绘制图片
*
* @param context
* @param vectorDrawableId
* @return
*/
private static Bitmap getBitmap(Context context, int vectorDrawableId) {
Bitmap bitmap = null;
if (Build.VERSION.SDK_INT > Build.VERSION_CODES.LOLLIPOP) {
Drawable vectorDrawable = context.getDrawable(vectorDrawableId);
bitmap = Bitmap.createBitmap(DensityUtil.dip2px(context, 40),
DensityUtil.dip2px(context, 20), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
vectorDrawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
vectorDrawable.draw(canvas);
} else {
bitmap = BitmapFactory.decodeResource(context.getResources(), vectorDrawableId);
}
return bitmap;
}
5、创建点击事件的回调
public void setOnClick(onClick onClick) {
this.onClick = onClick;
}
public interface onClick {
void onClick(View view);
}
6、提供给外界调用的方式。如果不想要编辑按钮可以设置为字符串
public void setText(String start, String content, String end) {
this.start = start;
this.content = content;
this.end = end;
mPaint.setTextSize(textSize);
invalidate();
}
7、使用方式(布局中的代码)
<com.wisdom.tdweilaiapp.device.weight.CustomTextView
android:id="@+id/classDesc"
android:layout_width="match_parent"
android:layout_height="500dp"
android:layout_marginLeft="@dimen/width_30"
android:layout_marginTop="@dimen/width_8"
android:layout_marginRight="@dimen/width_30"
android:textColor="@color/color_999999"
android:textSize="14sp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@id/classStudentNum" />
8、使用方式。代码中的调用
CustomTextView classDesc = findviewbyid(R.id.classdesc);
classDesc.setText("班级介绍:", model.getRemark()+"学员人数学员人数学员人数学员人数学员人数学员人数学员人数dfasdfasdfsdfasfasfdsfa", "编辑");