SlideringDrawer的使用(抽屉效果)http://www.itlanbao.com/code/20150825/10000/100476.html
一 SlidingDrawer 这个类,也就是所谓的"抽屉"类。它的用法很简单,要包括handle ,和content .
handle 就是当你点击它的时候,content 要么抽抽屉要么关抽屉。
这是上下拉抽屉的效果,将 SlidingDrawer属性设置为android:orientation="vertical"即可
这是左右拉抽屉的效果,将 SlidingDrawer属性设置为android:orientation="horizontal"即可。
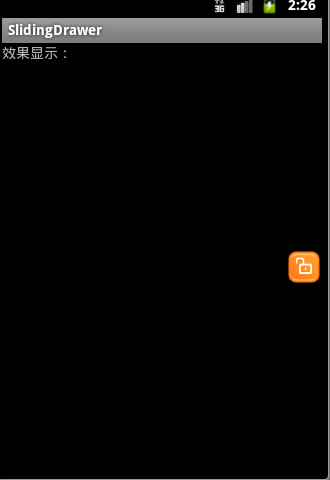
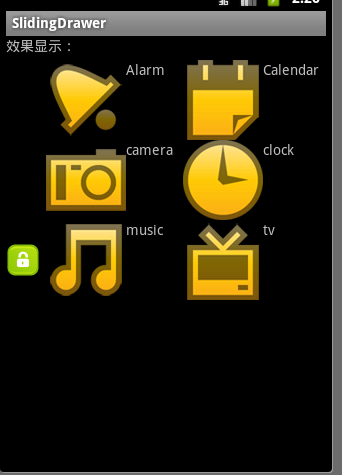
二、重要属性
android:allowSingleTap:指示是否可以通过handle打开或关闭
android:animateOnClick:指示是否当使用者按下手柄打开/关闭时是否该有一个动画。
android:content:隐藏的内容
android:handle:handle(手柄)
三、重要方法
animateClose():关闭时实现动画。
close():即时关闭
getContent():获取内容
isMoving():指示SlidingDrawer是否在移动。
isOpened():指示SlidingDrawer是否已全部打开
lock():屏蔽触摸事件。
setOnDrawerCloseListener(SlidingDrawer.OnDrawerCloseListener onDrawerCloseListener):SlidingDrawer关闭时调用
unlock():解除屏蔽触摸事件。
toggle():切换打开和关闭的抽屉SlidingDrawer。
上面例子的布局文件:
- <?xml version="1.0" encoding="utf-8"?>
- <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical" android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical" android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <TextView android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:text="效果显示:" />
- <SlidingDrawer android:id="@+id/drawer1"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="horizontal"
- android:handle="@+id/layout1"
- android:content="@+id/mycontent1">
- <LinearLayout android:id="@id/layout1"
- android:orientation="vertical"
- android:layout_width="35sp"
- android:layout_height="wrap_content"
- android:gravity="center">
- <ImageView android:id="@+id/myImage"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/open" />
- </LinearLayout>
- <GridView android:id="@id/mycontent1" android:layout_width="fill_parent"
- android:layout_height="fill_parent" android:numColumns="2"
- android:gravity="center" />
- </SlidingDrawer>
- </LinearLayout>
- </FrameLayout>
java文件:
- import android.app.Activity;
- import android.os.Bundle;
- import android.widget.GridView;
- import android.widget.ImageView;
- import android.widget.SlidingDrawer;
- import android.widget.SlidingDrawer.OnDrawerCloseListener;
- import android.widget.SlidingDrawer.OnDrawerOpenListener;
- import android.widget.SlidingDrawer.OnDrawerScrollListener;
- public class SlidingDrawerActivity extends Activity {
- /** Called when the activity is first created. */
- private SlidingDrawer sd;
- private GridView gv;
- private ImageView iv;
- private int [] itemIcons = new int []
- { R.drawable.alarm, R.drawable.calendar, R.drawable.camera, R.drawable.clock, R.drawable.music, R.drawable.tv };
- private String[] itemString = new String[]{"Alarm","Calendar","camera","clock","music","tv"};
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.slidrawer);
- init();
- GridAdapter adapter = new GridAdapter(SlidingDrawerActivity.this,itemString,itemIcons);
- gv.setAdapter(adapter);
- /* 设定SlidingDrawer被打开的事件处理 */
- sd.setOnDrawerOpenListener(new OnDrawerOpenListener() {
- @Override
- public void onDrawerOpened() {
- // TODO Auto-generated method stub
- iv.setImageResource(R.drawable.close);
- }
- });
- /* 设定SlidingDrawer被关闭的事件处理 */
- sd.setOnDrawerCloseListener(new OnDrawerCloseListener()
- {
- public void onDrawerClosed()
- {
- iv.setImageResource(R.drawable.open);
- }
- });
- sd.setOnDrawerScrollListener(new OnDrawerScrollListener() {
- @Override
- public void onScrollStarted() {
- // TODO Auto-generated method stub
- System.out.println("start");
- }
- @Override
- public void onScrollEnded() {
