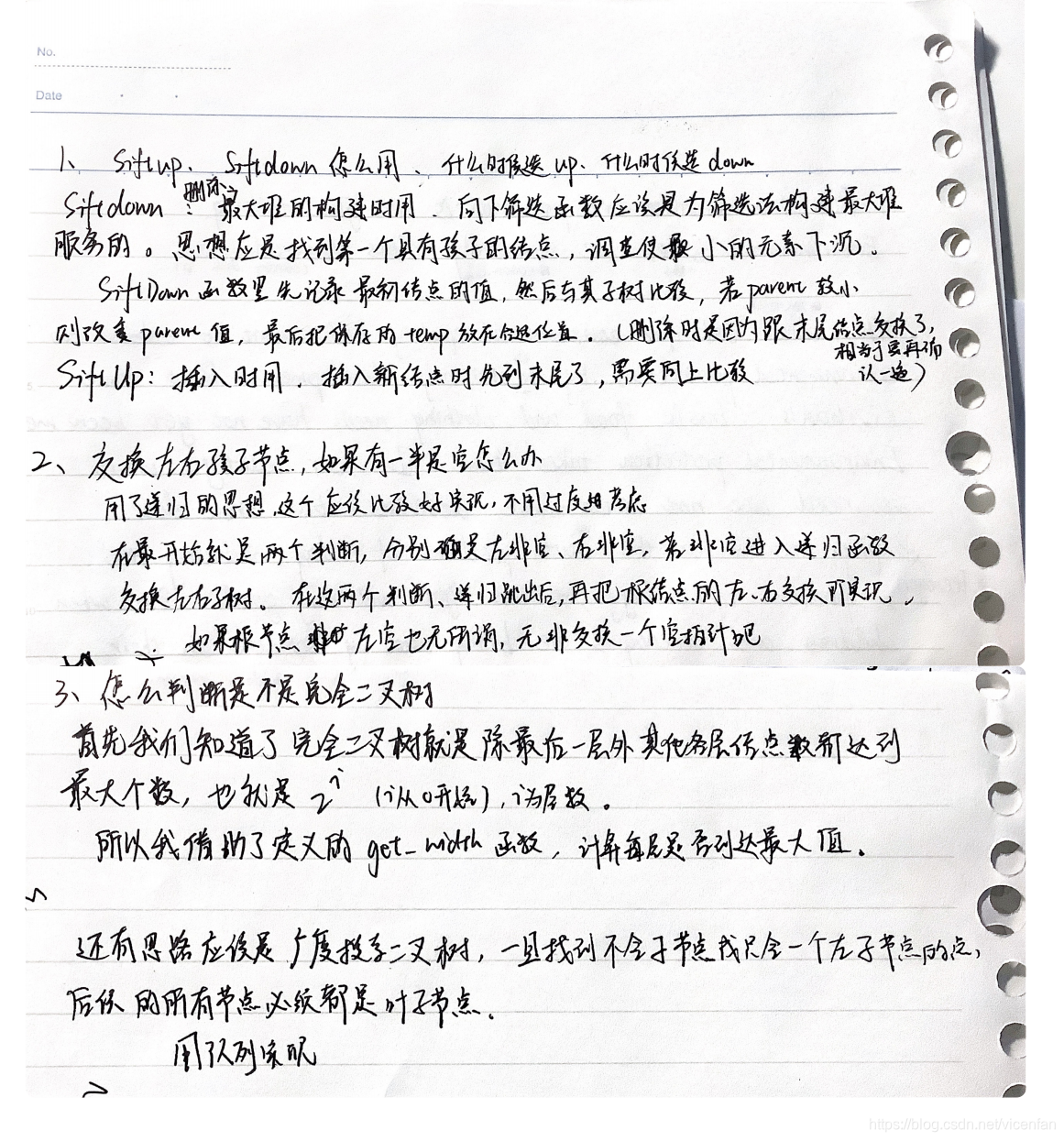
#include <iostream>
#include <stdlib.h>
using namespace std;
template <class T>
class MaxHeap
{
private:
T *heapArray;
int CurrentSize;
int MaxSize;
public:
MaxHeap(T *array, int num, int max)
{
this->heapArray = array;
this->CurrentSize = num;
this->MaxSize = max;
}
virtual ~MaxHeap(){};
bool isLeaf(int pos) const;
int leftchild(int pos) const;
int rightchild(int pos) const;
int parent(int pos) const;
void BuildHeap();
void SiftDown(int left);
void SiftUp(int pos);
bool Remove(int pos, T &node);
bool Insert(const T &newNode);
T &RemoveMax();
void visit();
};
template <class T>
void MaxHeap<T>::BuildHeap()
{
for (int i = CurrentSize / 2 - 1; i >= 0; i--)
SiftDown(i);
}
template <class T>
bool MaxHeap<T>::isLeaf(int pos) const
{
if (pos >= CurrentSize)
{
cout << "越界" << endl;
return (false);
}
else if (pos > (CurrentSize - 1) / 2)
return (true);
else
return (false);
}
template <class T>
int MaxHeap<T>::leftchild(int pos) const
{
return (2 * pos + 1);
}
template <class T>
int MaxHeap<T>::rightchild(int pos) const
{
return (2 * pos + 2);
}
template <class T>
int MaxHeap<T>::parent(int pos) const
{
return ((pos - 1) / 2);
}
template <class T>
bool MaxHeap<T>::Remove(int pos, T &node)
{
if (pos >= 0 && pos <= MaxSize - 1)
{
node = heapArray[pos];
heapArray[pos] = heapArray[CurrentSize - 1];
SiftDown(pos);
CurrentSize--;
return true;
}
else
{
cout << "空堆" << endl;
return false;
}
}
template <class T>
void MaxHeap<T>::SiftDown(int left)
{
int parent = left;
int child = 2 * parent + 1;
T temp = heapArray[parent];
while (child < CurrentSize)
{
if (child < CurrentSize - 1 && heapArray[child] < heapArray[child + 1])
{
child++;
}
if (temp < heapArray[child])
{
heapArray[parent] = heapArray[child];
parent = child;
child = 2 * child + 1;
}
else
{
break;
}
}
heapArray[parent] = temp;
}
template <class T>
void MaxHeap<T>::SiftUp(int pos)
{
int child = pos;
int parent = (child - 1) / 2;
T temp = heapArray[child];
while (parent >= 0 && child >= 1)
{
if (temp > heapArray[parent])
{
heapArray[child] = heapArray[parent];
child = parent;
parent = (child - 1) / 2;
}
else
{
break;
}
}
heapArray[child] = temp;
}
template <class T>
bool MaxHeap<T>::Insert(const T &newNode)
{
CurrentSize++;
if (CurrentSize > MaxSize)
{
cout << "堆满" << endl;
