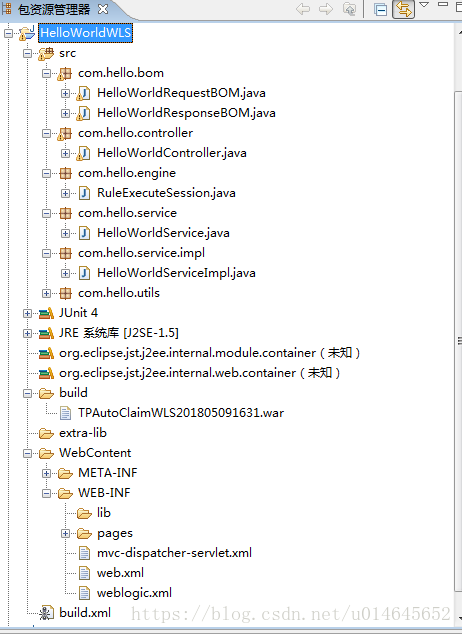
BOM
1. HelloWorldRequestBOM.java:
package com.hello.bom;
import java.io.Serializable;
public class HelloWorldRequestBOM implements Serializable {
private String requestType="";
private String name="";
private int age=0;
private String result="";
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRequestType() {
return requestType;
}
public void setRequestType(String requestType) {
this.requestType = requestType;
}
public String getResult() {
return result;
}
public void setResult(String result) {
this.result = result;
}
}
2. HelloWorldResponseBOM.java:
package com.hello.bom;
import java.io.Serializable;
public class HelloWorldResponseBOM implements Serializable {
private String requestType="";
private String name="";
private int age=0;
private String result="";
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRequestType() {
return requestType;
}
public void setRequestType(String requestType) {
this.requestType = requestType;
}
public String getResult() {
return result;
}
public void setResult(String result) {
this.result = result;
}
}
controller
1. HelloWorldController.java:
package com.hello.controller
import java.io.IOException
import java.io.PrintWriter
import java.util.Date
import javax.annotation.Resource
import javax.servlet.http.HttpServletRequest
import javax.servlet.http.HttpServletResponse
import org.springframework.stereotype.Controller
import org.springframework.web.bind.annotation.RequestMapping
import com.hello.bom.HelloWorldRequestBOM
import com.hello.bom.HelloWorldResponseBOM
import com.hello.service.HelloWorldService
import com.hello.utils.Byte2StringUtils
import com.hello.utils.ObjectXmlConvertor
@Controller
@RequestMapping(value = "/helloWorldService")
public class HelloWorldController {
@Resource(name = "HelloWorldServiceImpl")
private HelloWorldService servcie
private final static String errorMsg = "规则库调用异常,堆栈信息如下:\n"
@RequestMapping(params = "method=helloWorldRule")
public void helloWorldRule(HttpServletRequest request,
HttpServletResponse response) throws Exception {
String data = null
try {
String xml = Byte2StringUtils.toString(request.getReader())
if (xml == null || "".equals(xml)) {
String ret = new Date().toLocaleString()
+ ",helloWorldRule调用失败,接口xml传值为空"
System.out.println(ret)
data = ret
} else {
System.out.println("xml start:" + xml + "\n")
HelloWorldRequestBOM requestDto = (HelloWorldRequestBOM) ObjectXmlConvertor
.xml2javaBean(xml, HelloWorldRequestBOM.class)
HelloWorldResponseBOM responseBOM = servcie
.helloWorldService(requestDto)
data = ObjectXmlConvertor.javaBean2Xml(responseBOM)
}
} catch (Exception e) {
e.printStackTrace()
data = errorMsg + e.getMessage()
}
sendResponseData(response, data)
}
private void sendResponseData(HttpServletResponse response, String data) {
try {
response.setCharacterEncoding("UTF-8")
response.setContentType("text/html; charset=utf-8")
PrintWriter out = response.getWriter()
out.println(data)
out.close()
} catch (IOException e) {
e.printStackTrace()
}
}
}
engine
1. RuleExecuteSession.java:
package com.hello.engine;
import ilog.rules.bres.session.IlrRuleSessionProvider;
import ilog.rules.bres.session.IlrRuleSessionProviderFactory;
import ilog.rules.bres.session.IlrSessionExecutionResult;
import ilog.rules.bres.session.IlrSessionExecutionSettings;
import ilog.rules.bres.session.IlrSessionParameters;
import ilog.rules.bres.session.IlrSessionRequest;
import ilog.rules.bres.session.IlrSessionResponse;
import ilog.rules.bres.session.IlrStatelessRuleSession;