- // TODO Auto-generated method stub
- System.out.println("end");
- }
- });
- }
- private void init(){
- sd = (SlidingDrawer) findViewById(R.id.drawer1);
- gv = (GridView) findViewById(R.id.mycontent1);
- iv = (ImageView) findViewById(R.id.myImage);
- }
- }
- package com.shao.slider;
- import android.content.Context;
- import android.view.LayoutInflater;
- import android.view.View;
- import android.view.ViewGroup;
- import android.widget.BaseAdapter;
- import android.widget.ImageView;
- import android.widget.TextView;
- public class GridAdapter extends BaseAdapter {
- private Context context;
- private String[] itemString;
- private int[] itemIcons;
- public GridAdapter(Context con,String[] itemString,int[] itemIcons){
- context = con;
- this.itemString = itemString;
- this.itemIcons = itemIcons;
- }
- @Override
- public int getCount() {
- // TODO Auto-generated method stub
- return itemIcons.length;
- }
- @Override
- public Object getItem(int position) {
- // TODO Auto-generated method stub
- return itemString[position];
- }
- @Override
- public long getItemId(int position) {
- // TODO Auto-generated method stub
- return position;
- }
- @Override
- public View getView(int position, View convertView, ViewGroup parent) {
- // TODO Auto-generated method stub
- LayoutInflater inflater = LayoutInflater.from(context);
- /* 使用item.xml为每几个item的Layout */
- View v = inflater.inflate(R.layout.item, null);
- /* 取得View */
- ImageView iv = (ImageView) v.findViewById(R.id.item_grid);
- TextView tv = (TextView) v.findViewById(R.id.item_text);
- /* 设定显示的Image与文? */
- iv.setImageResource(itemIcons[position]);
- tv.setText(itemString[position]);
- return v;
- }
- }
还有注意的是:
SlidingDrawer should be used as an overlay inside layouts. This means SlidingDrawer should only be used inside of a FrameLayout or a RelativeLayout for instance,如果显示的时候不正常,考虑上面的原因。
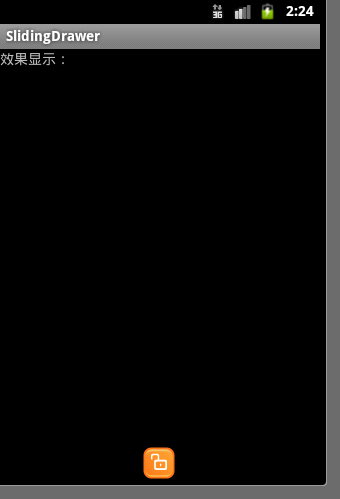
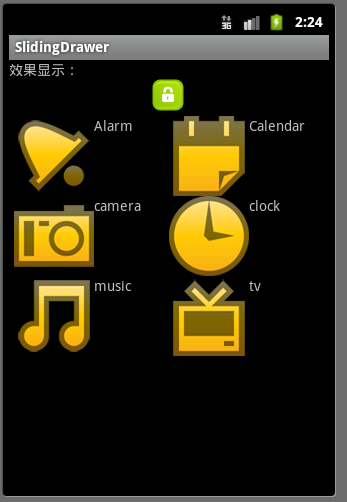
二、重要属性
android:allowSingleTap:指示是否可以通过handle打开或关闭
android:animateOnClick:指示是否当使用者按下手柄打开/关闭时是否该有一个动画。
android:content:隐藏的内容
android:handle:handle(手柄)
三、重要方法
animateClose():关闭时实现动画。
close():即时关闭
getContent():获取内容
isMoving():指示SlidingDrawer是否在移动。
isOpened():指示SlidingDrawer是否已全部打开
lock():屏蔽触摸事件。
setOnDrawerCloseListener(SlidingDrawer.OnDrawerCloseListener onDrawerCloseListener):SlidingDrawer关闭时调用
unlock():解除屏蔽触摸事件。
toggle():切换打开和关闭的抽屉SlidingDrawer。
上面例子的布局文件:
- <?xml version="1.0" encoding="utf-8"?>
- <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical" android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical" android:layout_width="fill_parent"
- android:layout_height="fill_parent">
- <TextView android:layout_width="fill_parent"
- android:layout_height="wrap_content"
- android:text="效果显示:" />
- <SlidingDrawer android:id="@+id/drawer1"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- android:orientation="horizontal"
- android:handle="@+id/layout1"
- android:content="@+id/mycontent1">
- <LinearLayout android:id="@id/layout1"
- android:orientation="vertical"
- android:layout_width="35sp"
- android:layout_height="wrap_content"
- android:gravity="center">
- <ImageView android:id="@+id/myImage"
- android:layout_width="wrap_content"
- android:layout_height="wrap_content"
- android:src="@drawable/open" />
- </LinearLayout>
- <GridView android:id="@id/mycontent1" android:layout_width="fill_parent"
- android:layout_height="fill_parent" android:numColumns="2"
- android:gravity="center" />