return false;
}
else
{
heapArray[CurrentSize - 1] = newNode;
SiftUp(CurrentSize - 1);
return true;
}
}
template <class T>
void MaxHeap<T>::visit()
{
for (int i = 0; i < CurrentSize; i++)
cout << heapArray[i] << " ";
cout << endl;
}
template <class T>
T &MaxHeap<T>::RemoveMax()
{
if (CurrentSize <= 0)
{
cout << "空堆" << endl;
exit;
}
else
{
T node;
Remove(0, node);
return node;
}
}
int main()
{
int count, maxSize;
cin >> count >> maxSize;
int iValue;
int *a = new int[count];
for (int i = 0; i < count; i++)
{
cin >> iValue;
a[i] = iValue;
}
MaxHeap<int> maxheap(a, count, maxSize);
int temp;
maxheap.BuildHeap();
cout << "构建堆后为:";
maxheap.visit();
cin >> iValue;
if (maxheap.isLeaf(iValue))
cout << "位置" << iValue << "是叶结点" << endl;
else
cout << "位置" << iValue << "不是叶结点" << endl;
maxheap.visit();
maxheap.RemoveMax();
cout << "移除最大值后:";
maxheap.visit();
cin >> iValue;
maxheap.Remove(iValue, temp);
cout << "删除下标为" << iValue << "的元素之后为:";
maxheap.visit();
cout << "删除下标为" << iValue << "的元素为" << temp << endl;
cin >> iValue;
maxheap.Insert(iValue);
cout << "插入" << iValue << "后为:";
maxheap.visit();
system("pause");
return (0);
}
#include <iostream>
#include <math.h>
#include <queue>
using namespace std;
class binarytreenode
{
int data;
public:
binarytreenode *leftchild;
binarytreenode *rightchild;
binarytreenode(int &d)
{
data = d;
leftchild = NULL;
rightchild = NULL;
}
int &get_data() { return data; }
void change_data(int &n) { data = n; }
~binarytreenode(){};
};
class binarytree
{
binarytreenode *root;
static int times;
int size;
public:
binarytree()
{
size = 0;
root = NULL;
}
int get_size() { return size; }
binarytreenode *get_root() { return root; }
void creat()
{
binarytreenode *prev;
cout << "输入int型数据:(以0结束)";
int temp;
cin >> temp;
while (temp != 0)
{
if (root == NULL)
{
root = new binarytreenode(temp);
size++;
prev = root;
}
else
{
prev = root;
size++;
for (;;)
{
if (temp < prev->get_data())
{
if (prev->leftchild == NULL)
{
prev->leftchild = new binarytreenode(temp);
break;
}
prev = prev->leftchild;
}
else
{
if (prev->rightchild == NULL)
{
prev->rightchild = new binarytreenode(temp);
break;
}
prev = prev->rightchild;
}
}
}
cin >> temp;
}
}
void levelorder()
{
binarytreenode *p = get_root();
queue<binarytreenode *> que;
cout << "广度遍历结果: ";
if (p != NULL)