import java.io.Serializable;
import com.hello.bom.HelloWorldRequestBOM;
import com.hello.bom.HelloWorldResponseBOM;
public class RuleExecuteSession implements Serializable {
private static final long serialVersionUID = -5923262005370268548L;
private static final String helloWorldRequestBOM_in = "helloWorldRequestBOM";
private static final String helloWorldResponseBOM_out = "helloWorldResponseBOM";
/**
* 执行helloworld提示规则
*
* @param bom
* @param rulePath
* @return
* @throws Exception
*/
public Object runDocumentRules(HelloWorldRequestBOM bom,
HelloWorldResponseBOM resultBOM, String rulePath) throws Exception {
String sessionType = "IlrSimpleRuleSessionProvider";
IlrRuleSessionProvider provider = new IlrRuleSessionProviderFactory.Builder(
sessionType).build();
IlrStatelessRuleSession rs = provider.createStatelessRuleSession();
IlrSessionRequest request = new IlrSessionRequest(rulePath);
IlrSessionExecutionSettings settings = request.getExecutionSettings();
IlrSessionParameters pars = settings.getInputParameters();
pars.setParameter(helloWorldRequestBOM_in, bom);
pars.setParameter(helloWorldResponseBOM_out, resultBOM);
IlrSessionResponse response = rs.executeRules(request);
System.out.println("------------执行helloworld提示规则-----------------");
IlrSessionExecutionResult result = response.getExecutionResult();
IlrSessionParameters outPars = result.getOutputParameters();
resultBOM = (HelloWorldResponseBOM) outPars
.getObjectValue(helloWorldResponseBOM_out);
return resultBOM;
}
}
service
1. HelloWorldService.java:
package com.hello.service.impl;
import org.springframework.stereotype.Service;
import com.hello.bom.HelloWorldRequestBOM;
import com.hello.bom.HelloWorldResponseBOM;
import com.hello.engine.RuleExecuteSession;
import com.hello.service.HelloWorldService;
@Service("HelloWorldServiceImpl")
public class HelloWorldServiceImpl implements HelloWorldService {
private RuleExecuteSession session = new RuleExecuteSession();
public HelloWorldResponseBOM helloWorldService(
HelloWorldRequestBOM requestBOM) {
HelloWorldResponseBOM responseBOM = new HelloWorldResponseBOM();
try {
System.out.println("helloWorldService开始执行规则*******************:");
session.runDocumentRules(
requestBOM, responseBOM,
"/HelloWorldRuleApp/HelloWorldRule");
System.out.println("helloWorldService请求成功*******************:");
} catch (Exception e) {
e.printStackTrace();
}
return responseBOM;
}
}
utils
1. Byte2StringUtils.java
package com.hello.utils;
import java.io.BufferedReader;
import java.io.IOException;
public class Byte2StringUtils {
public static String toString(BufferedReader br) throws IOException {
String inputLine = null;
StringBuffer sb = new StringBuffer();
try {
while ((inputLine = br.readLine()) != null) {
sb.append(inputLine);
}
} catch (IOException e) {
e.printStackTrace();
throw e;
}finally{
br.close();
}
return sb.toString();
}
}
2. JavaBeanUtils.java:
package com.hello.utils;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class JavaBeanUtils {
@SuppressWarnings("unchecked")
public static Method getGetMethod(Class objectClass, String fieldName) throws SecurityException, NoSuchMethodException {
StringBuffer sb = new StringBuffer();
sb.append("get");
sb.append(fieldName.substring(0, 1).toUpperCase());
sb.append(fieldName.substring(1));
return objectClass.getMethod(sb.toString());
}
@SuppressWarnings("unchecked")
public static Method getSetMethod(Class objectClass, String fieldName) throws SecurityException, NoSuchFieldException, NoSuchMethodException {
Class[] parameterTypes = new Class[1];