- </SlidingDrawer>
- </LinearLayout>
- </FrameLayout>
java文件:
- import android.app.Activity;
- import android.os.Bundle;
- import android.widget.GridView;
- import android.widget.ImageView;
- import android.widget.SlidingDrawer;
- import android.widget.SlidingDrawer.OnDrawerCloseListener;
- import android.widget.SlidingDrawer.OnDrawerOpenListener;
- import android.widget.SlidingDrawer.OnDrawerScrollListener;
- public class SlidingDrawerActivity extends Activity {
- /** Called when the activity is first created. */
- private SlidingDrawer sd;
- private GridView gv;
- private ImageView iv;
- private int [] itemIcons = new int []
- { R.drawable.alarm, R.drawable.calendar, R.drawable.camera, R.drawable.clock, R.drawable.music, R.drawable.tv };
- private String[] itemString = new String[]{"Alarm","Calendar","camera","clock","music","tv"};
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.slidrawer);
- init();
- GridAdapter adapter = new GridAdapter(SlidingDrawerActivity.this,itemString,itemIcons);
- gv.setAdapter(adapter);
- /* 设定SlidingDrawer被打开的事件处理 */
- sd.setOnDrawerOpenListener(new OnDrawerOpenListener() {
- @Override
- public void onDrawerOpened() {
- // TODO Auto-generated method stub
- iv.setImageResource(R.drawable.close);
- }
- });
- /* 设定SlidingDrawer被关闭的事件处理 */
- sd.setOnDrawerCloseListener(new OnDrawerCloseListener()
- {
- public void onDrawerClosed()
- {
- iv.setImageResource(R.drawable.open);
- }
- });
- sd.setOnDrawerScrollListener(new OnDrawerScrollListener() {
- @Override
- public void onScrollStarted() {
- // TODO Auto-generated method stub
- System.out.println("start");
- }
- @Override
- public void onScrollEnded() {
- // TODO Auto-generated method stub
- System.out.println("end");
- }
- });
- }
- private void init(){
- sd = (SlidingDrawer) findViewById(R.id.drawer1);
- gv = (GridView) findViewById(R.id.mycontent1);
- iv = (ImageView) findViewById(R.id.myImage);
- }
- }
- package com.shao.slider;
- import android.content.Context;
- import android.view.LayoutInflater;
- import android.view.View;
- import android.view.ViewGroup;
- import android.widget.BaseAdapter;
- import android.widget.ImageView;
- import android.widget.TextView;
- public class GridAdapter extends BaseAdapter {
- private Context context;
- private String[] itemString;
- private int[] itemIcons;
- public GridAdapter(Context con,String[] itemString,int[] itemIcons){
- context = con;
- this.itemString = itemString;
- this.itemIcons = itemIcons;
- }
- @Override
- public int getCount() {
- // TODO Auto-generated method stub
- return itemIcons.length;
- }
- @Override
- public Object getItem(int position) {
- // TODO Auto-generated method stub
- return itemString[position];
- }
- @Override
- public long getItemId(int position) {
- // TODO Auto-generated method stub
- return position;
- }
- @Override
- public View getView(int position, View convertView, ViewGroup parent) {
- // TODO Auto-generated method stub
- LayoutInflater inflater = LayoutInflater.from(context);
- /* 使用item.xml为每几个item的Layout */
- View v = inflater.inflate(R.layout.item, null);
- /* 取得View */
- ImageView iv = (ImageView) v.findViewById(R.id.item_grid);
- TextView tv = (TextView) v.findViewById(R.id.item_text);
- /* 设定显示的Image与文? */
- iv.setImageResource(itemIcons[position]);
- tv.setText(itemString[position]);
- return v;
- }
- }
还有注意的是:
SlidingDrawer should be used as an overlay inside layouts. This means SlidingDrawer should only be used inside of a FrameLayout or a RelativeLayout for instance,如果显示的时候不正常,考虑上面的原因。