que.push(p);
else
cout << "二叉树为空!";
while (!que.empty())
{
if (p != NULL)
{
cout << p->get_data() << " ";
if (p->leftchild != NULL)
que.push(p->leftchild);
if (p->rightchild != NULL)
que.push(p->rightchild);
que.pop();
if (!que.empty())
p = que.front();
}
}
cout << endl;
}
int degree1(binarytreenode *p)
{
int count_1 = 0;
binarytreenode *stack[100];
int top = 0;
while (p || top != 0)
{
while (p)
{
stack[top] = p;
top++;
if ((p->leftchild == NULL && p->rightchild != NULL) || (p->rightchild == NULL && p->leftchild != NULL))
{
count_1++;
}
p = p->leftchild;
}
if (top)
{
p = stack[--top];
p = p->rightchild;
}
}
return count_1;
}
int degree2(binarytreenode *p)
{
int count_2 = 0;
binarytreenode *stack[100];
int top = 0;
while (p || top != 0)
{
while (p)
{
stack[top] = p;
top++;
if ((p->leftchild != NULL && p->rightchild != NULL))
{
count_2++;
}
p = p->leftchild;
}
if (top)
{
p = stack[--top];
p = p->rightchild;
}
}
return count_2;
}
int degree0(binarytreenode *p)
{
int count_0 = 0;
binarytreenode *stack[100];
int top = 0;
while (p || top != 0)
{
while (p)
{
stack[top] = p;
top++;
if (p->leftchild == NULL && p->rightchild == NULL)
{
count_0++;
}
p = p->leftchild;
}
if (top)
{
p = stack[--top];
p = p->rightchild;
}
}
return count_0;
}
int get_height(binarytreenode *p)
{
if (p == NULL)
{
return 0;
}
int count_h = 1;
if (p->leftchild == NULL && p->rightchild == NULL)
{
count_h = 1;
}
else
{
count_h = count_h + ((get_height(p->leftchild) < get_height(p->rightchild)) ? get_height(p->rightchild) : get_height(p->leftchild));
}
return count_h;
}
void get_width(binarytreenode *p, int i, int wide[])
{
wide[i++]++;
if (p->leftchild != NULL)
get_width(p->leftchild, i, wide);
if (p->rightchild != NULL)
get_width(p->rightchild, i, wide);
}
int get_max_width()
{
int height = get_height(root);
int wide[height];
for (int i = 0; i < height; i++)
{
wide[i] = 0;
}
get_width(root, 0, wide);
int max = wide[0];
for (int i = 0; i < height; i++)
{
if (wide[i] > max)
{
max = wide[i];
}
}
return max;
}
int get_max(binarytreenode *p)
{
int max = p->get_data();
if (p == NULL)
{
max = 0;
}
while (p->rightchild != NULL)
{
p = p->rightchild;
}
max = p->get_data();
return max;
}
void change_children(binarytreenode *p)
{
if (p->leftchild != NULL)
change_children(p->leftchild);
