Java 8 new features with Example
https://www.youtube.com/playlist?list=PLsyeobzWxl7qbvNnJKjYbkTLn2w3eRy1Q
一、可以在接口中写方法体
在接口中增加新方法时,可以带方法体。
带来的好处:
当新接口发布时,以前实现该接口的类,无需改动。
即:无需实现新添加的方法。
语法:使用 default 关键字
注意:
1、实现多个接口时,接口中有重名的方法体的实现。
如果同时实现两个具有相同方法名且实现了该方法的接口,
需要提供自己对该方法的实现。才可以解决冲突。
sayHello() 重复,类 C 需要实现自己的 sayHello()
2、类方法的 优先级 高于 接口方法
接口 A 和 类 B 中都定义了方法 sayHello() 的实现,
类 C 优先使用 类 B 的方法。
3、接口中不可以重写 java.lang.Object 里面的方法
4、可以在接口中定义 static 方法
二、Functional Programming VS. Object Oriented Programming
Functional Programming with Java 8
https://www.youtube.com/watch?v=LcfzV38YDu4
面向函数的编程(Functional Programming)是指对于只有一个方法的接口可以使用 Lambda 表达式实现。
只有一个方法的接口很好的一个例子就是: java.lang.Comparable 接口。
它只有一个方法: compareTo(Object obj)
三、Lambda Expression ( -> 斜着看 )
Lambda Expression VS. Anonymous inner class
对于只有一个方法的接口的实现类,写实现类时,只需提供( 参数 + Lambda + 方法体 )即可。
说明:
四、为 java.lang.Iterable 接口增加了 forEach() 方法
NOTE:
1、java 8 之前, java.lang.Iterable 接口只有一个方法:
java.util.Iterator<T> iterator()
2、java.util.Collection 接口,继承了 java.lang.Iterable 接口。
五、Streaming API
Java collections got a new package java.util.Stream.
classes in this package provide a stream API.
And supports functional-style operations on streams of elements.
Stream API enables bulk operations like sequential or parallel map-readuce on Collections.
-
So, if you know, in this world, it's an information age, we have a concept of "Big Data", "Haddoop", we have to process huge amount of data.
1) In stream we have two types of methods:
1. Intermediate method. Like: filter(), map()
lazy, it can't give result immediately until you call a terminate method.
2. Terminate method. Like: findFirst(), forEach()
Example Given:
2) Stream is once used, it can't be reused:
更多:
Java 9 新特性
http://programtalk.com/java/java-9-new-features/
引用:
Java 8 Stream API Features Lambda Expression
https://www.youtube.com/playlist?list=PLsyeobzWxl7otduRddQWYTQezVul0xIX6
http://programtalk.com/java/java-8-new-features/
https://www.youtube.com/playlist?list=PLsyeobzWxl7qbvNnJKjYbkTLn2w3eRy1Q
一、可以在接口中写方法体
在接口中增加新方法时,可以带方法体。
带来的好处:
当新接口发布时,以前实现该接口的类,无需改动。
即:无需实现新添加的方法。
语法:使用 default 关键字
- interface A{
- void show();
- default void sayHello(){
- System.out.println("Hello, World!");
- }
- }
注意:
1、实现多个接口时,接口中有重名的方法体的实现。
如果同时实现两个具有相同方法名且实现了该方法的接口,
需要提供自己对该方法的实现。才可以解决冲突。
sayHello() 重复,类 C 需要实现自己的 sayHello()
- interface A{
- void showA();
- default void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- interface B{
- void showB();
- default void sayHello(){
- System.out.println("Hello, B!");
- }
- }
- class C implements A,B{
- public void sayHello(){
- System.out.println("Hello, C!");
- }
- public static void main(String[] args){
- C c = new C();
- c.sayHello(); // Hello, C!
- }
- }
2、类方法的 优先级 高于 接口方法
接口 A 和 类 B 中都定义了方法 sayHello() 的实现,
类 C 优先使用 类 B 的方法。
- interface A{
- void showA();
- default void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- class B{
- public void sayHello(){
- System.out.println("Hello, B!");
- }
- }
- class C extends B implements A{
- public static void main(String[] args){
- C c = new C();
- c.sayHello(); // Hello, B!
- }
- }
3、接口中不可以重写 java.lang.Object 里面的方法
- interface A {
- // can't define a equals method in interface.