android仿腾讯安全管家首页抽屉效果
- 博客分类:
- android
转载请说明出处
最近在做公司新产品的设计,看到腾讯安全管家首页的抽屉效果设计的挺不错,一方面可以讲经常使用的功能模块直接显示给用户,另一方面将用户不常用的功能模块隐藏起来,而这些功能模块的显示和隐藏可以通过一个抽屉组建实现。所以我们想将这个设计理念加入到我们的产品中。腾讯安全管家效果图如下:
虽然android 文档中向我们提供了一个叫SlidingDrawer的抽屉组建,但是这个组建的使用限制比较多,也实现不了我们想要的效果。故到网上搜了一会,也没看到有开发者写这样的组建。所以只能靠自己了,但是在网上还是看到了一下有价值的参考案例。
不费话了,直接上实现后的效果图:
下面是自定义组建的实现代码
- import android.content.Context;
- import android.os.AsyncTask;
- import android.util.Log;
- import android.view.GestureDetector;
- import android.view.MotionEvent;
- import android.view.View;
- import android.widget.Button;
- import android.widget.LinearLayout;
-
- public class CustomSlidingDrawer extends LinearLayout implements GestureDetector.OnGestureListener, View.OnTouchListener{
- private final static String TAG = "CustomSlidingDrawer";
-
- private Button btnHandler;
- private LinearLayout container;
- private int mBottonMargin=0;
- private GestureDetector mGestureDetector;
- private boolean mIsScrolling=false;
- private float mScrollY;
- private int moveDistance;
-
- public CustomSlidingDrawer(Context context,View otherView,int width,int height, int hideDistance) {
- super(context);
- moveDistance = hideDistance;
- //定义手势识别
- mGestureDetector = new GestureDetector(context,this);
- mGestureDetector.setIsLongpressEnabled(false);
-
- //改变CustomSlidingDrawer附近组件的属性
- LayoutParams otherLP=(LayoutParams) otherView.getLayoutParams();
- //这一步很重要,要不组建不会显示
- otherLP.weight=1;
- otherView.setLayoutParams(otherLP);
-
- //设置CustomSlidingDrawer本身的属性
- LayoutParams lp=new LayoutParams(width, height);
- lp.bottomMargin = -moveDistance;
- mBottonMargin=Math.abs(lp.bottomMargin);
- this.setLayoutParams(lp);
- this.setOrientation(LinearLayout.VERTICAL);
- //this.setBackgroundColor(Color.BLUE);
-
- //设置Handler的属性
- btnHandler=new Button(context);
- btnHandler.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT, 35));
- btnHandler.setOnTouchListener(this);
- this.addView(btnHandler);
-
- //设置Container的属性
- container=new LinearLayout(context);
- //container.setBackgroundColor(Color.GREEN);
- container.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT,
- LayoutParams.MATCH_PARENT));
- this.addView(container);
- }
-
- /**
- * 把View放在CustomSlidingDrawer的Container
- * @param v
- */
- public void fillPanelContainer(View v)
- {
- container.addView(v);
- }
-
- /**
- * 异步移动CustomSlidingDrawer
- */
- class AsynMove extends AsyncTask<Integer, Integer, Void> {
- @Override
- protected Void doInBackground(Integer... params) {
- Log.e(TAG, "AsynMove doInBackground");
- int times;
- if (mBottonMargin % Math.abs(params[0]) == 0)// 整除
- times = mBottonMargin / Math.abs(params[0]);
- else
- // 有余数
- times = mBottonMargin / Math.abs(params[0]) + 1;
- for (int i = 0; i < times; i++) {
- publishProgress(params);
- }
- return null;
- }
- @Override
- protected void onProgressUpdate(Integer... params) {
- Log.e(TAG, "AsynMove onProgressUpdate");
- LayoutParams lp = (LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (params[0] < 0)
- lp.bottomMargin = Math.max(lp.bottomMargin + params[0],
- (-mBottonMargin));
- else
- lp.bottomMargin = Math.min(lp.bottomMargin + params[0], 0);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- }
- }
-
- @Override
- public boolean onDown(MotionEvent e) {
- mScrollY=0;
- mIsScrolling=false;
- return false;
- }
-
- @Override
- public boolean onSingleTapUp(MotionEvent e) {
- LayoutParams lp = (LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin < 0)
