14、COMMAND—俺有一个MM家里管得特别严,没法见面,只好借助于她弟弟在我们俩之间传送信息,她对我有什么指示,就写一张纸条让她弟弟带给我。
这不,她弟弟又传送过来一个COMMAND,为了感谢他,我请他吃了碗杂酱面,哪知道他说:"我同时给我姐姐三个男朋友送COMMAND,就数你最小气,才请我吃面。",:-(
命令模式:命令模式把一个请求或者操作封装到一个对象中。命令模式把发出命令的责任和执行命令的责任分割开,委派给不同的对象。
the command pattern is a design pattern in which an object is used to represent and encapsulate all the information needed to call a method at a later time. This information includes the method name, the object that owns the method and values for the method parameters.
Three terms always associated with the command pattern are client, invoker and receiver. The client instantiates the command object and provides the information required to call the method at a later time. The invoker decides when the method should be called. The receiver is an instance of the class that contains the method's code.
Using command objects makes it easier to construct general components that need to delegate, sequence or execute method calls at a time of their choosing without the need to know the owner of the method or the method parameters.
命令模式允许请求的一方和发送的一方独立开来,使得请求的一方不必知道接收请求的一方的接口,更不必知道请求是怎么被接收,以及操作是否执行,
何时被执行以及是怎么被执行的。系统支持命令的撤消。
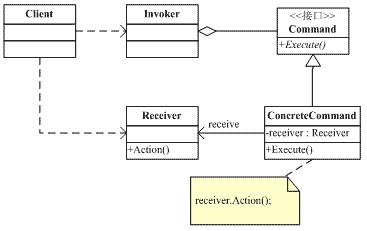
典型的Command模式需要有一个接口.接口中有一个统一的方法,这就是"将命令/请求封装为对象":
public interface Command {
public abstract void execute ( );
}
《Java与模式》一书中的例子如下:
/**
* 客户端(Client)代码
*/
public class Client {
public static void main(String[] args) {
Receiver receiver = new Receiver();
Command command = new ConcreteCommand(receiver);
Invoker invoker = new Invoker(command);
invoker.action();
}
}
/**
* 请求者(Invoker)角色代码
*/
public class Invoker {
private Command command;
public Invoker(Command command) {
this.command = command;
}
public void action() {
command.execute();
}
}
/**
* 接收者(Receiver)角色代码
*/
public class Receiver {
public Receiver() {
//write code here
}
public void action() {
System.out.println(""Action has been taken);
}
}
/**
* 抽象命令角色由Command接口扮演
*/
public class Command {
void execute();
}
/**
* 具体命令类
*/
public class ConcreteCommand implements Command {
private Receiver receiver;
public ConcreteCommand(Receiver receiver) {
this.receiver = receiver;
}
public void execute() {
receiver.action();
}
}
再用某公司写的Command模式,简化代码写个大概样子:
/**
* 客户端(Client)代码
*/
public class Client {
public static void main(String[] args) {
String receiverName = "xAO";
String methodName = "methodA";
//receiverName这里用Spring的依赖注入来创建Receiver对象
Command command = new ConcreteCommand(receiverName);
command.getParameters.put("method", methodName); //实际代码做了封装,并不是这样直接写的。这里简化一下
command.getParameters.put("param1", "param1"); //传递参数
command.getParameters.put("param2", "param2");
Result result = getNewInvoker.execute(command); //创建Invoker对象,并调用receiver执行最终的方法
}
}
/**
* 请求者(Invoker)角色代码
*/
public class Invoker {
private Command command;
public Invoker(Command command) {
this.command = command;
}
public void action() {
command.execute();
}
private BeanFactoryService beanFactory;
public Result execute(Command command) {
ApplicationObject ao = beanFactory.getBean(command.getName(), ApplicationObject.class, new XXProcessor(command));
return ao.execute(); //这里就是接收者Receiver来执行具体方法: xAO.methodA();
}
}
/**
* 抽象命令角色由Command接口扮演
*/
public class Command {
//...
//没有execute()方法
}
/**
* 具体命令类
*/
public class ConcreteCommand implements Command {
//也没有execute()方法
//...
}