Field field = objectClass.getDeclaredField(fieldName);
parameterTypes[0] = field.getType();
StringBuffer sb = new StringBuffer();
sb.append("set");
sb.append(fieldName.substring(0, 1).toUpperCase());
sb.append(fieldName.substring(1));
Method method = objectClass.getMethod(sb.toString(), parameterTypes);
return method;
}
public static void invokeSet(Object o, String fieldName, Object value) throws SecurityException, NoSuchFieldException, NoSuchMethodException, IllegalArgumentException, IllegalAccessException, InvocationTargetException {
Method method = getSetMethod(o.getClass(), fieldName);
if(value!=null)
method.invoke(o, new Object[] { value });
}
public static Object invokeGet(Object o, String fieldName) throws SecurityException, NoSuchMethodException, IllegalArgumentException, IllegalAccessException, InvocationTargetException {
Method method = getGetMethod(o.getClass(), fieldName);
return method.invoke(o, new Object[0]);
}
}
3. ObjectXmlConvertor.java:
package com.hello.utils
import java.io.ByteArrayInputStream
import java.io.ByteArrayOutputStream
import java.io.InputStream
import java.io.OutputStream
import org.apache.commons.logging.Log
import org.apache.commons.logging.LogFactory
import com.wutka.jox.JOXBeanInputStream
import com.wutka.jox.JOXBeanOutputStream
public class ObjectXmlConvertor {
protected static final Log logger = LogFactory.getLog(ObjectXmlConvertor.class)
public static String javaBean2Xml(Object o){
OutputStream xmlData = null
try {
xmlData = new ByteArrayOutputStream()
JOXBeanOutputStream joxOut = new JOXBeanOutputStream(xmlData,"gbk")
joxOut.writeObject(o.getClass().getName(), o)
} catch (Exception e) {
e.printStackTrace()
logger.error("JavaBean To XML Error",e)
}
return xmlData.toString()
}
public static Object xml2javaBean(String xml,Class className){
try {
InputStream in = new ByteArrayInputStream(xml.getBytes("gbk"))
JOXBeanInputStream joxIn = new JOXBeanInputStream(in)
Object o = (Object) joxIn.readObject(className)
return o
} catch (Exception e) {
e.printStackTrace()
logger.error("XML To JavaBean Error",e)
return null
}
}
}
4. mvc-dispatcher-servlet.xml:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd">
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix">
<value>/WEB-INF/pages/</value>
</property>
<property name="suffix">
<value>.jsp</value>
</property>
</bean>
<context:component-scan base-package="com.hello" />
</beans>
5. web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
<display-name>Spring Web MVC Application</display-name>
<servlet>
<servlet-name>mvc-dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>mvc-dispatcher</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/mvc-dispatcher-servlet.xml</param-value>
</context-param>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<resource-ref>
<res-ref-name>eis/XUConnectionFactory</res-ref-name>
<res-type>javax.resource.cci.ConnectionFactory</res-type>
<res-auth>Application</res-auth>
<res-sharing-scope>Unshareable</res-sharing-scope>
</resource-ref>
</web-app>
<?xml version="1.0" encoding="UTF-8"?>
<weblogic-web-app>
<context-root>/HelloWorldWLS/</context-root>
<container-descriptor>
<prefer-web-inf-classes>true</prefer-web-inf-classes>
</container-descriptor>
<resource-description>
<res-ref-name>eis/XUConnectionFactory</res-ref-name>
<jndi-name>eis/XUConnectionFactory</jndi-name>
</resource-description>
<session-descriptor>
<cookie-name>ILOGRESConsoleCookie222</cookie-name>
</session-descriptor>
<jsp-descriptor>
<precompile>true</precompile>
</jsp-descriptor>
</weblogic-web-app>