- new AsynMove().execute(new Integer[] { moveDistance });// 正数展开
- else if (lp.bottomMargin >= 0)
- new AsynMove().execute(new Integer[] { -moveDistance });// 负数收缩
- return false;
- }
-
- @Override
- public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX,
- float distanceY) {
- mIsScrolling=true;
- mScrollY+=distanceY;
- LayoutParams lp=(LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin < -1 && mScrollY > 0) {//往上拖拉
- lp.bottomMargin = Math.min((lp.bottomMargin + (int) mScrollY),0);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- } else if (lp.bottomMargin > -(mBottonMargin) && mScrollY < 0) {//往下拖拉
- lp.bottomMargin = Math.max((lp.bottomMargin + (int) mScrollY),-mBottonMargin);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- }
- return false;
- }
-
- @Override
- public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
- float velocityY) {return false;}
- @Override
- public void onLongPress(MotionEvent e) {}
- @Override
- public void onShowPress(MotionEvent e) {}
-
- @Override
- public boolean onTouch(View v, MotionEvent event) {
- if(event.getAction()==MotionEvent.ACTION_UP && //onScroll时的ACTION_UP
- mIsScrolling==true)
- {
- LayoutParams lp=(LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin >= (-mBottonMargin/2)) {//往上超过一半
- new AsynMove().execute(new Integer[] { moveDistance });// 正数展开
- }
- else if (lp.bottomMargin < (-mBottonMargin/2)) {//往下拖拉
- new AsynMove().execute(new Integer[] { -moveDistance });// 负数收缩
- }
- }
- return mGestureDetector.onTouchEvent(event);
- }
- }
下面是demo的源码:
最近在做公司新产品的设计,看到腾讯安全管家首页的抽屉效果设计的挺不错,一方面可以讲经常使用的功能模块直接显示给用户,另一方面将用户不常用的功能模块隐藏起来,而这些功能模块的显示和隐藏可以通过一个抽屉组建实现。所以我们想将这个设计理念加入到我们的产品中。腾讯安全管家效果图如下:


虽然android 文档中向我们提供了一个叫SlidingDrawer的抽屉组建,但是这个组建的使用限制比较多,也实现不了我们想要的效果。故到网上搜了一会,也没看到有开发者写这样的组建。所以只能靠自己了,但是在网上还是看到了一下有价值的参考案例。
不费话了,直接上实现后的效果图:


下面是自定义组建的实现代码
- import android.content.Context;
- import android.os.AsyncTask;
- import android.util.Log;
- import android.view.GestureDetector;
- import android.view.MotionEvent;
- import android.view.View;
- import android.widget.Button;
- import android.widget.LinearLayout;
- public class CustomSlidingDrawer extends LinearLayout implements GestureDetector.OnGestureListener, View.OnTouchListener{
- private final static String TAG = "CustomSlidingDrawer";
- private Button btnHandler;
- private LinearLayout container;
- private int mBottonMargin=0;
- private GestureDetector mGestureDetector;
- private boolean mIsScrolling=false;
- private float mScrollY;
- private int moveDistance;
- public CustomSlidingDrawer(Context context,View otherView,int width,int height, int hideDistance) {
- super(context);
- moveDistance = hideDistance;
- //定义手势识别
- mGestureDetector = new GestureDetector(context,this);
- mGestureDetector.setIsLongpressEnabled(false);
- //改变CustomSlidingDrawer附近组件的属性
- LayoutParams otherLP=(LayoutParams) otherView.getLayoutParams();
- //这一步很重要,要不组建不会显示
- otherLP.weight=1;
- otherView.setLayoutParams(otherLP);
- //设置CustomSlidingDrawer本身的属性
- LayoutParams lp=new LayoutParams(width, height);
- lp.bottomMargin = -moveDistance;
- mBottonMargin=Math.abs(lp.bottomMargin);
- this.setLayoutParams(lp);
- this.setOrientation(LinearLayout.VERTICAL);
- //this.setBackgroundColor(Color.BLUE);
- //设置Handler的属性
- btnHandler=new Button(context);
- btnHandler.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT, 35));
- btnHandler.setOnTouchListener(this);
- this.addView(btnHandler);
