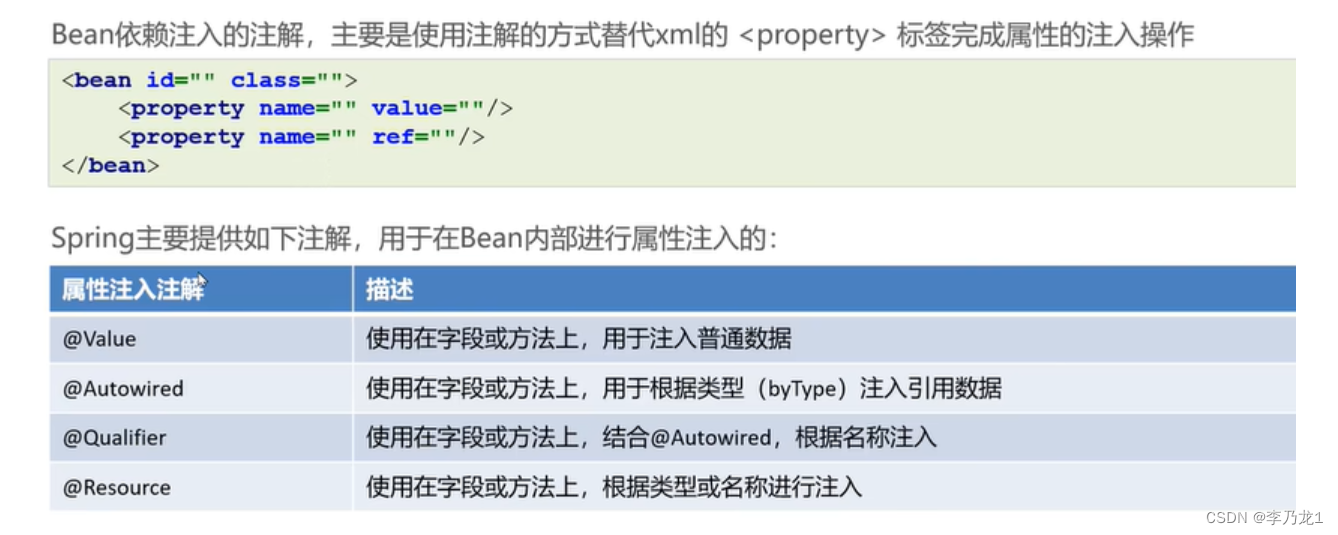
1,@Value
1.1 新建配置文件 constant.properties
con.username=linailong
1.2 修改 applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 开启注解扫描 识别 @Component 通过springbean的后处理器实现-->
<context:component-scan base-package="com.study"/>
<context:property-placeholder location="classpath:constant.properties"/>
</beans>
1.3 修改 UserService
package com.study.service;
public interface UserService {
void showUserName();
}
1.4 修改 UserServiceImpl
package com.study.service.impl;
import com.study.service.UserService;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component("userService")
//@Scope("singleton") //是否是单例 prototype singleton
//@Lazy(value = true)
public class UserServiceImpl implements UserService {
@Value("${con.username}")
private String username;
public UserServiceImpl() {
System.out.println("UserServiceImpl 实例化");
}
public void showUserName() {
System.out.println(username);
}
// @PostConstruct
// public void Construct(){
// System.out.println("Construct--");
// }
//
// @PreDestroy
// public void Destory(){
// System.out.println("Destory -- ");
// }
}
1.5 修改测试类 ApplicationContextDemo01
package com.study.demo;
import com.study.service.UserService;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class ApplicationContextDemo01 {
public static void main(String[] args) {
ClassPathXmlApplicationContext context =
new ClassPathXmlApplicationContext("applicationContext.xml");
UserService userService1 = (UserService)context.getBean("userService");
userService1.showUserName();
}
}

2,@Autowired
2.1 修改 UserService
package com.study.service;
public interface UserService {
void showUserName();
void showUserDao();
}
2.2 修改 UserServiceImpl
package com.study.service.impl;
import com.study.dao.UserDao;
import com.study.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
@Component("userService")
public class UserServiceImpl implements UserService {
@Value("${con.username}")
private String username;
@Autowired
// @Qualifier("userDao")
// @Resource()
private UserDao userDao;
public UserServiceImpl() {
System.out.println("UserServiceImpl 实例化");
}
public void showUserName() {
System.out.println(username);
}
public void showUserDao() {
System.out.println(userDao);
}
}
2.3 修改 ApplicationContextDemo01
package com.study.demo;
import com.study.service.UserService;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class ApplicationContextDemo01 {
public static void main(String[] args) {
ClassPathXmlApplicationContext context =
new ClassPathXmlApplicationContext("applicationContext.xml");
UserService userService1 = (UserService)context.getBean("userService");
userService1.showUserDao();
}
}