- default public boolean equals(){
- }
- }
4、可以在接口中定义 static 方法
- interface A{
- void showA();
- static void sayHello(){
- System.out.println("Hello, A!");
- }
- }
- class C {
- public static void main(String[] args){
- A.sayHello(); // Hello, A!
- }
- }
二、Functional Programming VS. Object Oriented Programming
Functional Programming with Java 8
https://www.youtube.com/watch?v=LcfzV38YDu4
面向函数的编程(Functional Programming)是指对于只有一个方法的接口可以使用 Lambda 表达式实现。
只有一个方法的接口很好的一个例子就是: java.lang.Comparable 接口。
它只有一个方法: compareTo(Object obj)
三、Lambda Expression ( -> 斜着看 )

Lambda Expression VS. Anonymous inner class
对于只有一个方法的接口的实现类,写实现类时,只需提供( 参数 + Lambda + 方法体 )即可。
- interface A{
- void show();
- }
- public class LambdaDemo{
- public static void main(String[] args){
- A obj;
- // old
- obj = new A(){
- public void show(){
- System.out.println("Hello");
- }
- }
- // java 8
- obj = () -> {
- System.out.println("Multiple");
- System.out.println("Lines ... ");
- }
- // or
- obj = () -> System.out.println("Single line.");
- }
- }
说明:
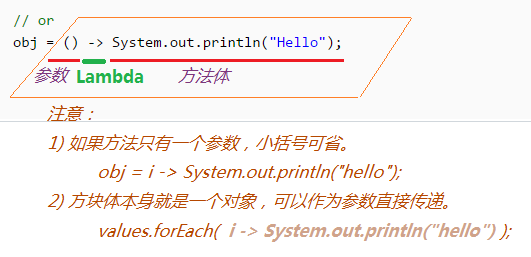
四、为 java.lang.Iterable 接口增加了 forEach() 方法
NOTE:
1、java 8 之前, java.lang.Iterable 接口只有一个方法:
java.util.Iterator<T> iterator()
2、java.util.Collection 接口,继承了 java.lang.Iterable 接口。
- import java.util.Arrays;
- import java.util.List;
- import java.util.function.Consumer;
- class IConsumer implements Consumer<Integer>{
- @Override
- public void accept(Integer t) {
- System.out.println(t);
- }
- }
- public class Java8ForEach {
- public static void main(String[] args) {
- List<Integer> list =Arrays.asList(1,2,3,5,6);
- // normal loop
- for(Integer i : list){
- System.out.println(i);
- }
- // Java 8 forEach - normal
- Consumer<Integer> action = new IConsumer();
- list.forEach(action);
- // Java 8 forEach - use lambda expressions.
- // see how we do it in one liner
- list.forEach(each -> System.out.println(each));
- }
- }
五、Streaming API
Java collections got a new package java.util.Stream.
classes in this package provide a stream API.
And supports functional-style operations on streams of elements.
Stream API enables bulk operations like sequential or parallel map-readuce on Collections.
- //Java 7 or earlier:
- public List listFilter(List<Integer> bigList){
- List<Integer> filteredList = new ArrayList<>();
- for (Integer p : bigList) {
- if (p > 40) {
- filteredList.add(p);
- }
- }
- return filteredList;
- }
- //Java 8:
- public List listFilter(List<integer> bigList){
- return bigList
- .stream()
- .filter(p -> p > 40)
- .collect(Collectors.toList());
- }
-
So, if you know, in this world, it's an information age, we have a concept of "Big Data", "Haddoop", we have to process huge amount of data.
1) In stream we have two types of methods:
1. Intermediate method. Like: filter(), map()
lazy, it can't give result immediately until you call a terminate method.
2. Terminate method. Like: findFirst(), forEach()
Example Given:
- List<Integer> values = Arrays.asList(4,5,6,7,8);
- values.stream().filter(i->{
- Sysout.out.println("Hello");
- return true;
- });
2) Stream is once used, it can't be reused:
- Stream<Integer> s = values.stream();
- s.forEach(Sysout.out::println); // works
- s.forEach(Sysout.out::println); // throws Exception
更多:
Java 9 新特性
http://programtalk.com/java/java-9-new-features/
引用:
Java 8 Stream API Features Lambda Expression
https://www.youtube.com/playlist?list=PLsyeobzWxl7otduRddQWYTQezVul0xIX6
http://programtalk.com/java/java-8-new-features/