if (p->rightchild != NULL)
change_children(p->rightchild);
binarytreenode *temp;
temp = p->leftchild;
p->leftchild = p->rightchild;
p->rightchild = temp;
}
int find_father(binarytreenode *num, binarytreenode *&fa)
{
binarytreenode *p = root;
int flag = 0;
while (p != NULL)
{
if (p->get_data() > num->get_data())
{
fa = p;
flag = 1;
p = p->leftchild;
}
else if (p->get_data() <= num->get_data() && p != num)
{
fa = p;
flag = 2;
p = p->rightchild;
}
else
{
break;
}
}
return flag;
}
int stackDel[100];
int top = 0;
void del_leaf(binarytreenode *p)
{
binarytreenode *ptr = p;
if (ptr->leftchild != NULL)
{
if (ptr->leftchild->leftchild == NULL && ptr->leftchild->rightchild == NULL)
{
stackDel[top++] = ptr->leftchild->get_data();
delete ptr->leftchild;
ptr->leftchild = NULL;
}
else
{
del_leaf(ptr->leftchild);
}
}
if (ptr->rightchild != NULL)
{
if (ptr->rightchild->leftchild == NULL && ptr->rightchild->rightchild == NULL)
{
stackDel[top++] = ptr->rightchild->get_data();
delete ptr->rightchild;
ptr->rightchild = NULL;
}
else
{
del_leaf(ptr->rightchild);
}
}
}
bool leaf(binarytreenode *pCur)
{
if (pCur->leftchild == NULL && pCur->rightchild == NULL)
return true;
return false;
}
bool wanquan(binarytreenode *pRoot)
{
int height = get_height(root);
int wide[height];
for (int i = 0; i < height; i++)
{
wide[i] = 0;
}
get_width(root, 0, wide);
for (int i = 0; i < height - 1; i++)
{
if (wide[i] != pow(2, i))
return false;
}
return true;
}
};
int binarytree::times = 0;
int main()
{
binarytree tree;
tree.creat();
tree.levelorder();
cout << "度为1的结点个数: " << tree.degree1(tree.get_root()) << endl;
cout << "度为2的结点个数: " << tree.degree2(tree.get_root()) << endl;
cout << "度为0的结点个数: " << tree.degree0(tree.get_root()) << endl;
cout << "二叉树高度: " << tree.get_height(tree.get_root()) << endl;
cout << "二叉树宽度: " << tree.get_max_width() << endl;
cout << "最大值:" << tree.get_max(tree.get_root()) << endl;
cout << "删除叶节点:" << endl;
tree.del_leaf(tree.get_root());
for (tree.top = tree.top - 1; tree.top >= 0; tree.top--)
{
cout << tree.stackDel[tree.top] << "删除成功!" << endl;
}
tree.levelorder();
cout << "交换左右孩子. . ." << endl;
tree.change_children(tree.get_root());
tree.levelorder();
if (tree.wanquan(tree.get_root()))
cout << "该二叉树是完全二叉树" << endl;
else
cout << "该二叉树不是完全二叉树" << endl;
system("pause");
return 0;
}