- //设置Container的属性
- container=new LinearLayout(context);
- //container.setBackgroundColor(Color.GREEN);
- container.setLayoutParams(new LayoutParams(LayoutParams.MATCH_PARENT,
- LayoutParams.MATCH_PARENT));
- this.addView(container);
- }
- /**
- * 把View放在CustomSlidingDrawer的Container
- * @param v
- */
- public void fillPanelContainer(View v)
- {
- container.addView(v);
- }
- /**
- * 异步移动CustomSlidingDrawer
- */
- class AsynMove extends AsyncTask<Integer, Integer, Void> {
- @Override
- protected Void doInBackground(Integer... params) {
- Log.e(TAG, "AsynMove doInBackground");
- int times;
- if (mBottonMargin % Math.abs(params[0]) == 0)// 整除
- times = mBottonMargin / Math.abs(params[0]);
- else
- // 有余数
- times = mBottonMargin / Math.abs(params[0]) + 1;
- for (int i = 0; i < times; i++) {
- publishProgress(params);
- }
- return null;
- }
- @Override
- protected void onProgressUpdate(Integer... params) {
- Log.e(TAG, "AsynMove onProgressUpdate");
- LayoutParams lp = (LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (params[0] < 0)
- lp.bottomMargin = Math.max(lp.bottomMargin + params[0],
- (-mBottonMargin));
- else
- lp.bottomMargin = Math.min(lp.bottomMargin + params[0], 0);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- }
- }
- @Override
- public boolean onDown(MotionEvent e) {
- mScrollY=0;
- mIsScrolling=false;
- return false;
- }
- @Override
- public boolean onSingleTapUp(MotionEvent e) {
- LayoutParams lp = (LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin < 0)
- new AsynMove().execute(new Integer[] { moveDistance });// 正数展开
- else if (lp.bottomMargin >= 0)
- new AsynMove().execute(new Integer[] { -moveDistance });// 负数收缩
- return false;
- }
- @Override
- public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX,
- float distanceY) {
- mIsScrolling=true;
- mScrollY+=distanceY;
- LayoutParams lp=(LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin < -1 && mScrollY > 0) {//往上拖拉
- lp.bottomMargin = Math.min((lp.bottomMargin + (int) mScrollY),0);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- } else if (lp.bottomMargin > -(mBottonMargin) && mScrollY < 0) {//往下拖拉
- lp.bottomMargin = Math.max((lp.bottomMargin + (int) mScrollY),-mBottonMargin);
- CustomSlidingDrawer.this.setLayoutParams(lp);
- }
- return false;
- }
- @Override
- public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
- float velocityY) {return false;}
- @Override
- public void onLongPress(MotionEvent e) {}
- @Override
- public void onShowPress(MotionEvent e) {}
- @Override
- public boolean onTouch(View v, MotionEvent event) {
- if(event.getAction()==MotionEvent.ACTION_UP && //onScroll时的ACTION_UP
- mIsScrolling==true)
- {
- LayoutParams lp=(LayoutParams) CustomSlidingDrawer.this.getLayoutParams();
- if (lp.bottomMargin >= (-mBottonMargin/2)) {//往上超过一半
- new AsynMove().execute(new Integer[] { moveDistance });// 正数展开
- }
- else if (lp.bottomMargin < (-mBottonMargin/2)) {//往下拖拉
- new AsynMove().execute(new Integer[] { -moveDistance });// 负数收缩
- }
- }
- return mGestureDetector.onTouchEvent(event);
- }
- }
下面是demo的源码:
style="font: 12px/18px Helvetica, Tahoma, Arial, sans-serif; text-align: left; color: rgb(0, 0, 0); text-transform: none; text-indent: 0px; letter-spacing: normal; word-spacing: 0px; white-space: normal; widows: 1; font-size-adjust: none; font-stretch: normal; -webkit-text-stroke-width: 0px;" height="90" src="http://ff20081528.iteye.com/iframe_ggbd/187" frameborder="0" width="680" scrolling="no">